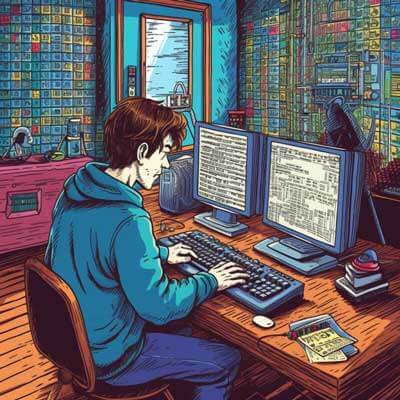
Table of Contents
Optimizing WSGI Server Configurations for Production using Gunicorn and uWSGI
When it comes to deploying Flask applications in production, optimizing the WSGI server configuration is crucial for achieving high performance and scalability. Two popular choices for WSGI servers are Gunicorn and uWSGI. In this section, we will explore the benefits and considerations of using these servers and provide examples of optimized configurations.
Related Article: How to End Python Programs
Gunicorn
Gunicorn (Green Unicorn) is a widely-used WSGI server that is known for its simplicity and ease of use. It is designed to be a pre-fork worker model server, which means it creates a pool of worker processes to handle incoming requests. This approach allows Gunicorn to handle multiple requests concurrently, making it suitable for applications with moderate traffic.
To optimize Gunicorn for production, it is recommended to configure the number of worker processes and the number of threads per worker based on the available system resources. The general rule of thumb is to have 2-4 worker processes per CPU core, with each worker having multiple threads. This allows Gunicorn to fully utilize the available CPU cores and handle concurrent requests efficiently.
Here is an example of a Gunicorn configuration file (gunicorn.conf.py
):
bind = '0.0.0.0:8000' workers = 4 threads = 2 worker_class = 'sync'
In this example, we bind Gunicorn to listen on all network interfaces (0.0.0.0
) on port 8000. We configure Gunicorn to use 4 worker processes and each worker process has 2 threads. The worker_class
is set to 'sync'
, which means Gunicorn will use synchronous workers. This configuration is suitable for applications that do not have long-running tasks or I/O-bound operations.
uWSGI
uWSGI is another popular WSGI server that provides more advanced features and flexibility compared to Gunicorn. It supports multiple worker models, including a pre-fork model similar to Gunicorn, as well as an asynchronous event-driven model. uWSGI is known for its high performance and ability to handle a large number of concurrent connections.
To optimize uWSGI for production, it is important to configure the appropriate worker model, the number of worker processes, and other parameters based on the specific requirements of the application. For example, if the application is I/O-bound or has long-running tasks, using the asynchronous worker model can provide better performance.
Here is an example of a uWSGI configuration file (uwsgi.ini
) for an application using the asynchronous worker model:
[uwsgi] http-timeout = 86400 http-timeout-keepalive = 1800 http-timeout-keepalive-requests = 100 http-timeout-keepalive = 1800 http-timeout-keepalive-requests = 100 http-timeout-keepalive = 1800 http-timeout-keepalive-requests = 100 http-timeout-keepalive = 1800 http-timeout-keepalive-requests = 100
In this example, we set the http-timeout
parameter to 86400 seconds (24 hours) to allow long-running connections. The http-timeout-keepalive
parameter is set to 1800 seconds (30 minutes) to keep idle connections alive for a certain period of time. The http-timeout-keepalive-requests
parameter is set to 100 to limit the number of requests per connection to prevent potential resource exhaustion.
Benefits of Using Docker and Kubernetes for Flask App Deployment
Docker and Kubernetes have revolutionized the way we deploy and manage applications, including Flask apps. They provide numerous benefits that make the deployment process more efficient, scalable, and reliable. In this section, we will explore the advantages of using Docker and Kubernetes for Flask app deployment and provide examples of how to leverage these technologies.
Related Article: Working with Numpy Concatenate
Docker
Docker is a containerization platform that allows you to package your application along with its dependencies into a standardized unit called a container. Containers provide isolation, portability, and consistency, making it easier to deploy and manage applications across different environments.
One of the main benefits of using Docker for Flask app deployment is the ability to create reproducible and self-contained environments. With Docker, you can define a Dockerfile that specifies the base image, dependencies, and configuration of your Flask app. This ensures that the app will run consistently across different environments, from development to production.
Here is an example of a Dockerfile for a Flask app:
FROM python:3.9-slim WORKDIR /app COPY requirements.txt . RUN pip install --no-cache-dir -r requirements.txt COPY . . CMD ["python", "app.py"]
In this example, we start with a base image (python:3.9-slim
) and set the working directory to /app
. We copy the requirements.txt
file and install the dependencies using pip
. Finally, we copy the rest of the application files and specify the command to run the Flask app (app.py
).
Another benefit of Docker is the ability to easily scale and distribute your Flask app. Docker allows you to run multiple instances of your app as containers, which can be distributed across multiple machines or orchestrated using a container orchestration platform like Kubernetes.
Kubernetes
Kubernetes is a useful container orchestration platform that automates the deployment, scaling, and management of containerized applications. It provides a highly available and scalable infrastructure for running Flask apps in production.
One of the main benefits of using Kubernetes for Flask app deployment is the ability to easily scale your app based on the incoming traffic. Kubernetes allows you to define a replica set, which specifies the desired number of instances (pods) of your app to run. The replica set can automatically scale up or down based on the defined metrics, such as CPU usage or request throughput.
Here is an example of a Kubernetes deployment configuration file (deployment.yaml
) for a Flask app:
apiVersion: apps/v1 kind: Deployment metadata: name: flask-app spec: replicas: 3 selector: matchLabels: app: flask-app template: metadata: labels: app: flask-app spec: containers: - name: flask-app image: my-flask-app:latest ports: - containerPort: 8000
In this example, we define a deployment with 3 replicas of our Flask app. The deployment ensures that the desired number of pods is running and automatically manages scaling and rolling updates. The app is exposed on port 8000, which can be accessed through a Kubernetes service.
Another benefit of Kubernetes is its built-in load balancing capabilities. Kubernetes provides a service abstraction that automatically load balances traffic to your Flask app across the available replicas. This ensures that the incoming requests are evenly distributed and improves the overall availability and performance of your app.
Handling High Traffic and Load Balancing for Flask Apps
Flask apps can face high traffic and it is important to implement strategies for handling such traffic and load balancing the requests. In this section, we will explore different techniques and tools that can be used to handle high traffic and distribute the load across multiple instances of a Flask app.
Load Balancers
A load balancer is a device or software that distributes incoming network traffic across multiple servers or instances. Load balancers play a crucial role in handling high traffic for Flask apps by ensuring that the requests are evenly distributed and no single server becomes overwhelmed.
One popular load balancer for Flask apps is Nginx. Nginx can be configured as a reverse proxy to distribute incoming requests to multiple instances of a Flask app. It uses various algorithms, such as round-robin or least connections, to determine which instance should handle each request.
Here is an example of an Nginx configuration file (nginx.conf
) for load balancing a Flask app:
http { upstream flask_backend { server backend1:8000; server backend2:8000; server backend3:8000; } server { listen 80; location / { proxy_pass http://flask_backend; } } }
In this example, we define an upstream block that specifies the backend servers (instances of the Flask app) and their respective ports. The proxy_pass
directive is used to forward the requests to the upstream servers. Nginx will distribute the incoming requests across the backend servers based on the configured algorithm.
Related Article: Python Super Keyword Tutorial
Scaling Flask Apps
Scaling a Flask app involves adding more instances or resources to handle increased traffic. There are multiple strategies for scaling Flask apps, including vertical scaling and horizontal scaling.
Vertical scaling involves increasing the resources (CPU, memory) of the existing server or instance running the Flask app. This can be done by upgrading the hardware or changing the instance type in the case of cloud-based deployments. Vertical scaling is suitable for handling moderate increases in traffic but has limitations in terms of scalability.
Horizontal scaling involves adding more instances of the Flask app to handle increased traffic. This can be achieved by running multiple instances of the app on different servers or using containerization platforms like Docker and Kubernetes. Horizontal scaling provides better scalability and fault tolerance as the load is distributed across multiple instances.
For example, in a Kubernetes cluster, you can scale the number of replicas of a Flask app by updating the deployment configuration:
kubectl scale deployment flask-app --replicas=5
This command will increase the number of replicas of the Flask app to 5, effectively scaling it horizontally. Kubernetes will automatically create and manage the additional pods to handle the increased traffic.
Caching and CDN
Caching is another effective strategy for handling high traffic and reducing the load on Flask apps. Caching involves storing the results of computationally expensive or frequently accessed operations and serving them directly from the cache instead of re-computing them for each request.
Flask provides various caching extensions, such as Flask-Cache or Flask-Caching, that integrate with popular caching backends like Redis or Memcached. These extensions allow you to cache the results of database queries, rendered templates, or any other expensive operations.
from flask import Flask from flask_caching import Cache app = Flask(__name__) cache = Cache(app, config={'CACHE_TYPE': 'simple'}) @app.route('/users') @cache.cached(timeout=60) def get_users(): # Expensive database query users = User.query.all() return render_template('users.html', users=users)
In this example, we use Flask-Caching to cache the result of the get_users
endpoint for 60 seconds. Subsequent requests to the same endpoint within the cache timeout will be served directly from the cache, reducing the load on the database.
Additionally, utilizing a Content Delivery Network (CDN) can greatly improve the performance and scalability of Flask apps. A CDN is a network of servers distributed globally that caches and serves static assets, such as images, CSS, and JavaScript files, from the server closest to the user.
Role of WSGI in Flask App Development
WSGI (Web Server Gateway Interface) is a standard interface between web servers and web applications for Python. It defines a simple and consistent way for web servers to communicate with web applications, allowing for interoperability and ease of deployment.
In the context of Flask app development, WSGI is the underlying layer that handles the communication between the Flask app and the web server. It acts as a bridge, translating incoming HTTP requests from the web server to a format that the Flask app can understand, and vice versa.
WSGI provides a set of conventions and specifications that Flask apps must adhere to in order to be compatible with WSGI servers. It defines a callable object, often referred to as the "application object", that represents the Flask app. This object is responsible for handling incoming requests and returning appropriate responses.
The WSGI server, such as Gunicorn or uWSGI, is responsible for running the Flask app as a WSGI application. It listens for incoming requests, passes them to the WSGI application, and returns the responses back to the client.
Here is an example of a simple Flask app that adheres to the WSGI interface:
from flask import Flask app = Flask(__name__) @app.route('/') def hello(): return 'Hello, World!' if __name__ == '__main__': app.run()
In this example, the Flask app is defined using the Flask
class, and the @app.route
decorator is used to define a route for the root URL ("/"). When the WSGI server receives a request for the root URL, it will invoke the hello
function and return the response to the client.
It is important to note that Flask apps can be run directly using the built-in development server, but this is not recommended for production use. Instead, a WSGI server should be used to run Flask apps in production to ensure better performance, scalability, and reliability.
Performance and Scalability Differences between Gunicorn and uWSGI
Gunicorn and uWSGI are both popular choices for running Flask apps in production, but they have differences in terms of performance and scalability. In this section, we will compare these two servers and discuss their strengths and weaknesses.
Related Article: How to Use Python Pip Install on MacOS
Gunicorn
Gunicorn is known for its simplicity and ease of use. It follows a pre-fork worker model, which means it creates a pool of worker processes to handle incoming requests. Each worker process can handle one request at a time, making it suitable for applications with moderate traffic.
The main advantage of Gunicorn is its simplicity and straightforward configuration. It does not require much configuration to get started and provides good performance out of the box. Gunicorn is also compatible with a wide range of web servers, making it easy to integrate into existing infrastructure.
However, Gunicorn may not be the best choice for high-traffic or highly concurrent applications. Since each worker process can handle only one request at a time, the performance may degrade under heavy load. Additionally, Gunicorn does not support asynchronous workers, which limits its ability to handle I/O-bound operations efficiently.
uWSGI
uWSGI is a more advanced and feature-rich WSGI server compared to Gunicorn. It supports multiple worker models, including a pre-fork model similar to Gunicorn, as well as an asynchronous event-driven model. uWSGI is known for its high performance and ability to handle a large number of concurrent connections.
The main advantage of uWSGI is its flexibility and scalability. It provides various configuration options that allow fine-tuning for specific application requirements. The asynchronous worker model in uWSGI allows it to handle I/O-bound operations efficiently, making it suitable for applications that involve a lot of network or database interactions.
However, uWSGI has a steeper learning curve compared to Gunicorn due to its advanced features and configuration options. It requires more setup and configuration to achieve optimal performance. uWSGI also has more dependencies and may require additional components like a web server or load balancer to be fully operational.
In terms of performance and scalability, uWSGI generally outperforms Gunicorn in high-traffic scenarios and applications with heavy I/O operations. Its asynchronous worker model and ability to handle a large number of concurrent connections make it a better choice for demanding applications.
Deploying a Flask App using Docker and Kubernetes
Docker and Kubernetes provide a useful combination for deploying Flask apps in a scalable and reliable manner. In this section, we will walk through the process of deploying a Flask app using Docker and Kubernetes, from containerizing the app to deploying it to a Kubernetes cluster.
Containerizing the Flask App
The first step in deploying a Flask app using Docker and Kubernetes is to containerize the app. Containerization allows us to package the app along with its dependencies into a self-contained unit that can be easily deployed and managed.
To containerize a Flask app, we need to create a Dockerfile that specifies the base image, dependencies, and configuration of the app. Here is an example of a Dockerfile for a Flask app:
FROM python:3.9-slim WORKDIR /app COPY requirements.txt . RUN pip install --no-cache-dir -r requirements.txt COPY . . CMD ["python", "app.py"]
In this example, we start with a base image (python:3.9-slim
) and set the working directory to /app
. We copy the requirements.txt
file and install the dependencies using pip
. Finally, we copy the rest of the application files and specify the command to run the Flask app (app.py
).
To build the Docker image, navigate to the directory containing the Dockerfile and run the following command:
docker build -t my-flask-app:latest .
This command will build the Docker image with the tag my-flask-app:latest
. The image can be pushed to a container registry for later deployment.
Related Article: How to Use Matplotlib for Chinese Text in Python
Creating a Kubernetes Deployment
Once the Flask app is containerized, we can deploy it to a Kubernetes cluster. The first step is to create a deployment, which defines the desired state of the app and manages the lifecycle of the app's pods.
Here is an example of a Kubernetes deployment configuration file (deployment.yaml
) for the Flask app:
apiVersion: apps/v1 kind: Deployment metadata: name: flask-app spec: replicas: 3 selector: matchLabels: app: flask-app template: metadata: labels: app: flask-app spec: containers: - name: flask-app image: my-flask-app:latest ports: - containerPort: 8000
In this example, we define a deployment with 3 replicas of our Flask app. The deployment ensures that the desired number of pods is running and automatically manages scaling and rolling updates. The app is exposed on port 8000, which can be accessed through a Kubernetes service.
To create the deployment, run the following command:
kubectl apply -f deployment.yaml
This command will create the deployment based on the configuration specified in the deployment.yaml
file.
Exposing the Flask App with a Kubernetes Service
To make the Flask app accessible from outside the Kubernetes cluster, we need to create a service. A Kubernetes service provides a stable network endpoint to access the app and can load balance traffic across the app's replicas.
Here is an example of a Kubernetes service configuration file (service.yaml
) for the Flask app:
apiVersion: v1 kind: Service metadata: name: flask-app spec: selector: app: flask-app ports: - protocol: TCP port: 80 targetPort: 8000 type: LoadBalancer
In this example, we define a service with the name flask-app
and select the pods labeled with app: flask-app
. The service exposes port 80 and forwards traffic to port 8000 of the app's pods. The type
is set to LoadBalancer
, which will allocate an external IP address for the service.
To create the service, run the following command:
kubectl apply -f service.yaml
This command will create the service based on the configuration specified in the service.yaml
file. The external IP address assigned to the service can be obtained using the following command:
kubectl get services
The Flask app should now be accessible using the external IP address assigned to the service.
Strategies for Scaling Flask Apps to Handle Increased Traffic
Flask apps can experience increased traffic due to various factors, such as popularity, marketing campaigns, or seasonal events. To handle this increased traffic, it is important to implement strategies for scaling the app. In this section, we will explore different strategies for scaling Flask apps to handle increased traffic.
Vertical Scaling
Vertical scaling involves increasing the resources (CPU, memory) of the existing server or instance running the Flask app. This can be done by upgrading the hardware or changing the instance type in the case of cloud-based deployments.
Vertical scaling is suitable for handling moderate increases in traffic but has limitations in terms of scalability. It can help improve the performance of the app by providing more resources, but there is a limit to how much the app can scale vertically.
To vertically scale a Flask app, you can increase the CPU and memory allocation of the server or instance running the app. This can be done by upgrading the hardware or changing the instance type to a more useful one.
Related Article: How To Filter Dataframe Rows Based On Column Values
Horizontal Scaling
Horizontal scaling involves adding more instances of the Flask app to handle increased traffic. This can be achieved by running multiple instances of the app on different servers or using containerization platforms like Docker and Kubernetes.
Horizontal scaling provides better scalability and fault tolerance as the load is distributed across multiple instances. Each instance can handle a portion of the incoming traffic, allowing the app to handle a higher overall load.
To horizontally scale a Flask app, you can add more instances or replicas of the app. This can be done by running multiple instances of the app on different servers or by increasing the number of replicas in a containerization platform like Docker or Kubernetes.
For example, in a Kubernetes cluster, you can scale the number of replicas of a Flask app by updating the deployment configuration:
kubectl scale deployment flask-app --replicas=5
This command will increase the number of replicas of the Flask app to 5, effectively scaling it horizontally. Kubernetes will automatically create and manage the additional pods to handle the increased traffic.
Auto Scaling
Auto scaling is a dynamic scaling strategy that automatically adjusts the number of instances or resources based on predefined metrics, such as CPU usage or request throughput. Auto scaling allows the Flask app to scale up or down based on the current demand, ensuring optimal performance and cost efficiency.
Auto scaling can be implemented using various tools and platforms, such as AWS Auto Scaling, Kubernetes Horizontal Pod Autoscaler, or custom scripts. These tools monitor the specified metrics and automatically adjust the app's capacity by adding or removing instances as necessary.
For example, with the Kubernetes Horizontal Pod Autoscaler, you can define the desired minimum and maximum number of replicas for a Flask app and set the target CPU or request utilization. The autoscaler will monitor the app's CPU or request utilization and adjust the number of replicas accordingly.
kubectl autoscale deployment flask-app --cpu-percent=70 --min=2 --max=10
This command will create an autoscaler that scales the number of replicas of the Flask app based on CPU utilization. If the CPU utilization exceeds 70%, the autoscaler will increase the number of replicas up to a maximum of 10. If the CPU utilization decreases below the threshold, the autoscaler will decrease the number of replicas down to a minimum of 2.
Auto scaling allows Flask apps to handle traffic spikes and fluctuations in demand without manual intervention, ensuring optimal performance and cost efficiency.
Implementing Load Balancing for Flask Apps
Load balancing is a crucial component of deploying Flask apps in production to ensure optimal performance, high availability, and scalability. In this section, we will explore different load balancing strategies and tools that can be used to distribute the incoming traffic across multiple instances of a Flask app.
Nginx as a Load Balancer
Nginx is a popular choice for load balancing Flask apps. It can be configured as a reverse proxy to distribute incoming requests to multiple instances of the app. Nginx uses various algorithms, such as round-robin or least connections, to determine which instance should handle each request.
To configure Nginx as a load balancer for a Flask app, you can create an Nginx configuration file that defines the upstream servers and load balancing strategy. Here is an example:
http { upstream flask_backend { server backend1:8000; server backend2:8000; server backend3:8000; } server { listen 80; location / { proxy_pass http://flask_backend; } } }
In this example, we define an upstream block that specifies the backend servers (instances of the Flask app) and their respective ports. The proxy_pass
directive is used to forward the requests to the upstream servers. Nginx will distribute the incoming requests across the backend servers based on the configured load balancing algorithm.
Nginx can be installed on a separate server or run as a container alongside the Flask app. It acts as a reverse proxy, forwarding the requests to the backend servers and returning the responses to the clients.
Related Article: Reading Binary Data Structures with Python
Kubernetes Service Load Balancing
If you are using Kubernetes to deploy your Flask app, you can leverage the built-in load balancing capabilities of Kubernetes services. A Kubernetes service provides a stable network endpoint to access the app and can load balance traffic across the available replicas.
To create a load-balanced service for a Flask app in Kubernetes, you can define a service configuration file that specifies the app's replicas and the load balancing strategy. Here is an example:
apiVersion: v1 kind: Service metadata: name: flask-app spec: selector: app: flask-app ports: - protocol: TCP port: 80 targetPort: 8000 type: LoadBalancer
In this example, we define a service with the name flask-app
and select the pods labeled with app: flask-app
. The service exposes port 80 and forwards traffic to port 8000 of the app's pods. The type
is set to LoadBalancer
, which will allocate an external IP address for the service.
Kubernetes will automatically load balance the incoming traffic across the available replicas of the Flask app, ensuring that the requests are evenly distributed and improving the overall availability and performance of the app.
Best Practices for Optimizing WSGI Server Configurations for Flask Apps
Optimizing the WSGI server configuration is crucial for achieving high performance and scalability in Flask apps. In this section, we will discuss some best practices for optimizing WSGI server configurations for Flask apps.
Tuning the Number of Worker Processes
The number of worker processes in a WSGI server determines the concurrency level and the number of requests that can be handled simultaneously. It is important to tune the number of worker processes based on the available system resources and the expected traffic load.
As a general rule of thumb, you should aim to have 2-4 worker processes per CPU core. This allows the WSGI server to fully utilize the available CPU cores and handle concurrent requests efficiently. However, the optimal number of worker processes may vary depending on the specific requirements of the app.
For example, in Gunicorn, you can configure the number of worker processes using the --workers
option:
gunicorn --workers 4 app:app
In uWSGI, you can configure the number of worker processes using the --processes
option:
uwsgi --http :8000 --wsgi-file app.py --processes 4
It is recommended to monitor the performance of the WSGI server and adjust the number of worker processes as needed to optimize the app's performance.
Configuring the Number of Threads per Worker
In addition to the number of worker processes, it is also important to configure the number of threads per worker. The number of threads determines the level of concurrency within each worker process.
The optimal number of threads per worker depends on the specific requirements of the app and the nature of the workload. If the app is CPU-bound, it is generally recommended to have fewer threads per worker to avoid excessive context switching. If the app is I/O-bound or has a lot of blocking operations, it may benefit from having more threads per worker to overlap I/O operations.
In Gunicorn, you can configure the number of threads per worker using the --threads
option:
gunicorn --workers 4 --threads 2 app:app
In uWSGI, you can configure the number of threads per worker using the --threads
option:
uwsgi --http :8000 --wsgi-file app.py --processes 4 --threads 2
It is recommended to experiment with different configurations and monitor the app's performance to find the optimal number of threads per worker.
Related Article: How to Use the IsAlpha Function in Python
Choosing the Right Worker Model
WSGI servers like Gunicorn and uWSGI support different worker models, such as pre-fork, asynchronous, or multi-threaded. The choice of the worker model depends on the specific requirements of the app and the nature of the workload.
Pre-fork worker models, like the one used by Gunicorn, create a pool of worker processes to handle incoming requests. Each worker process can handle one request at a time. This model is suitable for applications with moderate traffic and that do not have long-running tasks or I/O-bound operations.
Asynchronous worker models, like the one supported by uWSGI, are designed to handle I/O-bound operations efficiently. They use non-blocking I/O and event-driven programming to overlap I/O operations and maximize throughput. This model is suitable for applications that involve a lot of network or database interactions.
Multi-threaded worker models can handle multiple requests concurrently within each worker process. They are suitable for applications that are CPU-bound and can benefit from parallel processing.
It is important to choose the right worker model based on the specific requirements and characteristics of the app. Analyzing the workload, measuring the performance, and experimenting with different worker models can help optimize the app's performance.
Advantages of uWSGI over Gunicorn for Flask App Deployment
Both uWSGI and Gunicorn are popular choices for running Flask apps in production, but they have differences in terms of features and performance. In this section, we will discuss the advantages of uWSGI over Gunicorn for Flask app deployment.
Advanced Features
uWSGI provides more advanced features and flexibility compared to Gunicorn. It supports multiple worker models, including a pre-fork model similar to Gunicorn, as well as an asynchronous event-driven model.
The asynchronous worker model in uWSGI allows it to handle I/O-bound operations efficiently. It uses non-blocking I/O and event-driven programming to overlap I/O operations and maximize throughput. This can greatly improve the performance of Flask apps that involve a lot of network or database interactions.
In addition to the worker models, uWSGI provides various configuration options and plugins that allow fine-tuning for specific application requirements. This level of customization and flexibility can be beneficial for optimizing the performance of Flask apps in production.
Scalability and Performance
uWSGI is known for its high performance and ability to handle a large number of concurrent connections. Its asynchronous worker model and support for non-blocking I/O allow it to handle I/O-bound operations efficiently, making it suitable for demanding applications.
uWSGI is also designed to be highly scalable. It can handle a large number of worker processes and distribute the incoming requests across them. This scalability allows Flask apps to handle high traffic and scale horizontally as the demand increases.
Related Article: How to Save and Load Objects Using pickle.load in Python
Support for Multiple Protocols
uWSGI supports multiple protocols, including HTTP, uwsgi, and FastCGI. This allows Flask apps to be deployed in various environments and integrated with different web servers or load balancers.
The support for multiple protocols makes uWSGI a versatile choice for Flask app deployment. It provides flexibility and interoperability, allowing Flask apps to be integrated into existing infrastructure seamlessly.
Extensive Community and Ecosystem
uWSGI has a large and active community, with extensive documentation, tutorials, and examples available. It is widely used in production environments and has been battle-tested in various scenarios.
The extensive community and ecosystem around uWSGI provide a wealth of resources and support for Flask app deployment. This can be beneficial for developers who are new to uWSGI and need guidance or assistance in optimizing their Flask apps.
Additional Resources
- Optimizing WSGI Server Configurations for Production in Flask