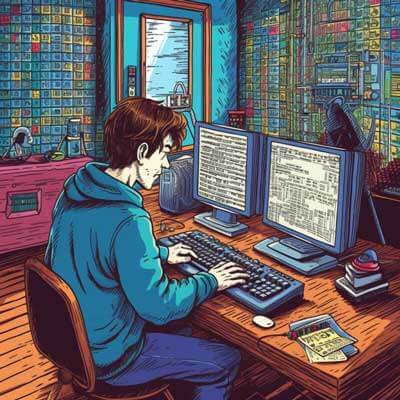
Table of Contents
Configuring pg_hba.conf in PostgreSQL
The pg_hba.conf file in PostgreSQL is responsible for controlling client authentication. It determines which clients are allowed to connect to the PostgreSQL server and how they are authenticated. This configuration file is located in the data directory of your PostgreSQL installation.
The file consists of a series of lines, each specifying a connection type, a database name, a user name, an IP address range, and the authentication method to be used. By modifying this file, you can control who can access your PostgreSQL database and how they are authenticated.
Here is an example of a pg_hba.conf file:
# TYPE DATABASE USER ADDRESS METHOD # "local" is for Unix domain socket connections only local all all trust # IPv4 local connections: host all all 127.0.0.1/32 md5 # IPv6 local connections: host all all ::1/128 md5
In this example, we have three lines. The first line allows local connections from any user and any database without requiring authentication. The second and third lines allow connections from the localhost IP address (127.0.0.1 for IPv4 and ::1 for IPv6) for all users and all databases, but require password authentication.
It's important to note that changes made to the pg_hba.conf file require a restart of the PostgreSQL server for the changes to take effect.
Related Article: Detecting and Resolving Deadlocks in PostgreSQL Databases
Understanding Authentication in PostgreSQL
Authentication in PostgreSQL is the process of verifying the identity of a user or client connecting to the database server. PostgreSQL supports various authentication methods, including trust, password, ident, peer, md5, scram-sha-256, and certificate-based authentication.
- Trust: This method allows any user to connect without providing a password. It is the least secure method and should only be used in trusted environments.
- Password: This method requires users to provide a password for authentication. The password is stored in the database's user table and is encrypted for security.
- Ident: This method uses the ident server on the client machine to verify the client's identity. It is commonly used on Unix-based systems.
- Peer: This method authenticates the client based on the operating system user name. It is commonly used on Unix-based systems.
- MD5: This method stores encrypted passwords on the server and requires clients to provide the password in MD5-encrypted format.
- SCRAM-SHA-256: This method provides a more secure way of storing passwords on the server and authenticating clients using the Salted Challenge Response Authentication Mechanism (SCRAM).
- Certificate-based authentication: This method uses SSL certificates to authenticate clients. It provides strong security but requires more configuration.
The authentication method is specified in the pg_hba.conf file for each connection type, database, and user. By understanding the different authentication methods, you can choose the appropriate method based on your security requirements.
Establishing a Connection to a PostgreSQL Database
To establish a connection to a PostgreSQL database, you need to provide the necessary connection details, including the host, port, database name, user name, and password. There are several ways to establish a connection, depending on the programming language or tool you are using.
Here is an example of establishing a connection to a PostgreSQL database using Python and the psycopg2 library:
import psycopg2 # Connection details host = 'localhost' port = '5432' database = 'mydatabase' user = 'myuser' password = 'mypassword' # Establish a connection conn = psycopg2.connect( host=host, port=port, database=database, user=user, password=password ) # Perform database operations # ... # Close the connection conn.close()
In this example, we import the psycopg2 library and define the connection details. We then use the psycopg2.connect()
function to establish a connection to the PostgreSQL database. After performing the necessary database operations, we close the connection using the close()
method.
The connection details may vary depending on your PostgreSQL setup, so make sure to provide the correct values for the host, port, database, user, and password.
Troubleshooting 'Can't Access' Error in PostgreSQL
If you encounter a "can't access" error when trying to connect to a PostgreSQL database, there are several steps you can take to troubleshoot and resolve the issue.
1. Check the PostgreSQL server status: Make sure the PostgreSQL server is running and accessible. You can use the pg_ctl
command to start, stop, or restart the server.
2. Verify the connection details: Double-check the connection details, including the host, port, database name, user name, and password. Ensure that you are using the correct values and that there are no typos or errors.
3. Check the pg_hba.conf file: Review the pg_hba.conf file to ensure that the authentication method and access rules are correctly configured for the connection type, database, and user. Make sure that the authentication method allows the user to connect and that the IP address or range is correct.
4. Test the connection using psql: Use the psql command-line tool to test the connection. Open a terminal or command prompt and run the following command, replacing the connection details with your own:
psql -h localhost -p 5432 -U myuser -d mydatabase
If the connection is successful, you should see the psql command prompt. If not, an error message will be displayed, providing more information about the issue.
5. Check firewall settings: If you are connecting to a remote PostgreSQL server, ensure that the necessary ports (usually 5432) are open in the firewall. Consult your system administrator or network administrator for assistance with firewall configuration.
6. Check log files: Review the PostgreSQL log files for any error messages or warnings that may indicate the cause of the connection issue. The log files are typically located in the data directory of your PostgreSQL installation.
Related Article: How to Check and Change Postgresql's Default Port
Resolving Permission Issues in PostgreSQL
Permission issues in PostgreSQL can occur when a user does not have the necessary privileges to perform a certain operation, such as creating or modifying database objects, executing queries, or accessing specific tables or columns.
To resolve permission issues in PostgreSQL, you can take the following steps:
1. Check the user's privileges: Verify the privileges assigned to the user by querying the pg_roles
system catalog table. Use the following SQL statement to list the privileges for a specific user:
SELECT rolname, rolsuper, rolcreaterole, rolcreatedb FROM pg_roles WHERE rolname = 'myuser';
This query will return information about the user's superuser status, role creation privilege, and database creation privilege.
2. Grant necessary privileges: If the user does not have the required privileges, you can grant them using the GRANT
statement. For example, to grant the user the ability to create databases, you can use the following SQL statement:
GRANT CREATEDB TO myuser;
Similarly, you can grant other privileges such as CREATE TABLE
, SELECT
, INSERT
, UPDATE
, DELETE
, etc.
3. Grant privileges at the object level: If the permission issue is specific to a particular table or object, you can grant privileges at the object level using the GRANT
statement. For example, to grant SELECT
privilege on a table to a user, you can use the following SQL statement:
GRANT SELECT ON tablename TO myuser;
Replace tablename
with the name of the table.
4. Check for role membership: If the user is a member of a role that has conflicting privileges, the permissions may be inherited from the role. Use the SET ROLE
statement to temporarily disable role membership and check if the permission issue persists.
5. Restart the PostgreSQL server: Some privilege changes may require a restart of the PostgreSQL server to take effect. If you have made any changes to user privileges, restart the server and test the permissions again.
The Role of SQL in Accessing a PostgreSQL Database
SQL (Structured Query Language) plays a vital role in accessing and interacting with a PostgreSQL database. It is a standard language for managing relational databases and provides a wide range of commands and statements for querying, inserting, updating, and deleting data.
Here are some key aspects of SQL in the context of accessing a PostgreSQL database:
1. Querying data: SQL allows you to retrieve data from a PostgreSQL database by using the SELECT
statement. You can specify the columns to retrieve, apply filtering conditions, sort the results, and perform various calculations and aggregations.
Example:
SELECT column1, column2 FROM tablename WHERE condition;
2. Modifying data: SQL provides statements for inserting, updating, and deleting data in a PostgreSQL database. The INSERT
statement is used to add new rows, the UPDATE
statement is used to modify existing rows, and the DELETE
statement is used to remove rows from a table.
Example:
INSERT INTO tablename (column1, column2) VALUES (value1, value2); UPDATE tablename SET column1 = newvalue WHERE condition; DELETE FROM tablename WHERE condition;
3. Creating and modifying database objects: SQL allows you to create and modify database objects such as tables, views, indexes, constraints, and functions. The CREATE
statement is used to create new objects, while the ALTER
statement is used to modify existing objects.
Example:
CREATE TABLE tablename ( column1 datatype, column2 datatype ); ALTER TABLE tablename ADD COLUMN newcolumn datatype; CREATE INDEX indexname ON tablename (column);
4. Managing transactions: SQL provides commands for managing transactions in PostgreSQL. Transactions allow you to group multiple SQL statements into a single logical unit of work that is executed atomically. You can start a transaction with the BEGIN
statement, commit it with the COMMIT
statement, or roll it back with the ROLLBACK
statement.
Example:
BEGIN; -- SQL statements COMMIT;
These are just a few examples of the role of SQL in accessing a PostgreSQL database. SQL is a useful language that allows you to perform a wide range of operations, from simple queries to complex data manipulations and database management tasks.
Setting Up Access Control in PostgreSQL
Access control in PostgreSQL is essential for securing your database and protecting sensitive data. By setting up access control, you can control who can connect to the database, what actions they can perform, and which data they can access.
To set up access control in PostgreSQL, you can follow these steps:
1. Configure authentication methods: Update the pg_hba.conf file to specify the authentication methods for different types of connections, such as local, host, hostssl, and hostnossl. You can choose from various authentication methods, including trust, password, md5, scram-sha-256, and certificate-based authentication.
Example:
# TYPE DATABASE USER ADDRESS METHOD # "local" is for Unix domain socket connections only local all all md5 # IPv4 local connections: host all all 127.0.0.1/32 md5 # IPv6 local connections: host all all ::1/128 md5
2. Create database users: Use the CREATE ROLE
statement to create database users with the necessary privileges. You can specify the user name, password, and role attributes such as superuser status, role creation privilege, and database creation privilege.
Example:
CREATE ROLE myuser WITH LOGIN PASSWORD 'mypassword';
3. Grant privileges: Use the GRANT
statement to grant privileges to users or roles. You can grant privileges at the database level, table level, or even column level. Specify the privilege, the target object, and the user or role to which the privilege is granted.
Example:
GRANT SELECT, INSERT, UPDATE, DELETE ON mytable TO myuser;
4. Revoke privileges: If necessary, you can revoke privileges from users or roles using the REVOKE
statement. Specify the privilege, the target object, and the user or role from which the privilege is revoked.
Example:
REVOKE INSERT, UPDATE ON mytable FROM myuser;
5. Audit user activity: Set up auditing or monitoring mechanisms to track user activity in the PostgreSQL database. This can include logging user logins, queries executed, modifications made to the database, and other relevant information.
Example:
# Enable logging of all statements log_statement = 'all' # Specify log file location log_directory = '/var/log/postgresql'
Understanding Queries in PostgreSQL
Queries are an essential part of working with a PostgreSQL database. They allow you to retrieve, manipulate, and analyze data stored in the database. PostgreSQL supports a rich set of query capabilities, including filtering, sorting, aggregating, and joining data from multiple tables.
Here are some key aspects of queries in PostgreSQL:
1. SELECT statement: The SELECT statement is used to retrieve data from one or more tables in the database. It allows you to specify the columns to retrieve, apply filtering conditions using the WHERE clause, sort the results using the ORDER BY clause, and perform various calculations and aggregations using functions.
Example:
SELECT column1, column2 FROM tablename WHERE condition ORDER BY column1 ASC;
2. Filtering data: You can filter data in a query using the WHERE clause. This allows you to specify conditions that the retrieved rows must satisfy. You can use comparison operators, logical operators, and functions to build complex filtering conditions.
Example:
SELECT column1, column2 FROM tablename WHERE column1 > 10 AND column2 LIKE '%abc%';
3. Sorting data: You can sort the retrieved data using the ORDER BY clause. This allows you to specify one or more columns by which the results should be sorted. You can specify the sort order as ASC (ascending) or DESC (descending).
Example:
SELECT column1, column2 FROM tablename ORDER BY column1 ASC, column2 DESC;
4. Aggregating data: PostgreSQL provides various aggregate functions for performing calculations on groups of rows. These functions allow you to calculate sums, averages, counts, maximums, minimums, and other aggregations.
Example:
SELECT category, COUNT(*) AS count FROM products GROUP BY category ORDER BY count DESC;
5. Joining tables: You can combine data from multiple tables in a query using the JOIN operation. This allows you to retrieve related data by matching values in common columns between the tables.
Example:
SELECT orders.order_id, customers.customer_name FROM orders INNER JOIN customers ON orders.customer_id = customers.customer_id;
These are just a few examples of the query capabilities in PostgreSQL. By understanding and utilizing these features, you can retrieve and manipulate data efficiently and effectively.
Related Article: Determining the Status of a Running Query in PostgreSQL
Configuring a PostgreSQL Node
In PostgreSQL, a node refers to an instance of the database server running on a specific host and port. Configuring a PostgreSQL node involves setting up various parameters and options to optimize performance, manage resources, and ensure data integrity.
Here are some key aspects of configuring a PostgreSQL node:
1. PostgreSQL configuration file: The main configuration file for a PostgreSQL node is postgresql.conf. This file contains various settings that control the behavior of the database server, such as memory allocation, disk storage, connection limits, logging, and replication.
Example:
# Memory settings shared_buffers = 4GB work_mem = 16MB # Disk storage settings data_directory = '/var/lib/postgresql/12/main' max_wal_size = 1GB # Connection settings max_connections = 100 # Logging settings log_directory = '/var/log/postgresql' log_statement = 'all' # Replication settings wal_level = replica
2. Resource management: PostgreSQL allows you to manage system resources to optimize performance and prevent resource exhaustion. You can configure parameters such as shared_buffers, work_mem, maintenance_work_mem, and temp_buffers to control memory usage. You can also set limits on the number of connections, background processes, and disk I/O.
3. Security settings: PostgreSQL provides various security-related configuration options to protect your data. You can enable or disable SSL encryption, specify authentication methods and access control rules in the pg_hba.conf file, and configure password encryption algorithms.
4. Logging and monitoring: Configuring logging and monitoring options allows you to track and analyze the activity of your PostgreSQL node. You can specify log file locations, log levels, and log rotation settings. You can also enable query logging, transaction logging, and statement logging for auditing and troubleshooting purposes.
5. Replication and high availability: If you are setting up a replicated or high-availability PostgreSQL cluster, you need to configure parameters related to replication, such as wal_level, max_wal_size, and synchronous_commit. These settings determine how data is replicated and synchronized between the primary and standby nodes.
These are just a few examples of the configuration options available for a PostgreSQL node. By carefully configuring these parameters based on your specific requirements and hardware capabilities, you can optimize the performance, security, and reliability of your PostgreSQL database.
Main Features of PostgreSQL
PostgreSQL is a feature-rich and useful open-source relational database management system (RDBMS). It provides a wide range of features that make it a popular choice for both small-scale and enterprise-level applications. Here are some of the main features of PostgreSQL:
1. ACID compliance: PostgreSQL follows the principles of ACID (Atomicity, Consistency, Isolation, Durability) to ensure data integrity and reliability. It guarantees that database transactions are processed in a reliable and consistent manner.
2. Extensibility: PostgreSQL allows you to extend its functionality by creating custom data types, operators, and functions. You can also write stored procedures, triggers, and user-defined aggregate functions using various programming languages such as SQL, PL/pgSQL, Python, and more.
3. JSON and NoSQL support: PostgreSQL includes native support for storing, querying, and manipulating JSON data. It provides a rich set of functions and operators for working with JSON documents, making it a suitable choice for hybrid applications that require both structured and unstructured data.
4. Full-text search: PostgreSQL offers advanced full-text search capabilities, allowing you to perform complex text searches on large volumes of data. It supports features such as stemming, ranking, phrase searching, and search result highlighting.
5. Geospatial data support: PostgreSQL includes support for storing and querying geospatial data, making it ideal for applications that require location-based services and mapping functionality. It provides a range of functions and operators for working with geospatial data types such as points, lines, and polygons.
6. Replication and high availability: PostgreSQL supports various replication methods, including asynchronous and synchronous replication, logical replication, and streaming replication. These features enable you to create highly available database clusters and ensure data redundancy.
7. Foreign data wrappers: PostgreSQL allows you to access data stored in external data sources using foreign data wrappers. This feature enables you to query and join data from different databases and systems, such as Oracle, MySQL, MongoDB, and more, seamlessly within PostgreSQL.
8. Parallel query execution: PostgreSQL supports parallel query execution, allowing queries to be divided into smaller tasks and executed concurrently across multiple CPU cores. This feature improves query performance and scalability on multi-core systems.
9. Advanced indexing options: PostgreSQL provides various indexing options, including B-tree, Hash, GiST (Generalized Search Tree), GIN (Generalized Inverted Index), and SP-GiST (Space-Partitioned Generalized Search Tree). These indexing methods optimize query performance for different types of data and query patterns.
10. Advanced security features: PostgreSQL offers robust security features, including SSL encryption, user authentication, access control lists (ACLs), and row-level security. It provides granular control over user privileges and data visibility, ensuring data confidentiality and integrity.
Additional Resources
- PostgreSQL: Documentation: 10: 19.3. The pg_hba.conf File