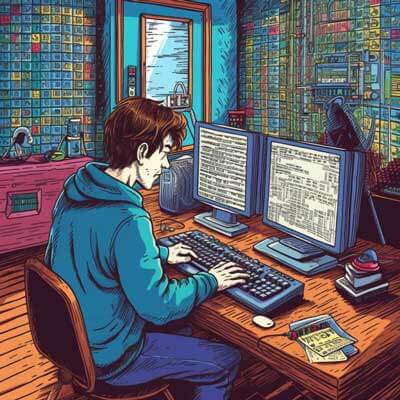
- Introduction to Time Sleep Function
- The Syntax of Time Sleep
- Use Cases: Slowing Down Loops
- Use Cases: Simulating Latency
- Use Cases: Scheduling Tasks
- Best Practices: Avoiding Infinite Sleep
- Best Practices: Using Time Sleep Responsibly
- Real World Example: Web Page Scraping
- Real World Example: Progress Bar
- Performance Considerations: Impact on CPU Usage
- Performance Considerations: Time Sleep vs Busy Waiting
- Advanced Techniques: Combining with Threading
- Advanced Techniques: Use in Asynchronous Programming
- Code Snippet: Basic Time Sleep Usage
- Code Snippet: Using Time Sleep in a Loop
- Code Snippet: Time Sleep with Threading
- Code Snippet: Time Sleep in Async IO
- Error Handling: Common Mistakes
- Error Handling: Exceptions and Time Sleep
Introduction to Time Sleep Function
The time.sleep()
function is a useful tool in Python for introducing delays or pauses in the execution of a program. It allows you to control the timing of your code, whether you want to slow down loops, simulate latency, schedule tasks, or perform other time-related operations. With time.sleep()
, you can add pauses between different actions, making your code more efficient and responsive.
Related Article: String Comparison in Python: Best Practices and Techniques
The Syntax of Time Sleep
The syntax for time.sleep()
is straightforward. It takes a single argument, which represents the number of seconds to pause the execution of the program. Here’s the syntax:
import time time.sleep(seconds)
The seconds
argument is a floating-point number indicating the duration of the pause. For example, time.sleep(2)
will pause the execution for 2 seconds.
Use Cases: Slowing Down Loops
One common use case of time.sleep()
is slowing down loops to control the rate at which iterations occur. Let’s say you have a loop that performs a certain task repeatedly, and you want to introduce a delay between iterations. Here’s an example:
import time for i in range(5): print("Iteration", i) time.sleep(1)
In this example, the loop will print “Iteration” followed by the current value of i
, and then pause for 1 second before moving on to the next iteration. This can be useful when you want to limit the speed of a loop, prevent overwhelming external resources, or create a more natural flow in your program.
Use Cases: Simulating Latency
Another use case for time.sleep()
is simulating latency, especially when testing network-related functionality. For instance, if you are developing a web application and want to test its behavior under different network conditions, you can use time.sleep()
to introduce artificial delays. Here’s an example:
import time print("Sending request...") time.sleep(3) print("Received response!")
In this example, the program will display “Sending request…”, pause for 3 seconds to simulate network latency, and then print “Received response!”. Simulating latency can help you identify and fix potential issues related to slow network connections or asynchronous behavior.
Related Article: How To Limit Floats To Two Decimal Points In Python
Use Cases: Scheduling Tasks
time.sleep()
can also be used for scheduling tasks within your program. For example, if you want to execute a specific function at a later time, you can combine time.sleep()
with a function call. Here’s an example:
import time def scheduled_task(): print("Executing scheduled task") print("Task scheduled for later") time.sleep(5) scheduled_task()
In this example, the program will print “Task scheduled for later”, pause for 5 seconds using time.sleep()
, and then execute the scheduled_task()
function. This can be helpful when you need to delay the execution of certain actions or synchronize tasks with external events.
Best Practices: Avoiding Infinite Sleep
While time.sleep()
is a powerful tool, it’s important to use it responsibly and avoid situations where your program gets stuck in an infinite sleep. For example, if you mistakenly call time.sleep()
with a large value or forget to include a condition for waking up, your program may appear unresponsive. It’s crucial to double-check the duration of your sleep and ensure that there’s a way to interrupt or exit the sleep when necessary.
Best Practices: Using Time Sleep Responsibly
To use time.sleep()
responsibly, consider the following best practices:
1. Always have a clear purpose for using time.sleep()
and ensure it aligns with your program’s logic.
2. Double-check the duration of the sleep to prevent unnecessary delays or excessive pauses.
3. Use conditional statements or other mechanisms to control when to exit the sleep, ensuring your program remains responsive.
4. Avoid using time.sleep()
in performance-critical sections of your code, as it can introduce unnecessary delays.
Related Article: How To Rename A File With Python
Real World Example: Web Page Scraping
Web scraping is a common task in which data is extracted from websites. time.sleep()
can be useful in web scraping scenarios to space out requests and avoid overwhelming the target server. Here’s an example of using time.sleep()
in a web scraping script:
import requests import time urls = ["https://example.com/page1", "https://example.com/page2", "https://example.com/page3"] for url in urls: response = requests.get(url) print("Scraping", url) # Process the response data time.sleep(2) # Pause for 2 seconds between requests
In this example, the script sends HTTP requests to multiple URLs in a loop, scraping data from each page. The time.sleep(2)
statement introduces a 2-second delay between requests, preventing rapid-fire requests that could trigger anti-scraping measures or cause server overload.
Real World Example: Progress Bar
When performing time-consuming operations, such as file downloads or large computations, you might want to provide a progress indication to the user. time.sleep()
can be used to control the speed at which the progress bar updates. Here’s an example:
import time total_progress = 100 for progress in range(total_progress): update_progress_bar(progress) time.sleep(0.1) # Pause for 0.1 seconds between updates
In this example, the loop updates a progress bar by calling the update_progress_bar()
function for each progress value. The time.sleep(0.1)
statement introduces a 0.1-second pause between updates, giving the user time to perceive the progress visually.
Performance Considerations: Impact on CPU Usage
It’s important to note that time.sleep()
does not consume CPU resources during the sleep period. Instead, it relinquishes the CPU to other processes or threads, allowing them to utilize the available resources. This can be beneficial in situations where you want to reduce CPU usage and avoid unnecessary computations while waiting for a specific condition or time interval.
Related Article: How To Check If List Is Empty In Python
Performance Considerations: Time Sleep vs Busy Waiting
When waiting for a condition to be met, it’s common to consider two approaches: using time.sleep()
or busy waiting. Busy waiting involves repeatedly checking the condition in a loop without any sleep or pause. While busy waiting can provide quicker responsiveness, it consumes CPU resources and can lead to high CPU usage. On the other hand, time.sleep()
allows the CPU to be used by other processes, resulting in lower CPU usage. However, it may introduce a slight delay before the condition is met.
Advanced Techniques: Combining with Threading
In more complex scenarios, time.sleep()
can be combined with threading to introduce delays in specific threads while allowing others to continue execution. This can be useful when coordinating multiple tasks or implementing time-based synchronization. Here’s an example of using time.sleep()
with threading:
import threading import time def task_a(): print("Task A started") time.sleep(2) print("Task A completed") def task_b(): print("Task B started") time.sleep(1) print("Task B completed") thread_a = threading.Thread(target=task_a) thread_b = threading.Thread(target=task_b) thread_a.start() thread_b.start() thread_a.join() thread_b.join() print("All tasks completed")
In this example, task_a()
and task_b()
are executed concurrently in separate threads. The time.sleep()
calls within each task introduce delays, allowing other threads to execute in the meantime. By using thread_a.join()
and thread_b.join()
, we ensure that the main thread waits for the completion of both tasks before printing “All tasks completed”.
Advanced Techniques: Use in Asynchronous Programming
time.sleep()
can also be used in combination with asynchronous programming techniques, such as asyncio, to introduce delays between asynchronous tasks. This can be helpful when coordinating the timing of multiple asynchronous operations. Here’s an example:
import asyncio async def task_a(): print("Task A started") await asyncio.sleep(2) print("Task A completed") async def task_b(): print("Task B started") await asyncio.sleep(1) print("Task B completed") async def main(): await asyncio.gather(task_a(), task_b()) asyncio.run(main())
In this example, task_a()
and task_b()
are defined as asynchronous functions, and asyncio.sleep()
is used instead of time.sleep()
to introduce the delays. The asyncio.gather()
function is used to concurrently execute both tasks, and asyncio.run()
is used to run the main()
coroutine.
Related Article: How To Check If a File Exists In Python
Code Snippet: Basic Time Sleep Usage
Here’s a basic code snippet demonstrating the usage of time.sleep()
:
import time print("Before sleep") time.sleep(2) print("After sleep")
This code will print “Before sleep”, pause for 2 seconds using time.sleep()
, and then print “After sleep”.
Code Snippet: Using Time Sleep in a Loop
Here’s an example of using time.sleep()
in a loop to introduce a delay between iterations:
import time for i in range(5): print("Iteration", i) time.sleep(1)
In this code snippet, the loop will print “Iteration” followed by the current value of i
, and then pause for 1 second before moving on to the next iteration.
Code Snippet: Time Sleep with Threading
Here’s an example of combining time.sleep()
with threading to introduce delays in specific threads:
import threading import time def task_a(): print("Task A started") time.sleep(2) print("Task A completed") def task_b(): print("Task B started") time.sleep(1) print("Task B completed") thread_a = threading.Thread(target=task_a) thread_b = threading.Thread(target=task_b) thread_a.start() thread_b.start() thread_a.join() thread_b.join() print("All tasks completed")
In this code snippet, task_a()
and task_b()
are executed concurrently in separate threads. The time.sleep()
calls within each task introduce delays, allowing other threads to execute in the meantime. The main thread waits for the completion of both tasks using thread_a.join()
and thread_b.join()
, and then prints “All tasks completed”.
Related Article: How to Use Inline If Statements for Print in Python
Code Snippet: Time Sleep in Async IO
Here’s an example of using time.sleep()
in combination with asyncio to introduce delays between asynchronous tasks:
import asyncio async def task_a(): print("Task A started") await asyncio.sleep(2) print("Task A completed") async def task_b(): print("Task B started") await asyncio.sleep(1) print("Task B completed") async def main(): await asyncio.gather(task_a(), task_b()) asyncio.run(main())
In this code snippet, task_a()
and task_b()
are defined as asynchronous functions, and asyncio.sleep()
is used instead of time.sleep()
to introduce the delays. The asyncio.gather()
function is used to concurrently execute both tasks, and asyncio.run()
is used to run the main()
coroutine.
Error Handling: Common Mistakes
When using time.sleep()
, it’s important to be aware of common mistakes that can lead to unexpected behavior or errors. Some common mistakes include:
1. Forgetting to import the time
module: Make sure to include the import time
statement at the beginning of your script or module when using time.sleep()
.
2. Using time.sleep()
in performance-critical sections: Avoid using time.sleep()
in sections of code where performance is crucial, as it can introduce unnecessary delays.
3. Using time.sleep()
with negative values: The time.sleep()
function expects a non-negative argument. Using a negative value will result in a ValueError
.
Error Handling: Exceptions and Time Sleep
When using time.sleep()
, it’s important to handle any exceptions that may occur during the sleep period. For example, if an interrupt signal is received while sleeping, the program should gracefully handle the interruption. Here’s an example of handling exceptions with time.sleep()
:
import time try: print("Before sleep") time.sleep(5) print("After sleep") except KeyboardInterrupt: print("Sleep interrupted by user")
In this example, if the user interrupts the sleep by pressing Ctrl+C, a KeyboardInterrupt
exception is raised. The except KeyboardInterrupt
block catches the exception and handles it by printing “Sleep interrupted by user”.