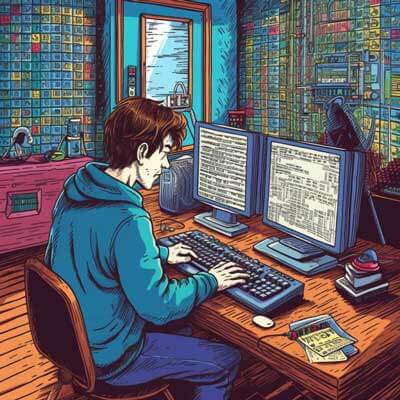
- Implementing Single Sign-On (SSO) with OAuth2 providers in FastAPI
- Integrating Role-Based Access Control (RBAC) with Enterprise Directory Services
- Best Practices for Implementing Audit Trails in FastAPI Apps
- Compliance Considerations in FastAPI App Development
- FastAPI Authentication and Authorization Mechanisms
- Python Libraries for Single Sign-On (SSO) with OAuth2 Providers
- FastAPI Integration with Enterprise Directory Services
- Benefits of Role-Based Access Control (RBAC) in FastAPI Apps
- FastAPI Plugins and Extensions for Implementing Audit Trails
- Legal and Regulatory Requirements for Compliance in FastAPI App Development
- Additional Resources
Implementing Single Sign-On (SSO) with OAuth2 providers in FastAPI
Single Sign-On (SSO) is a popular authentication mechanism that allows users to log in once and gain access to multiple applications without the need to provide credentials again. FastAPI, a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints, provides built-in support for implementing SSO with OAuth2 providers.
To implement SSO with OAuth2 providers in FastAPI, you can use the fastapi_auth
library, which provides an easy-to-use interface for handling OAuth2 authentication. This library supports various OAuth2 providers such as Google, Facebook, and GitHub. Here’s an example of how to implement SSO with Google as the OAuth2 provider:
First, install the fastapi_auth
library using pip:
pip install fastapi_auth
Next, create a new file called oauth.py
and add the following code:
from fastapi import Depends, FastAPI from fastapi_auth import AuthJWT app = FastAPI() authjwt = AuthJWT(app) @app.get("/") def homepage(): return {"message": "Welcome to the homepage!"} @app.get("/login") def login(auth=Depends(authjwt)): return auth.authorize_redirect() @app.get("/callback") def callback(auth=Depends(authjwt)): auth.authorize_access_token() return {"message": "Successfully logged in!"}
In the above code, we import the necessary dependencies from fastapi
and fastapi_auth
. We create a FastAPI app and initialize the AuthJWT
instance. The /login
endpoint redirects the user to the Google OAuth2 login page, and the /callback
endpoint handles the OAuth2 callback after successful authentication.
To start the FastAPI server, run the following command:
uvicorn oauth:app --reload
Now, when you visit http://localhost:8000/login
, you will be redirected to the Google OAuth2 login page. After successful authentication, you will be redirected to http://localhost:8000/callback
and see the message “Successfully logged in!”.
Related Article: How To Exit Python Virtualenv
Integrating Role-Based Access Control (RBAC) with Enterprise Directory Services
Role-Based Access Control (RBAC) is a widely-used authorization mechanism that provides fine-grained control over user permissions based on their roles within an organization. FastAPI allows seamless integration with enterprise directory services such as LDAP and Active Directory to implement RBAC.
To integrate RBAC with enterprise directory services in FastAPI, you can use the python-ldap
library, which provides a Python interface to LDAP directory servers. Here’s an example of how to integrate RBAC with LDAP:
First, install the python-ldap
library using pip:
pip install python-ldap
Next, create a new file called rbac.py
and add the following code:
import ldap def authenticate(username, password): ldap_server = "ldap://example.com" ldap_base_dn = "dc=example,dc=com" try: ldap_conn = ldap.initialize(ldap_server) ldap_conn.simple_bind_s(f"cn={username},{ldap_base_dn}", password) ldap_conn.unbind_s() return True except ldap.INVALID_CREDENTIALS: return False except ldap.SERVER_DOWN: return False def is_user_admin(username): # Check if the user is an admin in the enterprise directory service return True if username == "admin" else False @app.get("/") def homepage(username: str, password: str): if authenticate(username, password): if is_user_admin(username): return {"message": "Welcome, admin!"} else: return {"message": "Welcome, user!"} else: return {"message": "Invalid credentials!"}
In the above code, we define the authenticate
function, which authenticates the user against the LDAP server using the provided username and password. We also define the is_user_admin
function, which checks if the user is an admin in the enterprise directory service.
The /
endpoint takes the username
and password
as query parameters and returns a welcome message based on the user’s role. If the user is an admin, the message “Welcome, admin!” is returned; otherwise, the message “Welcome, user!” is returned.
To start the FastAPI server, run the following command:
uvicorn rbac:app --reload
Now, when you visit http://localhost:8000/?username=admin&password=admin
, you will see the message “Welcome, admin!”. If you visit http://localhost:8000/?username=user&password=user
, you will see the message “Welcome, user!”.
Best Practices for Implementing Audit Trails in FastAPI Apps
Implementing audit trails in FastAPI apps is essential for tracking and recording user actions in order to maintain accountability, monitor compliance, and investigate security incidents. Here are some best practices for implementing audit trails in FastAPI apps:
1. Define Audit Log Model: Create a database model to represent the audit log entries. This model should contain fields such as the user ID, action performed, timestamp, and any relevant data associated with the action.
2. Log Actions: Capture and log relevant user actions throughout the application. This can be done by adding logging statements at critical points in your code where actions are performed or data is modified.
3. Include Relevant Information: Ensure that the audit log includes all relevant information about the user action, such as the user ID, IP address, request method, request URL, and any parameters or payload data.
4. Encrypt Sensitive Information: If the audit log includes sensitive information, such as personally identifiable information (PII), ensure that it is encrypted or obfuscated to protect user privacy.
5. Implement Log Retention and Rotation: Define a log retention policy that specifies how long audit logs should be retained. Implement log rotation to manage log file sizes and prevent them from growing indefinitely.
6. Centralize Log Storage: Store audit logs in a centralized location, such as a database or a dedicated logging service. This allows for easy searching, analysis, and reporting of audit log data.
7. Secure Access to Audit Logs: Restrict access to audit logs to only authorized personnel. Implement proper authentication and authorization mechanisms to ensure that only authorized users can access the audit log data.
Here’s an example of how to implement audit trails in FastAPI using SQLAlchemy as the ORM and PostgreSQL as the database:
First, install the necessary libraries using pip:
<a href="https://www.squash.io/troubleshooting-pip-install-failures-with-fastapi/">pip install fastapi</a> sqlalchemy databases[postgresql]
Next, create a new file called audit.py
and add the following code:
from fastapi import FastAPI from sqlalchemy import Column, DateTime, Integer, String, create_engine from sqlalchemy.ext.declarative import declarative_base from sqlalchemy.orm import sessionmaker app = FastAPI() Base = declarative_base() class AuditLog(Base): __tablename__ = "audit_logs" id = Column(Integer, primary_key=True, autoincrement=True) user_id = Column(Integer) action = Column(String) timestamp = Column(DateTime) engine = create_engine("postgresql://user:password@localhost/database") SessionLocal = sessionmaker(bind=engine, autocommit=False, autoflush=False) @app.get("/") def homepage(): session = SessionLocal() audit_log = AuditLog(user_id=1, action="Performed action", timestamp=datetime.now()) session.add(audit_log) session.commit() session.close() return {"message": "Action performed and logged successfully!"}
In the above code, we define the AuditLog
model using SQLAlchemy. We create an instance of the SessionLocal
class to manage database sessions. The /
endpoint adds a new audit log entry to the database and returns a success message.
To start the FastAPI server, run the following command:
uvicorn audit:app --reload
Now, when you visit http://localhost:8000/
, the action will be performed, and the audit log entry will be added to the database.
Compliance Considerations in FastAPI App Development
Developing FastAPI apps that comply with legal and regulatory requirements is crucial for organizations operating in industries such as healthcare, finance, and government. Here are some compliance considerations to keep in mind when developing FastAPI apps:
1. Data Privacy: Ensure that your FastAPI app handles personal data in compliance with applicable data protection laws, such as the General Data Protection Regulation (GDPR) in the European Union. Implement mechanisms to obtain user consent for data processing and provide users with control over their data.
2. Secure Communication: Protect sensitive data transmitted over the network by using secure communication protocols such as HTTPS. Implement proper encryption and authentication mechanisms to prevent unauthorized access to data.
3. Access Control: Implement robust authentication and authorization mechanisms to control access to sensitive features and data within your FastAPI app. Use strong password policies, multi-factor authentication, and role-based access control (RBAC) to enforce access control policies.
4. Audit Trails: Implement audit trails to track and record user actions within your FastAPI app. This helps with accountability, monitoring compliance, and investigating security incidents.
5. Vulnerability Management: Regularly scan your FastAPI app for security vulnerabilities and proactively address any identified issues. Keep all software components up to date with the latest security patches and follow secure coding practices.
6. Incident Response: Have a well-defined incident response plan in place to handle security incidents and data breaches. This includes procedures for identifying, containing, eradicating, and recovering from security incidents, as well as protocols for notifying affected individuals and regulatory authorities, if required.
7. Compliance Documentation: Maintain proper documentation of your FastAPI app’s compliance efforts, including policies, procedures, risk assessments, and training records. This documentation helps demonstrate compliance during audits and regulatory inspections.
Related Article: How to Integrate Python with MySQL for Database Queries
FastAPI Authentication and Authorization Mechanisms
FastAPI provides various built-in mechanisms for authentication and authorization, allowing you to secure your API endpoints and control access to resources. Here are some of the authentication and authorization mechanisms available in FastAPI:
1. JWT (JSON Web Tokens): FastAPI has built-in support for JWT authentication using the fastapi_jwt_auth
library. JWTs are secure and compact tokens that can be used to authenticate and authorize users. You can define JWT authentication using decorators in FastAPI routes.
2. OAuth2: FastAPI supports OAuth2 authentication, which allows users to authenticate with external providers such as Google, Facebook, and GitHub. You can use the fastapi_auth
library to implement OAuth2 authentication in your FastAPI app.
3. Basic Authentication: FastAPI supports Basic Authentication, which is a simple username and password-based authentication mechanism. You can use the fastapi.security.HTTPBasic
class to implement Basic Authentication in your FastAPI app.
4. Bearer Authentication: FastAPI supports Bearer Authentication, which is a token-based authentication mechanism commonly used with APIs. You can use the fastapi.security.HTTPBearer
class to implement Bearer Authentication in your FastAPI app.
5. Role-Based Access Control (RBAC): FastAPI allows you to implement RBAC, which provides fine-grained control over user permissions based on their roles. You can define roles and permissions using decorators in FastAPI routes.
Here’s an example of how to implement JWT authentication in FastAPI using the fastapi_jwt_auth
library:
First, install the necessary libraries using pip:
pip install fastapi fastapi_jwt_auth
Next, create a new file called auth.py
and add the following code:
from fastapi import Depends, FastAPI from fastapi_jwt_auth import AuthJWT app = FastAPI() authjwt = AuthJWT(app) @app.get("/login") def login(auth=Depends(authjwt)): access_token = auth.create_access_token(subject="user@example.com") return {"access_token": access_token} @app.get("/protected") def protected(auth=Depends(authjwt)): auth.jwt_required() current_user = auth.get_jwt_subject() return {"message": f"Welcome, {current_user}!"}
In the above code, we import the necessary dependencies from fastapi
and fastapi_jwt_auth
. We create a FastAPI app and initialize the AuthJWT
instance. The /login
endpoint generates and returns an access token using the create_access_token
method. The /protected
endpoint is protected with JWT authentication using the jwt_required
decorator.
To start the FastAPI server, run the following command:
uvicorn auth:app --reload
Now, when you visit http://localhost:8000/login
, you will receive an access token. To access the protected endpoint, include the access token in the Authorization header as a Bearer token:
curl -H "Authorization: Bearer " http://localhost:8000/protected
You will receive a response with the message “Welcome, user@example.com!”.
Python Libraries for Single Sign-On (SSO) with OAuth2 Providers
Implementing Single Sign-On (SSO) with OAuth2 providers in FastAPI can be made easier with the help of various Python libraries. These libraries provide abstractions and utilities to handle the complexities of OAuth2 authentication and provide a seamless integration experience. Here are some popular Python libraries for SSO with OAuth2 providers:
1. fastapi_auth
: This library provides an easy-to-use interface for implementing OAuth2 authentication in FastAPI. It supports various OAuth2 providers such as Google, Facebook, and GitHub. It handles the OAuth2 flow and provides decorators to protect FastAPI routes.
2. authlib
: Authlib is a useful authentication and authorization library for Python. It supports a wide range of OAuth2 providers and provides a high-level API for handling OAuth2 authentication. It can be used with FastAPI to implement SSO with OAuth2 providers.
3. python-social-auth
: Python Social Auth is a popular library that provides support for authentication with various social media platforms, including OAuth2 providers. It simplifies the integration process by providing pre-built authentication backends for popular providers such as Google, Facebook, and GitHub.
4. django-allauth
: Although primarily designed for Django, django-allauth can be used as a standalone library to implement OAuth2 authentication. It supports a wide range of OAuth2 providers and provides customizable templates and views for handling the authentication flow.
5. requests-oauthlib
: Requests OAuthlib is a library that provides support for OAuth1 and OAuth2 authentication in Python. It allows you to make authenticated requests to OAuth2 providers and handles the OAuth2 flow transparently.
These libraries provide different levels of abstraction and flexibility, so you can choose the one that best fits your requirements and project structure when implementing SSO with OAuth2 providers in FastAPI.
FastAPI Integration with Enterprise Directory Services
FastAPI can be seamlessly integrated with enterprise directory services such as LDAP and Active Directory to provide authentication and authorization capabilities. This integration allows organizations to leverage their existing user management infrastructure and implement secure access control mechanisms. Here’s how you can integrate FastAPI with enterprise directory services:
1. Choose an LDAP Library: FastAPI does not provide built-in support for LDAP integration, so you will need to choose an LDAP library to handle the integration. Some popular choices include python-ldap
, ldap3
, and pyldap
. These libraries provide Python interfaces to LDAP directory servers and handle the low-level communication with the LDAP server.
2. Connect to the LDAP Server: Use the chosen LDAP library to establish a connection to the LDAP server. This typically involves providing the server URL, credentials, and other necessary configuration parameters.
3. Authenticate Users: Implement a mechanism to authenticate users against the LDAP server. This typically involves binding to the LDAP server with the user’s credentials and checking the result of the authentication attempt.
4. Retrieve User Attributes: Once the user is authenticated, retrieve relevant user attributes from the LDAP server. This may include information such as username, email address, group membership, and other user-specific data.
5. Implement Authorization: Based on the retrieved user attributes, implement an authorization mechanism to control access to resources in your FastAPI app. This can be done using role-based access control (RBAC) or other access control mechanisms supported by FastAPI.
Here’s an example of how to integrate FastAPI with an LDAP server using the python-ldap
library:
First, install the python-ldap
library using pip:
pip install python-ldap
Next, create a new file called ldap.py
and add the following code:
import ldap def authenticate(username, password): ldap_server = "ldap://example.com" ldap_base_dn = "dc=example,dc=com" try: ldap_conn = ldap.initialize(ldap_server) ldap_conn.simple_bind_s(f"cn={username},{ldap_base_dn}", password) ldap_conn.unbind_s() return True except ldap.INVALID_CREDENTIALS: return False except ldap.SERVER_DOWN: return False @app.get("/") def homepage(username: str, password: str): if authenticate(username, password): return {"message": "Authenticated successfully!"} else: return {"message": "Invalid credentials!"}
In the above code, we define the authenticate
function, which authenticates the user against the LDAP server using the provided username and password. The /
endpoint takes the username
and password
as query parameters and returns a success message if the authentication is successful.
To start the FastAPI server, run the following command:
uvicorn ldap:app --reload
Now, when you visit http://localhost:8000/?username=user&password=password
, you will see the message “Authenticated successfully!” if the authentication is successful. Otherwise, you will see the message “Invalid credentials!”.
Related Article: 16 Amazing Python Libraries You Can Use Now
Benefits of Role-Based Access Control (RBAC) in FastAPI Apps
Role-Based Access Control (RBAC) is a widely-used authorization mechanism that provides fine-grained control over user permissions based on their roles. Implementing RBAC in FastAPI apps offers several benefits:
1. Granular Access Control: RBAC allows you to define and enforce access control policies at a granular level. You can assign roles to users and define permissions for each role, allowing you to control access to specific API endpoints or resources within your FastAPI app.
2. Simplified Authorization Logic: RBAC simplifies the authorization logic in your FastAPI app. Instead of checking individual permissions for each user, you can check if the user has a specific role that grants the required permissions. This reduces code complexity and makes it easier to manage and maintain the authorization logic.
3. Flexibility and Scalability: RBAC provides a flexible and scalable approach to access control. As your FastAPI app grows and evolves, you can easily add new roles and permissions to accommodate changing requirements without modifying the core authentication and authorization logic.
4. Separation of Concerns: RBAC promotes a separation of concerns by separating the definition of roles and permissions from the application logic. This allows developers to focus on implementing business logic while security administrators can manage access control policies independently.
5. Auditability and Compliance: RBAC enables better auditability and compliance with regulatory requirements. By logging and tracking user actions based on their roles, you can easily monitor and review user activity, maintain accountability, and demonstrate compliance during audits.
Here’s an example of how to implement RBAC in FastAPI using decorators:
from fastapi import Depends, FastAPI from enum import Enum app = FastAPI() class Role(str, Enum): ADMIN = "admin" USER = "user" def has_role(role: Role = Role.USER): def decorator(func): async def wrapper(*args, **kwargs): # Check if the user has the required role if get_user_role() == role: return await func(*args, **kwargs) else: return {"message": "Unauthorized"} return wrapper return decorator def get_user_role(): # Logic to retrieve the user's role from the database or other source return Role.ADMIN @app.get("/") @has_role(Role.ADMIN) async def homepage(): return {"message": "Welcome, admin!"} @app.get("/user") @has_role(Role.USER) async def userpage(): return {"message": "Welcome, user!"}
In the above code, we define the Role
enum, which represents the available roles in our FastAPI app. The has_role
decorator checks if the user has the required role and returns an unauthorized response if not. The /
endpoint is protected with the has_role(Role.ADMIN)
decorator, allowing only users with the admin role to access it. The /user
endpoint is protected with the has_role(Role.USER)
decorator, allowing only users with the user role to access it.
To start the FastAPI server, run the following command:
uvicorn rbac:app --reload
Now, when you visit http://localhost:8000/
, you will see the message “Welcome, admin!” if the user has the admin role. If you visit http://localhost:8000/user
, you will see the message “Welcome, user!” if the user has the user role. Otherwise, you will receive an unauthorized response.
FastAPI Plugins and Extensions for Implementing Audit Trails
FastAPI provides a plugin system and various extensions that can be used to implement audit trails in your FastAPI apps. These plugins and extensions simplify the process of capturing and logging user actions, making it easier to maintain accountability, monitor compliance, and investigate security incidents. Here are some popular FastAPI plugins and extensions for implementing audit trails:
1. fastapi-audit-trails
: This plugin provides an easy-to-use interface for capturing and logging user actions in FastAPI apps. It automatically logs requests and responses, including relevant information such as user ID, IP address, request method, request URL, and any parameters or payload data.
2. fastapi-logging
: This extension allows you to configure logging in your FastAPI app, including audit logging. It provides options to log user actions to different output destinations such as the console, files, or external logging services.
3. fastapi-events
: FastAPI Events is a built-in mechanism that allows you to define event handlers that are executed at specific points during the request-response cycle. You can use this mechanism to capture and log user actions as events, providing a flexible way to implement audit trails.
4. fastapi-middleware
: FastAPI Middleware allows you to define custom middleware components that are executed before and after each request. You can use middleware components to capture and log user actions, adding audit log entries to a database or external logging service.
Here’s an example of how to implement audit trails in FastAPI using the fastapi-audit-trails
plugin:
First, install the fastapi-audit-trails
plugin using pip:
pip install fastapi-audit-trails
Next, create a new file called audit.py
and add the following code:
from fastapi import FastAPI from fastapi_audit_trails import AuditTrailMiddleware app = FastAPI() app.add_middleware(AuditTrailMiddleware) @app.get("/") def homepage(): return {"message": "Welcome to the homepage!"}
In the above code, we import the necessary dependencies from fastapi
and fastapi_audit_trails
. We create a FastAPI app and add the AuditTrailMiddleware
as a middleware component. The /
endpoint returns a welcome message.
To start the FastAPI server, run the following command:
uvicorn audit:app --reload
Now, when you visit http://localhost:8000/
, the request and response will be logged automatically by the AuditTrailMiddleware
.
Legal and Regulatory Requirements for Compliance in FastAPI App Development
Developing FastAPI apps that comply with legal and regulatory requirements is essential for organizations operating in various industries. Compliance ensures that your FastAPI app meets specific standards, protects user privacy, and maintains data security. Here are some common legal and regulatory requirements to consider when developing FastAPI apps:
1. General Data Protection Regulation (GDPR): If your FastAPI app processes personal data of individuals in the European Union (EU), you need to comply with the GDPR. Ensure that you obtain proper consent for data processing, provide transparent privacy policies, and implement mechanisms to respect individuals’ rights, such as the right to access and delete their personal data.
2. Health Insurance Portability and Accountability Act (HIPAA): If your FastAPI app handles protected health information (PHI) in the United States, you need to comply with HIPAA. Implement proper security controls, including access controls, encryption, and audit trails, to protect PHI and ensure the privacy and security of healthcare data.
3. Payment Card Industry Data Security Standard (PCI DSS): If your FastAPI app processes credit card payments, you must comply with PCI DSS. This standard defines requirements for secure handling of credit card data, including encryption, access controls, and regular vulnerability assessments.
4. California Consumer Privacy Act (CCPA): If your FastAPI app collects personal information from California residents, you must comply with the CCPA. This law grants California residents specific rights regarding their personal data, such as the right to know what data is being collected and the right to request deletion of their data.
5. Sarbanes-Oxley Act (SOX): If your FastAPI app is used by publicly traded companies in the United States, you need to comply with SOX. This law imposes requirements for financial reporting and internal controls to ensure accuracy and reliability of financial statements.
6. Accessibility Standards: Ensure that your FastAPI app meets accessibility standards, such as the Web Content Accessibility Guidelines (WCAG), to ensure equal access to individuals with disabilities. This includes providing alternative text for images, keyboard navigation support, and proper color contrast.
7. Intellectual Property Rights: Respect intellectual property rights when developing FastAPI apps. Do not use copyrighted material without proper authorization, and ensure that your app does not infringe on trademarks or patents.
8. Data Breach Notification Laws: Be aware of data breach notification laws in your jurisdiction. If your FastAPI app experiences a security breach that compromises personal data, you may be required to notify affected individuals and regulatory authorities within a specified timeframe.
It is important to consult legal and compliance professionals to ensure that your FastAPI app meets all relevant legal and regulatory requirements based on your specific industry and geographical location.
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
Additional Resources
– FastAPI OAuth2 Authentication
– Implementing Audit Trails in FastAPI