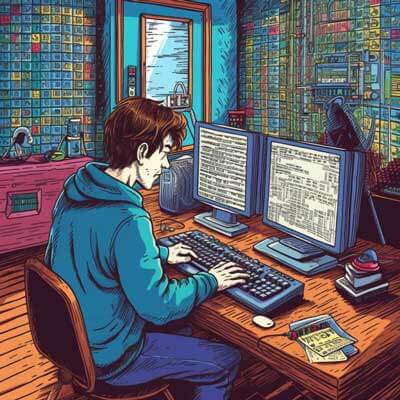
- Benefits of Spatial Indexes in PostgreSQL
- Storing and Querying Geospatial Data in PostgreSQL
- Introduction to PostGIS and its Relation to Spatial Queries in PostgreSQL
- Understanding the R-tree Index in PostgreSQL for Efficient Spatial Queries
- Performing KNN Search in PostgreSQL
- Finding Nearest Neighbors in PostgreSQL
- Differences Between the Geography and Geometry Datatypes in PostgreSQL
- Utilizing Bounding Boxes in Spatial Queries with PostgreSQL
- Exploring Different Types of Geometries in Spatial Queries with PostgreSQL
- Performing Spatial Queries to Find Points within a Certain Distance in PostgreSQL
- Additional Resources
Benefits of Spatial Indexes in PostgreSQL
Spatial indexes in PostgreSQL provide several benefits that make spatial queries more efficient. By using spatial indexes, you can improve the performance of your queries, especially when dealing with large datasets. Here are some key benefits of spatial indexes in PostgreSQL:
1. Faster Query Execution: Spatial indexes allow PostgreSQL to quickly narrow down the search space when executing spatial queries. This is achieved by organizing the spatial data in a data structure that optimizes spatial search operations.
2. Reduced I/O Operations: With spatial indexes, PostgreSQL can minimize the number of disk I/O operations required to retrieve the data relevant to a spatial query. This results in faster query execution times and improved overall system performance.
3. Efficient Range Searches: Spatial indexes enable efficient range searches, allowing you to query for spatial objects within a specified area or range. This is particularly useful when dealing with geospatial data such as points, polygons, or lines.
4. Support for Spatial Operators: PostgreSQL’s spatial indexes support various spatial operators, such as intersects, contains, and overlaps. These operators enable you to perform complex spatial queries by combining multiple conditions.
To illustrate the benefits of spatial indexes, let’s consider an example where we have a table named “locations” with a spatial column “geom” representing the geometry of each location. We want to find all locations within a certain distance from a given point:
-- Create a spatial index on the "geom" column CREATE INDEX locations_geom_idx ON locations USING GIST (geom); -- Query for locations within a certain distance from a point SELECT * FROM locations WHERE ST_DWithin( geom, ST_SetSRID(ST_Point(42.3601, -71.0589), 4326), 1000 );
In the example above, the spatial index on the “geom” column allows PostgreSQL to efficiently search for locations within the specified distance from the given point, resulting in faster query execution.
Related Article: PostgreSQL HyperLogLog (HLL) & Cardinality Estimation
Storing and Querying Geospatial Data in PostgreSQL
PostgreSQL provides several data types for storing and querying geospatial data. These data types include “geometry” and “geography”, each with its own characteristics and use cases.
1. Geometry Data Type:
The “geometry” data type in PostgreSQL is used to store 2D geometric objects such as points, lines, and polygons. Geometry objects can be defined in various coordinate systems, including Cartesian (X, Y) or geographic (longitude, latitude) coordinates.
To store a geometry object in a table, you can define a column with the “geometry” data type. Here’s an example:
CREATE TABLE buildings ( id SERIAL PRIMARY KEY, name VARCHAR(100), location GEOMETRY(Point, 4326) );
In the example above, the “location” column is of type “geometry” and stores 2D points in the WGS 84 coordinate system (EPSG:4326).
To query geometry data, you can use a variety of spatial functions and operators provided by PostgreSQL’s PostGIS extension. For example, you can use the “ST_Intersects” function to find all buildings that intersect a given polygon:
SELECT * FROM buildings WHERE ST_Intersects( location, ST_GeomFromText('POLYGON((0 0, 0 10, 10 10, 10 0, 0 0))') );
The example above retrieves all buildings whose “location” intersects with the specified polygon.
2. Geography Data Type:
The “geography” data type in PostgreSQL is used to store geospatial data in a geographic coordinate system, such as latitude and longitude. Unlike the “geometry” data type, which operates in a Cartesian coordinate system, the “geography” data type takes into account the curvature of the Earth.
To store a geography object in a table, you can define a column with the “geography” data type. Here’s an example:
CREATE TABLE cities ( id SERIAL PRIMARY KEY, name VARCHAR(100), location GEOGRAPHY(Point, 4326) );
In the example above, the “location” column is of type “geography” and stores 2D points in the WGS 84 coordinate system (EPSG:4326).
To query geography data, you can use the same spatial functions and operators as with the “geometry” data type. However, the calculations performed on geography data take into account the curvature of the Earth, allowing for accurate distance and area calculations.
Introduction to PostGIS and its Relation to Spatial Queries in PostgreSQL
PostGIS is a useful extension for PostgreSQL that adds support for geospatial data and enables advanced spatial querying capabilities. It provides a set of functions and operators for manipulating and analyzing geospatial data, as well as spatial indexes for efficient querying.
One of the key features of PostGIS is its support for the Open Geospatial Consortium (OGC) standards, which ensures compatibility with other geospatial tools and datasets. PostGIS supports both the “geometry” and “geography” data types, allowing you to work with different coordinate systems and perform precise geospatial calculations.
To use PostGIS, you need to install it as an extension in your PostgreSQL database. Here’s how you can install PostGIS:
1. Ensure that you have PostgreSQL installed on your system.
2. Use the following command to install PostGIS:
CREATE EXTENSION IF NOT EXISTS postgis;
Once PostGIS is installed, you can start using its functions and operators for spatial querying. For example, you can use the “ST_Intersects” function to find all points that intersect a given polygon:
SELECT * FROM points WHERE ST_Intersects( geom, ST_GeomFromText('POLYGON((0 0, 0 10, 10 10, 10 0, 0 0))') );
In the example above, the “ST_Intersects” function checks if each point’s geometry intersects with the specified polygon’s geometry.
PostGIS also provides spatial indexing capabilities, which can significantly improve the performance of spatial queries. By creating a spatial index on a geometry or geography column, you can speed up queries that involve spatial relationships, such as intersects, contains, or within.
To create a spatial index, you can use the “CREATE INDEX” statement with the “USING GIST” option. Here’s an example:
CREATE INDEX points_geom_idx ON points USING GIST (geom);
In the example above, a spatial index named “points_geom_idx” is created on the “geom” column of the “points” table.
Understanding the R-tree Index in PostgreSQL for Efficient Spatial Queries
The R-tree index is a data structure used in PostgreSQL to efficiently index and query spatial data. It is specifically designed for spatial indexing and provides excellent performance for spatial queries.
The R-tree index organizes spatial objects into a tree structure, where each node represents a bounding box that encloses a group of objects. The bounding boxes are recursively split and grouped together to form the tree structure. This allows for efficient spatial search operations by narrowing down the search space based on the bounding boxes.
Here’s an example to illustrate the concept of the R-tree index:
-- Create a table with a geometry column CREATE TABLE cities ( id SERIAL PRIMARY KEY, name VARCHAR(100), location GEOMETRY(Point, 4326) ); -- Create an R-tree index on the "location" column CREATE INDEX cities_location_idx ON cities USING GIST (location);
In the example above, we create a table named “cities” with a geometry column “location” to store the spatial data. We then create an R-tree index named “cities_location_idx” on the “location” column using the “CREATE INDEX” statement with the “USING GIST” option.
Now let’s consider a query that finds all cities within a certain distance from a given point:
SELECT * FROM cities WHERE ST_DWithin( location, ST_SetSRID(ST_Point(42.3601, -71.0589), 4326), 1000 );
The “ST_DWithin” function checks if the distance between each city’s location and the given point is within the specified distance (1000 units in this case). The R-tree index on the “location” column allows PostgreSQL to efficiently narrow down the search space and retrieve the relevant cities, resulting in faster query execution.
The R-tree index in PostgreSQL is suitable for both the “geometry” and “geography” data types. However, it is important to note that the R-tree index is most effective when the objects being indexed have a similar size. If the objects vary significantly in size, such as having a large variation in area or extent, the R-tree index may not perform optimally.
Overall, the R-tree index in PostgreSQL provides an efficient and scalable solution for spatial indexing and querying, making it a valuable tool for working with geospatial data.
Related Article: How to Check if a Table Exists in PostgreSQL
Performing KNN Search in PostgreSQL
K-Nearest Neighbor (KNN) search is a common spatial query operation that finds the K nearest spatial objects to a given point. This type of query is useful in various applications, such as finding the nearest store, restaurant, or point of interest.
PostgreSQL provides support for KNN search through its PostGIS extension. With PostGIS, you can perform KNN search queries efficiently using the KNN operators and functions.
To perform a KNN search in PostgreSQL, follow these steps:
1. Ensure that you have PostGIS installed and enabled in your PostgreSQL database.
2. Create a table with a geometry column to store the spatial data. For example:
CREATE TABLE points ( id SERIAL PRIMARY KEY, name VARCHAR(100), location GEOMETRY(Point, 4326) );
3. Insert some data into the table:
INSERT INTO points (name, location) VALUES ('Point A', ST_SetSRID(ST_Point(42.3601, -71.0589), 4326)), ('Point B', ST_SetSRID(ST_Point(42.3612, -71.0571), 4326)), ('Point C', ST_SetSRID(ST_Point(42.3594, -71.0597), 4326)), ('Point D', ST_SetSRID(ST_Point(42.3628, -71.0578), 4326));
4. Create an index on the geometry column for efficient KNN search:
CREATE INDEX points_location_idx ON points USING GIST (location);
5. Perform a KNN search query to find the K nearest points to a given location:
SELECT * FROM points ORDER BY location <-> ST_SetSRID(ST_Point(42.3601, -71.0589), 4326) LIMIT 3;
In the example above, the “” operator is used to calculate the distance between each point’s location and the given location. The query orders the points by distance in ascending order and limits the result to the top 3 nearest points.
Finding Nearest Neighbors in PostgreSQL
Finding the nearest neighbors of a given spatial object is a common spatial query operation that can be efficiently performed in PostgreSQL with the help of spatial indexes and functions provided by the PostGIS extension.
To find the nearest neighbors in PostgreSQL, follow these steps:
1. Ensure that you have PostGIS installed and enabled in your PostgreSQL database.
2. Create a table with a geometry column to store the spatial data. For example:
CREATE TABLE points ( id SERIAL PRIMARY KEY, name VARCHAR(100), location GEOMETRY(Point, 4326) );
3. Insert some data into the table:
INSERT INTO points (name, location) VALUES ('Point A', ST_SetSRID(ST_Point(42.3601, -71.0589), 4326)), ('Point B', ST_SetSRID(ST_Point(42.3612, -71.0571), 4326)), ('Point C', ST_SetSRID(ST_Point(42.3594, -71.0597), 4326)), ('Point D', ST_SetSRID(ST_Point(42.3628, -71.0578), 4326));
4. Create an index on the geometry column for efficient nearest neighbor search:
CREATE INDEX points_location_idx ON points USING GIST (location);
5. Perform a nearest neighbor search query to find the nearest neighbors of a given point:
SELECT * FROM points ORDER BY location <-> ST_SetSRID(ST_Point(42.3601, -71.0589), 4326) LIMIT 3;
In the example above, the “” operator is used to calculate the distance between each point’s location and the given point’s location. The query orders the points by distance in ascending order and limits the result to the top 3 nearest neighbors.
Differences Between the Geography and Geometry Datatypes in PostgreSQL
PostgreSQL provides two main datatypes for storing and querying geospatial data: “geometry” and “geography”. While both datatypes are used to represent spatial objects, they have some key differences in terms of their usage and underlying representation.
1. Geometry Datatype:
The “geometry” datatype in PostgreSQL is used to store 2D geometric objects such as points, lines, and polygons. It operates in a Cartesian coordinate system and does not take into account the curvature of the Earth.
Geometry objects can be defined in various coordinate systems, including Cartesian (X, Y) or geographic (longitude, latitude) coordinates. They can also be transformed between different coordinate systems using functions provided by the PostGIS extension.
The “geometry” datatype is suitable for representing objects on a flat surface, such as buildings, roads, or city boundaries. It provides precise geometric calculations and supports a wide range of spatial operations, such as intersection, distance calculation, and area calculation.
Here’s an example of creating a table with a “geometry” column in PostgreSQL:
CREATE TABLE buildings ( id SERIAL PRIMARY KEY, name VARCHAR(100), location GEOMETRY(Point, 4326) );
2. Geography Datatype:
The “geography” datatype in PostgreSQL is used to store geospatial data in a geographic coordinate system, such as latitude and longitude. It takes into account the curvature of the Earth and provides accurate distance and area calculations.
Geography objects are defined in a spherical coordinate system and can represent objects on the Earth’s surface. The “geography” datatype supports various geodetic operations, such as calculating distances along the Earth’s surface and finding the shortest path between two points.
The “geography” datatype is suitable for representing objects that span a large area, such as continents, countries, or natural features. It provides accurate spatial calculations that take into account the Earth’s shape and can be used for various geospatial analysis tasks.
Here’s an example of creating a table with a “geography” column in PostgreSQL:
CREATE TABLE countries ( id SERIAL PRIMARY KEY, name VARCHAR(100), boundary GEOGRAPHY(Polygon, 4326) );
– The “geometry” datatype operates in a Cartesian coordinate system, while the “geography” datatype operates in a geographic coordinate system.
– The “geometry” datatype does not take into account the Earth’s curvature, while the “geography” datatype provides accurate calculations that consider the Earth’s shape.
– The “geometry” datatype is suitable for representing objects on a flat surface, while the “geography” datatype is suitable for representing objects on the Earth’s surface.
The choice between the “geometry” and “geography” datatypes depends on the specific use case and the requirements of the spatial data being stored and queried.
Related Article: Applying Aggregate Functions in PostgreSQL WHERE Clause
Utilizing Bounding Boxes in Spatial Queries with PostgreSQL
Bounding boxes are a useful concept in spatial queries that can significantly improve the efficiency of query execution. A bounding box, also known as an envelope, is a rectangular area that completely encloses a spatial object. By utilizing bounding boxes, you can quickly filter out irrelevant objects and reduce the search space for spatial queries.
PostgreSQL, with the help of the PostGIS extension, provides functions for creating and working with bounding boxes. These functions allow you to generate bounding boxes for spatial objects, check if two bounding boxes intersect or contain each other, and use bounding boxes to optimize spatial queries.
To utilize bounding boxes in spatial queries with PostgreSQL, follow these steps:
1. Ensure that you have PostGIS installed and enabled in your PostgreSQL database.
2. Create a table with a geometry column to store the spatial data. For example:
CREATE TABLE buildings ( id SERIAL PRIMARY KEY, name VARCHAR(100), location GEOMETRY(Polygon, 4326) );
3. Insert some data into the table:
INSERT INTO buildings (name, location) VALUES ('Building A', ST_SetSRID(ST_MakeEnvelope(10, 10, 20, 20), 4326)), ('Building B', ST_SetSRID(ST_MakeEnvelope(15, 15, 25, 25), 4326)), ('Building C', ST_SetSRID(ST_MakeEnvelope(30, 30, 40, 40), 4326));
In the example above, we create a table named “buildings” with a geometry column “location” to store the spatial data. We then insert some buildings into the table, each represented by a bounding box using the “ST_MakeEnvelope” function.
4. Perform a spatial query using bounding boxes:
SELECT * FROM buildings WHERE location && ST_SetSRID(ST_MakeEnvelope(5, 5, 15, 15), 4326);
In the example above, the “&&” operator checks if the bounding box of each building’s location intersects with the specified bounding box. The query retrieves all buildings whose bounding boxes intersect with the specified bounding box.
Exploring Different Types of Geometries in Spatial Queries with PostgreSQL
PostgreSQL, with the help of the PostGIS extension, provides support for various types of geometries that can be used in spatial queries. These geometry types allow you to represent different spatial objects, such as points, lines, polygons, and more.
Here are some commonly used geometry types in PostgreSQL:
1. Point:
The “Point” geometry type represents a single point in a Cartesian coordinate system. It consists of X and Y coordinates that define the position of the point.
To create a point in PostgreSQL, you can use the “ST_Point” function. Here’s an example:
SELECT ST_Point(1, 2);
The example above creates a point with X coordinate 1 and Y coordinate 2.
2. LineString:
The “LineString” geometry type represents a sequence of connected line segments. It can be used to represent lines, curves, or any other continuous path.
To create a LineString in PostgreSQL, you can use the “ST_LineString” function. Here’s an example:
SELECT ST_LineString(ARRAY[ST_Point(1, 2), ST_Point(3, 4), ST_Point(5, 6)]);
The example above creates a LineString that consists of three points: (1, 2), (3, 4), and (5, 6).
3. Polygon:
The “Polygon” geometry type represents a closed shape with straight edges. It is defined by an outer ring and zero or more inner rings. Each ring is a sequence of points that define the vertices of the polygon.
To create a polygon in PostgreSQL, you can use the “ST_Polygon” function. Here’s an example:
SELECT ST_Polygon( ARRAY[ST_Point(0, 0), ST_Point(0, 5), ST_Point(5, 5), ST_Point(5, 0), ST_Point(0, 0)] );
The example above creates a square polygon with vertices at (0, 0), (0, 5), (5, 5), and (5, 0).
4. MultiPoint, MultiLineString, MultiPolygon:
PostgreSQL also provides support for multi-geometries, which allow you to represent collections of points, line strings, or polygons.
To create a multi-geometry in PostgreSQL, you can use the “ST_MultiPoint”, “ST_MultiLineString”, or “ST_MultiPolygon” function. Here’s an example of creating a MultiPoint:
SELECT ST_MultiPoint(ARRAY[ST_Point(1, 2), ST_Point(3, 4)]);
The example above creates a MultiPoint that consists of two points: (1, 2) and (3, 4).
Performing Spatial Queries to Find Points within a Certain Distance in PostgreSQL
Spatial queries that involve finding points within a certain distance from a given location are common in geospatial applications. PostgreSQL, with the help of the PostGIS extension, provides functions and operators that allow you to perform such queries efficiently.
To perform a spatial query to find points within a certain distance in PostgreSQL, follow these steps:
1. Ensure that you have PostGIS installed and enabled in your PostgreSQL database.
2. Create a table with a geometry column to store the spatial data. For example:
CREATE TABLE points ( id SERIAL PRIMARY KEY, name VARCHAR(100), location GEOMETRY(Point, 4326) );
3. Insert some data into the table:
INSERT INTO points (name, location) VALUES ('Point A', ST_SetSRID(ST_Point(42.3601, -71.0589), 4326)), ('Point B', ST_SetSRID(ST_Point(42.3612, -71.0571), 4326)), ('Point C', ST_SetSRID(ST_Point(42.3594, -71.0597), 4326)), ('Point D', ST_SetSRID(ST_Point(42.3628, -71.0578), 4326));
In the example above, we create a table named “points” with a geometry column “location” to store the spatial data. We then insert some points into the table using the “ST_SetSRID” and “ST_Point” functions.
4. Perform a spatial query to find points within a certain distance:
SELECT * FROM points WHERE ST_DWithin( location, ST_SetSRID(ST_Point(42.3601, -71.0589), 4326), 1000 );
In the example above, the “ST_DWithin” function checks if each point’s location is within the specified distance (1000 units in this case) from the given location. The query retrieves all points that satisfy this condition.
Related Article: How to Convert Columns to Rows in PostgreSQL
Additional Resources
– What is Spatial Indexing and How Does It Improve Query Performance?
– Bounding Box Query to Find Objects within a Specific Area