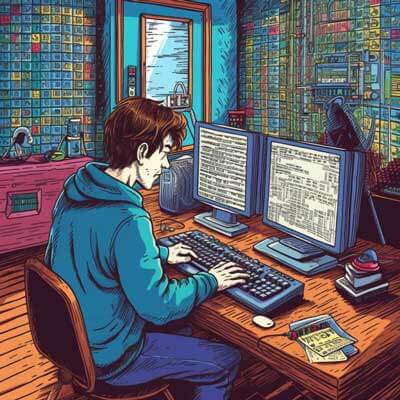
- i18n and Flask-Babel
- Implementing Internationalization and Localization with Flask-Babel
- Translations and Message Catalogs
- Pluralization
- Strategies for Handling Multi-language Data Storage and Retrieval
- Database Encoding
- Unicode Strings in Python
- Character Encoding in HTTP Requests and Responses
- Understanding Unicode and its Importance in Flask
- Flask’s Handling of Different Character Encodings
- The Difference Between UTF-8 and UTF-16
- Exploring Byte Order Marks (BOM) and their Relevance in Text Encoding
- Best Practices for Encoding and Decoding Text Data in Flask
- Use Unicode Strings
- Specify Character Encoding in HTTP Responses
- Use the Correct Character Encoding for Database Storage
- Handle Input Data Correctly
- Leveraging Flask-Babel for Internationalization and Localization in Flask
- Translations and Message Catalogs
- Pluralization
- Date and Number Formatting
- Common Challenges with Multi-language Data in Flask
- Character Encoding Mismatch
- Handling Unicode Strings
- Translating Text and Managing Translations
- Handling Pluralization
- Date and Number Formatting
- Additional Resources
i18n and Flask-Babel
Internationalization, often abbreviated as i18n, is the process of designing and adapting software to support multiple languages and locales. It involves translating user interfaces, messages, and content into different languages, as well as adapting formats, date and time representations, and other cultural aspects to suit diverse regions and countries.
Flask-Babel is a popular Python library that simplifies the process of implementing internationalization and localization in Flask applications. It provides a set of tools and utilities for managing translations, handling pluralization, formatting dates and numbers, and more. With Flask-Babel, developers can easily create multilingual applications that cater to a global audience.
To get started with Flask-Babel, you’ll need to install it in your Flask project. You can do this using pip, the Python package installer, by running the following command:
pip install Flask-Babel
Once Flask-Babel is installed, you can import it into your Flask application and initialize it with the app object. Here’s an example of how to do this:
from flask import Flask from flask_babel import Babel app = Flask(__name__) babel = Babel(app)
Related Article: How To Exit Python Virtualenv
Implementing Internationalization and Localization with Flask-Babel
Flask-Babel makes it easy to implement internationalization and localization in your Flask application. It provides a simple API for managing translations and handling language-specific content. Let’s explore some of the key features of Flask-Babel and how to use them effectively.
Translations and Message Catalogs
At the heart of Flask-Babel is the concept of translations and message catalogs. A message catalog is a collection of translated strings for a specific language. These strings are organized into message keys, which serve as identifiers for the translated content.
To create and manage translations in Flask-Babel, you’ll need to use the gettext
function. This function takes a message key as input and returns the translated string for the current language. Here’s an example of how to use gettext
in your Flask application:
from flask_babel import gettext @app.route('/') def hello(): message = gettext('Hello, World!') return message
In the example above, the gettext
function is used to translate the message key 'Hello, World!'
into the appropriate language-specific string. The translated string is then returned as the response from the hello
route.
To provide translations for different languages, you’ll need to create message catalogs for each language. These catalogs are typically stored in .po
files, which are human-readable files that contain the message keys and their corresponding translations.
Flask-Babel provides a command-line interface for managing message catalogs. You can use the pybabel
command to extract messages from your Flask application, initialize message catalogs for different languages, and update existing catalogs with new translations.
Here’s an example of how to extract messages from your Flask application and initialize a message catalog for a specific language:
pybabel extract -F babel.cfg -o messages.pot . pybabel init -i messages.pot -d translations -l fr
In the example above, the pybabel extract
command is used to extract messages from the Flask application and generate a .pot
file, which serves as a template for the translations. The pybabel init
command is then used to initialize a message catalog for the French language (-l fr
), using the .pot
file as the basis.
Once you have initialized the message catalog, you can start adding translations for the message keys. This can be done manually by editing the .po
file, or you can use translation services or tools to assist with the process.
Pluralization
Pluralization is a common requirement in internationalization, as different languages have different rules for plural forms. Flask-Babel provides a convenient ngettext
function for handling pluralization in your Flask application.
The ngettext
function takes three arguments: the singular form of the message, the plural form of the message, and the number that determines the plural form. Here’s an example of how to use ngettext
in your Flask application:
from flask_babel import ngettext @app.route('/products/') def products(num): message = ngettext('1 product', '{} products', num).format(num) return message
In the example above, the ngettext
function is used to handle pluralization for the message '1 product'
and '{} products'
. The num
variable is used to determine the appropriate plural form, and the resulting message is returned as the response from the products
route.
Related Article: How to Integrate Python with MySQL for Database Queries
Strategies for Handling Multi-language Data Storage and Retrieval
When working with multi-language data in Flask, it’s important to consider how you store and retrieve that data to ensure proper handling of different languages and character encodings. Here are some strategies to consider when dealing with multi-language data in Flask:
Database Encoding
One of the first considerations when handling multi-language data is the encoding used by your database. It’s important to ensure that your database is configured to use a character encoding that supports the languages and characters you are working with.
For example, if you are working with languages that use non-Latin characters, such as Chinese or Arabic, you may need to use a Unicode encoding like UTF-8 or UTF-16. These encodings can handle a wide range of characters from different languages and are well-supported by most modern databases.
To configure the encoding for your database, you will typically need to set the character set and collation options when creating your database tables. Consult your database documentation for specific instructions on how to set the encoding for your database.
Unicode Strings in Python
In Python, Unicode strings are used to represent text data that may contain characters from different languages and character sets. When working with multi-language data in Flask, it’s important to ensure that your strings are properly encoded as Unicode.
To create a Unicode string in Python, you can use the u
prefix before the string literal. Here’s an example:
text = u'Hello, 世界!'
In the example above, the u
prefix indicates that the string 'Hello, 世界!'
should be treated as a Unicode string. This allows the string to contain characters from different languages and character sets.
When working with multi-language data in Flask, it’s important to ensure that your strings are properly encoded as Unicode throughout your application. This includes any input data from users, as well as any data retrieved from your database or external sources.
Related Article: 16 Amazing Python Libraries You Can Use Now
Character Encoding in HTTP Requests and Responses
When sending and receiving data over HTTP in Flask, it’s important to consider the character encoding used in the requests and responses. By default, Flask uses the UTF-8 character encoding for both requests and responses, which is a widely supported encoding that can handle a wide range of characters from different languages.
When handling multi-language data in Flask, you should ensure that your HTTP requests and responses are properly encoded using the appropriate character encoding. This can be done by setting the Content-Type
header in your responses to specify the character encoding used, and by configuring your HTTP client to use the appropriate character encoding for requests.
Here’s an example of how to set the Content-Type
header in a Flask response to specify the UTF-8 character encoding:
from flask import Flask, Response app = Flask(__name__) @app.route('/') def hello(): message = u'Hello, 世界!' return Response(message, content_type='text/plain; charset=utf-8')
In the example above, the content_type
parameter of the Response
object is set to 'text/plain; charset=utf-8'
, which specifies that the response should be encoded using the UTF-8 character encoding.
Understanding Unicode and its Importance in Flask
Unicode is a character encoding standard that aims to represent all characters from all writing systems in a consistent and unambiguous way. It provides a unique code point for each character, allowing different languages and characters to be represented and processed correctly.
In Flask, Unicode is important for handling multi-language data and ensuring that text data is properly encoded and decoded. By using Unicode, Flask can handle characters from different languages and character sets, allowing you to create multilingual applications that cater to a global audience.
Flask uses Unicode strings internally to represent text data. This allows Flask to handle characters from different languages and character sets, and ensures that text data is properly encoded and decoded throughout the application.
When working with text data in Flask, it’s important to ensure that your strings are properly encoded as Unicode. This includes any input data from users, as well as any data retrieved from your database or external sources.
To create a Unicode string in Flask, you can use the u
prefix before the string literal. Here’s an example:
text = u'Hello, 世界!'
In the example above, the u
prefix indicates that the string 'Hello, 世界!'
should be treated as a Unicode string. This allows the string to contain characters from different languages and character sets.
Flask’s Handling of Different Character Encodings
Flask is built on top of the Werkzeug WSGI library, which provides a useful and flexible framework for handling HTTP requests and responses. Werkzeug includes support for handling different character encodings, allowing Flask to handle multi-language data and ensure that text data is properly encoded and decoded.
When handling HTTP requests in Flask, Werkzeug automatically decodes the request body using the character encoding specified in the request headers. This allows Flask to work with text data in its native Unicode form, regardless of the character encoding used in the request.
When sending HTTP responses in Flask, Werkzeug automatically encodes the response body using the character encoding specified in the Content-Type
header. This ensures that the response is properly encoded and can be correctly interpreted by the client.
Flask also provides a convenient request.form
object for accessing form data submitted in POST requests. By default, Flask automatically decodes the form data using the character encoding specified in the request headers, allowing you to work with the form data as Unicode strings.
Overall, Flask’s handling of different character encodings is transparent and seamless, allowing you to focus on developing your application without having to worry about the intricacies of character encoding.
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
The Difference Between UTF-8 and UTF-16
UTF-8 and UTF-16 are both character encodings that can represent characters from different languages and character sets. However, they differ in how they encode and represent characters, as well as in their storage efficiency and compatibility with existing systems.
UTF-8 is a variable-length encoding that uses 8-bit code units to represent characters. It can represent the entire Unicode character set using one to four bytes, depending on the character. UTF-8 is backward-compatible with ASCII, as the first 128 characters in the Unicode character set correspond to the ASCII character set.
UTF-16, on the other hand, is a fixed-length encoding that uses 16-bit code units to represent characters. It can represent the entire Unicode character set using one or two 16-bit code units, depending on the character. UTF-16 is not backward-compatible with ASCII, as it uses two bytes to represent ASCII characters.
The main difference between UTF-8 and UTF-16 lies in their storage efficiency. UTF-8 is more efficient for representing ASCII characters, as they only require one byte, whereas UTF-16 requires two bytes for all characters. However, UTF-16 is more efficient for representing non-ASCII characters, as they can be represented using two bytes, whereas UTF-8 requires three or four bytes.
Another difference between UTF-8 and UTF-16 is their compatibility with existing systems and protocols. UTF-8 is widely supported and is the default character encoding for many systems and protocols, including HTTP and HTML. UTF-16, on the other hand, is less commonly used and may require additional configuration and handling to ensure proper compatibility.
When working with multi-language data in Flask, it’s important to consider the character encoding used by your database and external systems. If your database uses UTF-8, it’s recommended to use UTF-8 in your Flask application to ensure compatibility and consistency. However, if you are working with systems that use UTF-16, you may need to configure your Flask application accordingly.
Exploring Byte Order Marks (BOM) and their Relevance in Text Encoding
A Byte Order Mark (BOM) is a special Unicode character that is used to indicate the byte order of a text file or stream encoded in UTF-16 or UTF-32. It consists of a sequence of bytes at the beginning of the file that serves as a signature to identify the encoding and byte order used.
In UTF-16, the BOM is represented by the character U+FEFF (ZERO WIDTH NO-BREAK SPACE). In UTF-32, the BOM is represented by the character U+0000FEFF (ZERO WIDTH NO-BREAK SPACE).
The presence of a BOM at the beginning of a text file or stream can be used by applications to determine the encoding and byte order used. However, the use of BOMs is not required for UTF-8 encoding, as UTF-8 does not have different byte orders.
In the context of Flask and text encoding, BOMs are typically not relevant, as Flask uses UTF-8 as the default character encoding. UTF-8 does not require a BOM to determine the encoding, as it uses a variable-length encoding scheme that can be identified based on the byte patterns of the encoded characters.
However, when working with external systems or libraries that expect a BOM, it may be necessary to include a BOM at the beginning of your text files or streams. This can be done by explicitly encoding the text data using the appropriate encoding and including the BOM character at the beginning.
For example, if you need to generate a CSV file with UTF-16 encoding and a BOM, you can use the utf-16
encoding and include the BOM character at the beginning of the file. Here’s an example of how to do this in Flask:
from flask import Flask, Response app = Flask(__name__) @app.route('/csv') def csv(): data = u'1,2,3\n4,5,6\n' bom = u'\ufeff' response = Response(bom + data, content_type='text/csv; charset=utf-16') return response
In the example above, the bom
variable contains the BOM character for UTF-16 encoding. The data
variable contains the CSV data. The Response
object is then created with the BOM character followed by the data, and the content_type
parameter is set to 'text/csv; charset=utf-16'
to specify the UTF-16 encoding.
Best Practices for Encoding and Decoding Text Data in Flask
When working with text data in Flask, it’s important to follow best practices for encoding and decoding to ensure that your data is properly handled and can be correctly interpreted by your application and external systems.
Here are some best practices for encoding and decoding text data in Flask:
Related Article: Converting Integer Scalar Arrays To Scalar Index In Python
Use Unicode Strings
Unicode strings should be used to represent text data in Flask. By using Unicode strings, you can ensure that your strings can handle characters from different languages and character sets, and that they are properly encoded and decoded throughout your application.
To create a Unicode string in Flask, use the u
prefix before the string literal. Here’s an example:
text = u'Hello, 世界!'
In the example above, the u
prefix indicates that the string 'Hello, 世界!'
should be treated as a Unicode string.
Specify Character Encoding in HTTP Responses
When sending text data in HTTP responses, it’s important to specify the character encoding used in the Content-Type
header. This ensures that the recipient knows how to interpret the response and can correctly decode the text data.
In Flask, you can set the Content-Type
header using the content_type
parameter of the Response
object. Here’s an example:
from flask import Flask, Response app = Flask(__name__) @app.route('/') def hello(): message = u'Hello, 世界!' return Response(message, content_type='text/plain; charset=utf-8')
In the example above, the content_type
parameter is set to 'text/plain; charset=utf-8'
, which specifies the UTF-8 character encoding.
Use the Correct Character Encoding for Database Storage
When working with a database in Flask, it’s important to ensure that the character encoding used by the database matches the encoding used by your application. This ensures that text data is stored and retrieved correctly, without any loss or corruption of data.
Most modern databases, including MySQL and PostgreSQL, support Unicode encodings like UTF-8, which can handle characters from different languages and character sets. When creating your database tables, make sure to specify the character encoding and collation options to match the encoding used by your application.
Related Article: How To Convert A Tensor To Numpy Array In Tensorflow
Handle Input Data Correctly
When handling input data from users, it’s important to ensure that the data is properly encoded and decoded to handle different languages and character sets. Flask takes care of decoding the request body for you, but you should still be aware of the encoding used and make sure to handle the data appropriately.
Flask provides the request.form
object for accessing form data submitted in POST requests. By default, Flask automatically decodes the form data using the character encoding specified in the request headers, allowing you to work with the form data as Unicode strings.
If you are working with file uploads, Flask provides the request.files
object for accessing the uploaded files. The files are automatically streamed and saved to a temporary location, but you should still be aware of the encoding used and handle the file contents appropriately.
Leveraging Flask-Babel for Internationalization and Localization in Flask
Flask-Babel is a useful library that simplifies the process of implementing internationalization and localization in Flask applications. It provides a set of tools and utilities for managing translations, handling pluralization, formatting dates and numbers, and more.
To leverage Flask-Babel for internationalization and localization in Flask, you’ll need to install it in your Flask project. You can do this using pip, the Python package installer, by running the following command:
pip install Flask-Babel
Once Flask-Babel is installed, you can import it into your Flask application and initialize it with the app object. Here’s an example of how to do this:
from flask import Flask from flask_babel import Babel app = Flask(__name__) babel = Babel(app)
Flask-Babel provides a number of features and utilities for managing translations and handling language-specific content. Here are some of the key features of Flask-Babel:
Translations and Message Catalogs
At the core of Flask-Babel is the concept of translations and message catalogs. A message catalog is a collection of translated strings for a specific language. These strings are organized into message keys, which serve as identifiers for the translated content.
Flask-Babel provides a gettext
function for managing translations. This function takes a message key as input and returns the translated string for the current language. Here’s an example of how to use gettext
in your Flask application:
from flask_babel import gettext @app.route('/') def hello(): message = gettext('Hello, World!') return message
In the example above, the gettext
function is used to translate the message key 'Hello, World!'
into the appropriate language-specific string. The translated string is then returned as the response from the hello
route.
To provide translations for different languages, you’ll need to create message catalogs for each language. These catalogs are typically stored in .po
files, which are human-readable files that contain the message keys and their corresponding translations.
Flask-Babel provides a command-line interface for managing message catalogs. You can use the pybabel
command to extract messages from your Flask application, initialize message catalogs for different languages, and update existing catalogs with new translations.
Here’s an example of how to extract messages from your Flask application and initialize a message catalog for a specific language:
pybabel extract -F babel.cfg -o messages.pot . pybabel init -i messages.pot -d translations -l fr
In the example above, the pybabel extract
command is used to extract messages from the Flask application and generate a .pot
file, which serves as a template for the translations. The pybabel init
command is then used to initialize a message catalog for the French language (-l fr
), using the .pot
file as the basis.
Once you have initialized the message catalog, you can start adding translations for the message keys. This can be done manually by editing the .po
file, or you can use translation services or tools to assist with the process.
Related Article: How to Normalize a Numpy Array to a Unit Vector in Python
Pluralization
Pluralization is a common requirement in internationalization, as different languages have different rules for plural forms. Flask-Babel provides a convenient ngettext
function for handling pluralization in your Flask application.
The ngettext
function takes three arguments: the singular form of the message, the plural form of the message, and the number that determines the plural form. Here’s an example of how to use ngettext
in your Flask application:
from flask_babel import ngettext @app.route('/products/') def products(num): message = ngettext('1 product', '{} products', num).format(num) return message
In the example above, the ngettext
function is used to handle pluralization for the message '1 product'
and '{} products'
. The num
variable is used to determine the appropriate plural form, and the resulting message is returned as the response from the products
route.
Date and Number Formatting
Flask-Babel also provides utilities for formatting dates and numbers according to the conventions of different locales. This allows you to present dates and numbers in a way that is familiar and readable to users from different regions and countries.
The format_date
function can be used to format dates, while the format_number
function can be used to format numbers. Here’s an example of how to use these functions in your Flask application:
from flask_babel import format_date, format_number from datetime import datetime @app.route('/date') def date(): today = datetime.now() formatted_date = format_date(today, format='short') return formatted_date @app.route('/number') def number(): amount = 1234.56 formatted_number = format_number(amount, locale='en_US') return formatted_number
In the example above, the format_date
function is used to format the current date (today
) into a short date format. The resulting formatted date is then returned as the response from the date
route.
Similarly, the format_number
function is used to format the amount
variable into a number format using the en_US
locale. The resulting formatted number is then returned as the response from the number
route.
Common Challenges with Multi-language Data in Flask
Handling multi-language data in Flask can present several challenges, particularly when it comes to character encodings, database storage, and user input. Here are some common challenges you may encounter when working with multi-language data in Flask:
Related Article: How to Adjust Font Size in a Matplotlib Plot
Character Encoding Mismatch
One of the most common challenges with multi-language data is a character encoding mismatch. This occurs when the character encoding used by your application does not match the character encoding used by your database or external systems.
To avoid character encoding mismatches, it’s important to ensure that your application, database, and external systems are all configured to use the same character encoding. This typically involves setting the appropriate character set and collation options when creating your database tables and configuring your application to use the same encoding.
Handling Unicode Strings
Working with Unicode strings can be challenging, especially when it comes to handling different languages and character sets. It’s important to ensure that your strings are properly encoded and decoded throughout your application to handle different languages and characters correctly.
In Flask, you can use Unicode strings to represent text data. By using Unicode strings, you can ensure that your strings can handle characters from different languages and character sets, and that they are properly encoded and decoded throughout your application.
Translating Text and Managing Translations
Managing translations can be a complex task, particularly when dealing with a large number of message keys and multiple languages. It’s important to have a system in place for managing translations and keeping them up to date as your application evolves.
Flask-Babel provides tools and utilities for managing translations, including a command-line interface for extracting messages from your Flask application, initializing message catalogs for different languages, and updating existing catalogs with new translations.
Related Article: How to Position the Legend Outside the Plot in Matplotlib
Handling Pluralization
Pluralization is another common challenge when working with multi-language data. Different languages have different rules for plural forms, and it’s important to handle pluralization correctly to ensure that your application displays the appropriate message for different quantities.
Flask-Babel provides a convenient ngettext
function for handling pluralization in your Flask application. This function takes the singular and plural forms of a message, as well as the number that determines the plural form, and returns the appropriate translated message.
Date and Number Formatting
Formatting dates and numbers according to the conventions of different locales can also be a challenge when working with multi-language data. It’s important to present dates and numbers in a way that is familiar and readable to users from different regions and countries.
Flask-Babel provides utilities for formatting dates and numbers according to the conventions of different locales. This allows you to present dates and numbers in a way that is familiar and readable to users from different regions and countries.