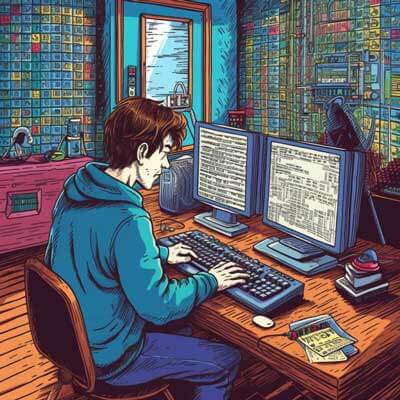
- Syntax for Creating a PostgreSQL Array Literal
- Inserting an Array Literal into a PostgreSQL Database
- Using a PostgreSQL Array Literal in a WHERE Clause
- Advantages of Using Array Literals in PostgreSQL
- Limitations of Using Array Literals in PostgreSQL
- Retrieving Data from a PostgreSQL Array Literal
- Updating or Deleting Specific Elements in a PostgreSQL Array Literal
- Nesting Array Literals in PostgreSQL
- Difference between PostgreSQL Array Literal and Array Column
- Support for Array Literals in Other Databases
- Additional Resources
Syntax for Creating a PostgreSQL Array Literal
In PostgreSQL, an array literal is a way to represent an array value directly in SQL queries or statements. The syntax for creating a PostgreSQL array literal is straightforward. You enclose the elements of the array within curly braces {}
and separate them with commas. Here is an example:
SELECT ARRAY[1, 2, 3, 4, 5];
This query will return the array [1, 2, 3, 4, 5]
. You can use any valid data type for the elements of the array. For example, you can create an array of strings like this:
SELECT ARRAY['apple', 'banana', 'orange'];
This query will return the array ['apple', 'banana', 'orange']
.
You can also create multi-dimensional arrays by nesting the array literals. Here is an example:
SELECT ARRAY[[1, 2], [3, 4], [5, 6]];
This query will return the two-dimensional array [[1, 2], [3, 4], [5, 6]]
.
Related Article: Tutorial: Using isNumeric Function in PostgreSQL
Inserting an Array Literal into a PostgreSQL Database
To insert an array literal into a PostgreSQL database, you can use the INSERT
statement along with the VALUES
clause. Here is an example:
INSERT INTO fruits (name, colors) VALUES ('apple', ARRAY['red', 'green', 'yellow']);
This query will insert a row into the fruits
table with the name ‘apple’ and the colors ['red', 'green', 'yellow']
.
You can also insert multiple rows with array literals using a single INSERT
statement. Here is an example:
INSERT INTO fruits (name, colors) VALUES ('apple', ARRAY['red', 'green', 'yellow']), ('banana', ARRAY['yellow']), ('orange', ARRAY['orange']);
This query will insert three rows into the fruits
table.
Using a PostgreSQL Array Literal in a WHERE Clause
You can use a PostgreSQL array literal in a WHERE
clause to filter rows based on the values in the array. Here is an example:
SELECT name FROM fruits WHERE 'red' = ANY (colors);
This query will return the names of all fruits that have the color ‘red’ in their colors
array.
You can also use array literals in combination with other operators and functions in the WHERE
clause. Here is an example:
SELECT name FROM fruits WHERE array_length(colors, 1) > 2;
This query will return the names of all fruits that have more than two colors in their colors
array.
Advantages of Using Array Literals in PostgreSQL
Using array literals in PostgreSQL offers several advantages:
1. Compact representation: Array literals provide a concise and readable way to represent arrays directly in SQL queries or statements.
2. Simplified data manipulation: With array literals, you can easily insert, update, and delete array values in a database without the need for complex data transformations.
3. Efficient storage and retrieval: PostgreSQL optimizes the storage and retrieval of array values, making them performant for operations such as searching, filtering, and aggregating.
4. Flexibility in data modeling: Array literals allow you to model data with variable-length arrays, which can be useful in scenarios where the number of elements in an array may vary.
5. Compatibility with other PostgreSQL features: Array literals seamlessly integrate with other PostgreSQL features like array functions, array operators, and array indexing, providing a useful toolset for working with array data.
Related Article: How to Use the ISNULL Function in PostgreSQL
Limitations of Using Array Literals in PostgreSQL
While array literals in PostgreSQL offer many benefits, they also have some limitations:
1. Lack of type safety: Array literals do not enforce type constraints on the elements of the array. It is possible to insert values of different data types into the same array column, which can lead to data integrity issues.
2. Limited indexing options: PostgreSQL supports indexing on array columns, but it has limitations when it comes to indexing individual elements within an array. Array literals cannot be used to take advantage of these indexing capabilities.
3. Potential performance impact: Working with large arrays or performing complex operations on array literals can have a performance impact. It is important to carefully consider the size and complexity of array operations to avoid performance bottlenecks.
Retrieving Data from a PostgreSQL Array Literal
To retrieve data from a PostgreSQL array literal, you can use the SELECT
statement along with the appropriate column name. Here is an example:
SELECT colors FROM fruits;
This query will return the colors
array from all rows in the fruits
table.
You can also use array functions to extract specific elements or perform operations on the array literal. Here is an example:
SELECT colors[1] FROM fruits;
This query will return the first element of the colors
array from all rows in the fruits
table.
Updating or Deleting Specific Elements in a PostgreSQL Array Literal
To update or delete specific elements in a PostgreSQL array literal, you can use the array manipulation functions provided by PostgreSQL. Here is an example:
UPDATE fruits SET colors = array_remove(colors, 'red') WHERE 'red' = ANY (colors);
This query will remove the color ‘red’ from the colors
array of all fruits that have it.
You can also use array functions to update or delete specific elements based on their position in the array. Here is an example:
UPDATE fruits SET colors[1] = 'blue' WHERE 'red' = ANY (colors);
This query will replace the first element of the colors
array with ‘blue’ for all fruits that have ‘red’ in their colors
array.
Related Article: Integrating PostgreSQL While Loop into Database Operations
Nesting Array Literals in PostgreSQL
PostgreSQL allows you to nest array literals to create multi-dimensional arrays. Here is an example:
SELECT ARRAY[[1, 2], [3, 4], [5, 6]];
This query will return the two-dimensional array [[1, 2], [3, 4], [5, 6]]
.
You can nest array literals to any depth, creating arrays of arrays. Here is an example:
SELECT ARRAY[[1, 2], ARRAY['a', 'b', 'c'], ARRAY[TRUE, FALSE]];
This query will return the three-dimensional array [[1, 2], ['a', 'b', 'c'], [TRUE, FALSE]]
.
Difference between PostgreSQL Array Literal and Array Column
In PostgreSQL, an array literal is a way to represent an array value directly in SQL queries or statements. It is a temporary and immediate representation of an array.
On the other hand, an array column is a column type that can store array values in a PostgreSQL table. It provides a persistent and structured way to store and retrieve array data.
The main difference between a PostgreSQL array literal and an array column is their purpose and scope. Array literals are used in SQL queries or statements to represent array values temporarily, while array columns are used to store array values persistently in a database table.
Support for Array Literals in Other Databases
Array literals are a feature specific to PostgreSQL and may not be supported in other databases. However, many databases provide similar functionality for working with arrays.
For example, in MySQL, you can use the FIND_IN_SET
function to search for a value within a comma-separated list. In Oracle, you can use the TABLE
function to convert a comma-separated list into a table.
It is important to consult the documentation of the specific database you are using to understand its support for array-like structures and how to work with them effectively.
Related Article: Tutorial: Modulo Operator in PostgreSQL Databases