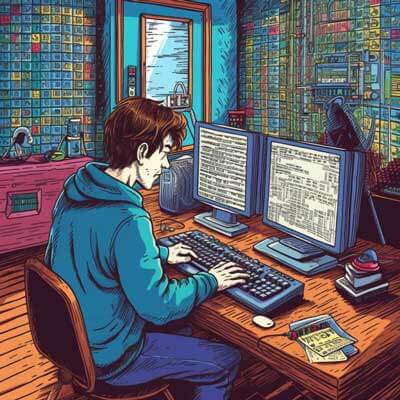
- Applying Aggregate Functions in the WHERE Clause
- Optimizing Queries with Aggregate Functions
- Grouping Data using Aggregate Functions
- Filtering Data with Aggregate Functions
- Limitations and Restrictions of Aggregate Functions in the WHERE Clause
- Improving Query Performance with Aggregate Functions
- Syntax for Using Aggregate Functions in the WHERE Clause
- Combining Multiple Aggregate Functions in the WHERE Clause
- Alternative Approaches to Using Aggregate Functions in the WHERE Clause
- Additional Resources
Aggregate functions in PostgreSQL are used to perform calculations on a set of values and return a single value as the result. These functions are often used in conjunction with the GROUP BY clause to calculate summary statistics for groups of rows.
Here are some common aggregate functions in PostgreSQL:
1. COUNT: This function returns the number of rows in a group or the number of non-null values in a column.
SELECT COUNT(*) FROM employees;
2. SUM: This function calculates the sum of values in a column.
SELECT SUM(salary) FROM employees;
3. AVG: This function calculates the average of values in a column.
SELECT AVG(salary) FROM employees;
4. MAX: This function returns the maximum value in a column.
SELECT MAX(salary) FROM employees;
5. MIN: This function returns the minimum value in a column.
SELECT MIN(salary) FROM employees;
These are just a few examples of the aggregate functions available in PostgreSQL. There are many more functions that can be used to perform various calculations on data.
Applying Aggregate Functions in the WHERE Clause
In PostgreSQL, aggregate functions are typically used in the SELECT clause to calculate summary statistics for groups of rows. However, it is also possible to use aggregate functions in the WHERE clause to filter data based on aggregate calculations.
Let’s consider an example to illustrate this. Suppose we have a table called “sales” with columns “id”, “product”, “quantity”, and “price”. We want to retrieve all the products that have a total sales value greater than 10000. We can use the SUM function in the WHERE clause to accomplish this:
SELECT product, SUM(quantity * price) AS total_sales FROM sales GROUP BY product HAVING SUM(quantity * price) > 10000;
In this example, the SUM function is used in the SELECT clause to calculate the total sales value for each product. The HAVING clause is then used in conjunction with the WHERE clause to filter the rows based on the aggregate calculation.
Related Article: PostgreSQL HyperLogLog (HLL) & Cardinality Estimation
Optimizing Queries with Aggregate Functions
When using aggregate functions in the WHERE clause, it is important to optimize your queries to ensure optimal performance. Here are some tips to consider:
1. Indexing: Make sure you have appropriate indexes on columns used in the WHERE clause and the GROUP BY clause to improve query performance.
2. Use Subqueries: Instead of using aggregate functions directly in the WHERE clause, consider using subqueries to calculate the aggregate values first and then filter the results based on those values.
3. Limit the Result Set: If possible, limit the number of rows returned by the query using the LIMIT clause. This can significantly improve query performance, especially when dealing with large datasets.
4. Use EXPLAIN: Use the EXPLAIN command to analyze the execution plan of your query and identify any performance bottlenecks. This can help you make informed decisions on how to optimize your query.
Grouping Data using Aggregate Functions
Aggregate functions in PostgreSQL are commonly used in conjunction with the GROUP BY clause to group rows based on one or more columns. This allows you to perform calculations on subsets of data rather than the entire dataset.
Let’s consider an example to illustrate this. Suppose we have a table called “orders” with columns “order_id”, “customer_id”, and “order_date”. We want to calculate the total number of orders placed by each customer. We can use the COUNT function in combination with the GROUP BY clause to achieve this:
SELECT customer_id, COUNT(*) AS total_orders FROM orders GROUP BY customer_id;
In this example, the COUNT function is used to calculate the total number of orders for each customer. The result is grouped by the “customer_id” column using the GROUP BY clause.
Filtering Data with Aggregate Functions
Aggregate functions in PostgreSQL can also be used to filter data based on aggregate calculations. This can be achieved using the HAVING clause, which is similar to the WHERE clause but operates on aggregate calculations rather than individual rows.
Let’s consider an example to illustrate this. Suppose we have a table called “products” with columns “product_id”, “category_id”, and “price”. We want to retrieve all the categories that have an average price greater than 100. We can use the AVG function in the HAVING clause to accomplish this:
SELECT category_id, AVG(price) AS average_price FROM products GROUP BY category_id HAVING AVG(price) > 100;
In this example, the AVG function is used to calculate the average price for each category. The HAVING clause is then used to filter the rows based on the aggregate calculation.
Related Article: How to Check if a Table Exists in PostgreSQL
Limitations and Restrictions of Aggregate Functions in the WHERE Clause
While aggregate functions can be useful tools for data analysis, there are some limitations and restrictions when using them in the WHERE clause in PostgreSQL.
1. Aggregate functions cannot be used directly in the WHERE clause: PostgreSQL does not allow aggregate functions to be used directly in the WHERE clause. Instead, you need to use subqueries or the HAVING clause to filter data based on aggregate calculations.
2. Aggregate functions cannot reference individual rows: Aggregate functions operate on groups of rows rather than individual rows. Therefore, they cannot be used to reference individual rows in the WHERE clause.
3. Aggregate functions cannot be used in combination with other non-aggregate functions: PostgreSQL does not allow aggregate functions to be used in combination with other non-aggregate functions in the WHERE clause. If you need to perform calculations on individual rows, you should use subqueries or other techniques.
4. Aggregate functions may have performance implications: Using aggregate functions in the WHERE clause can have performance implications, especially when dealing with large datasets. It is important to optimize your queries and consider the performance implications of using aggregate functions.
Improving Query Performance with Aggregate Functions
When using aggregate functions in the WHERE clause, there are several strategies you can employ to improve query performance:
1. Use appropriate indexes: Ensure that you have appropriate indexes on the columns used in the WHERE clause and the GROUP BY clause to improve query performance.
2. Optimize your query: Analyze the execution plan of your query using the EXPLAIN command and identify any performance bottlenecks. Consider using subqueries or other techniques to optimize your query.
3. Limit the result set: If possible, limit the number of rows returned by the query using the LIMIT clause. This can significantly improve query performance, especially when dealing with large datasets.
4. Cache frequently used aggregate values: If you have frequently used aggregate values, consider caching them in a separate table or using materialized views to improve query performance.
5. Use appropriate hardware and configuration: Ensure that your PostgreSQL server is running on appropriate hardware and that it is properly configured for your workload. This can have a significant impact on query performance.
Syntax for Using Aggregate Functions in the WHERE Clause
To use aggregate functions in the WHERE clause in PostgreSQL, you need to use subqueries or the HAVING clause. Here is the syntax for each approach:
Using subqueries:
SELECT column1, column2, ... FROM table_name WHERE aggregate_function(column) (operator) (value);
Using the HAVING clause:
SELECT column1, column2, ... FROM table_name GROUP BY column HAVING aggregate_function(column) (operator) (value);
In both cases, the aggregate function is used to perform calculations on a column or expression. The result of the aggregate function is then compared to a value using an operator.
Related Article: How to Convert Columns to Rows in PostgreSQL
Combining Multiple Aggregate Functions in the WHERE Clause
It is possible to combine multiple aggregate functions in the WHERE clause in PostgreSQL to perform more complex calculations. This can be achieved using subqueries or the HAVING clause.
Let’s consider an example to illustrate this. Suppose we have a table called “orders” with columns “order_id”, “customer_id”, “order_date”, and “total_amount”. We want to retrieve all the customers who have placed more than 100 orders with a total amount greater than 10000. We can use multiple aggregate functions in the WHERE clause to accomplish this:
Using subqueries:
SELECT customer_id FROM ( SELECT customer_id, COUNT(*) AS total_orders, SUM(total_amount) AS total_amount FROM orders GROUP BY customer_id ) subquery WHERE total_orders > 100 AND total_amount > 10000;
Using the HAVING clause:
SELECT customer_id, COUNT(*) AS total_orders, SUM(total_amount) AS total_amount FROM orders GROUP BY customer_id HAVING COUNT(*) > 100 AND SUM(total_amount) > 10000;
In both cases, multiple aggregate functions are used to calculate the total number of orders and the total amount for each customer. The result is then filtered based on the aggregate calculations using the WHERE clause or the HAVING clause.
Alternative Approaches to Using Aggregate Functions in the WHERE Clause
If you find that using aggregate functions in the WHERE clause is not suitable for your specific use case, there are alternative approaches you can consider:
1. Using subqueries: Instead of using aggregate functions directly in the WHERE clause, you can use subqueries to calculate the aggregate values first and then filter the results based on those values.
2. Using temporary tables: You can calculate the aggregate values using aggregate functions in a temporary table and then join the temporary table with the main table to filter the results based on the aggregate calculations.
3. Using window functions: Window functions in PostgreSQL allow you to perform calculations on a subset of rows without grouping the entire result set. You can use window functions to calculate aggregate values and then filter the results based on those values.
4. Using common table expressions (CTEs): CTEs in PostgreSQL allow you to define a temporary result set that can be referenced multiple times within a query. You can use CTEs to calculate the aggregate values and then filter the results based on those values.
These alternative approaches can provide more flexibility and control over the calculations and filtering process, depending on your specific requirements. It is important to carefully consider the pros and cons of each approach and choose the one that best fits your needs.