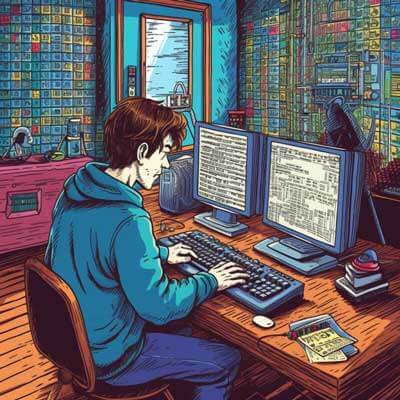
Table of Contents
Understanding the ETL Process
The ETL (Extract, Transform, Load) process is a common data integration technique used to extract data from various sources, transform it into a suitable format, and load it into a target system for analysis or further processing. In the context of PostgreSQL databases, the ETL process involves extracting data from the database, transforming it if necessary, and loading it into another system or application.
The ETL process typically consists of the following steps:
1. Extraction: Data is extracted from the source system, in this case, a PostgreSQL database. This can involve querying the database directly or using specialized tools or frameworks.
2. Transformation: The extracted data is transformed to meet the requirements of the target system. This may include cleaning and filtering the data, aggregating or summarizing it, or performing calculations or calculations.
3. Loading: The transformed data is loaded into the target system, which can be another database, a data warehouse, a reporting tool, or an analytics platform.
Let's look at an example of extracting data from a PostgreSQL database using Python:
import psycopg2 # Connect to the PostgreSQL database conn = psycopg2.connect(database="mydatabase", user="myuser", password="mypassword", host="localhost", port="5432") # Create a cursor object to execute SQL queries cur = conn.cursor() # Execute a SELECT query to retrieve data cur.execute("SELECT * FROM mytable") # Fetch all the rows returned by the query rows = cur.fetchall() # Iterate over the rows and print the data for row in rows: print(row) # Close the cursor and the connection cur.close() conn.close()
In this example, we use the psycopg2 library to connect to a PostgreSQL database, execute a SELECT query to retrieve data from the "mytable" table, and print the results.
Related Article: How to Use Nested Queries in Databases
Exploring Database Extraction Techniques
There are several techniques and tools available for extracting data from PostgreSQL databases. Let's explore a few of them:
1. SQL Queries: The most basic and common method of extracting data from a PostgreSQL database is by writing SQL queries. You can use the SELECT statement to retrieve specific data from one or more tables based on your requirements. SQL queries provide flexibility and control over the extraction process.
Here's an example of extracting data using a SQL query:
SELECT * FROM mytable WHERE column = 'value'
This query retrieves all rows from the "mytable" table where the value in the "column" column matches the specified value.
2. pg_dump: The pg_dump command-line tool is another popular method for extracting data from PostgreSQL databases. It creates a binary backup of the database or specific tables, which can be restored later using the pg_restore command.
To extract data using pg_dump, you can run the following command:
pg_dump -U username -d dbname -t tablename > backup.sql
This command creates a backup file named "backup.sql" containing the data from the specified table.
3. ETL Tools: There are also dedicated ETL (Extract, Transform, Load) tools available that provide a graphical interface for extracting data from PostgreSQL databases. These tools allow you to define extraction rules, apply transformations, and load the data into various target systems.
Some popular ETL tools for PostgreSQL include Talend, Pentaho Data Integration, and Apache Nifi.
Data Migration in PostgreSQL
Data migration is the process of transferring data from one system to another, typically involving a change in the underlying data model or structure. In the context of PostgreSQL databases, data migration can be performed using various techniques and tools.
One common approach to data migration in PostgreSQL is to use the pg_dump and pg_restore commands. The pg_dump command creates a binary backup of the database, including its schema and data, while the pg_restore command restores the backup to a new or existing database.
Here's an example of performing a data migration using pg_dump and pg_restore:
1. Create a backup of the source database:
pg_dump -U username -d sourcedb > backup.sql
This command creates a backup file named "backup.sql" containing the data from the source database.
2. Restore the backup to the target database:
pg_restore -U username -d targetdb backup.sql
This command restores the backup file to the target database, effectively migrating the data from the source to the target.
It's important to note that data migration may involve additional steps, such as mapping or transforming the data to match the target schema or structure. This can be done using SQL queries or ETL tools, depending on the complexity of the migration.
Exporting a PostgreSQL Database
Exporting a PostgreSQL database involves creating a backup of the database in a format that can be easily transferred or imported into another system. There are several methods and tools available for exporting PostgreSQL databases.
One common method is to use the pg_dump command-line tool, which creates a binary backup of the database. The backup file can then be transferred to another system and restored using the pg_restore command.
Here's an example of exporting a PostgreSQL database using pg_dump:
pg_dump -U username -d dbname > backup.sql
This command creates a backup file named "backup.sql" containing the schema and data of the specified database.
Another method for exporting a PostgreSQL database is to use the COPY command. The COPY command allows you to export data from a table to a file in various formats, such as CSV or JSON. This can be useful when you only need to export specific tables or a subset of the data.
Here's an example of exporting data from a PostgreSQL table using the COPY command:
COPY mytable TO '/path/to/export.csv' CSV HEADER;
This command exports the data from the "mytable" table to a CSV file named "export.csv" with a header row.
In addition to these methods, there are also third-party tools and graphical interfaces available for exporting PostgreSQL databases, such as pgAdmin and DBeaver.
Related Article: Tutorial: Modulo Operator in PostgreSQL Databases
Performing Data Extraction in PostgreSQL
Performing data extraction in PostgreSQL involves retrieving data from one or more tables based on specific criteria or conditions. This can be done using SQL queries, which allow you to filter, sort, and aggregate the data as needed.
Let's look at some examples of data extraction in PostgreSQL:
1. Basic Data Extraction:
To extract all rows from a table, you can use the following SQL query:
SELECT * FROM mytable;
This query retrieves all columns and rows from the "mytable" table.
2. Filtering Data:
To extract rows that meet specific criteria, you can use the WHERE clause in your SQL query. For example, to extract all rows where the value in the "column" column is equal to 'value', you can use the following query:
SELECT * FROM mytable WHERE column = 'value';
This query retrieves all rows from the "mytable" table where the value in the "column" column matches the specified value.
3. Aggregating Data:
To extract aggregated data, such as the sum, average, or count of a column, you can use aggregate functions in your SQL query. For example, to extract the total sum of the "amount" column in the "orders" table, you can use the following query:
SELECT SUM(amount) FROM orders;
This query returns the sum of all values in the "amount" column.
These examples demonstrate the basic concepts of data extraction in PostgreSQL. Depending on your specific requirements, you can use various SQL clauses, functions, and techniques to extract and manipulate data from PostgreSQL databases.
Tools for PostgreSQL Data Extraction
There are several tools available for extracting data from PostgreSQL databases, ranging from command-line utilities to graphical interfaces. These tools provide different features and capabilities, allowing you to extract data in various formats and perform complex extraction tasks.
Here are some popular tools for PostgreSQL data extraction:
1. pg_dump and pg_restore: As mentioned earlier, pg_dump and pg_restore are command-line tools provided by PostgreSQL for creating backups and restoring them. These tools are widely used for basic data extraction tasks.
2. Talend Open Studio: Talend Open Studio is a useful open-source ETL (Extract, Transform, Load) tool that supports PostgreSQL as a data source. It provides a graphical interface for designing data extraction workflows and offers a wide range of features for data integration and transformation.
3. Pentaho Data Integration: Pentaho Data Integration, also known as Kettle, is another popular open-source ETL tool that supports PostgreSQL. It allows you to visually design data extraction processes and provides extensive support for data integration, transformation, and loading.
4. Apache Nifi: Apache Nifi is a data integration tool that provides a web-based interface for designing and executing data flows. It supports PostgreSQL as a data source and offers a wide range of processors for extracting, transforming, and loading data.
These are just a few examples of tools available for PostgreSQL data extraction. Depending on your specific requirements and preferences, you can choose the tool that best fits your needs.
Replicating Data in PostgreSQL
Replication in PostgreSQL is the process of creating and maintaining a replica (copy) of a database on another system. Replication is commonly used for various purposes, such as improving performance, increasing availability, or facilitating data analysis and reporting.
There are several replication methods available in PostgreSQL, including:
1. Streaming Replication: Streaming replication is the most common method of replication in PostgreSQL. It uses a primary-secondary (master-slave) architecture, where changes made to the primary database are streamed (replicated) to one or more secondary databases in near real-time.
To enable streaming replication, you need to configure the primary and secondary servers and set up a replication slot. The primary server continuously streams the changes to the secondary server(s), which can be used for read-only queries or as a failover mechanism in case the primary server fails.
2. Logical Replication: Logical replication is a newer replication method introduced in PostgreSQL 10. It allows you to replicate individual tables or specific sets of data based on defined replication rules. Unlike streaming replication, which replicates the entire database, logical replication provides more flexibility and granularity.
To set up logical replication, you need to configure the primary and replica servers and define replication publications and subscriptions. The primary server publishes changes to the replica server(s) based on the defined rules, allowing you to replicate only the data you need.
3. Third-Party Replication Solutions: In addition to the built-in replication methods, there are also third-party replication solutions available for PostgreSQL, such as Bucardo and Slony-I. These tools provide additional features and flexibility for replicating data in PostgreSQL.
It's important to note that replication in PostgreSQL is not limited to a single master-slave relationship. You can set up cascading replication, where a secondary server acts as both a replica of the primary server and a master for additional secondary servers.
Synchronizing Data between PostgreSQL Databases
Synchronizing data between PostgreSQL databases involves keeping the data in multiple databases consistent and up to date. This can be necessary when you have multiple database instances that need to share the same data or when you need to merge changes made in different databases.
There are several methods and tools available for synchronizing data between PostgreSQL databases:
1. Trigger-Based Replication: Trigger-based replication is a method where you use database triggers to capture changes made in one database and replicate them to another database. Whenever a change (insert, update, delete) is made in the source database, the trigger captures the change and applies it to the target database.
To set up trigger-based replication, you need to create triggers on the source tables to capture the changes and write custom logic to apply the changes to the target tables. This method requires careful planning and implementation to ensure consistency and avoid conflicts.
2. Logical Replication: As mentioned earlier, logical replication is a built-in replication method in PostgreSQL that allows you to replicate individual tables or specific sets of data. Logical replication can be used for synchronizing data between databases by defining replication publications and subscriptions.
To synchronize data using logical replication, you need to configure the primary and replica servers, define the replication publications on the primary server, and create replication subscriptions on the replica server(s). The primary server publishes changes to the replica server(s) based on the defined rules, keeping the data in sync.
3. Third-Party Data Integration Tools: There are also third-party data integration tools available that provide synchronization capabilities for PostgreSQL databases. These tools allow you to define data synchronization workflows, map the data between databases, and schedule the synchronization process.
Some popular data integration tools for PostgreSQL include Talend Open Studio, Pentaho Data Integration, and Apache Nifi.
These methods and tools provide different levels of flexibility and control over the data synchronization process. Depending on your specific requirements, you can choose the method or tool that best fits your needs.
Related Article: How to Create a PostgreSQL Read Only User
Performing ETL in PostgreSQL
Performing ETL (Extract, Transform, Load) in PostgreSQL involves extracting data from one or more sources, transforming it to meet the requirements of the target system, and loading it into PostgreSQL for further analysis or processing.
There are several approaches and tools available for performing ETL in PostgreSQL:
1. SQL Queries: The most basic approach to ETL in PostgreSQL is by writing SQL queries to extract, transform, and load the data. You can use the SELECT statement to extract data from the source system, apply transformations using SQL functions and expressions, and use the INSERT statement to load the transformed data into PostgreSQL.
Here's an example of performing ETL in PostgreSQL using SQL queries:
-- Extract data from the source system SELECT * FROM sourcedb.sourcetable; -- Transform the data SELECT column1, column2 + column3 AS transformed_column FROM sourcedb.sourcetable; -- Load the transformed data into PostgreSQL INSERT INTO targetdb.targettable (column1, transformed_column) VALUES ('value1', 123);
2. ETL Tools: There are also dedicated ETL tools available that provide a graphical interface for designing and executing ETL workflows. These tools allow you to define data extraction rules, apply transformations, and load the data into PostgreSQL or other target systems.
Some popular ETL tools for PostgreSQL include Talend Open Studio, Pentaho Data Integration, and Apache Nifi.
Here's an example of performing ETL in PostgreSQL using Talend Open Studio:
1. Define the data extraction rule to extract data from the source system.
2. Apply transformations to the extracted data, such as cleaning, filtering, aggregating, or joining.
3. Load the transformed data into PostgreSQL using the PostgreSQL output component.
These are just a few examples of how you can perform ETL in PostgreSQL. The approach and tools you choose will depend on your specific requirements, data sources, and data volumes.
Backing up a PostgreSQL Database
Backing up a PostgreSQL database is essential to protect your data from accidental loss, hardware failures, or other unforeseen events. There are several methods and tools available for backing up PostgreSQL databases.
One common method is to use the pg_dump command-line tool, which creates a binary backup of the database. The backup file can then be stored on a separate storage device or transferred to another system for safekeeping.
Here's an example of backing up a PostgreSQL database using pg_dump:
pg_dump -U username -d dbname > backup.sql
This command creates a backup file named "backup.sql" containing the schema and data of the specified database.
Another method for backing up a PostgreSQL database is to use the pg_basebackup command-line tool. This tool creates a physical backup of the entire database cluster, including the data directory and configuration files. The backup can then be restored to a new or existing PostgreSQL installation.
Here's an example of backing up a PostgreSQL database using pg_basebackup:
pg_basebackup -U username -D /path/to/backup/directory -F t -X stream -Z 6
This command creates a tar-format backup of the database cluster and compresses it using gzip with a compression level of 6. The backup is stored in the specified directory.
In addition to these methods, there are also third-party tools and graphical interfaces available for backing up PostgreSQL databases, such as pgAdmin and Barman.
It's important to regularly schedule backups and store them in a secure location to ensure the availability and integrity of your data. Additionally, consider implementing a backup retention policy to manage disk space and optimize backup and restore times.