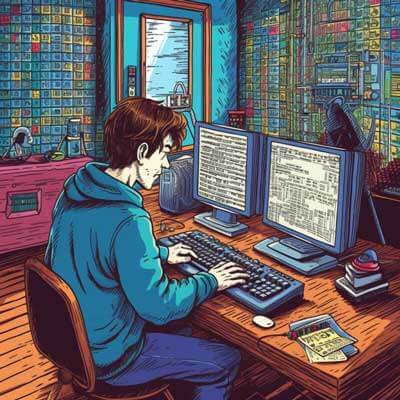
Table of Contents
When creating scatter plots using pyplot in Python, you may want to adjust the size of the markers to better visualize your data. This can be done easily by specifying the marker size parameter in the plt.scatter() function. In this guide, we will explore different ways to adjust the marker size in a scatter plot using Python.
Method 1: Using the s parameter
One way to adjust the marker size in a scatter plot is by using the 's' parameter in the plt.scatter() function. The 's' parameter allows you to specify the size of the markers in points.
Here's an example that demonstrates how to adjust the marker size using the 's' parameter:
import matplotlib.pyplot as plt x = [1, 2, 3, 4, 5] y = [6, 7, 8, 9, 10] marker_size = [50, 100, 150, 200, 250] plt.scatter(x, y, s=marker_size) plt.show()
In this example, we have defined three lists: 'x', 'y', and 'marker_size'. The 'x' and 'y' lists represent the x and y coordinates of the data points, and the 'marker_size' list specifies the size of each marker.
Related Article: How To Manually Raise An Exception In Python
Method 2: Using the size parameter
Another way to adjust the marker size in a scatter plot is by using the 'size' parameter in the plt.scatter() function. The 'size' parameter allows you to specify the size of the markers in pixels.
Here's an example that demonstrates how to adjust the marker size using the 'size' parameter:
import matplotlib.pyplot as plt x = [1, 2, 3, 4, 5] y = [6, 7, 8, 9, 10] marker_size = [50, 100, 150, 200, 250] plt.scatter(x, y, size=marker_size) plt.show()
In this example, we have defined the same 'x', 'y', and 'marker_size' lists as in the previous example. However, instead of using the 's' parameter, we use the 'size' parameter to specify the marker size in pixels.
Best Practices
Related Article: Implementing a cURL command in Python
When adjusting the marker size in a scatter plot, it is important to consider the following best practices:
1. Choose an appropriate marker size: The marker size should be chosen based on the data and the desired visual representation. Too small marker sizes may make it difficult to distinguish individual data points, while too large marker sizes may lead to cluttered plots.
2. Use consistent marker sizes: If you are comparing multiple scatter plots or displaying different data sets on the same plot, it is important to use consistent marker sizes across the plots. This will make it easier for viewers to compare and interpret the data.
3. Consider other visual cues: Marker size is just one aspect of a scatter plot's visual representation. Consider other visual cues such as color, shape, and transparency to convey additional information or highlight specific patterns in the data.