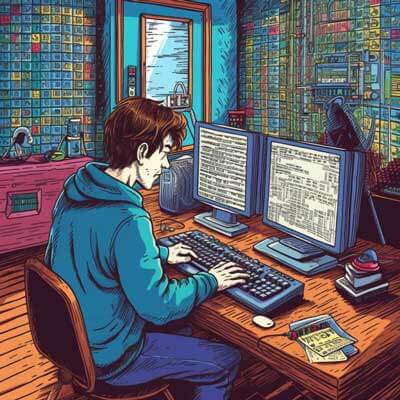
- Introduction
- Flask-SocketIO and WebSockets
- Bi-Directional Communication with Flask-SocketIO
- Real-Time Chat Rooms
- Notifications in Real-Time
- Building Live Data Dashboards
- Deployment Considerations for Real-Time Features
- Flask-SocketIO for WebSocket-Based Communication
- Examples of Real-Time Features with Flask-SocketIO
- Deploying WebSocket Apps and Considerations
- The Role of Reverse Proxies in WebSocket App Deployment
- Additional Resources
Introduction
Real-time features have become a key requirement in modern web applications. Whether it’s building live data dashboards, real-time chat rooms, or notifications systems, the ability to update information in real-time provides a dynamic and interactive user experience. In this article, we will explore how to implement real-time features using Flask-SocketIO and WebSockets. We will dive into the concepts of bi-directional communication, building chat rooms, implementing notifications, and creating live data dashboards. Additionally, we will discuss deployment considerations and the role of reverse proxies in WebSocket app deployment.
Related Article: How To Exit Python Virtualenv
Flask-SocketIO and WebSockets
Flask-SocketIO is a Flask extension that allows easy integration of WebSocket-based bi-directional communication in Flask applications. WebSockets provide a persistent connection between the client and the server, allowing real-time data transfer without the overhead of HTTP polling. This makes WebSockets an ideal choice for implementing real-time features in web applications.
Bi-Directional Communication with Flask-SocketIO
Flask-SocketIO enables bi-directional communication between the client and the server using WebSockets. This means that both the client and the server can send and receive messages in real-time. Let’s take a look at an example of how to implement bi-directional communication using Flask-SocketIO.
First, we need to install Flask-SocketIO. Open your terminal and run the following command:
pip install flask-socketio
Once Flask-SocketIO is installed, we can create a basic Flask-SocketIO application. Here’s an example of a simple chat application:
from flask import Flask, render_template from flask_socketio import SocketIO, emit app = Flask(__name__) app.config['SECRET_KEY'] = 'secret!' socketio = SocketIO(app) @app.route('/') def index(): return render_template('index.html') @socketio.on('message') def handle_message(message): emit('message', message, broadcast=True) if __name__ == '__main__': socketio.run(app)
In this example, we define a route for the homepage (‘/’), which renders an HTML template called ‘index.html’. We also define a SocketIO event handler for the ‘message’ event. When a client sends a message, the server broadcasts the message to all connected clients.
To test the application, create an ‘index.html’ file in a ‘templates’ directory with the following content:
<title>Chat Application</title> <div id="messages"></div> <button>Send</button> var socket = io(); function sendMessage() { var message = document.getElementById('message').value; socket.emit('message', message); } socket.on('message', function(message) { var messagesDiv = document.getElementById('messages'); messagesDiv.innerHTML += '<p>' + message + '</p>'; });
This HTML template sets up a basic chat interface with an input field and a button to send messages. The JavaScript code uses the Socket.IO library to establish a WebSocket connection with the server. When the ‘Send’ button is clicked, the client emits a ‘message’ event with the input value. The server broadcasts the message to all connected clients, and each client appends the message to the ‘messages’ div.
To run the application, execute the following command in your terminal:
python app.py
Open your browser and navigate to ‘http://localhost:5000’. You should see the chat interface. Open multiple tabs or windows with the same URL to simulate multiple users. Now, when you send a message in one tab, it will appear in real-time in all other tabs.
Real-Time Chat Rooms
Chat rooms are a common real-time feature in web applications. They allow users to communicate with each other in real-time. Let’s explore how to build a real-time chat room using Flask-SocketIO.
To implement a chat room, we need to modify our previous example. Instead of broadcasting messages to all connected clients, we will create separate rooms for different chat groups.
Here’s an updated version of the previous example:
from flask import Flask, render_template from flask_socketio import SocketIO, emit, join_room, leave_room app = Flask(__name__) app.config['SECRET_KEY'] = 'secret!' socketio = SocketIO(app) @app.route('/') def index(): return render_template('index.html') @socketio.on('join') def handle_join(data): room = data['room'] join_room(room) emit('message', f'User has joined the room: {room}', room=room) @socketio.on('leave') def handle_leave(data): room = data['room'] leave_room(room) emit('message', f'User has left the room: {room}', room=room) @socketio.on('message') def handle_message(data): room = data['room'] message = data['message'] emit('message', message, room=room) if __name__ == '__main__': socketio.run(app)
In this updated example, we introduce two new SocketIO event handlers: ‘join’ and ‘leave’. When a user joins a room, we use the ‘join_room’ function to add them to the specified room. Similarly, when a user leaves a room, we use the ‘leave_room’ function to remove them from the room.
To test the chat room functionality, update the ‘index.html’ file with the following changes:
<title>Chat Application</title> <div id="messages"></div> <button>Join Room</button> <button>Leave Room</button> <button>Send</button> var socket = io(); function joinRoom() { var room = document.getElementById('room').value; socket.emit('join', { room: room }); } function leaveRoom() { var room = document.getElementById('room').value; socket.emit('leave', { room: room }); } function sendMessage() { var room = document.getElementById('room').value; var message = document.getElementById('message').value; socket.emit('message', { room: room, message: message }); } socket.on('message', function(message) { var messagesDiv = document.getElementById('messages'); messagesDiv.innerHTML += '<p>' + message + '</p>'; });
This updated HTML template adds two input fields for specifying the room name. The ‘Join Room’ and ‘Leave Room’ buttons call the respective JavaScript functions, which emit the ‘join’ and ‘leave’ events with the room name. The ‘Send’ button also includes the room name when emitting the ‘message’ event.
With these updates, each user can join a specific room by entering the room name and clicking ‘Join Room’. They can then send messages to the room or leave the room using the ‘Leave Room’ button.
Related Article: How to Integrate Python with MySQL for Database Queries
Notifications in Real-Time
Real-time notifications are another common feature in web applications. They allow users to receive updates and alerts in real-time. Let’s see how we can implement real-time notifications using Flask-SocketIO.
To implement real-time notifications, we need to modify our Flask-SocketIO application to send notifications to connected clients. Here’s an example:
from flask import Flask, render_template from flask_socketio import SocketIO, emit app = Flask(__name__) app.config['SECRET_KEY'] = 'secret!' socketio = SocketIO(app) @app.route('/') def index(): return render_template('index.html') @socketio.on('connect') def handle_connect(): emit('notification', 'You are now connected!') if __name__ == '__main__': socketio.run(app)
In this example, we introduce a new SocketIO event handler: ‘connect’. This event is triggered when a client connects to the server. In the event handler, we emit a ‘notification’ event with a welcome message.
To test the notification functionality, update the ‘index.html’ file with the following changes:
<title>Notification Application</title> <div id="notification"></div> var socket = io(); socket.on('notification', function(message) { var notificationDiv = document.getElementById('notification'); notificationDiv.innerHTML += '<p>' + message + '</p>'; });
This updated HTML template includes a ‘notification’ div where the notification messages will be displayed. The JavaScript code listens for the ‘notification’ event and appends the received message to the ‘notification’ div.
With these updates, when a client connects to the server, they will receive a notification message displayed on the webpage.
Building Live Data Dashboards
Live data dashboards are an essential part of many applications, providing real-time visualizations and insights. Let’s explore how to build a live data dashboard using Flask-SocketIO.
To build a live data dashboard, we need to continuously update the data on the client side. Here’s an example of how to achieve this using Flask-SocketIO:
from flask import Flask, render_template from flask_socketio import SocketIO, emit import random import time app = Flask(__name__) app.config['SECRET_KEY'] = 'secret!' socketio = SocketIO(app) @app.route('/') def index(): return render_template('index.html') @socketio.on('connect') def handle_connect(): emit('data', get_live_data(), broadcast=True) while True: time.sleep(1) emit('data', get_live_data(), broadcast=True) def get_live_data(): return random.randint(0, 100) if __name__ == '__main__': socketio.run(app)
In this example, we introduce a new function called ‘get_live_data’ that returns a random number between 0 and 100. In the ‘connect’ event handler, we emit the initial data to all connected clients using the ‘broadcast=True’ parameter. We then enter a loop where we continuously emit the updated data to all clients every second.
To display the live data on the client side, update the ‘index.html’ file with the following changes:
<title>Live Data Dashboard</title> <div id="data"></div> var socket = io(); socket.on('data', function(data) { var dataDiv = document.getElementById('data'); dataDiv.innerHTML = '<p>' + data + '</p>'; });
This updated HTML template includes a ‘data’ div where the live data will be displayed. The JavaScript code listens for the ‘data’ event and updates the content of the ‘data’ div with the received data.
With these updates, the live data dashboard will continuously update the displayed value with a random number between 0 and 100.
Deployment Considerations for Real-Time Features
When deploying applications with real-time features, there are several considerations to take into account. Let’s explore some of the deployment considerations for real-time features using Flask-SocketIO.
Handling Multiple Workers
When deploying a Flask-SocketIO application, it’s important to consider how multiple workers will handle the WebSocket connections. By default, Flask-SocketIO uses a single-threaded server, which means that all WebSocket connections are handled by a single process. This can lead to performance limitations and scalability issues.
To handle multiple workers, you can use a production-ready server such as Gunicorn or uWSGI. These servers can spawn multiple worker processes, allowing each process to handle a portion of the WebSocket connections. This improves performance and scalability.
Here’s an example of how to deploy a Flask-SocketIO application using Gunicorn:
gunicorn -k flask_sockets.worker app:app
In this example, we use the ‘flask_sockets.worker’ worker class provided by Gunicorn, which is specifically designed for Flask-SocketIO applications.
Load Balancing and Session Affinity
When deploying a Flask-SocketIO application with multiple workers, it’s important to consider load balancing and session affinity. Load balancing ensures that incoming WebSocket connections are evenly distributed among the available workers. Session affinity, also known as sticky sessions, ensures that all requests from the same client are always routed to the same worker.
Load balancing and session affinity can be achieved using a reverse proxy such as Nginx or HAProxy. These proxies sit in front of the application servers and handle the incoming WebSocket connections, distributing them among the available workers while maintaining session affinity.
Here’s an example of an Nginx configuration for load balancing and session affinity:
http { upstream backend { server 127.0.0.1:8000; server 127.0.0.1:8001; } server { listen 80; server_name example.com; location / { proxy_pass http://backend; proxy_http_version 1.1; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection "upgrade"; } } }
In this example, we define an upstream block with the addresses of the backend servers. The ‘proxy_pass’ directive is used to forward requests to the backend servers. The ‘proxy_http_version’ and ‘proxy_set_header’ directives are used to enable WebSocket support.
Related Article: 16 Amazing Python Libraries You Can Use Now
Flask-SocketIO for WebSocket-Based Communication
Flask-SocketIO provides a seamless integration of WebSocket-based bi-directional communication in Flask applications. With its easy-to-use API and useful features, Flask-SocketIO simplifies the implementation of real-time features such as chat rooms, notifications, and live data dashboards.
To get started with Flask-SocketIO, you need to install the ‘flask-socketio’ package using pip:
pip install flask-socketio
Once installed, you can import the ‘SocketIO’ class from the ‘flask_socketio’ module and create a Flask-SocketIO application. The ‘SocketIO’ class takes a Flask application instance as its argument.
from flask import Flask from flask_socketio import SocketIO app = Flask(__name__) socketio = SocketIO(app)
With the Flask-SocketIO application created, you can define event handlers for different WebSocket events such as ‘connect’, ‘disconnect’, and custom events. These event handlers can send and receive messages in real-time.
@socketio.on('connect') def handle_connect(): print('Client connected') @socketio.on('disconnect') def handle_disconnect(): print('Client disconnected') @socketio.on('message') def handle_message(data): print('Received message:', data) socketio.emit('response', 'Message received')
In this example, we define event handlers for the ‘connect’, ‘disconnect’, and ‘message’ events. The ‘handle_connect’ and ‘handle_disconnect’ functions are triggered when a client connects or disconnects from the server, respectively. The ‘handle_message’ function is triggered when a client sends a ‘message’ event and responds with a ‘response’ event.
To run a Flask-SocketIO application, you need to call the ‘run’ method of the ‘SocketIO’ object.
if __name__ == '__main__': socketio.run(app)
With these basic building blocks, you can start building real-time features in your Flask applications using Flask-SocketIO and WebSockets.
Examples of Real-Time Features with Flask-SocketIO
Flask-SocketIO provides a useful platform for implementing real-time features in web applications. Let’s explore a couple of examples to demonstrate the capabilities of Flask-SocketIO.
Real-Time Chat Application
A real-time chat application is a classic example of a real-time feature. With Flask-SocketIO, building a real-time chat application becomes straightforward. Here’s an example of a simple chat application using Flask-SocketIO:
from flask import Flask, render_template from flask_socketio import SocketIO, emit app = Flask(__name__) socketio = SocketIO(app) @app.route('/') def index(): return render_template('index.html') @socketio.on('message') def handle_message(message): emit('message', message, broadcast=True) if __name__ == '__main__': socketio.run(app)
In this example, we define a route for the homepage (‘/’) that renders an HTML template. We also define a SocketIO event handler for the ‘message’ event. When a client sends a message, the server broadcasts the message to all connected clients.
To test the chat application, create an ‘index.html’ file in a ‘templates’ directory with the following content:
<title>Chat Application</title> <div id="messages"></div> <button>Send</button> var socket = io(); function sendMessage() { var message = document.getElementById('message').value; socket.emit('message', message); } socket.on('message', function(message) { var messagesDiv = document.getElementById('messages'); messagesDiv.innerHTML += '<p>' + message + '</p>'; });
This HTML template sets up a basic chat interface with an input field and a button to send messages. The JavaScript code uses the Socket.IO library to establish a WebSocket connection with the server. When the ‘Send’ button is clicked, the client emits a ‘message’ event with the input value. The server broadcasts the message to all connected clients, and each client appends the message to the ‘messages’ div.
With this chat application, multiple users can connect to the server and send messages in real-time. The messages will be displayed on all connected clients.
Real-Time Stock Price Updates
Real-time stock price updates are another common use case for real-time features. With Flask-SocketIO, it’s easy to implement real-time stock price updates. Here’s an example:
from flask import Flask, render_template from flask_socketio import SocketIO, emit import random import time app = Flask(__name__) socketio = SocketIO(app) @app.route('/') def index(): return render_template('index.html') @socketio.on('connect') def handle_connect(): emit('price', get_stock_price()) while True: time.sleep(1) emit('price', get_stock_price()) def get_stock_price(): return random.uniform(100, 200) if __name__ == '__main__': socketio.run(app)
In this example, we define a route for the homepage (‘/’) that renders an HTML template. We also define a SocketIO event handler for the ‘connect’ event. When a client connects to the server, we emit the initial stock price using the ‘price’ event. We then enter a loop where we continuously emit updated stock prices to the client every second.
To display the stock price updates, create an ‘index.html’ file in a ‘templates’ directory with the following content:
<title>Stock Price Updates</title> <div id="price"></div> var socket = io(); socket.on('price', function(price) { var priceDiv = document.getElementById('price'); priceDiv.innerHTML = '<p>' + price.toFixed(2) + '</p>'; });
This HTML template includes a ‘price’ div where the stock price updates will be displayed. The JavaScript code listens for the ‘price’ event and updates the content of the ‘price’ div with the received stock price.
With this stock price updates example, the client will continuously receive random stock prices between 100 and 200, displayed with two decimal places.
Deploying WebSocket Apps and Considerations
Deploying WebSocket apps requires careful consideration of various factors. Let’s explore the deployment process and important considerations when deploying WebSocket apps.
Production-Ready Server
When deploying a WebSocket app, it’s important to use a production-ready server that can handle WebSocket connections efficiently. While Flask includes a built-in development server, it is not suitable for production deployments.
Popular choices for production-ready servers include Gunicorn and uWSGI. These servers are designed to handle high loads and can efficiently manage WebSocket connections. They also provide features such as worker processes to handle multiple connections and improve scalability.
To deploy a WebSocket app using Gunicorn, you can use the following command:
gunicorn -k flask_sockets.worker app:app
In this example, we use the ‘flask_sockets.worker’ worker class provided by Gunicorn, which is specifically designed for Flask-SocketIO applications.
Load Balancing and Scaling
When deploying a WebSocket app, it’s important to consider load balancing and scaling. Load balancing ensures that incoming WebSocket connections are evenly distributed among multiple servers, improving performance and reliability.
A popular approach to load balancing WebSocket connections is to use a reverse proxy such as Nginx or HAProxy. These proxies sit in front of the WebSocket app servers and handle the incoming WebSocket connections. They can distribute the connections among multiple app servers using various algorithms such as round-robin or least connections.
Scaling WebSocket apps can be achieved by adding more app servers behind the load balancer. As the number of WebSocket connections increases, additional servers can be added to handle the load. The load balancer will automatically distribute the connections among the available servers.
Session Affinity
WebSocket connections may require session affinity, also known as sticky sessions, to ensure that all requests from the same client are always routed to the same server. This is particularly important for applications that rely on maintaining state between multiple WebSocket messages.
Session affinity can be achieved by configuring the load balancer to use a session-based algorithm for distributing WebSocket connections. This ensures that once a client establishes a WebSocket connection with a specific server, all subsequent requests from that client are routed to the same server.
Security Considerations
When deploying WebSocket apps, security considerations are crucial. WebSocket connections are vulnerable to various attacks such as cross-site scripting (XSS), cross-site request forgery (CSRF), and denial-of-service (DoS). It’s important to implement appropriate security measures to protect WebSocket connections and the underlying application.
Some security measures to consider include:
– Enforcing secure WebSocket connections using TLS/SSL certificates.
– Implementing authentication and authorization mechanisms to ensure that only authorized clients can establish WebSocket connections.
– Implementing rate limiting and throttling to prevent DoS attacks.
– Using secure coding practices to protect against XSS and CSRF attacks.
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
The Role of Reverse Proxies in WebSocket App Deployment
Reverse proxies play a crucial role in WebSocket app deployment. They act as intermediaries between clients and app servers, handling the WebSocket connections and distributing them among multiple servers. Let’s explore the role of reverse proxies in WebSocket app deployment and some important considerations.
WebSocket Protocol Support
One of the primary roles of reverse proxies is to provide WebSocket protocol support. WebSocket connections use a different protocol than regular HTTP connections, and not all web servers or load balancers natively support WebSocket connections.
Reverse proxies such as Nginx and HAProxy are capable of handling both regular HTTP requests and WebSocket connections. They can upgrade the connection to the WebSocket protocol when necessary and relay the WebSocket messages between clients and app servers.
Load Balancing WebSocket Connections
Another important role of reverse proxies is load balancing WebSocket connections. When multiple app servers are deployed to handle WebSocket connections, the reverse proxy can distribute the connections among the available servers to ensure optimal performance and scalability.
Reverse proxies use various load balancing algorithms such as round-robin, least connections, or IP hashing to distribute WebSocket connections. This ensures that the WebSocket connections are evenly distributed among the app servers, preventing any single server from becoming overloaded.
Session Affinity and Sticky Sessions
WebSocket connections may require session affinity, also known as sticky sessions, to ensure that all requests from the same client are always routed to the same server. This is particularly important for applications that rely on maintaining state between multiple WebSocket messages.
Reverse proxies can be configured to use session-based algorithms for load balancing WebSocket connections. This ensures that once a client establishes a WebSocket connection with a specific server, all subsequent requests from that client are routed to the same server. This allows the WebSocket app to maintain session state and ensures consistent behavior for the client.
SSL/TLS Termination
Reverse proxies can also handle SSL/TLS termination, offloading the SSL/TLS encryption and decryption from the app servers. This reduces the computational load on the app servers and improves performance.
Security and Access Control
Reverse proxies can also provide an additional layer of security and access control for WebSocket connections. They can enforce authentication and authorization mechanisms, ensuring that only authorized clients can establish WebSocket connections.
Reverse proxies can also implement rate limiting and throttling to protect the app servers from DoS attacks. By limiting the number of WebSocket connections per client or per IP address, they can prevent malicious clients from overwhelming the app servers.
Web Application Firewall (WAF) Integration
Some reverse proxies also integrate with web application firewalls (WAFs), providing an additional layer of protection against various attacks such as XSS and SQL injection. WAFs can inspect the WebSocket messages and block any malicious or suspicious traffic before it reaches the app servers.
Additional Resources
– Flask-SocketIO Documentation
– Flask-SocketIO GitHub Repository
– Real-time Chat with Flask-SocketIO