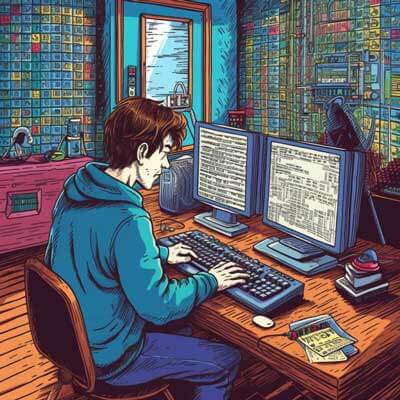
To replace regex patterns in Python, you can use the re
module, which provides functions for working with regular expressions. The re.sub()
function is particularly useful for replacing regex patterns in strings.
Here are two possible ways to replace regex patterns in Python:
Using re.sub()
The re.sub()
function allows you to replace occurrences of a regex pattern in a string with a specified replacement. The syntax for using re.sub()
is as follows:
re.sub(pattern, replacement, string, count=0, flags=0)
– pattern
: The regex pattern to be replaced.
– replacement
: The string to replace the matching occurrences of the pattern.
– string
: The input string in which to perform the replacement.
– count
(optional): The maximum number of replacements to make. If omitted or set to 0, all occurrences will be replaced.
– flags
(optional): Additional flags that modify the behavior of the pattern matching.
Here’s an example that demonstrates the usage of re.sub()
:
import re string = "Hello, World! How are you?" pattern = r"[aeiou]" replacement = "*" new_string = re.sub(pattern, replacement, string) print(new_string) # Output: "H*ll*, W*rld! H*w *r* y**?"
In this example, the regex pattern [aeiou]
matches any vowel in the input string. The occurrences of the vowels are replaced with asterisks using the re.sub()
function.
Related Article: How To Limit Floats To Two Decimal Points In Python
Using regex groups and backreferences
Another approach to replacing regex patterns in Python is by using regex groups and backreferences. This allows you to capture parts of the matched pattern and include them in the replacement string.
To define a group in a regex pattern, you can enclose the desired part of the pattern in parentheses ()
. You can then refer to the captured groups using backreferences in the replacement string.
Here’s an example that demonstrates the usage of regex groups and backreferences:
import re string = "Hello, World!" pattern = r"(Hello), (World)" replacement = r"\2, \1" new_string = re.sub(pattern, replacement, string) print(new_string) # Output: "World, Hello!"
In this example, the regex pattern (Hello), (World)
captures the words “Hello” and “World” as separate groups. In the replacement string r"\2, \1"
, the backreferences \2
and \1
refer to the second and first captured groups respectively. This swaps the positions of “Hello” and “World” in the output string.
Reasons for using regex replacements in Python
The question of how to replace regex in Python may arise for various reasons. Some potential reasons include:
– Data cleaning and transformation: When working with textual data, there may be a need to clean or transform it based on specific patterns. Regular expressions provide a powerful and flexible way to define these patterns and perform replacements.
– Text processing and parsing: Regular expressions are commonly used for text processing tasks such as extracting specific information from a text or splitting a string into meaningful parts. In many cases, replacing certain patterns or segments of a string is a crucial step in achieving the desired parsing or processing outcome.
– String manipulation and formatting: Regex replacements can be useful for modifying the format or structure of strings. For example, you may want to reformat dates or numbers in a specific way, or replace certain substrings with different values.
Best practices and considerations
When working with regex replacements in Python, consider the following best practices:
– Use raw strings (r"..."
) for regex patterns and replacements to avoid unwanted escape sequences. Raw strings treat backslashes as literal characters, which is important for regex patterns that often contain backslashes.
– Test your regex patterns thoroughly to ensure they match the desired parts of the string. Python’s re
module provides various flags that can modify the pattern matching behavior. Be aware of these flags and use them when appropriate.
– When the replacement string involves backreferences, make sure to escape any backslashes that are meant to be literal characters. This can be done by using double backslashes (\\
).
– Consider the performance implications of your regex patterns, especially when dealing with large strings or processing a large number of strings. Complex patterns can be computationally expensive and may lead to slower execution times.
– If you need to perform multiple regex replacements on the same string, it may be more efficient to compile the regex pattern using re.compile()
and reuse the compiled pattern object.
– In cases where the replacements are more complex or involve dynamic logic, consider using a callback function with re.sub()
. This allows you to define custom logic for the replacement based on the matched pattern.
Related Article: How To Rename A File With Python
Alternative ideas and suggestions
While using re.sub()
is a common and effective way to replace regex patterns in Python, there are alternative approaches and libraries available that you may consider depending on your specific requirements:
– If you need to perform more advanced text processing tasks, consider using the regex
module, which provides additional features and syntax compared to the standard re
module. The regex
module supports more powerful regex capabilities, including recursive patterns, named groups, and lookarounds.
– If your regex replacements involve complex transformations or involve multiple steps, you might benefit from using a parsing library like pyparsing
or a string manipulation library like textwrap
or stringtemplate
instead of solely relying on regex patterns.
– In some cases, it may be more appropriate to use string methods or other string manipulation functions provided by Python’s standard library instead of regular expressions. For simple replacements or known patterns, using string methods like str.replace()
or str.translate()
can be more efficient and readable.
– If your primary goal is to simply remove or replace specific characters or substrings in a string, you can also use Python’s built-in string methods like str.replace()
or str.translate()
instead of regular expressions. This can be particularly useful for cases where the replacement pattern is fixed and does not require the flexibility of regex.