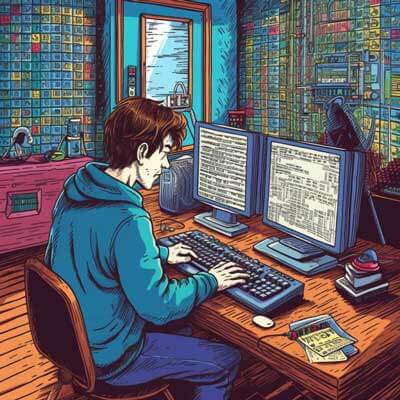
- Syntax for Updating a JSONB Field in PostgreSQL
- Setting a New Value in a JSONB Column in PostgreSQL
- Updating an Array Inside a JSONB Column in PostgreSQL
- Example of Updating a JSONB Column in PostgreSQL
- Best Practices for Updating a JSONB Field in PostgreSQL
- Limitations and Performance Concerns when Updating JSONB Data in PostgreSQL
- Updating a Specific Key-Value Pair in a JSONB Field in PostgreSQL
- Difference Between Updating a JSONB Column and Updating a Regular Column in PostgreSQL
- Updating Multiple JSONB Fields at Once in PostgreSQL
In PostgreSQL, the JSONB data type allows you to store and manipulate JSON data efficiently. JSONB columns can store JSON objects, arrays, or scalar values. Updating a JSONB column involves modifying the existing JSON data to add, modify, or remove values.
To update a JSONB column in PostgreSQL, you can use the UPDATE
statement with the SET
clause. The SET
clause allows you to specify the new value for the JSONB column. Additionally, you can use the ->
and ->>
operators to navigate and modify specific elements within the JSONB data.
Let’s explore the syntax for updating a JSONB field in PostgreSQL.
Syntax for Updating a JSONB Field in PostgreSQL
The syntax for updating a JSONB field in PostgreSQL is as follows:
UPDATE table_name SET column_name = new_value WHERE condition;
In this syntax:
– table_name
is the name of the table where the JSONB column is located.
– column_name
is the name of the JSONB column to be updated.
– new_value
is the new value that will replace the existing JSONB data.
– condition
is an optional clause that specifies which rows should be updated.
To update a JSONB field, you can also use the jsonb_set
function. The jsonb_set
function allows you to modify specific elements within the JSONB data by providing a path and a new value.
The syntax for using the jsonb_set
function to update a JSONB field is as follows:
UPDATE table_name SET column_name = jsonb_set(column_name, path, new_value) WHERE condition;
In this syntax:
– table_name
is the name of the table where the JSONB column is located.
– column_name
is the name of the JSONB column to be updated.
– path
is the path to the element within the JSONB data that you want to update.
– new_value
is the new value that will replace the existing value at the specified path.
– condition
is an optional clause that specifies which rows should be updated.
Now, let’s see how to set a new value in a JSONB column in PostgreSQL.
Related Article: How to Export a PostgreSQL Query to CSV
Setting a New Value in a JSONB Column in PostgreSQL
To set a new value in a JSONB column in PostgreSQL, you can use the ->
or ->>
operators to navigate to the specific element within the JSONB data and assign a new value to it.
Here’s an example that demonstrates how to set a new value in a JSONB column:
UPDATE employees SET data = data || '{"salary": 5000}' WHERE id = 1;
In this example, we have a table called employees
with a JSONB column named data
. We want to set a new value for the salary
key within the JSONB data. The ||
operator is used to concatenate the existing JSONB data with the new JSON object that contains the updated salary value.
Now, let’s look at how to update an array inside a JSONB column in PostgreSQL.
Updating an Array Inside a JSONB Column in PostgreSQL
To update an array inside a JSONB column in PostgreSQL, you can use the jsonb_set
function with the ||
operator.
Here’s an example that demonstrates how to update an array inside a JSONB column:
UPDATE employees SET data = jsonb_set(data, '{skills}', data->'skills' || '["Python", "Java"]') WHERE id = 1;
In this example, we have a table called employees
with a JSONB column named data
. The data
column contains a JSON object with an array of skills. We want to update the array by adding two new skills: “Python” and “Java”.
The jsonb_set
function is used to update the skills
array by concatenating the existing array with the new array using the ||
operator.
Next, let’s see an example of updating a JSONB column in PostgreSQL.
Example of Updating a JSONB Column in PostgreSQL
Suppose we have a table called products
with the following schema:
CREATE TABLE products ( id SERIAL <a href="https://www.squash.io/exploring-sql-join-conditions-the-role-of-primary-keys/">PRIMARY KEY</a>, name TEXT, attributes JSONB );
The products
table has three columns: id
, name
, and attributes
. The attributes
column is of type JSONB and stores additional information about each product.
Let’s say we want to update the attributes
column for a specific product with a new value. We can use the following SQL statement:
UPDATE products SET attributes = jsonb_set(attributes, '{color}', '"red"') WHERE id = 1;
In this example, we are updating the attributes
column for the product with an id
of 1. We use the jsonb_set
function to set the value of the color
key to "red"
.
Now, let’s discuss some best practices for updating a JSONB field in PostgreSQL.
Related Article: Processing Array_Agg Data in JSON Format in PostgreSQL
Best Practices for Updating a JSONB Field in PostgreSQL
When updating a JSONB field in PostgreSQL, it is important to follow some best practices to ensure efficient and effective updates:
1. Use the jsonb_set
function: The jsonb_set
function provides a flexible and useful way to update specific elements within a JSONB column. It allows you to specify a path and a new value, making it easy to update nested elements.
2. Use indexes wisely: If you frequently update a JSONB field, consider adding indexes to improve query performance. PostgreSQL allows you to create indexes on specific elements within a JSONB column using the jsonb_path_ops
operator class.
3. Consider using triggers: If you have complex logic for updating a JSONB field, consider using triggers. Triggers allow you to define custom actions that are automatically executed before or after an update operation.
4. Be mindful of performance: Updating a JSONB field can be slower compared to updating regular columns. This is because the entire JSONB value needs to be rewritten when a modification is made. Keep this in mind when designing your database schema and consider using JSONB fields only when necessary.
Now, let’s discuss the limitations and performance concerns when updating JSONB data in PostgreSQL.
Limitations and Performance Concerns when Updating JSONB Data in PostgreSQL
Updating JSONB data in PostgreSQL comes with a few limitations and performance concerns that you should be aware of:
1. Performance impact: Updating a JSONB column can be slower compared to updating regular columns, especially when the JSONB data is large. This is because the entire JSONB value needs to be rewritten when a modification is made.
2. Indexing limitations: PostgreSQL allows you to create indexes on specific elements within a JSONB column using the jsonb_path_ops
operator class. However, these indexes have limitations. They are not suitable for all types of queries and may not be as efficient as regular indexes.
3. Lack of strict schema enforcement: JSONB columns do not enforce a strict schema, which means that you can insert data with different structures into the same column. While this flexibility can be useful in some cases, it can also lead to data inconsistencies and make it harder to enforce data integrity.
4. Limited query capabilities: While PostgreSQL provides useful functions and operators for querying JSONB data, the query capabilities are still limited compared to a dedicated NoSQL database. If you have complex querying requirements, consider using a dedicated NoSQL solution instead.
Despite these limitations and performance concerns, PostgreSQL’s JSONB data type provides a flexible and efficient way to store and update JSON data within a relational database.
Next, let’s explore how to update a specific key-value pair in a JSONB field in PostgreSQL.
Updating a Specific Key-Value Pair in a JSONB Field in PostgreSQL
To update a specific key-value pair in a JSONB field in PostgreSQL, you can use the jsonb_set
function with the ||
operator.
Here’s an example that demonstrates how to update a specific key-value pair in a JSONB field:
UPDATE products SET attributes = jsonb_set(attributes, '{price}', '19.99') WHERE id = 1;
In this example, we are updating the attributes
column for the product with an id
of 1. We use the jsonb_set
function to set the value of the price
key to '19.99'
.
Now, let’s compare updating a JSONB column and updating a regular column in PostgreSQL.
Difference Between Updating a JSONB Column and Updating a Regular Column in PostgreSQL
Updating a JSONB column and updating a regular column in PostgreSQL are two different operations with their own considerations.
When updating a JSONB column, the entire JSONB value needs to be rewritten when a modification is made. This can be slower compared to updating a regular column, especially when the JSONB data is large. Additionally, updating specific elements within a JSONB column requires the use of functions like jsonb_set
or operators like ->
and ->>
.
On the other hand, updating a regular column is a straightforward operation. You can simply update the value of the column using the SET
clause in the UPDATE
statement. There are no additional considerations or functions needed to update specific elements within the column.
In terms of querying, JSONB columns provide more flexibility and querying capabilities compared to regular columns. PostgreSQL provides a rich set of functions and operators for querying JSONB data, allowing you to perform complex queries on the JSONB column.
Now, let’s explore how to update multiple JSONB fields at once in PostgreSQL.
Updating Multiple JSONB Fields at Once in PostgreSQL
To update multiple JSONB fields at once in PostgreSQL, you can use the jsonb_set
function multiple times within the UPDATE
statement.
Here’s an example that demonstrates how to update multiple JSONB fields at once:
UPDATE products SET attributes = jsonb_set(jsonb_set(attributes, '{price}', '19.99'), '{color}', '"red"') WHERE id = 1;
In this example, we are updating the attributes
column for the product with an id
of 1. We use the jsonb_set
function twice, first to update the price
key to '19.99'
and then to update the color
key to "red"
.