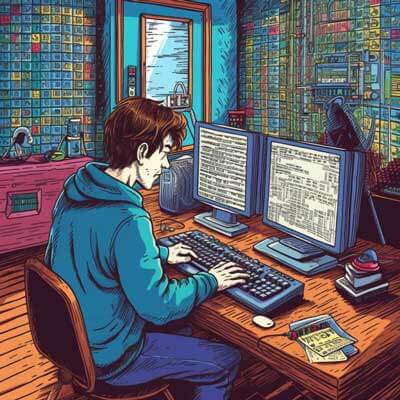
To find the maximum value of an integer in Java, you can use the Integer.MAX_VALUE
constant. This constant represents the largest possible value that an int
variable can hold. Here are two possible ways to find the max value of an integer in Java:
Using the Integer.MAX_VALUE constant
One straightforward way to find the max value of an integer in Java is by using the Integer.MAX_VALUE
constant. This constant represents the largest possible value that can be stored in an int
variable. Here is an example:
int maxValue = Integer.MAX_VALUE; System.out.println("The maximum value of an integer is: " + maxValue);
The above code snippet assigns the value of Integer.MAX_VALUE
to the maxValue
variable and then prints it to the console. When you run this code, you will see the maximum value of an integer displayed in the console.
Related Article: How to Convert JsonString to JsonObject in Java
Using the Math.max() method
Another way to find the max value of an integer in Java is by using the Math.max()
method. This method returns the larger of the two arguments passed to it. By passing Integer.MAX_VALUE
and any other integer value as arguments, you can determine the maximum value. Here is an example:
int maxValue = Math.max(Integer.MAX_VALUE, 0); System.out.println("The maximum value of an integer is: " + maxValue);
In the above code snippet, the Math.max()
method is used to compare Integer.MAX_VALUE
and 0
. Since Integer.MAX_VALUE
is the largest possible value, it will be returned as the maximum value. The code then prints the result to the console.
Best practices
– When working with integers in Java, it is essential to be aware of the maximum and minimum values that can be stored in an int
variable. The Integer.MAX_VALUE
constant represents the maximum value, while Integer.MIN_VALUE
represents the minimum value.
– It is crucial to handle potential overflow issues when dealing with large numbers. Java provides alternative data types like Long
for storing larger values than what int
can hold.
– When comparing integers, consider using the Math.max()
method instead of writing custom logic. This approach improves code readability and reduces the chance of introducing bugs.
For more information on working with integers in Java, you can refer to the official Java documentation on the Integer class: Integer Documentation.
Related Article: How to Implement a Delay in Java Using java wait seconds