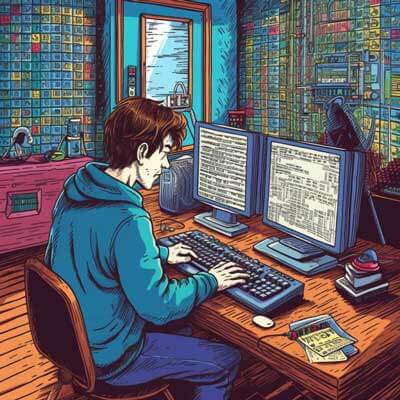
To convert an integer scalar array to a scalar index in Python, you can use the item
method, which returns the value of a single element in a scalar array. This allows you to access and use a single value from the array as a scalar index.
Here are two possible ways to convert an integer scalar array to a scalar index in Python:
Method 1: Using the item
method
The item
method is a built-in method in Python’s NumPy library that allows you to convert a scalar array to a scalar index. Here’s an example:
import numpy as np # Create an integer scalar array array = np.array(5) # Convert the scalar array to a scalar index index = array.item() # Print the scalar index print(index)
This will output:
5
In this example, we create an integer scalar array array
with the value 5
. We then use the item
method to convert the scalar array to a scalar index and store it in the variable index
. Finally, we print the value of the scalar index, which is 5
.
Related Article: How To Exit Python Virtualenv
Method 2: Using indexing
Another way to convert an integer scalar array to a scalar index in Python is by using indexing. Since a scalar array contains only a single value, you can directly access that value using indexing. Here’s an example:
# Create an integer scalar array array = 10 # Convert the scalar array to a scalar index index = array # Print the scalar index print(index)
This will output:
10
In this example, we directly assign the value 10
to the variable array
, which is already a scalar array. We then assign the value of array
to the variable index
, effectively converting the scalar array to a scalar index. Finally, we print the value of the scalar index, which is 10
.
Why is this question asked?
The question “How to convert integer scalar arrays to scalar index in Python?” may arise in scenarios where you have an integer scalar array and you need to extract the single value from it to use as a scalar index. This can be useful in various programming tasks, such as accessing specific elements in an array or performing computations based on a single value within an array.
Potential reasons for asking this question could include:
1. Needing to access a specific element in an array: When working with arrays, you may often need to access a particular element based on its index. By converting an integer scalar array to a scalar index, you can easily access and manipulate specific elements in the array.
2. Performing computations using a single value: In some cases, you may only require a single value from an array to perform a computation or make a decision. By converting the integer scalar array to a scalar index, you can extract the necessary value and use it in your calculations or logic.
Suggestions and alternative ideas
If you frequently find yourself needing to convert integer scalar arrays to scalar indices, here are some suggestions and alternative ideas that may help:
1. Avoid using scalar arrays when possible: If you only need a single value, consider using a scalar variable instead of a scalar array. This can simplify your code and eliminate the need for conversion.
2. Use indexing directly: As shown in the second method above, you can directly assign the value of a scalar array to a scalar index. This avoids the need for an explicit conversion step.
3. Check array dimensions: Before attempting to convert an array to a scalar index, ensure that the array is indeed a scalar array. If the array has multiple dimensions or contains multiple values, you may need to reshape or manipulate the array before extracting a scalar index.
Related Article: How to Integrate Python with MySQL for Database Queries
Example of best practices
To ensure clarity and maintainability in your code, consider following these best practices when converting integer scalar arrays to scalar indices in Python:
1. Use meaningful variable names: Choose descriptive names for your variables to enhance readability and make your code more understandable. Avoid using generic names like array
or index
unless they accurately convey the purpose of the variable.
2. Add comments to explain intent: If the purpose of converting an integer scalar array to a scalar index is not immediately obvious, consider adding comments to explain why the conversion is necessary and how the resulting scalar index will be used.
3. Handle potential errors: When working with arrays, it’s important to handle potential errors that may arise from incorrect dimensions or unexpected data types. Use appropriate error handling techniques, such as try-except blocks, to handle exceptions and ensure that your code behaves as expected.