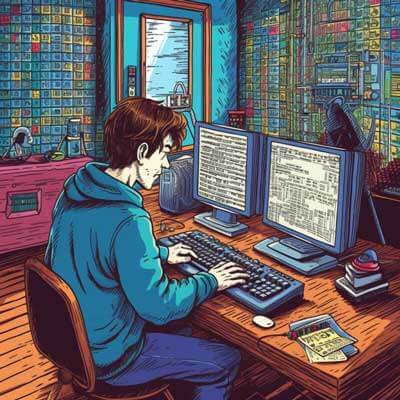
Table of Contents
Purpose of the isNumeric Function
The isNumeric function in PostgreSQL is a useful tool that allows users to determine whether a given value is numeric or not. It returns a Boolean value of true or false, depending on the validity of the input. This function is particularly useful when dealing with user input or data from external sources, where it is important to ensure that the data being processed is of the correct numeric type.
To understand the purpose of the isNumeric function, let's consider an example scenario. Suppose you have a web application that takes user input for age, and you want to validate whether the input is a valid numeric value or not. Using the isNumeric function, you can easily check if the input is a number or not before storing it in the database or performing any calculations on it.
Here is an example of how you can use the isNumeric function in PostgreSQL:
SELECT '123'::text, isNumeric('123')
In this example, the isNumeric function is used to check if the value '123' is numeric. The function returns true, indicating that '123' is indeed a valid numeric value.
Another example can be validating a phone number entered by a user. Let's say you have a table called users
with a column named phone_number
of type character varying. You can use the isNumeric function to ensure that the phone number entered by a user contains only numeric digits:
SELECT phone_number FROM users WHERE isNumeric(phone_number)
In this example, the query selects the phone_number
column from the users
table, but only for rows where the phone_number
is numeric. This can be useful when you want to filter out any non-numeric phone numbers from your result set.
Overall, the isNumeric function serves the purpose of validating numeric data in PostgreSQL, ensuring data integrity and avoiding potential errors or inconsistencies in your database.
Related Article: Storing Select Query Results in Variables in PostgreSQL
Handling Numeric Data Types in PostgreSQL
PostgreSQL provides a variety of numeric data types to handle different kinds of numerical data. These data types include integer, decimal, floating-point, and more. Each data type has its own range of values and precision, allowing you to choose the most appropriate type for your specific use case.
Let's take a look at some of the commonly used numeric data types in PostgreSQL:
- Integer: The integer data type is used to store whole numbers without decimal places. It has a range of -2147483648 to 2147483647.
CREATE TABLE employees ( employee_id serial PRIMARY KEY, age integer );
In this example, the age
column is defined as an integer data type to store the age of employees.
- Decimal: The decimal data type is used to store numbers with a fixed number of decimal places. It is commonly used for financial calculations or any situation where precision is important.
CREATE TABLE products ( product_id serial PRIMARY KEY, price decimal(10, 2) );
In this example, the price
column is defined as a decimal data type with a precision of 10 and a scale of 2, allowing for a maximum of 10 digits with 2 decimal places.
- Floating-point: The floating-point data types, such as float and real, are used to store approximate numeric values. They are suitable for scientific calculations or situations where precision is not critical.
CREATE TABLE measurements ( measurement_id serial PRIMARY KEY, temperature float );
In this example, the temperature
column is defined as a float data type to store temperature measurements.
PostgreSQL also provides additional numeric data types, such as numeric, serial, and money, which offer more flexibility and functionality for specific use cases.
When handling numeric data in PostgreSQL, it is important to choose the appropriate data type based on the range of values and precision needed. This ensures accurate calculations, efficient storage, and proper data representation in your database.
Database Management Systems Beyond PostgreSQL
While PostgreSQL is a useful and feature-rich database management system (DBMS), there are several other popular DBMS options available in the market. Each DBMS has its own strengths, weaknesses, and unique features that cater to different use cases and requirements.
Here are a few other DBMS options beyond PostgreSQL:
- MySQL: MySQL is an open-source relational database management system that is widely used for web applications. It offers excellent performance, scalability, and ease of use. MySQL is known for its speed, reliability, and compatibility with various operating systems.
- Oracle Database: Oracle Database is a commercial relational database management system that is widely used in enterprise environments. It offers advanced features, high scalability, and robust security options. Oracle Database is known for its reliability, performance, and support for large-scale applications.
- Microsoft SQL Server: Microsoft SQL Server is a relational database management system developed by Microsoft. It is widely used in Windows-based environments and offers excellent integration with Microsoft tools and technologies. SQL Server provides advanced features, scalability, and strong security options.
- MongoDB: MongoDB is a popular NoSQL database management system that is designed for handling unstructured and semi-structured data. It offers high scalability, flexible data models, and seamless horizontal scaling. MongoDB is known for its ease of use, agility, and support for large-scale distributed systems.
These are just a few examples of the many DBMS options available in the market. The choice of DBMS depends on various factors such as the nature of the application, scalability requirements, performance needs, and budget constraints.
When selecting a DBMS, it is important to consider factors such as data consistency, availability, security, scalability, and ease of use. Additionally, compatibility with existing systems, community support, and vendor reputation should also be taken into account.
Validating Numeric Data in PostgreSQL
Validating numeric data is a crucial step in ensuring data integrity and avoiding errors in your PostgreSQL database. The isNumeric function in PostgreSQL provides a convenient way to validate whether a given value is numeric or not.
Here are a few examples of how you can use the isNumeric function to validate numeric data in PostgreSQL:
Example 1: Validating an Integer
SELECT isNumeric('123') AS is_integer;
In this example, the isNumeric function is used to check if the value '123' is numeric. The function returns true, indicating that '123' is a valid integer.
Example 2: Validating a Decimal
SELECT isNumeric('12.34') AS is_decimal;
In this example, the isNumeric function is used to check if the value '12.34' is numeric. The function returns true, indicating that '12.34' is a valid decimal number.
Example 3: Validating a Non-Numeric Value
SELECT isNumeric('abc') AS is_numeric;
In this example, the isNumeric function is used to check if the value 'abc' is numeric. The function returns false, indicating that 'abc' is not a valid numeric value.
Related Article: Detecting and Resolving Deadlocks in PostgreSQL Databases
The Role of SQL in Database Operations
Structured Query Language (SQL) plays a vital role in performing various database operations, including data retrieval, manipulation, and management. SQL is a standard language for interacting with relational database management systems (RDBMS) like PostgreSQL.
Here are some of the key roles of SQL in database operations:
- Data Retrieval: SQL allows you to retrieve data from a database using the SELECT statement. You can specify the columns to retrieve, apply filters using WHERE clauses, join multiple tables, aggregate data using functions like COUNT and SUM, and sort the result set.
SELECT first_name, last_name FROM employees WHERE department = 'IT' ORDER BY last_name ASC;
In this example, the SQL query retrieves the first name and last name of employees from the 'employees' table, filtering only those in the 'IT' department, and sorting the result set by last name in ascending order.
- Data Manipulation: SQL enables you to modify data in a database using statements like INSERT, UPDATE, and DELETE. You can insert new records, update existing records, or delete records based on specific conditions.
INSERT INTO employees (first_name, last_name, department) VALUES ('John', 'Doe', 'IT'); UPDATE employees SET department = 'HR' WHERE last_name = 'Smith'; DELETE FROM employees WHERE department = 'Sales';
In these examples, SQL statements are used to insert a new employee record, update the department of an employee, and delete employees from the 'employees' table.
- Data Definition: SQL allows you to define and modify the structure of a database using statements like CREATE, ALTER, and DROP. You can create tables, modify table structures, add or remove columns, define constraints, and create indexes.
CREATE TABLE employees ( employee_id serial PRIMARY KEY, first_name varchar(50), last_name varchar(50), department varchar(50) ); ALTER TABLE employees ADD COLUMN email varchar(100); DROP TABLE employees;
In these examples, SQL statements are used to create a new 'employees' table, add a new column to the table, and drop the 'employees' table.
- Data Control: SQL allows you to control access to data and enforce security measures using statements like GRANT and REVOKE. You can grant or revoke privileges to users or roles, specify access permissions on tables or columns, and manage user roles and permissions.
GRANT SELECT, INSERT, UPDATE, DELETE ON employees TO user1; REVOKE INSERT ON employees FROM user2;
In these examples, SQL statements are used to grant SELECT, INSERT, UPDATE, and DELETE privileges to 'user1' on the 'employees' table and revoke the INSERT privilege from 'user2'.
- Data Manipulation Language (DML): SQL provides a set of statements known as DML statements, which include SELECT, INSERT, UPDATE, and DELETE. These statements allow you to manipulate data within a database.
- Data Definition Language (DDL): SQL provides a set of statements known as DDL statements, which include CREATE, ALTER, and DROP. These statements allow you to define and modify the structure of a database.
SQL serves as a useful and flexible language for interacting with databases, allowing developers and database administrators to perform a wide range of operations, from simple data retrieval to complex data manipulation and management tasks.
Alternative Terms for PostgreSQL
PostgreSQL is a popular and widely-used open-source relational database management system (RDBMS). While "PostgreSQL" is its official name, there are also alternative terms or abbreviations that are commonly used to refer to this database system.
Here are a few alternative terms for PostgreSQL:
- Postgres: "Postgres" is a commonly used abbreviation for PostgreSQL. It is derived from the original name of the database system, "Postgres," which was later expanded to "PostgreSQL" to emphasize its SQL compatibility.
- PG: "PG" is another abbreviation often used to refer to PostgreSQL. It is derived from the initials of the original name, "Postgres."
- PSQL: "PSQL" is an abbreviation commonly used to refer to the PostgreSQL interactive terminal. It stands for "PostgreSQL SQL" and is used as a command-line tool to interact with a PostgreSQL database.
- PostgresSQL: "PostgresSQL" is an alternative spelling of "PostgreSQL" that is sometimes used interchangeably. It retains the original "Postgres" name and appends "SQL" to indicate its compatibility with the SQL standard.
These alternative terms are often used in various contexts, including documentation, discussions, and informal conversations. They offer shorter and more convenient ways to refer to PostgreSQL, while still maintaining the essence and recognition of the full name.
Advantages of Using PostgreSQL as a Database System
PostgreSQL is a useful and feature-rich open-source relational database management system (RDBMS) that offers numerous advantages for developers and organizations. Here are some of the key advantages of using PostgreSQL as a database system:
1. Open Source: PostgreSQL is an open-source database system, which means it is freely available and can be modified and customized according to specific requirements. The open-source nature allows for community contributions, continuous improvement, and a vibrant ecosystem of extensions and plugins.
2. Advanced Features: PostgreSQL offers a wide range of advanced features, including support for complex queries, full-text search, spatial data, JSON and XML data types, and advanced indexing options. It also provides support for ACID (Atomicity, Consistency, Isolation, Durability) properties, which ensure data integrity and reliability.
3. Scalability and Performance: PostgreSQL is designed to handle high volumes of data and concurrent users. It provides various mechanisms for scaling, such as table partitioning, parallel query execution, and support for advanced indexing techniques. PostgreSQL's query optimizer and efficient execution engine contribute to its excellent performance.
4. Extensibility: PostgreSQL offers extensibility through user-defined data types, functions, and operators. This allows developers to define custom data types and implement complex business logic within the database. Additionally, PostgreSQL supports multiple programming languages for writing stored procedures and triggers, including SQL, PL/pgSQL, Python, and more.
5. Cross-Platform Compatibility: PostgreSQL is compatible with various operating systems, including Linux, macOS, and Windows. This cross-platform compatibility allows developers to deploy PostgreSQL on their preferred operating system and seamlessly migrate databases between different environments.
6. Robustness and Reliability: PostgreSQL is known for its robustness and reliability. It provides features like point-in-time recovery, online backups, and replication options to ensure data availability and minimize downtime. PostgreSQL's handling of concurrent transactions and its crash recovery mechanisms contribute to its high reliability.
7. Community Support: PostgreSQL has a large and active community of developers, contributors, and users. The community provides extensive documentation, forums, mailing lists, and online resources to help users troubleshoot issues, share knowledge, and stay up to date with the latest developments in the PostgreSQL ecosystem.
8. Security: PostgreSQL offers robust security features, including role-based access control, SSL encryption, row-level security, and data encryption options. It also provides support for auditing and monitoring to track and analyze database activity. PostgreSQL's emphasis on security makes it suitable for handling sensitive and critical data.
9. SQL Compliance: PostgreSQL is highly compliant with the SQL standard, supporting a wide range of SQL features and syntax. This compliance ensures compatibility with existing SQL-based applications and allows for easy migration from other database systems.
10. Cost-Effective: As an open-source database system, PostgreSQL is a cost-effective choice for organizations. It eliminates the need for expensive licensing fees and provides flexibility in terms of hardware and infrastructure choices. The cost savings associated with PostgreSQL make it an attractive option for startups, small businesses, and organizations with budget constraints.
These advantages make PostgreSQL a popular choice for a wide range of applications, from small-scale projects to large enterprise systems. Its robustness, extensibility, performance, and cost-effectiveness contribute to its growing popularity in the software development community.
Ensuring Data Integrity in a PostgreSQL Database
Data integrity is a critical aspect of any database system, ensuring that data is accurate, consistent, and reliable. PostgreSQL provides various mechanisms and features to ensure data integrity, allowing developers and administrators to enforce rules and constraints on the data stored in the database.
Here are some of the ways to ensure data integrity in a PostgreSQL database:
1. Primary Key Constraints: A primary key constraint ensures that each row in a table has a unique identifier. It prevents duplicate entries and enforces the integrity of the data. In PostgreSQL, you can define a primary key constraint when creating a table or alter an existing table to add a primary key constraint.
CREATE TABLE employees ( employee_id serial PRIMARY KEY, first_name varchar(50), last_name varchar(50) );
In this example, the employee_id
column is defined as a primary key, ensuring that each employee has a unique identifier.
2. Foreign Key Constraints: A foreign key constraint establishes a relationship between two tables based on a common column. It ensures that the values in the foreign key column match the values in the referenced primary key column. This maintains the integrity of the data and enforces referential integrity.
CREATE TABLE departments ( department_id serial PRIMARY KEY, department_name varchar(50) ); CREATE TABLE employees ( employee_id serial PRIMARY KEY, first_name varchar(50), last_name varchar(50), department_id integer REFERENCES departments(department_id) );
In this example, the department_id
column in the employees
table is defined as a foreign key that references the department_id
column in the departments
table. This ensures that each employee is associated with a valid department.
3. Check Constraints: Check constraints allow you to specify conditions that must be true for the data in a column. They enforce data integrity by ensuring that only valid values are stored in the column.
CREATE TABLE employees ( employee_id serial PRIMARY KEY, first_name varchar(50), last_name varchar(50), age integer CHECK (age >= 18) );
In this example, the age
column is defined with a check constraint that ensures the age is greater than or equal to 18. This prevents the storage of invalid age values in the employees
table.
4. Unique Constraints: Unique constraints ensure that the values in a column or a group of columns are unique across the table. They prevent duplicate entries and help maintain data integrity.
CREATE TABLE employees ( employee_id serial PRIMARY KEY, email varchar(100) UNIQUE, phone_number varchar(20) UNIQUE );
In this example, the email
and phone_number
columns are defined as unique constraints, ensuring that no two employees have the same email or phone number.
5. Triggers: Triggers are database objects that are automatically executed in response to specific events, such as INSERT, UPDATE, or DELETE operations. Triggers can be used to enforce complex business rules and ensure data integrity.
CREATE OR REPLACE FUNCTION update_last_updated() RETURNS TRIGGER AS $$ BEGIN NEW.last_updated = NOW(); RETURN NEW; END; $$ LANGUAGE plpgsql; CREATE TRIGGER update_last_updated_trigger BEFORE UPDATE ON employees FOR EACH ROW EXECUTE FUNCTION update_last_updated();
In this example, a trigger is created to update the last_updated
column of the employees
table with the current timestamp whenever an update operation is performed on the table. This ensures that the last_updated
column is always up to date.
These are just a few examples of how you can ensure data integrity in a PostgreSQL database. By utilizing primary key constraints, foreign key constraints, check constraints, unique constraints, and triggers, you can enforce rules and constraints on the data, preventing inconsistencies and maintaining the integrity of your database.
Related Article: How to Use the ISNULL Function in PostgreSQL
Using the isNumeric Function in Other Database Systems
While the isNumeric function is specific to PostgreSQL, other database systems offer similar functionality to validate numeric data. Let's explore some alternative approaches in different database systems:
MySQL:
In MySQL, you can use the REGEXP function to validate numeric data. The REGEXP function allows you to match a string against a regular expression pattern. You can define a regular expression pattern to check if a string is numeric or not.
SELECT '123' REGEXP '^[0-9]+$' AS is_numeric;
In this example, the REGEXP function is used to check if the value '123' is numeric in MySQL. The function returns true if the value matches the regular expression pattern, which checks if the string contains only numeric digits.
Oracle Database:
In Oracle Database, you can use the REGEXP_LIKE function to validate numeric data. The REGEXP_LIKE function allows you to check if a string matches a regular expression pattern.
SELECT CASE WHEN REGEXP_LIKE('123', '^[0-9]+$') THEN 1 ELSE 0 END AS is_numeric FROM dual;
In this example, the REGEXP_LIKE function is used to check if the value '123' is numeric in Oracle Database. The function returns 1 if the value matches the regular expression pattern, which checks if the string contains only numeric digits.
Microsoft SQL Server:
In Microsoft SQL Server, you can use the ISNUMERIC function to check if a value is numeric. The ISNUMERIC function returns 1 if the value can be converted to a numeric data type; otherwise, it returns 0.
SELECT ISNUMERIC('123') AS is_numeric;
In this example, the ISNUMERIC function is used to check if the value '123' is numeric in Microsoft SQL Server. The function returns 1, indicating that '123' is a valid numeric value.
These are just a few examples of how you can validate numeric data in other database systems. While the specific functions and syntax may differ, the underlying concept remains the same: checking if a value is numeric or not.
Common Use Cases for PostgreSQL in Data Management
PostgreSQL is a versatile and useful database management system that can be used in a wide range of data management scenarios. Here are some common use cases where PostgreSQL excels:
1. Web Applications: PostgreSQL is a popular choice for web application development due to its scalability, reliability, and performance. It offers excellent support for handling concurrent users, large data sets, and complex queries. PostgreSQL's compatibility with various web development frameworks, such as Django and Ruby on Rails, makes it an ideal choice for building web applications.
2. Data Warehousing: PostgreSQL's advanced features, such as table partitioning, parallel query execution, and support for advanced indexing techniques, make it well-suited for data warehousing. It can handle large volumes of data and perform complex analytical queries efficiently. PostgreSQL's extensibility allows for the integration of custom data types and functions, enabling advanced analytics and reporting capabilities.
3. Geospatial Data Management: PostgreSQL has built-in support for geospatial data through its PostGIS extension. This extension provides spatial data types, functions, and operators for storing, indexing, and querying geospatial data. PostgreSQL's robust geospatial capabilities make it an excellent choice for applications that deal with location-based data, such as mapping, GIS, and logistics.
4. Scientific Research: PostgreSQL's support for advanced data types, such as arrays, JSON, and XML, makes it suitable for scientific research applications. It can store and process complex scientific data efficiently. PostgreSQL's extensibility allows researchers to define custom data types and implement domain-specific functions, enabling advanced data analysis and modeling.
5. Content Management Systems (CMS): PostgreSQL can be used as the backend database for content management systems, providing a reliable and scalable storage solution. Its support for complex data models, full-text search, and efficient query execution make it a good fit for managing content-heavy applications. PostgreSQL's security features and support for multiple programming languages also contribute to its suitability for CMS implementations.
6. Internet of Things (IoT): PostgreSQL's scalability, extensibility, and support for JSON data make it well-suited for IoT applications. It can handle large volumes of sensor data and store it efficiently. PostgreSQL's support for JSON allows for flexible schema management, making it easier to handle dynamic data structures commonly encountered in IoT scenarios.
7. Financial Systems: PostgreSQL's support for decimal data types, advanced indexing options, and security features make it a suitable choice for financial systems. It can handle complex financial calculations, ensure data accuracy and integrity, and enforce strict security measures. PostgreSQL's compatibility with various programming languages allows for seamless integration with financial applications.
These are just a few examples of the common use cases where PostgreSQL shines as a data management solution. Its versatility, performance, and extensive feature set make it a popular choice for a wide range of applications and industries. Whether you are building a web application, managing scientific data, or handling IoT sensor data, PostgreSQL provides a reliable and scalable foundation for your data management needs.
Additional Resources
- PostgreSQL - Official Website