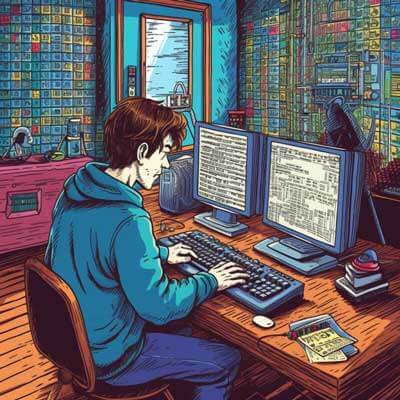
Table of Contents
Inner Join in PostgreSQL
In PostgreSQL, an inner join is used to combine rows from two or more tables based on a related column between them. The result of an inner join includes only the rows that have matching values in both tables.
Consider the following example where we have two tables, "customers" and "orders":
CREATE TABLE customers ( customer_id INT PRIMARY KEY, customer_name VARCHAR(100), city VARCHAR(50));CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT, order_date DATE, total_amount DECIMAL(10,2), FOREIGN KEY (customer_id) REFERENCES customers(customer_id));INSERT INTO customers (customer_id, customer_name, city)VALUES (1, 'John Doe', 'New York'), (2, 'Jane Smith', 'Los Angeles'), (3, 'Michael Johnson', 'Chicago');INSERT INTO orders (order_id, customer_id, order_date, total_amount)VALUES (101, 1, '2022-01-01', 100.00), (102, 2, '2022-01-02', 200.00), (103, 3, '2022-01-03', 300.00);
To perform an inner join on the "customers" and "orders" tables based on the "customer_id" column, you can use the following SQL query:
SELECT customers.customer_name, orders.order_date, orders.total_amountFROM customersINNER JOIN orders ON customers.customer_id = orders.customer_id;
This query will return the customer name, order date, and total amount for each order, where the customer ID matches between the two tables.
Related Article: How to Disable IPv6 in PostgreSQL Databases
Outer Join in PostgreSQL
In PostgreSQL, an outer join is used to combine rows from two or more tables, including unmatched rows from one or both tables. There are three types of outer joins in PostgreSQL: left outer join, right outer join, and full outer join.
A left outer join returns all rows from the left table, and the matched rows from the right table. If there is no match, NULL values are returned for the columns of the right table.
Consider the following example where we have two tables, "departments" and "employees":
CREATE TABLE departments ( department_id INT PRIMARY KEY, department_name VARCHAR(100));CREATE TABLE employees ( employee_id INT PRIMARY KEY, employee_name VARCHAR(100), department_id INT, FOREIGN KEY (department_id) REFERENCES departments(department_id));INSERT INTO departments (department_id, department_name)VALUES (1, 'Sales'), (2, 'Marketing'), (3, 'Finance');INSERT INTO employees (employee_id, employee_name, department_id)VALUES (101, 'John Doe', 1), (102, 'Jane Smith', 2), (103, 'Michael Johnson', NULL);
To perform a left outer join on the "departments" and "employees" tables based on the "department_id" column, you can use the following SQL query:
SELECT departments.department_name, employees.employee_nameFROM departmentsLEFT OUTER JOIN employees ON departments.department_id = employees.department_id;
This query will return the department name and employee name for each employee, including those without a department (NULL value for department_id).
Cross Join in PostgreSQL
In PostgreSQL, a cross join (also known as a Cartesian join) is used to combine every row from one table with every row from another table. It generates a result set with the total number of rows equal to the product of the number of rows in each table.
Consider the following example where we have two tables, "colors" and "sizes":
CREATE TABLE colors ( color_id INT PRIMARY KEY, color_name VARCHAR(100));CREATE TABLE sizes ( size_id INT PRIMARY KEY, size_name VARCHAR(100));INSERT INTO colors (color_id, color_name)VALUES (1, 'Red'), (2, 'Green');INSERT INTO sizes (size_id, size_name)VALUES (1, 'Small'), (2, 'Large');
To perform a cross join on the "colors" and "sizes" tables, you can use the following SQL query:
SELECT colors.color_name, sizes.size_nameFROM colorsCROSS JOIN sizes;
This query will return all possible combinations of color names and size names, resulting in a total of 4 rows.
Equijoin in PostgreSQL
In PostgreSQL, an equijoin is a type of join that combines rows from two or more tables based on equality between values in the specified columns. It is the most common type of join used in database queries.
Consider the following example where we have two tables, "employees" and "departments":
CREATE TABLE employees ( employee_id INT PRIMARY KEY, employee_name VARCHAR(100), department_id INT);CREATE TABLE departments ( department_id INT PRIMARY KEY, department_name VARCHAR(100));INSERT INTO employees (employee_id, employee_name, department_id)VALUES (101, 'John Doe', 1), (102, 'Jane Smith', 2), (103, 'Michael Johnson', 1);INSERT INTO departments (department_id, department_name)VALUES (1, 'Sales'), (2, 'Marketing');
To perform an equijoin on the "employees" and "departments" tables based on the "department_id" column, you can use the following SQL query:
SELECT employees.employee_name, departments.department_nameFROM employeesINNER JOIN departments ON employees.department_id = departments.department_id;
This query will return the employee name and department name for each employee, where the department ID matches between the two tables.
Related Article: Monitoring the PostgreSQL Service Health
Self Join in PostgreSQL
In PostgreSQL, a self join is a type of join where a table is joined with itself. It is useful when you want to combine rows from the same table based on a related column.
Consider the following example where we have a "employees" table that contains information about employees and their managers:
CREATE TABLE employees ( employee_id INT PRIMARY KEY, employee_name VARCHAR(100), manager_id INT);INSERT INTO employees (employee_id, employee_name, manager_id)VALUES (101, 'John Doe', 102), (102, 'Jane Smith', NULL), (103, 'Michael Johnson', 102);
To perform a self join on the "employees" table to get the manager names for each employee, you can use the following SQL query:
SELECT e.employee_name AS employee_name, m.employee_name AS manager_nameFROM employees eLEFT JOIN employees m ON e.manager_id = m.employee_id;
This query will return the employee name and manager name for each employee, including those without a manager (NULL value for manager_id).
Join Condition in PostgreSQL
In PostgreSQL, a join condition is used to specify the relationship between tables in a join operation. It defines how the rows from the tables should be combined based on the values of the specified columns.
The join condition is specified in the ON clause of the join statement. It typically includes an equality comparison between the columns that represent the relationship between the tables.
Consider the following example where we have two tables, "customers" and "orders":
CREATE TABLE customers ( customer_id INT PRIMARY KEY, customer_name VARCHAR(100), city VARCHAR(50));CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT, order_date DATE, total_amount DECIMAL(10,2), FOREIGN KEY (customer_id) REFERENCES customers(customer_id));
To join the "customers" and "orders" tables based on the "customer_id" column, the join condition would be:
customers.customer_id = orders.customer_id
This join condition ensures that only the rows with matching customer IDs are combined in the result.
Joining Two Tables in PostgreSQL
In PostgreSQL, joining two tables involves combining rows from each table based on a related column. This allows you to retrieve data from multiple tables in a single query.
To join two tables in PostgreSQL, you can use the JOIN keyword followed by the name of the second table and the ON keyword to specify the join condition. The join condition determines how the rows from the two tables should be combined.
Consider the following example where we have two tables, "customers" and "orders":
CREATE TABLE customers ( customer_id INT PRIMARY KEY, customer_name VARCHAR(100), city VARCHAR(50));CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT, order_date DATE, total_amount DECIMAL(10,2), FOREIGN KEY (customer_id) REFERENCES customers(customer_id));
To join the "customers" and "orders" tables based on the "customer_id" column, you can use the following SQL query:
SELECT customers.customer_name, orders.order_date, orders.total_amountFROM customersJOIN orders ON customers.customer_id = orders.customer_id;
This query will return the customer name, order date, and total amount for each order, where the customer ID matches between the two tables.
Types of Joins in PostgreSQL
In PostgreSQL, there are several types of joins that can be used to combine rows from two or more tables. The common types of joins include inner join, outer join, cross join, and self join.
- Inner Join: An inner join returns only the rows that have matching values in both tables.
- Outer Join: An outer join returns all rows from one table and the matched rows from the other table(s), including unmatched rows with NULL values.
- Cross Join: A cross join combines every row from one table with every row from another table, resulting in a Cartesian product.
- Self Join: A self join is used to join a table with itself, typically to combine rows based on a related column.
The choice of join type depends on the specific requirements of the query and the relationship between the tables.
Related Article: Tutorial: Using Navicat for PostgreSQL Database Management
Join Operator in PostgreSQL
In PostgreSQL, the join operator is used to combine rows from two or more tables based on a related column. The join operator is represented by the keyword "JOIN" and is typically used in conjunction with the "ON" keyword to specify the join condition.
Consider the following example where we have two tables, "employees" and "departments":
CREATE TABLE employees ( employee_id INT PRIMARY KEY, employee_name VARCHAR(100), department_id INT);CREATE TABLE departments ( department_id INT PRIMARY KEY, department_name VARCHAR(100));
To perform an inner join on the "employees" and "departments" tables based on the "department_id" column, you can use the following SQL query:
SELECT employees.employee_name, departments.department_nameFROM employeesJOIN departments ON employees.department_id = departments.department_id;
In this query, the join operator "JOIN" combines the rows from the "employees" and "departments" tables based on the equality of the "department_id" column.
Join Column in PostgreSQL
In PostgreSQL, a join column is a column or a set of columns used to establish a relationship between tables in a join operation. The join column is typically a primary key or a foreign key column that contains matching values between the tables.
Consider the following example where we have two tables, "customers" and "orders":
CREATE TABLE customers ( customer_id INT PRIMARY KEY, customer_name VARCHAR(100), city VARCHAR(50));CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT, order_date DATE, total_amount DECIMAL(10,2), FOREIGN KEY (customer_id) REFERENCES customers(customer_id));
In this example, the "customer_id" column in the "customers" table is the join column that establishes the relationship between the "customers" and "orders" tables. It is used to combine the rows from the two tables based on the matching customer IDs.
Difference Between Natural Join and Inner Join in PostgreSQL
In PostgreSQL, both natural join and inner join are used to combine rows from two or more tables based on a related column. However, there are some differences between the two.
An inner join returns only the rows that have matching values in both tables based on the specified join condition. It requires explicitly specifying the join condition using the "ON" keyword.
On the other hand, a natural join is a type of inner join that automatically matches the columns with the same name in the two tables. It does not require specifying the join condition explicitly.
Consider the following example where we have two tables, "employees" and "departments":
CREATE TABLE employees ( employee_id INT PRIMARY KEY, employee_name VARCHAR(100), department_id INT);CREATE TABLE departments ( department_id INT PRIMARY KEY, department_name VARCHAR(100));
To perform an inner join on the "employees" and "departments" tables based on the "department_id" column, you can use the following SQL query:
SELECT employees.employee_name, departments.department_nameFROM employeesINNER JOIN departments ON employees.department_id = departments.department_id;
To perform a natural join on the same tables, you can use the following SQL query:
SELECT employee_name, department_nameFROM employeesNATURAL JOIN departments;
In this query, the natural join automatically matches the "department_id" column in the two tables without explicitly specifying the join condition.
Working of Outer Join in PostgreSQL
In PostgreSQL, an outer join is used to combine rows from two or more tables, including unmatched rows from one or both tables. It allows you to retrieve data even if there is no matching value in the join column.
There are three types of outer joins in PostgreSQL: left outer join, right outer join, and full outer join.
- Left Outer Join: A left outer join returns all rows from the left table and the matched rows from the right table. If there is no match, NULL values are returned for the columns of the right table.
- Right Outer Join: A right outer join returns all rows from the right table and the matched rows from the left table. If there is no match, NULL values are returned for the columns of the left table.
- Full Outer Join: A full outer join returns all rows from both tables, including unmatched rows from either table. If there is no match, NULL values are returned for the columns of the other table.
Consider the following example where we have two tables, "departments" and "employees":
CREATE TABLE departments ( department_id INT PRIMARY KEY, department_name VARCHAR(100));CREATE TABLE employees ( employee_id INT PRIMARY KEY, employee_name VARCHAR(100), department_id INT, FOREIGN KEY (department_id) REFERENCES departments(department_id));
To perform a left outer join on the "departments" and "employees" tables based on the "department_id" column, you can use the following SQL query:
SELECT departments.department_name, employees.employee_nameFROM departmentsLEFT OUTER JOIN employees ON departments.department_id = employees.department_id;
This query will return the department name and employee name for each employee, including those without a department (NULL value for department_id).
Related Article: How to Truncate Tables in PostgreSQL
Cross Join and its Usage in PostgreSQL
In PostgreSQL, a cross join (also known as a Cartesian join) is used to combine every row from one table with every row from another table. It generates a result set with the total number of rows equal to the product of the number of rows in each table.
A cross join can be useful in scenarios where you need to generate all possible combinations of rows from two or more tables. However, it can also result in a large number of rows if the tables have a significant number of rows.
Consider the following example where we have two tables, "colors" and "sizes":
CREATE TABLE colors ( color_id INT PRIMARY KEY, color_name VARCHAR(100));CREATE TABLE sizes ( size_id INT PRIMARY KEY, size_name VARCHAR(100));
To perform a cross join on the "colors" and "sizes" tables, you can use the following SQL query:
SELECT colors.color_name, sizes.size_nameFROM colorsCROSS JOIN sizes;
This query will return all possible combinations of color names and size names, resulting in a total of 4 rows.
Cross joins should be used with caution as they can quickly generate a large number of rows. It is important to consider the size of the tables and the desired result before using a cross join.
Equijoin vs Natural Join in PostgreSQL
In PostgreSQL, both equijoin and natural join are used to combine rows from two or more tables based on a related column. However, there are some differences between the two.
An equijoin is a type of join that combines rows based on equality between values in the specified columns. It requires explicitly specifying the join condition using the "ON" keyword.
A natural join, on the other hand, is a type of equijoin that automatically matches the columns with the same name in the two tables. It does not require specifying the join condition explicitly.
Consider the following example where we have two tables, "employees" and "departments":
CREATE TABLE employees ( employee_id INT PRIMARY KEY, employee_name VARCHAR(100), department_id INT);CREATE TABLE departments ( department_id INT PRIMARY KEY, department_name VARCHAR(100));
To perform an equijoin on the "employees" and "departments" tables based on the "department_id" column, you can use the following SQL query:
SELECT employees.employee_name, departments.department_nameFROM employeesINNER JOIN departments ON employees.department_id = departments.department_id;
To perform a natural join on the same tables, you can use the following SQL query:
SELECT employee_name, department_nameFROM employeesNATURAL JOIN departments;
In this query, the natural join automatically matches the "department_id" column in the two tables without explicitly specifying the join condition.
It is important to note that natural joins can be convenient when the column names are the same in both tables, but they may not always produce the desired results if the column names are not consistent or if there are additional columns with the same name.
Performing Self Join in PostgreSQL
In PostgreSQL, a self join is a type of join where a table is joined with itself. It is useful when you want to combine rows from the same table based on a related column.
Consider the following example where we have a "employees" table that contains information about employees and their managers:
CREATE TABLE employees ( employee_id INT PRIMARY KEY, employee_name VARCHAR(100), manager_id INT);INSERT INTO employees (employee_id, employee_name, manager_id)VALUES (101, 'John Doe', 102), (102, 'Jane Smith', NULL), (103, 'Michael Johnson', 102);
To perform a self join on the "employees" table to get the manager names for each employee, you can use the following SQL query:
SELECT e.employee_name AS employee_name, m.employee_name AS manager_nameFROM employees eLEFT JOIN employees m ON e.manager_id = m.employee_id;
This query will return the employee name and manager name for each employee, including those without a manager (NULL value for manager_id).
A self join can be useful in scenarios where you need to combine rows from the same table based on a related column, such as hierarchical data structures or parent-child relationships.
Importance of Join Condition in PostgreSQL
In PostgreSQL, the join condition plays a crucial role in combining rows from two or more tables in a join operation. It specifies how the rows should be matched and combined based on the values of the specified columns.
The join condition is specified in the ON clause of the join statement. It typically includes an equality comparison between the columns that represent the relationship between the tables.
Without a proper join condition, the join operation may produce incorrect or unexpected results. It is important to ensure that the join condition accurately reflects the relationship between the tables and the desired result.
Consider the following example where we have two tables, "customers" and "orders":
CREATE TABLE customers ( customer_id INT PRIMARY KEY, customer_name VARCHAR(100), city VARCHAR(50));CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT, order_date DATE, total_amount DECIMAL(10,2), FOREIGN KEY (customer_id) REFERENCES customers(customer_id));
To perform an inner join on the "customers" and "orders" tables based on the "customer_id" column, you can use the following SQL query:
SELECT customers.customer_name, orders.order_date, orders.total_amountFROM customersINNER JOIN orders ON customers.customer_id = orders.customer_id;
In this query, the join condition "customers.customer_id = orders.customer_id" ensures that only the rows with matching customer IDs are combined in the result.
It is important to carefully define and specify the join condition to ensure the accuracy and correctness of the join operation.
Related Article: Extracting the Month from a Date in PostgreSQL
Different Join Types in PostgreSQL
In PostgreSQL, there are several types of joins that can be used to combine rows from two or more tables based on a related column. The choice of join type depends on the specific requirements of the query and the relationship between the tables.
The common types of joins in PostgreSQL include:
- Inner Join: An inner join returns only the rows that have matching values in both tables based on the specified join condition.
- Left Outer Join: A left outer join returns all rows from the left table and the matched rows from the right table. If there is no match, NULL values are returned for the columns of the right table.
- Right Outer Join: A right outer join returns all rows from the right table and the matched rows from the left table. If there is no match, NULL values are returned for the columns of the left table.
- Full Outer Join: A full outer join returns all rows from both tables, including unmatched rows from either table. If there is no match, NULL values are returned for the columns of the other table.
- Cross Join: A cross join combines every row from one table with every row from another table, resulting in a Cartesian product.
- Self Join: A self join is used to join a table with itself, typically to combine rows based on a related column.
It is important to choose the appropriate join type based on the desired result and the relationship between the tables.
Join Operator in PostgreSQL
In PostgreSQL, the join operator is used to combine rows from two or more tables based on a related column. The join operator is represented by the keyword "JOIN" and is typically used in conjunction with the "ON" keyword to specify the join condition.
Consider the following example where we have two tables, "employees" and "departments":
CREATE TABLE employees ( employee_id INT PRIMARY KEY, employee_name VARCHAR(100), department_id INT);CREATE TABLE departments ( department_id INT PRIMARY KEY, department_name VARCHAR(100));
To perform an inner join on the "employees" and "departments" tables based on the "department_id" column, you can use the following SQL query:
SELECT employees.employee_name, departments.department_nameFROM employeesJOIN departments ON employees.department_id = departments.department_id;
In this query, the join operator "JOIN" combines the rows from the "employees" and "departments" tables based on the equality of the "department_id" column.
The join operator is an essential component of the join operation and allows you to combine rows from multiple tables based on the specified join condition.
Join Column Selection in PostgreSQL
In PostgreSQL, join column selection refers to the process of selecting the columns to include in the result set of a join operation. It allows you to choose the specific columns from the joined tables that you want to retrieve.
When performing a join operation, all columns from the joined tables are available in the result set by default. However, it is often unnecessary and inefficient to retrieve all columns, especially if they are not required for the query.
To select specific columns from the joined tables, you can list the column names after the SELECT keyword in the SQL query.
Consider the following example where we have two tables, "customers" and "orders":
CREATE TABLE customers ( customer_id INT PRIMARY KEY, customer_name VARCHAR(100), city VARCHAR(50));CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT, order_date DATE, total_amount DECIMAL(10,2), FOREIGN KEY (customer_id) REFERENCES customers(customer_id));
To perform an inner join on the "customers" and "orders" tables based on the "customer_id" column and select only the customer name and order date from the result, you can use the following SQL query:
SELECT customers.customer_name, orders.order_dateFROM customersINNER JOIN orders ON customers.customer_id = orders.customer_id;
In this query, the SELECT statement specifies the columns "customers.customer_name" and "orders.order_date" to be included in the result set.
Additional Resources
- PostgreSQL Joins