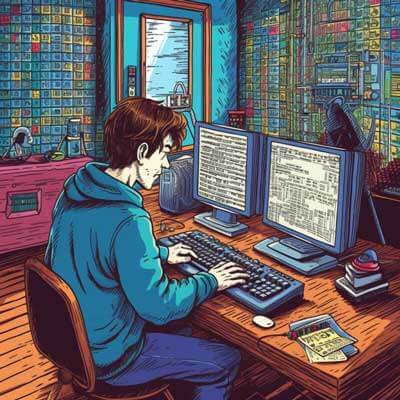
Table of Contents
PostgreSQL provides several built-in functions to calculate the length of a string. The most commonly used function is the length()
function, which returns the number of characters in a given string. Let's see an example:
SELECT length('Hello, World!') AS string_length;
The above query will return the length of the string "Hello, World!", which is 13. Note that the length function counts the number of characters, including spaces and special characters.
The Length Function
The length()
function in PostgreSQL is used to calculate the length of a string. It takes a string as an argument and returns the number of characters in that string. Let's consider another example:
SELECT length('12345') AS string_length;
In the above query, the length function will return the length of the string "12345", which is 5. The length function can also be used on columns in a table. We will explore this in more detail in the next section.
Related Article: How to Compare & Manipulate Dates in PostgreSQL
Calculating Character Length
In addition to the length()
function, PostgreSQL also provides the char_length()
function to calculate the number of characters in a string. The char_length()
function is similar to the length()
function, but it counts the number of characters instead of bytes. This is particularly useful when dealing with multibyte character encodings like UTF-8. Let's see an example:
SELECT char_length('こんにちは') AS character_length;
The above query will return the character length of the string "こんにちは", which is 5. Note that each character in this string is represented by 3 bytes in UTF-8 encoding.
The Char Length Function
The char_length()
function in PostgreSQL is used to calculate the number of characters in a string. It is similar to the length()
function, but it counts the number of characters instead of bytes. Let's consider another example:
SELECT char_length('Γειά σου') AS character_length;
In the above query, the char_length function will return the character length of the string "Γειά σου", which is 8. This is because each character in this string is represented by 2 bytes in UTF-8 encoding.
Getting the String Length in a PostgreSQL Table
To get the length of a string stored in a PostgreSQL table, you can use the length()
function along with a SELECT statement. Let's consider an example where we have a table called "users" with a column named "name":
CREATE TABLE users ( id serial <a href="https://www.squash.io/exploring-sql-join-conditions-the-role-of-primary-keys/">PRIMARY KEY</a>, name varchar(100) ); INSERT INTO users (name) VALUES ('John Doe'); INSERT INTO users (name) VALUES ('Jane Smith');
To retrieve the length of the names stored in the "users" table, we can use the following query:
SELECT name, length(name) AS name_length FROM users;
The above query will return the names along with their corresponding lengths. For example, it might return:
| name | name_length | --------------------------- | John Doe | 8 | | Jane Smith | 10 |
This allows you to easily retrieve the length of strings stored in a PostgreSQL table.
Related Article: How to Fix MySQL Error Code 1175 in Safe Update Mode
Using the Length Function on Text Data Types
In PostgreSQL, you can use the length()
function on text data types as well. Text data types are used to store strings of variable length. Let's consider an example where we have a table called "products" with a column named "description" of type text:
CREATE TABLE products ( id serial PRIMARY KEY, description text ); INSERT INTO products (description) VALUES ('This is a product'); INSERT INTO products (description) VALUES ('Another product');
To retrieve the length of the descriptions stored in the "products" table, we can use the following query:
SELECT description, length(description) AS description_length FROM products;
The above query will return the descriptions along with their corresponding lengths. For example, it might return:
| description | description_length | ----------------------------------------- | This is a product | 16 | | Another product | 15 |
This demonstrates that the length()
function can be used on text data types in PostgreSQL.
Finding String Length without Spaces in PostgreSQL
Sometimes, you may want to find the length of a string without counting the spaces. PostgreSQL provides the length()
function along with the replace()
function to achieve this. Let's consider an example:
SELECT length(replace('Hello, World!', ' ', '')) AS string_length_without_spaces;
The above query will return the length of the string "Hello, World!" without counting the spaces, which is 11. The replace()
function is used to replace spaces with an empty string, effectively removing them before calculating the length.
Getting the Length of a String in a Specific Encoding
In PostgreSQL, you can also get the length of a string in a specific encoding using the pg_encoding_len()
function. This function takes two arguments: the name of the encoding and the string itself. Let's see an example:
SELECT pg_encoding_len('UTF8', 'こんにちは') AS string_length_in_encoding;
The above query will return the length of the string "こんにちは" in the UTF-8 encoding, which is 15. Note that the length is counted in bytes, not characters.
Exploring the Maximum Length of a String in PostgreSQL
PostgreSQL has a maximum length for strings, which is determined by the data type used to store the string. The maximum length varies depending on the data type. Let's consider a few examples:
- The varchar
data type has a maximum length of 65535 bytes.
- The text
data type has no maximum length.
To find the maximum length of a string in PostgreSQL, you can use the pg_column_size()
function, which returns the size in bytes of a field's internal representation. Let's see an example:
SELECT pg_column_size('Hello, World!') AS max_string_length;
The above query will return the maximum length of the string "Hello, World!" in bytes. Note that this is not the same as the number of characters or the number of bytes used to store the string.
Related Article: Positioning WHERE Clause After JOINs in SQL Databases
Limitations on String Length in PostgreSQL
While PostgreSQL provides various functions to determine the length of a string, it is important to be aware of the limitations on string length. As mentioned earlier, the maximum length of a string is determined by the data type used to store the string. It is also worth noting that PostgreSQL has a maximum row size limit, which includes the combined size of all columns in a table. If the combined size exceeds this limit, you may encounter errors or performance issues.
Additionally, when using multibyte character encodings like UTF-8, it is important to consider that the length of a string may not always correspond to the number of characters. Some characters may require multiple bytes to store, which can affect the length calculation.
Additional Resources
- PostgreSQL: Documentation: 9.6: String Functions and Operators