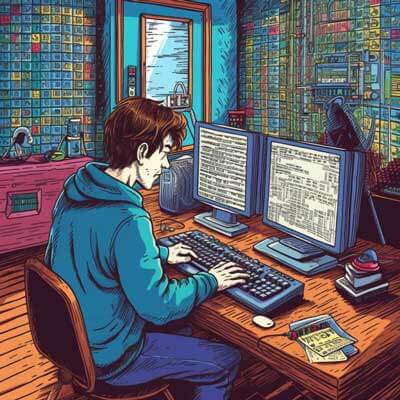
- Dealing with Non-Existent Relations in PostgreSQL: How to Handle Missing Data in a Postgres Database
- 1. Checking for the Existence of a Relation
- 2. Handling Non-Existent Relations in SQL Statements
- 3. Error Handling in Non-Existent Relations
- 4. Querying Non-Existent Relations
- Data Storage in PostgreSQL: Understanding the Storage Mechanisms Used by PostgreSQL
- 1. Heap Storage
- 2. Index Storage
- 3. TOAST (The Oversized-Attribute Storage Technique)
- Data Retrieval in PostgreSQL: Techniques for Efficiently Fetching Data from a PostgreSQL Database
- 1. Using Indexes
- 2. Using Query Optimization Techniques
- 3. Using Caching
- Data Querying in PostgreSQL: Advanced Querying Techniques in PostgreSQL
- 1. Window Functions
- 2. Common Table Expressions (CTEs)
- 3. Recursive Queries
- Data Manipulation in PostgreSQL: Performing CRUD Operations in a PostgreSQL Database
- 1. Creating Records
- 2. Reading Records
- 3. Updating Records
- 4. Deleting Records
- Data Modeling in PostgreSQL: Best Practices for Designing a Database Schema in PostgreSQL
- 1. Normalization
- 2. Establishing Relationships
- 3. Enforcing Data Integrity Constraints
- Advantages of Using PostgreSQL: Benefits and Features of PostgreSQL as a Database Management System
- 1. Reliability and Stability
- 2. Data Integrity and ACID Compliance
- 3. Extensibility and Flexibility
- 4. Advanced Querying and Optimization
- 5. Replication and High Availability
- 6. Scalability and Performance
- 7. Community Support and Ecosystem
- Additional Resources
Dealing with Non-Existent Relations in PostgreSQL: How to Handle Missing Data in a Postgres Database
In PostgreSQL, a relation refers to a table or view that stores data. When working with a PostgreSQL database, it is common to encounter situations where a relation does not exist. This can happen due to various reasons such as a typo in the table name, a table that has not been created yet, or a table that has been dropped.
Handling non-existent relations is an important aspect of database management and application development. In this tutorial, we will explore different techniques and best practices for dealing with non-existent relations in PostgreSQL.
Related Article: PostgreSQL HyperLogLog (HLL) & Cardinality Estimation
1. Checking for the Existence of a Relation
Before performing any operations on a relation, it is crucial to check if the relation exists in the database. PostgreSQL provides several ways to check for the existence of a relation. Let’s take a look at a few examples:
Example 1: Using the “pg_class” Catalog Table
SELECT EXISTS ( SELECT 1 FROM pg_class WHERE relname = 'employees' );
In this example, we use the “pg_class” catalog table to check if a relation with the name “employees” exists. The “pg_class” table stores metadata about relations in the database. If the relation exists, the query will return “true”; otherwise, it will return “false”.
Example 2: Using the “information_schema.tables” View
SELECT EXISTS ( SELECT 1 FROM information_schema.tables WHERE table_name = 'employees' );
In this example, we use the “information_schema.tables” view, which provides access to information about tables in the database. We check if a table with the name “employees” exists by querying the “table_name” column. If the table exists, the query will return “true”; otherwise, it will return “false”.
2. Handling Non-Existent Relations in SQL Statements
When writing SQL statements that involve relations, it is important to handle the case where the relation does not exist. One common approach is to use the “IF EXISTS” clause, which allows the statement to proceed only if the relation exists. Here’s an example:
Example 1: Dropping a Table if it Exists
DROP TABLE IF EXISTS employees;
In this example, the “DROP TABLE” statement is executed only if the “employees” table exists. If the table does not exist, the statement is ignored, preventing an error from being thrown.
Example 2: Creating a Table if it Does Not Exist
CREATE TABLE IF NOT EXISTS employees ( id SERIAL <a href="https://www.squash.io/exploring-sql-join-conditions-the-role-of-primary-keys/">PRIMARY KEY</a>, name VARCHAR(50) NOT NULL );
In this example, the “CREATE TABLE” statement is executed only if the “employees” table does not exist. If the table already exists, the statement is ignored.
3. Error Handling in Non-Existent Relations
When working with non-existent relations, it is important to handle errors gracefully. PostgreSQL provides mechanisms for error handling, such as using the “BEGIN” and “END” statements to define a block of code and the “EXCEPTION” clause to catch specific errors.
Example: Handling Non-Existent Table Error
BEGIN; -- Perform operations on the "employees" table -- ... EXCEPTION WHEN undefined_table THEN -- Handle the error gracefully -- ... END;
In this example, we encapsulate the code that operates on the “employees” table within a transaction block. If the “employees” table does not exist, the “EXCEPTION” clause catches the “undefined_table” error, allowing us to handle it gracefully.
Related Article: How to Check if a Table Exists in PostgreSQL
4. Querying Non-Existent Relations
When querying non-existent relations, it is important to handle the case where the relation does not exist. One approach is to use conditional statements, such as the “CASE” statement, to handle different scenarios.
Example: Querying a Table if it Exists
SELECT * FROM ( SELECT * FROM employees WHERE EXISTS ( SELECT 1 FROM information_schema.tables WHERE table_name = 'employees' ) ) AS result;
In this example, we use a subquery to check if the “employees” table exists in the database. If the table exists, the outer query selects all rows from the “employees” table. If the table does not exist, the outer query returns an empty result set.
Data Storage in PostgreSQL: Understanding the Storage Mechanisms Used by PostgreSQL
PostgreSQL is a useful open-source relational database management system that provides various storage mechanisms for efficient data storage and retrieval. Understanding these storage mechanisms is crucial for designing efficient database schemas and optimizing query performance. In this section, we will explore the different storage mechanisms used by PostgreSQL.
1. Heap Storage
The heap storage is the default storage mechanism used by PostgreSQL. It stores data in a simple, unordered format where each table is represented as a collection of pages. Each page contains a fixed number of rows, and when a page is full, a new page is allocated to store additional rows.
Example: Creating a Table using Heap Storage
CREATE TABLE employees ( id SERIAL PRIMARY KEY, name VARCHAR(50) NOT NULL );
In this example, we create a table called “employees” using the heap storage mechanism. The “id” column is defined as a serial primary key, which automatically generates a unique value for each row. The “name” column is defined as a non-null varchar with a maximum length of 50 characters.
Related Article: Applying Aggregate Functions in PostgreSQL WHERE Clause
2. Index Storage
Indexes are a crucial component of any database system as they enable efficient data retrieval by providing quick access to specific rows based on the values of indexed columns. PostgreSQL supports various types of indexes, including B-tree, hash, and GiST indexes.
Example: Creating an Index on a Table
CREATE INDEX idx_employees_name ON employees (name);
In this example, we create an index called “idx_employees_name” on the “name” column of the “employees” table. This index allows for fast retrieval of rows based on the values of the “name” column.
3. TOAST (The Oversized-Attribute Storage Technique)
TOAST is a storage technique used by PostgreSQL to handle large values that exceed the maximum size of a page. It allows for efficient storage and retrieval of large objects by storing them in separate TOAST tables and compressing them if necessary.
Example: Storing Large Values in a Table
CREATE TABLE products ( id SERIAL PRIMARY KEY, name VARCHAR(50) NOT NULL, description TEXT );
In this example, we create a table called “products” with a “description” column of type TEXT. If the size of the “description” value exceeds the maximum size of a page, PostgreSQL automatically stores it in a TOAST table.
Data Retrieval in PostgreSQL: Techniques for Efficiently Fetching Data from a PostgreSQL Database
Efficiently retrieving data from a PostgreSQL database is crucial for optimizing the performance of your applications. PostgreSQL provides various techniques and features that can be used to improve data retrieval performance. In this section, we will explore some of these techniques.
Related Article: How to Convert Columns to Rows in PostgreSQL
1. Using Indexes
Indexes are one of the most effective ways to improve data retrieval performance in PostgreSQL. They provide quick access to specific rows based on the values of indexed columns. PostgreSQL supports various types of indexes, including B-tree, hash, and GiST indexes.
Example: Querying a Table using an Index
SELECT * FROM employees WHERE name = 'John Doe';
In this example, we query the “employees” table to retrieve all rows where the value of the “name” column is ‘John Doe’. If an index exists on the “name” column, PostgreSQL can use it to quickly locate the relevant rows, improving the query performance.
2. Using Query Optimization Techniques
PostgreSQL includes a sophisticated query optimizer that automatically determines the most efficient execution plan for a given query. However, there are cases where manual query optimization techniques can further improve performance.
Example: Using the EXPLAIN Statement
EXPLAIN SELECT * FROM employees WHERE name = 'John Doe';
In this example, we use the EXPLAIN statement to analyze the execution plan of a query. The EXPLAIN statement provides valuable insights into how PostgreSQL plans to execute the query, including the order in which tables are accessed and the types of joins used. This information can help identify potential performance bottlenecks and optimize the query accordingly.
3. Using Caching
Caching is a technique that can significantly improve data retrieval performance by storing frequently accessed data in memory. PostgreSQL provides built-in caching mechanisms, such as the shared buffer cache, which caches data pages in memory to reduce disk I/O.
Example: Configuring the Shared Buffer Cache
To configure the shared buffer cache, you can modify the “shared_buffers” configuration parameter in the PostgreSQL configuration file (postgresql.conf).
shared_buffers = 2GB
In this example, we allocate 2GB of memory to the shared buffer cache. Increasing the size of the shared buffer cache can improve data retrieval performance by reducing the frequency of disk I/O operations.
Related Article: Detecting and Resolving Deadlocks in PostgreSQL Databases
Data Querying in PostgreSQL: Advanced Querying Techniques in PostgreSQL
PostgreSQL provides a rich set of advanced querying techniques that allow you to extract meaningful insights from your data. These techniques include window functions, common table expressions (CTEs), recursive queries, and more. In this section, we will explore some of these advanced querying techniques.
1. Window Functions
Window functions allow you to perform calculations across a set of rows that are related to the current row. They provide a flexible and useful way to analyze and manipulate data in a single query.
Example: Calculating Running Totals using Window Functions
SELECT id, name, salary, SUM(salary) OVER (ORDER BY id) AS running_total FROM employees;
In this example, we use the SUM window function to calculate the running total of the “salary” column. The OVER clause specifies the window frame, which in this case is ordered by the “id” column. The running total is calculated by summing the salaries of all previous rows.
2. Common Table Expressions (CTEs)
Common table expressions (CTEs) allow you to define temporary result sets that can be referenced multiple times within a single query. They provide a clean and readable way to break down complex queries into smaller, more manageable parts.
Example: Using a CTE to Calculate Employee Salaries
WITH employee_salaries AS ( SELECT id, name, salary FROM employees ) SELECT id, name, salary, salary * 12 AS annual_salary FROM employee_salaries;
In this example, we define a CTE called “employee_salaries” that selects the “id”, “name”, and “salary” columns from the “employees” table. We then use the CTE in the main query to calculate the annual salary by multiplying the monthly salary by 12.
Related Article: Executing Efficient Spatial Queries in PostgreSQL
3. Recursive Queries
Recursive queries allow you to query hierarchical data structures, such as organization charts or file systems, where each row references one or more rows in the same table. They provide a useful way to traverse and manipulate hierarchical data.
Example: Querying a Hierarchical Data Structure
Consider a table called “employees” with the following columns: “id”, “name”, and “manager_id”. The “manager_id” column references the “id” column of the employee’s manager.
WITH RECURSIVE employee_hierarchy AS ( SELECT id, name, manager_id, 0 AS level FROM employees WHERE id = 1 -- Starting employee ID UNION ALL SELECT e.id, e.name, e.manager_id, eh.level + 1 FROM employees e <a href="https://www.squash.io/tutorial-the-functionality-of-inner-join-in-sql/">INNER JOIN</a> employee_hierarchy eh ON e.manager_id = eh.id ) SELECT id, name, level FROM employee_hierarchy;
In this example, we define a recursive CTE called “employee_hierarchy” that selects the “id”, “name”, “manager_id”, and “level” columns from the “employees” table. The initial query selects the employee with the specified ID and sets the level to 0. The recursive part of the query joins the “employees” table with the CTE and increments the level by 1 for each level in the hierarchy.
Data Manipulation in PostgreSQL: Performing CRUD Operations in a PostgreSQL Database
Performing CRUD (Create, Read, Update, Delete) operations is a fundamental aspect of working with databases. PostgreSQL provides a wide range of features and techniques for manipulating data efficiently. In this section, we will explore how to perform CRUD operations in a PostgreSQL database.
1. Creating Records
To create records in a PostgreSQL database, you can use the INSERT statement. The INSERT statement allows you to insert one or more rows into a table.
Example: Inserting a Single Record
INSERT INTO employees (name, salary) VALUES ('John Doe', 5000);
In this example, we insert a single record into the “employees” table. We provide the values for the “name” and “salary” columns using the VALUES clause.
Example: Inserting Multiple Records
INSERT INTO employees (name, salary) VALUES ('John Doe', 5000), ('Jane Smith', 6000), ('Michael Johnson', 7000);
In this example, we insert multiple records into the “employees” table. We provide the values for the “name” and “salary” columns for each record using the VALUES clause.
Related Article: Preventing Locking Queries in Read-Only PostgreSQL Databases
2. Reading Records
To read records from a PostgreSQL database, you can use the SELECT statement. The SELECT statement allows you to retrieve data from one or more tables based on specified criteria.
Example: Selecting All Records
SELECT * FROM employees;
In this example, we select all records from the “employees” table. The asterisk (*) is used to represent all columns in the table.
Example: Selecting Specific Columns
SELECT name, salary FROM employees;
In this example, we select only the “name” and “salary” columns from the “employees” table. This can be useful when you only need specific columns in your query.
3. Updating Records
To update records in a PostgreSQL database, you can use the UPDATE statement. The UPDATE statement allows you to modify existing records in one or more tables.
Example: Updating a Single Record
UPDATE employees SET salary = 6000 WHERE id = 1;
In this example, we update the “salary” column of the employee with the ID equal to 1. We set the new value of the “salary” column to 6000.
Example: Updating Multiple Records
UPDATE employees SET salary = salary * 1.1 WHERE salary < 5000;
In this example, we update the "salary" column of all employees whose current salary is less than 5000. We multiply the current salary by 1.1 to give a 10% raise.
4. Deleting Records
To delete records from a PostgreSQL database, you can use the DELETE statement. The DELETE statement allows you to remove one or more rows from a table.
Example: Deleting a Single Record
DELETE FROM employees WHERE id = 1;
In this example, we delete the employee with the ID equal to 1 from the “employees” table.
Example: Deleting Multiple Records
DELETE FROM employees WHERE salary < 5000;
In this example, we delete all employees whose salary is less than 5000 from the "employees" table.
Related Article: Passing Query Results to a SQL Function in PostgreSQL
Data Modeling in PostgreSQL: Best Practices for Designing a Database Schema in PostgreSQL
Designing a well-structured and efficient database schema is essential for the success of any application. PostgreSQL provides a flexible and useful data modeling capability that allows you to define relationships between tables and enforce data integrity constraints. In this section, we will explore some best practices for designing a database schema in PostgreSQL.
1. Normalization
Normalization is the process of organizing data in a database to eliminate redundancy and improve data integrity. It involves breaking down the data into smaller, more manageable tables and establishing relationships between them.
Example: Normalizing a Database Schema
Consider a database schema for an e-commerce application. Instead of storing all customer information in a single table, we can normalize the schema by splitting the data into separate tables for customers, orders, and products. This allows for more efficient storage and retrieval of data.
2. Establishing Relationships
Establishing relationships between tables is crucial for maintaining data integrity and ensuring consistency. PostgreSQL supports various types of relationships, including one-to-one, one-to-many, and many-to-many relationships.
Example: Establishing a One-to-Many Relationship
Consider a database schema with two tables: “customers” and “orders”. Each customer can have multiple orders, but each order belongs to a single customer. We can establish a one-to-many relationship between the two tables by adding a foreign key column in the “orders” table that references the primary key column in the “customers” table.
Related Article: Resolving Access Issues with Query Pg Node in PostgreSQL
3. Enforcing Data Integrity Constraints
Data integrity constraints ensure the accuracy and consistency of data in a database. PostgreSQL provides various types of constraints, including primary key constraints, foreign key constraints, unique constraints, and check constraints.
Example: Adding Constraints to a Table
CREATE TABLE customers ( id SERIAL PRIMARY KEY, name VARCHAR(50) NOT NULL, email VARCHAR(100) UNIQUE );
In this example, we create a table called “customers” with a primary key constraint on the “id” column and a unique constraint on the “email” column. The primary key constraint ensures that each row in the table has a unique identifier, while the unique constraint ensures that each email address is unique.
Advantages of Using PostgreSQL: Benefits and Features of PostgreSQL as a Database Management System
PostgreSQL is a popular open-source relational database management system that offers numerous advantages and features. In this section, we will explore some of the benefits of using PostgreSQL for your database management needs.
1. Reliability and Stability
PostgreSQL is known for its reliability and stability. It is designed to handle high volumes of data and concurrent users without compromising performance. PostgreSQL has a proven track record of being reliable and stable, making it a trusted choice for mission-critical applications.
Related Article: Does PostgreSQL Have a Maximum SQL Query Length?
2. Data Integrity and ACID Compliance
PostgreSQL ensures data integrity and supports ACID (Atomicity, Consistency, Isolation, Durability) properties, which guarantee that database transactions are processed reliably. It provides mechanisms for enforcing data integrity constraints and supports transactional processing, allowing for the consistent and reliable management of data.
3. Extensibility and Flexibility
PostgreSQL is highly extensible and flexible. It provides a wide range of data types, including built-in and user-defined types, allowing you to store and manipulate data in a variety of formats. PostgreSQL also supports the creation of custom functions, operators, and aggregates, enabling you to extend the functionality of the database.
4. Advanced Querying and Optimization
PostgreSQL offers advanced querying capabilities, including support for window functions, common table expressions (CTEs), recursive queries, and more. It also includes a sophisticated query optimizer that automatically determines the most efficient execution plan for a given query, resulting in improved query performance.
Related Article: How to Use PostgreSQL SELECT INTO TEMP Table
5. Replication and High Availability
PostgreSQL supports various replication techniques, such as streaming replication and logical replication, which allow you to create replicas of your database for high availability and fault tolerance. Replication in PostgreSQL can be synchronous or asynchronous, giving you control over the trade-off between data consistency and performance.
6. Scalability and Performance
PostgreSQL is designed to scale both vertically and horizontally. It supports partitioning, which allows you to divide large tables into smaller manageable pieces for improved performance. PostgreSQL also provides various performance optimization techniques, such as indexing, query optimization, and caching, to ensure efficient data retrieval and processing.
7. Community Support and Ecosystem
PostgreSQL has a vibrant and active community of developers and users who contribute to its development and provide support. The PostgreSQL community offers extensive documentation, mailing lists, forums, and conferences, making it easy to find help and resources. Additionally, PostgreSQL has a rich ecosystem of extensions and tools that further enhance its functionality and usability.
Related Article: Exploring Natural Join in PostgreSQL Databases
Additional Resources
– Creating a Table – PostgreSQL Documentation
– Primary Keys – PostgreSQL Documentation
– Foreign Keys – PostgreSQL Documentation