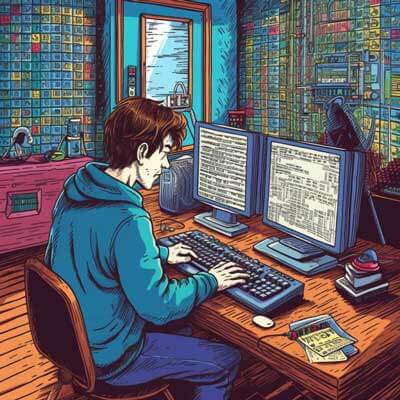
- Overview of SQL query length in PostgreSQL
- Factors that affect the length of SQL queries in PostgreSQL
- Understanding the maximum query length in PostgreSQL
- Exploring the size restriction for SQL queries in PostgreSQL
- Examining the query length constraint in PostgreSQL
- Implications of exceeding the maximum SQL query length in PostgreSQL
- Best practices for managing SQL query length in PostgreSQL
- Code snippet: Checking the length of a SQL query in PostgreSQL
- Code snippet: Splitting a long SQL query into multiple queries in PostgreSQL
- Code snippet: Handling long SQL queries with prepared statements in PostgreSQL
- Code snippet: Using temporary tables to manage large SQL queries in PostgreSQL
- Code snippet: Implementing pagination to mitigate SQL query length in PostgreSQL
- Code snippet: Using views or subqueries to simplify long SQL queries in PostgreSQL
- Code snippet: Leveraging indexing to improve performance of long SQL queries in PostgreSQL
- Code snippet: Utilizing query rewriting techniques to reduce SQL query length in PostgreSQL
- Common misconceptions about the maximum query length in PostgreSQL
- Additional limitations and considerations for SQL queries in PostgreSQL
- Comparison of maximum query lengths in different database systems
- Future enhancements and developments for SQL query length in PostgreSQL
- Additional Resources
Overview of SQL query length in PostgreSQL
PostgreSQL is a useful and feature-rich open-source relational database management system (RDBMS). It offers a wide range of capabilities for storing, retrieving, and manipulating data. One important aspect of working with databases is executing SQL queries to interact with the data. SQL queries are used to retrieve, insert, update, and delete data in a database.
When working with SQL queries, it is important to understand if there are any limitations on the length of the queries that can be executed in PostgreSQL. In other words, does PostgreSQL have a maximum SQL query length?
The answer is yes, PostgreSQL does have a maximum SQL query length. However, it is essential to note that the maximum query length is not a fixed value and can vary depending on various factors.
Related Article: PostgreSQL HyperLogLog (HLL) & Cardinality Estimation
Factors that affect the length of SQL queries in PostgreSQL
Several factors can affect the length of SQL queries in PostgreSQL. It is crucial to understand these factors as they can impact the maximum query length that can be executed.
1. Database Configuration: The maximum SQL query length can be influenced by the configuration settings of the PostgreSQL database. The max_query_size
parameter in the postgresql.conf
file determines the maximum size of a query that can be executed. This parameter can be adjusted to increase or decrease the maximum query length.
2. Network Limitations: The network infrastructure between the client and the PostgreSQL database can impose limitations on the maximum query length. For example, if there are firewalls, proxies, or other network devices in place, they may have their own restrictions on the size of data packets that can be transmitted.
3. Client Applications: The client applications used to execute SQL queries can also have limitations on the maximum query length. These limitations may be imposed by the programming language, libraries, or frameworks used to build the client application.
4. Memory Constraints: The available memory on the PostgreSQL server can impact the maximum query length. If the query requires a large amount of memory to execute, it may not be possible to execute queries of a certain length due to memory constraints.
Understanding the maximum query length in PostgreSQL
As mentioned earlier, the maximum query length in PostgreSQL is not a fixed value and can vary depending on various factors. By default, the maximum query length in PostgreSQL is 1 GB. However, this value can be changed by modifying the max_query_size
parameter in the postgresql.conf
file.
It is important to note that even though the maximum query length can be increased, executing extremely long queries can have performance implications. Longer queries require more resources and can take longer to execute, potentially impacting the overall performance of the database.
To check the current value of the max_query_size
parameter, you can use the following SQL query:
SHOW max_query_size;
To modify the value of the max_query_size
parameter, you can use the following SQL query:
SET max_query_size = '2GB';
Exploring the size restriction for SQL queries in PostgreSQL
While PostgreSQL does have a maximum query length, it is important to note that this limitation is not solely based on the number of characters in the query. The size restriction for SQL queries in PostgreSQL is determined by the maximum size of a query packet that can be transmitted over the network.
The maximum query packet size is controlled by the max_packet_size
parameter in the postgresql.conf
file. By default, the maximum packet size is 4 MB. However, this value can be modified to allow larger packets.
To check the current value of the max_packet_size
parameter, you can use the following SQL query:
SHOW max_packet_size;
To modify the value of the max_packet_size
parameter, you can use the following SQL query:
SET max_packet_size = '8MB';
It is important to note that changing the max_packet_size
parameter requires a server restart for the new value to take effect.
Related Article: How to Check if a Table Exists in PostgreSQL
Examining the query length constraint in PostgreSQL
PostgreSQL enforces a constraint on the length of SQL queries to ensure the stability and performance of the database. When executing a query, if the length of the query exceeds the maximum query length, PostgreSQL will raise an error and the query will not be executed.
To illustrate this, let’s consider an example where we attempt to execute a query that exceeds the maximum query length:
SELECT * FROM large_table WHERE column1 = 'some_value' AND column2 = 'some_other_value' AND ... (continued)
If the length of this query exceeds the maximum query length, PostgreSQL will raise an error similar to the following:
ERROR: query is too large
When working with large queries, it is important to be mindful of the maximum query length and ensure that queries are optimized and structured efficiently.
Implications of exceeding the maximum SQL query length in PostgreSQL
Exceeding the maximum SQL query length in PostgreSQL can have several implications. Some of the key implications are:
1. Performance Impact: Executing extremely long queries can have a significant impact on the performance of the database. Longer queries require more resources and can take longer to execute, potentially slowing down other queries and impacting the overall performance of the database.
2. Resource Consumption: Longer queries require more memory and disk space to execute. Exceeding the maximum query length can lead to increased resource consumption, potentially causing memory or disk space issues on the PostgreSQL server.
3. Network Limitations: As mentioned earlier, the maximum query length can be influenced by network limitations. Exceeding the maximum query length may result in network-related errors or failures, especially if there are restrictions on the size of data packets that can be transmitted.
4. Code Maintainability: Writing and managing extremely long queries can make code harder to read, understand, and maintain. It is generally considered a best practice to break down complex queries into smaller, more manageable parts to improve code maintainability.
To mitigate these implications, it is recommended to optimize SQL queries and ensure they are structured efficiently. This includes techniques such as using appropriate indexes, minimizing data retrieval, and utilizing query rewriting techniques.
Best practices for managing SQL query length in PostgreSQL
When working with SQL queries in PostgreSQL, it is important to follow best practices to manage query length effectively. Here are some best practices to consider:
1. Query Optimization: Optimize SQL queries to reduce their length and improve performance. This includes techniques such as using appropriate indexes, minimizing data retrieval, and utilizing query rewriting techniques.
2. Code Refactoring: Refactor long and complex queries into smaller, more manageable parts. This improves code maintainability and makes it easier to debug and optimize queries.
3. Use Prepared Statements: Utilize prepared statements to handle parameterized queries. Prepared statements can help reduce the length of queries by separating the query logic from the parameter values.
4. Use Temporary Tables: Consider using temporary tables to manage large SQL queries. Temporary tables can help break down complex queries into smaller parts, making them more manageable and improving performance.
5. Implement Pagination: Implement pagination techniques to limit the amount of data returned by a query. This can help reduce the length of queries and improve performance, especially when dealing with large result sets.
6. Use Views or Subqueries: Utilize views or subqueries to simplify long queries. Views and subqueries can help break down complex logic into smaller, more manageable parts, making queries more readable and maintainable.
7. Leverage Indexing: Use appropriate indexes to improve the performance of long queries. Indexing can help speed up data retrieval and reduce the length of queries by allowing the database to quickly locate the required data.
Related Article: Applying Aggregate Functions in PostgreSQL WHERE Clause
Code snippet: Checking the length of a SQL query in PostgreSQL
To check the length of a SQL query in PostgreSQL, you can use the length()
function to get the number of characters in the query. Here’s an example:
SELECT length('SELECT * FROM large_table WHERE column1 = ''some_value'' AND column2 = ''some_other_value''');
This query will return the length of the SQL query as an integer value.
Code snippet: Splitting a long SQL query into multiple queries in PostgreSQL
When working with long SQL queries in PostgreSQL, it is often beneficial to split them into multiple smaller queries. This can improve code maintainability and performance. Here’s an example of splitting a long SQL query into multiple queries:
-- Original long query SELECT * FROM large_table WHERE column1 = 'some_value' AND column2 = 'some_other_value' AND ... (continued) -- Split into multiple queries -- Query 1 WITH matching_rows AS ( SELECT * FROM large_table WHERE column1 = 'some_value' ) SELECT * FROM matching_rows WHERE column2 = 'some_other_value'; -- Query 2 WITH matching_rows AS ( SELECT * FROM large_table WHERE column1 = 'some_value' ) SELECT * FROM matching_rows WHERE column3 = 'another_value';
In this example, the original long query is split into two smaller queries using a common table expression (CTE). This makes the queries more manageable and easier to optimize.
Code snippet: Handling long SQL queries with prepared statements in PostgreSQL
Prepared statements can be used to handle long SQL queries in PostgreSQL. Prepared statements separate the query logic from the parameter values, making the queries more manageable. Here’s an example of using prepared statements in PostgreSQL:
-- Prepare the statement PREPARE my_statement (text, text) AS SELECT * FROM large_table WHERE column1 = $1 AND column2 = $2; -- Execute the prepared statement EXECUTE my_statement ('some_value', 'some_other_value');
In this example, the PREPARE
statement is used to prepare a query with placeholders for the parameters. The EXECUTE
statement is then used to execute the prepared statement with the actual parameter values.
Using prepared statements can help reduce the length of queries and improve performance, especially when dealing with parameterized queries.
Related Article: How to Convert Columns to Rows in PostgreSQL
Code snippet: Using temporary tables to manage large SQL queries in PostgreSQL
Temporary tables can be used to manage large SQL queries in PostgreSQL. Temporary tables provide a way to break down complex queries into smaller parts, making them more manageable and improving performance. Here’s an example of using temporary tables in PostgreSQL:
-- Create a temporary table CREATE TEMPORARY TABLE temp_table AS SELECT * FROM large_table WHERE column1 = 'some_value'; -- Query the temporary table SELECT * FROM temp_table WHERE column2 = 'some_other_value';
In this example, a temporary table is created to store a subset of data from the larger table. The subsequent query then operates on the temporary table, making the query more manageable and improving performance.
Temporary tables are automatically dropped at the end of the session or transaction, making them a convenient option for managing large queries.
Code snippet: Implementing pagination to mitigate SQL query length in PostgreSQL
Implementing pagination can help mitigate the impact of long SQL queries in PostgreSQL by limiting the amount of data returned. Here’s an example of implementing pagination in PostgreSQL using the OFFSET
and LIMIT
clauses:
SELECT * FROM large_table OFFSET 0 LIMIT 10;
In this example, the OFFSET
clause specifies the starting position of the result set, while the LIMIT
clause limits the number of rows returned. By adjusting the values of OFFSET
and LIMIT
, you can navigate through the result set in a paginated manner.
Implementing pagination can help reduce the length of queries and improve performance, especially when dealing with large result sets.
Code snippet: Using views or subqueries to simplify long SQL queries in PostgreSQL
Views and subqueries can be used to simplify long SQL queries in PostgreSQL. They provide a way to break down complex logic into smaller, more manageable parts, making queries more readable and maintainable. Here’s an example of using views and subqueries in PostgreSQL:
-- Create a view CREATE VIEW my_view AS SELECT * FROM large_table WHERE column1 = 'some_value'; -- Query the view SELECT * FROM my_view WHERE column2 = 'some_other_value';
In this example, a view is created to encapsulate a subset of data from the larger table. The subsequent query then operates on the view, making the query more manageable and improving readability.
Views and subqueries can simplify complex queries by breaking them down into smaller parts, making them easier to understand and maintain.
Related Article: Detecting and Resolving Deadlocks in PostgreSQL Databases
Code snippet: Leveraging indexing to improve performance of long SQL queries in PostgreSQL
Indexing can be leveraged to improve the performance of long SQL queries in PostgreSQL. Indexes allow the database to quickly locate the required data, reducing the length of queries and improving performance. Here’s an example of leveraging indexing in PostgreSQL:
-- Create an index CREATE INDEX my_index ON large_table (column1, column2); -- Query the table using the index SELECT * FROM large_table WHERE column1 = 'some_value' AND column2 = 'some_other_value';
In this example, an index is created on the relevant columns in the table. The subsequent query then utilizes the index to efficiently retrieve the required data.
Code snippet: Utilizing query rewriting techniques to reduce SQL query length in PostgreSQL
Query rewriting techniques can be used to reduce the length of SQL queries in PostgreSQL. These techniques involve rephrasing and restructuring queries to achieve the same result with a shorter query. Here’s an example of utilizing query rewriting techniques in PostgreSQL:
-- Original query SELECT * FROM large_table WHERE column1 = 'some_value' OR column1 = 'some_other_value'; -- Rewritten query using IN clause SELECT * FROM large_table WHERE column1 IN ('some_value', 'some_other_value');
In this example, the original query is rewritten using the IN
clause, which allows multiple values to be specified in a shorter syntax.
Common misconceptions about the maximum query length in PostgreSQL
There are a few common misconceptions about the maximum query length in PostgreSQL that are worth addressing:
1. Maximum Query Length Equals Maximum Characters: The maximum query length in PostgreSQL is not solely determined by the number of characters in the query. It is influenced by various factors, including network limitations, memory constraints, and database configuration settings.
2. Increasing Maximum Query Length Always Improves Performance: While it is possible to increase the maximum query length in PostgreSQL, executing extremely long queries can have performance implications. Longer queries require more resources and can take longer to execute, potentially impacting the overall performance of the database.
3. Maximum Query Length is the Same Across all Database Systems: The maximum query length can vary across different database systems. While PostgreSQL has its own maximum query length, other database systems may have different limitations. It is important to consult the documentation of the specific database system you are using to determine its maximum query length.
Related Article: Executing Efficient Spatial Queries in PostgreSQL
Additional limitations and considerations for SQL queries in PostgreSQL
In addition to the maximum query length, there are other limitations and considerations to keep in mind when working with SQL queries in PostgreSQL. Some of these include:
1. Query Complexity: Long queries are often more complex, which can make them harder to understand, optimize, and maintain. It is generally considered a best practice to break down complex queries into smaller, more manageable parts.
2. Execution Time: Long queries can take more time to execute, especially if they involve complex joins, aggregations, or table scans. It is important to optimize queries and ensure they are structured efficiently to minimize execution time.
3. Security: SQL queries are susceptible to SQL injection attacks if not properly handled. It is crucial to use parameterized queries or prepared statements to prevent such attacks.
4. Scalability: Long queries can put a strain on the resources of the PostgreSQL server, especially when dealing with large result sets or concurrent query execution. It is important to monitor the resource usage and consider scaling options if necessary.
5. Query Plan Optimization: PostgreSQL utilizes a query optimizer to determine the most efficient execution plan for a query. However, long and complex queries can pose challenges for the optimizer, potentially leading to suboptimal query plans. It is important to analyze and optimize query plans to ensure optimal performance.
Comparison of maximum query lengths in different database systems
The maximum query length can vary across different database systems. Here is a comparison of the maximum query lengths in popular database systems:
– PostgreSQL: By default, the maximum query length in PostgreSQL is 1 GB, but it can be changed by modifying the max_query_size
parameter. The maximum packet size is controlled by the max_packet_size
parameter.
– MySQL: The maximum query length in MySQL is determined by the value of the max_allowed_packet
parameter. By default, the maximum packet size is 4 MB.
– Oracle: The maximum query length in Oracle is determined by the maximum size of a VARCHAR2 column, which is 4000 bytes by default. However, it can be increased to a maximum of 32767 bytes.
– Microsoft SQL Server: The maximum query length in Microsoft SQL Server is 65,536 characters. However, the actual limit may be lower depending on the network infrastructure and client applications.
It is important to consult the documentation of the specific database system you are using to determine its maximum query length.
Future enhancements and developments for SQL query length in PostgreSQL
As PostgreSQL continues to evolve, there may be future enhancements and developments related to SQL query length. Here are some potential areas of improvement:
1. Dynamic Query Length: The ability to dynamically adjust the maximum query length based on workload and resource availability could provide more flexibility and optimize performance.
2. Query Compression: Implementing query compression techniques could reduce the size of queries, enabling more efficient transmission over the network and reducing resource consumption.
3. Query Streaming: Streaming query results could allow for the processing of large result sets without the need to retrieve the entire result set at once, potentially reducing the need for long queries.
4. Improved Error Handling: Enhancements to error handling mechanisms could provide more informative and actionable error messages when queries exceed the maximum length, helping developers troubleshoot and optimize their queries more effectively.
5. Automatic Query Optimization: Advances in query optimization algorithms could improve the performance of long queries by automatically optimizing the query plans based on the characteristics of the data and workload.
It is important to stay updated with the latest PostgreSQL releases and follow the release notes to be aware of any enhancements or developments related to SQL query length.
Related Article: Preventing Locking Queries in Read-Only PostgreSQL Databases
Additional Resources
– PostgreSQL Documentation
– PostgreSQL Documentation – Query Size Limit
– PostgreSQL Documentation – Maximum identifier length