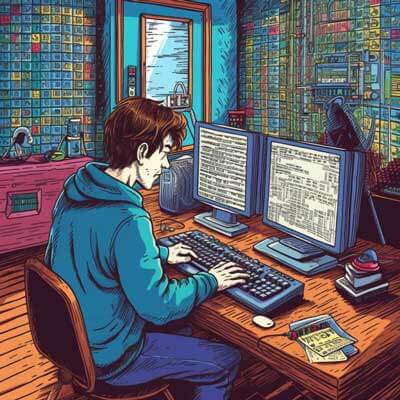
When performing a join operation in SQL, you can select specific columns from the joined tables to include in the result set. This can be useful when you only need certain information from the tables and want to minimize the amount of data returned.
To select specific columns in a join operation, you can use the SELECT
statement and specify the column names you want to retrieve. The selected columns can come from either or both of the joined tables.
Let’s consider an example to understand how to select specific columns in a join operation. Suppose we have two tables, orders
and customers
, with the following structures:
CREATE TABLE orders ( order_id INT, customer_id INT, order_date DATE, total_amount DECIMAL(10,2) ); CREATE TABLE customers ( customer_id INT, customer_name VARCHAR(50), email VARCHAR(50) );
We want to retrieve the customer name and order date for each order. To do this, we can use an inner join operation between the orders
and customers
tables, matching the customer_id
column. We will select only the customer_name
and order_date
columns:
SELECT customers.customer_name, orders.order_date FROM customers JOIN orders ON customers.customer_id = orders.customer_id;
This query will return the customer name and order date for each matching row in the orders
and customers
tables.
Selecting Specific Columns in SQL Join: Example 1:
Consider the following tables:
CREATE TABLE employees ( id INT, name VARCHAR(50), department_id INT ); CREATE TABLE departments ( id INT, department_name VARCHAR(50) );
We want to retrieve the names of employees along with their department names. To achieve this, we can use an inner join operation between the employees
and departments
tables, matching the department_id
column. We will select only the employees.name
and departments.department_name
columns:
SELECT employees.name, departments.department_name FROM employees JOIN departments ON employees.department_id = departments.id;
This query will return the names of employees along with their corresponding department names.
Related Article: Tutorial: ON for JOIN SQL in Databases
Selecting Specific Columns in SQL Join: Example 2:
Consider the following tables:
CREATE TABLE products ( id INT, name VARCHAR(50), category_id INT ); CREATE TABLE categories ( id INT, category_name VARCHAR(50) );
We want to retrieve the names of products along with their category names. To achieve this, we can use an inner join operation between the products
and categories
tables, matching the category_id
column. We will select only the products.name
and categories.category_name
columns:
SELECT products.name, categories.category_name FROM products JOIN categories ON products.category_id = categories.id;
This query will return the names of products along with their corresponding category names.
How to Perform SQL Join Operations
In SQL, join operations are used to combine rows from two or more tables based on a related column between them. Join operations allow you to retrieve data from multiple tables as a single result set, making it a useful feature in database management systems. There are several types of join operations, including inner join, outer join, left join, and right join, each serving a specific purpose.
To perform a join operation, you need to specify the tables you want to join and the related columns between them. The related columns are used to match the rows from different tables. The syntax for a join operation is as follows:
SELECT column_list FROM table1 JOIN table2 ON table1.column = table2.column;
In this syntax, table1
and table2
are the tables you want to join, and column
is the related column between them. The SELECT
statement is used to specify the columns you want to retrieve from the joined tables. The result set will contain the selected columns from both tables for the matched rows.
Let’s consider an example to understand how to perform a join operation. Suppose we have two tables, orders
and customers
, with the following structures:
CREATE TABLE orders ( order_id INT, customer_id INT, order_date DATE, total_amount DECIMAL(10,2) ); CREATE TABLE customers ( customer_id INT, customer_name VARCHAR(50), email VARCHAR(50) );
We want to retrieve the customer name and order date for each order. To do this, we can use an inner join operation between the orders
and customers
tables, matching the customer_id
column:
SELECT customers.customer_name, orders.order_date FROM customers JOIN orders ON customers.customer_id = orders.customer_id;
This query will return the customer name and order date for each matching row in the orders
and customers
tables.
Example 1:
Consider the following tables:
CREATE TABLE employees ( id INT, name VARCHAR(50), department_id INT ); CREATE TABLE departments ( id INT, department_name VARCHAR(50) );
We want to retrieve the names of employees along with their department names. To achieve this, we can use an inner join operation between the employees
and departments
tables, matching the department_id
column:
SELECT employees.name, departments.department_name FROM employees JOIN departments ON employees.department_id = departments.id;
This query will return the names of employees along with their corresponding department names.
Related Article: Analyzing SQL Join and Its Effect on Records
Example 2:
Consider the following tables:
CREATE TABLE products ( id INT, name VARCHAR(50), category_id INT ); CREATE TABLE categories ( id INT, category_name VARCHAR(50) );
We want to retrieve the names of products along with their category names. To achieve this, we can use an inner join operation between the products
and categories
tables, matching the category_id
column:
SELECT products.name, categories.category_name FROM products JOIN categories ON products.category_id = categories.id;
This query will return the names of products along with their corresponding category names.
Additional Resources
– SQL – SELECT Statement
– SQL Joins
– PostgreSQL – SELECT Statement