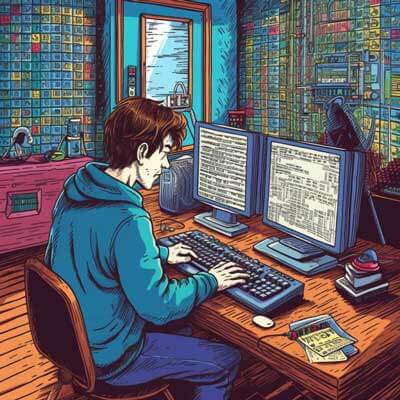
- Introduction
- Steps to Pass Query Results to a Function
- Using Query Results as Function Input
- Syntax for Passing Query Results to a Function
- Limitations and Restrictions of Passing Query Results to a Function
- Passing Multiple Columns or Rows as Function Parameters
- Best Practices for Passing Query Results to Functions
- Passing Subquery Results to a Function
- Handling Null Values in Query Results Passed to a Function
Introduction
PostgreSQL is a useful open-source relational database management system that offers a wide range of features and capabilities. One of the key strengths of PostgreSQL is its support for user-defined functions, which allow developers to extend the functionality of the database and perform complex operations. In this article, we will explore how to pass query results to a SQL function in PostgreSQL, enabling developers to leverage the power of SQL queries within their functions.
Related Article: PostgreSQL HyperLogLog (HLL) & Cardinality Estimation
Steps to Pass Query Results to a Function
To pass query results to a SQL function in PostgreSQL, follow these steps:
1. Create a function: Define a new function using the CREATE FUNCTION
statement. This function will receive the query results as input parameters. Specify the appropriate data types for the input parameters based on the expected query results.
2. Retrieve query results: Execute a SQL query to retrieve the desired results. This query can be as simple or as complex as needed, and can involve multiple tables, joins, conditions, and aggregations.
3. Call the function: Invoke the function and pass the query results as input parameters. Use the SELECT
statement to call the function and provide the query results as arguments.
4. Process the query results: Within the function, use the input parameters to perform any required processing or calculations. The function can access the query results as regular variables and use them in SQL statements or other logic.
5. Return the result: Optionally, the function can return a result value or a set of values using the RETURN
statement. This allows the function to provide the processed query results or any other computed values to the caller.
Using Query Results as Function Input
Let’s see an example of how to pass query results to a SQL function in PostgreSQL. Suppose we have a table called employees
with columns id
, name
, and salary
. We want to calculate the average salary of all employees whose names start with a specific letter. We can achieve this by creating a function that takes the query results as input and calculates the average salary.
Here’s how the function would look like:
CREATE FUNCTION calculate_average_salary(employees_cursor CURSOR) RETURNS FLOAT AS $$ DECLARE total_salary FLOAT := 0; num_employees INTEGER := 0; employee_record RECORD; BEGIN FOR employee_record IN employees_cursor LOOP total_salary := total_salary + employee_record.salary; num_employees := num_employees + 1; END LOOP; IF num_employees > 0 THEN RETURN total_salary / num_employees; ELSE RETURN 0; END IF; END; $$ LANGUAGE plpgsql;
In this example, the function calculate_average_salary
takes a cursor as an input parameter. The cursor represents the query results, which are iterated over using a FOR
loop. The salary of each employee is accumulated in the total_salary
variable, and the number of employees is counted using the num_employees
variable. Finally, the average salary is calculated by dividing the total_salary
by the num_employees
and returned as the result.
To call this function and pass the query results, we can use the following SQL statement:
SELECT calculate_average_salary(cursor(SELECT * FROM employees WHERE name LIKE 'A%'));
This statement retrieves all employees whose names start with the letter ‘A’ and passes the query results to the calculate_average_salary
function. The function then processes the query results and returns the average salary.
Syntax for Passing Query Results to a Function
To pass query results to a SQL function in PostgreSQL, you can use the following syntax:
SELECT function_name(cursor(query));
In this syntax, function_name
is the name of the function you want to call, cursor
is a keyword indicating that the query results should be treated as a cursor, and query
is the SQL query that retrieves the desired results.
Related Article: How to Check if a Table Exists in PostgreSQL
Limitations and Restrictions of Passing Query Results to a Function
While passing query results to a SQL function in PostgreSQL offers great flexibility and power, there are some limitations and restrictions to be aware of:
1. Data types: The function’s input parameters must be compatible with the data types of the query results. If there is a mismatch, you may encounter errors or unexpected behavior.
2. Query complexity: The query used to retrieve the results should be carefully constructed to ensure that it returns the desired data. Complex queries with multiple joins, aggregations, or subqueries may require additional considerations.
3. Performance impact: Passing query results to a function can have performance implications, especially if the query returns a large number of rows or involves complex calculations. Consider the performance impact when using this approach in production systems.
4. Modifying query results: If the function modifies the query results, such as updating or deleting rows, it may have unintended consequences. Ensure that the function’s logic is carefully designed to handle such scenarios.
Passing Multiple Columns or Rows as Function Parameters
In addition to passing query results as a whole, PostgreSQL also allows passing individual columns or rows as function parameters. This can be useful when you only need specific data from the query results or want to process each row separately.
To pass a specific column as a function parameter, you can use the following syntax:
SELECT function_name(column_name) FROM table_name;
In this example, function_name
is the name of the function, column_name
is the name of the column you want to pass, and table_name
is the name of the table containing the data.
To pass an entire row as a function parameter, you can use the following syntax:
SELECT function_name(row_variable) FROM table_name;
In this example, function_name
is the name of the function, row_variable
is a variable representing a row, and table_name
is the name of the table containing the data.
Best Practices for Passing Query Results to Functions
When passing query results to a SQL function in PostgreSQL, it is important to follow these best practices:
1. Validate input: Ensure that the input parameters are properly validated before using them in the function. Validate data types, lengths, and any other constraints to prevent potential errors or security vulnerabilities.
2. Optimize queries: Write efficient and optimized queries to retrieve the required data. Consider indexing, query rewriting, or other performance optimization techniques to minimize the impact on the database.
3. Handle errors: Implement error handling mechanisms within the function to handle potential errors gracefully. Use try-catch blocks or other error handling constructs to provide meaningful error messages and handle exceptional situations.
4. Test thoroughly: Test the function with various scenarios and datasets to ensure its correctness and performance. Use unit tests, integration tests, or other testing methodologies to validate the function’s behavior.
5. Document the function: Provide clear and comprehensive documentation for the function, including its purpose, input parameters, expected behavior, and any limitations or restrictions. This will help other developers understand and use the function effectively.
Related Article: Applying Aggregate Functions in PostgreSQL WHERE Clause
Passing Subquery Results to a Function
In PostgreSQL, it is also possible to pass the results of a subquery to a SQL function. A subquery is a query that is nested within another query and is used to retrieve a subset of data based on specific conditions.
To pass the results of a subquery to a function, you can use the following syntax:
SELECT function_name(subquery) FROM table_name;
In this syntax, function_name
is the name of the function, subquery
is the subquery that retrieves the desired results, and table_name
is the name of the table containing the data.
Here’s an example that demonstrates how to pass the results of a subquery to a function. Suppose we have a table called orders
with columns order_id
, customer_id
, and total_amount
. We want to calculate the average total amount for all orders made by customers who have made more than a certain number of orders. We can achieve this by creating a function that takes the subquery results as input and calculates the average total amount.
CREATE FUNCTION calculate_average_amount(orders_subquery subquery) RETURNS FLOAT AS $$ DECLARE total_amount FLOAT := 0; num_orders INTEGER := 0; order_record RECORD; BEGIN FOR order_record IN orders_subquery LOOP total_amount := total_amount + order_record.total_amount; num_orders := num_orders + 1; END LOOP; IF num_orders > 0 THEN RETURN total_amount / num_orders; ELSE RETURN 0; END IF; END; $$ LANGUAGE plpgsql;
To call this function and pass the results of a subquery, we can use the following SQL statement:
SELECT calculate_average_amount((SELECT * FROM orders WHERE customer_id IN (SELECT customer_id FROM customers WHERE num_orders > 10)));
This statement retrieves all orders made by customers who have made more than 10 orders and passes the subquery results to the calculate_average_amount
function. The function then processes the subquery results and returns the average total amount.
Handling Null Values in Query Results Passed to a Function
When passing query results to a SQL function in PostgreSQL, it is important to handle null values appropriately, as they can affect the function’s behavior and result.
To handle null values in query results passed to a function, you can use conditional statements or null handling functions such as COALESCE
or NULLIF
. These functions allow you to replace null values with a default value or perform specific actions based on the presence of null values.
Here’s an example that demonstrates how to handle null values in query results passed to a function. Suppose we have a table called products
with columns product_id
and price
. We want to calculate the total price of all products and handle null values in the price column.
CREATE FUNCTION calculate_total_price(products_cursor CURSOR) RETURNS FLOAT AS $$ DECLARE total_price FLOAT := 0; product_record RECORD; BEGIN FOR product_record IN products_cursor LOOP total_price := total_price + COALESCE(product_record.price, 0); END LOOP; RETURN total_price; END; $$ LANGUAGE plpgsql;
In this example, the COALESCE
function is used to handle null values in the price column. If the price is null, it is replaced with 0 before adding it to the total_price
variable. This ensures that null values do not affect the calculation.
To call this function and pass the query results, we can use the following SQL statement:
SELECT calculate_total_price(cursor(SELECT * FROM products));
This statement retrieves all products and passes the query results to the calculate_total_price
function. The function then processes the query results, handling null values in the price column, and returns the total price.