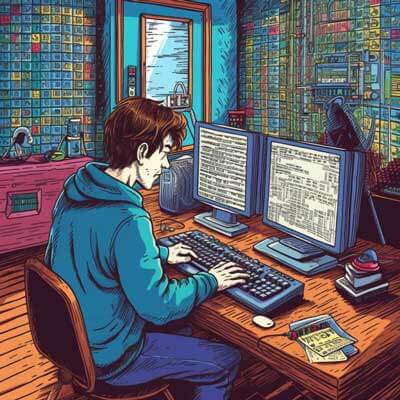
Matplotlib is a popular plotting library in Python that provides a wide range of functionality for creating visualizations. By default, Matplotlib generates static images of plots that need to be displayed using a separate window or saved to a file. However, there is a way to display the plots directly within the Jupyter Notebook or JupyterLab interface using the “inline” backend. In this guide, we will walk through the steps of using Matplotlib inline in Python.
Why is the question asked?
The question of how to use Matplotlib inline in Python is commonly asked by users who want to view their plots directly within the Jupyter Notebook or JupyterLab interface. This can be beneficial for several reasons:
1. Convenience: Displaying the plots inline eliminates the need to open a separate window or save the plots to a file, making it faster and more convenient to view and iterate on the plots.
2. Interactivity: When using the inline backend, the plots are displayed as interactive objects within the notebook, allowing users to zoom in, pan, and interact with the plots directly.
3. Seamless integration: By using Matplotlib inline, the plots become an integral part of the notebook, making it easier to share and collaborate on data analysis and visualization projects.
Related Article: How To Exit Python Virtualenv
Potential Reasons for Using Matplotlib Inline
There are several potential reasons why someone might want to use Matplotlib inline in Python:
1. Exploratory Data Analysis: When exploring a dataset and creating visualizations to gain insights, displaying the plots inline can provide a more interactive and streamlined workflow.
2. Presentations and Reports: If you are creating a presentation or a report using Jupyter Notebook, displaying the plots inline can make the document more self-contained and easier to share.
3. Teaching and Learning: In educational settings, displaying the plots inline can make it easier for students to follow along with the code and understand the visualizations.
How to Use Matplotlib Inline in Python
To use Matplotlib inline in Python, follow these steps:
1. Import the necessary libraries:
import matplotlib.pyplot as plt %matplotlib inline
2. Create your plot using Matplotlib:
x = [1, 2, 3, 4, 5] y = [10, 5, 7, 3, 8] plt.plot(x, y)
3. Display the plot:
plt.show()
4. Run the code cells in your Jupyter Notebook or JupyterLab interface.
Alternative Ideas
While using Matplotlib inline is a common and straightforward way to display plots in Jupyter Notebook or JupyterLab, there are also alternative ways to achieve similar results:
1. Jupyter Widgets: Jupyter Widgets provide a more interactive and flexible way to create visualizations in Jupyter Notebook. By using widgets, you can add interactive controls to your plots, allowing users to modify parameters and see the immediate effects.
2. Interactive Plotting Libraries: There are other plotting libraries in Python that provide interactive plotting capabilities out of the box, such as Plotly, Bokeh, and Altair. These libraries offer more advanced interactivity options and can be a good alternative to Matplotlib inline for certain use cases.
Related Article: How to Integrate Python with MySQL for Database Queries
Best Practices
When using Matplotlib inline in Python, it is good to follow these best practices:
1. Import the necessary libraries at the beginning of your notebook or script to ensure that all subsequent code cells use the inline backend.
2. Use clear and descriptive variable names when creating your plots to improve readability and maintainability.
3. Add labels, titles, and legends to your plots to provide context and make them easier to understand.
4. Use appropriate colors, line styles, and markers to distinguish different elements in your plots.
5. Consider using subplots to display multiple plots in a single figure, especially when comparing different datasets or visualizing multiple variables.
6. Include axis limits, ticks, and gridlines to provide additional information and improve the readability of your plots.
7. Use comments and markdown cells to explain the purpose and interpretation of your plots, making it easier for others to understand your code and visualizations.
Overall, using Matplotlib inline in Python can greatly enhance your data analysis and visualization workflow, especially when working with Jupyter Notebook or JupyterLab. By displaying the plots inline, you can save time and effort, and create more interactive and engaging visualizations.
For more information and examples, you can refer to the official Matplotlib documentation: https://matplotlib.org/