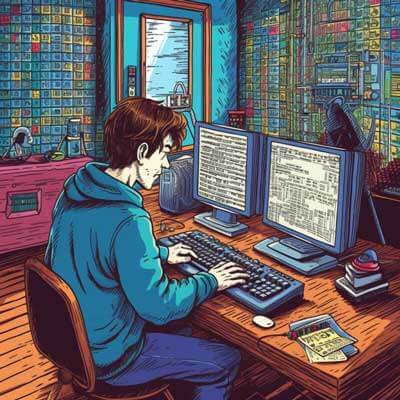
Table of Contents
Introduction to Nodemon
Nodemon is a tool that helps developers in the Node.js ecosystem by monitoring changes in their code and automatically restarting the application. This eliminates the need for manual restarts, saving valuable development time. Nodemon is particularly useful during the development process when frequent code changes are made and immediate feedback is desired.
Nodemon works by monitoring the files in a specified directory and detecting any changes. When a change is detected, Nodemon automatically restarts the application, allowing developers to see the changes in real-time. This is especially beneficial for web application development, where changes to server-side code should be reflected immediately.
Related Article: How to Use Closures with JavaScript
Installation Process of Nodemon
To install Nodemon, follow these steps:
1. Ensure that you have Node.js installed on your system. If not, you can download it from the official Node.js website: [Node.js](https://nodejs.org)
2. Open your terminal or command prompt.
3. Run the following command to install Nodemon globally:
npm install -g nodemon
4. Wait for the installation process to complete. Once finished, you can start using Nodemon in your projects.
Configuring Nodemon for your Projects
To configure Nodemon for your projects, you can create a nodemon.json
file in the root directory of your project. This file allows you to specify custom settings for Nodemon, such as the file extensions to monitor, the directories to ignore, and more.
Here is an example nodemon.json
file:
{ "ext": "js,json", "ignore": ["node_modules"], "verbose": true }
In this example, we have specified that Nodemon should monitor files with the .js
and .json
extensions. We have also instructed Nodemon to ignore the node_modules
directory and enable verbose output.
Using Nodemon to Monitor Changes
Once Nodemon is installed and configured, you can start using it to monitor changes in your code.
To use Nodemon, open your terminal or command prompt and navigate to the root directory of your project. Then, run the following command:
nodemon your_script.js
Replace your_script.js
with the name of the main script or entry point of your application. Nodemon will start monitoring the specified file and automatically restart the application whenever changes are detected.
Now, whenever you make changes to your code and save the file, Nodemon will automatically restart the application, reflecting the changes without the need for manual restarts.
Related Article: AI Implementations in Node.js with TensorFlow.js and NLP
Use Case: Nodemon in Web Application Development
Nodemon is particularly useful in web application development, where immediate feedback is crucial. Let's consider a scenario where you are developing a web application using Node.js and Express.js.
With Nodemon, you can start the development server by running the following command:
nodemon server.js
As you make changes to your code, such as modifying the route handlers or updating the middleware, Nodemon will automatically restart the server, allowing you to see the changes immediately in your browser.
This eliminates the need to manually stop and restart the server every time you make a change, resulting in a more efficient and productive development workflow.
Code Snippet: Basic Nodemon Usage
Here is a basic example of using Nodemon to monitor a Node.js script:
nodemon index.js
In this example, Nodemon will monitor the index.js
file for changes and automatically restart the script whenever a change is detected.
Code Snippet: Using Nodemon with Node.js Scripts
To use Nodemon with Node.js scripts, you can simply specify the script file as the argument when running Nodemon:
nodemon script.js
Nodemon will watch the specified file for changes and automatically restart the script whenever a change is detected.
Code Snippet: Nodemon with Express.js Server
When using Nodemon with an Express.js server, you can start the server using Nodemon instead of the regular node
command:
nodemon server.js
This will start the Express.js server and automatically restart it whenever changes are made to the server code.
Related Article: How to Reload a Page using Javascript
Code Snippet: Ignoring Files in Nodemon
Sometimes, you may want to exclude certain files or directories from being monitored by Nodemon. You can achieve this by using the --ignore
option followed by the file or directory name or pattern.
For example, to ignore the logs
directory and any files with the .log
extension, you can use the following command:
nodemon --ignore logs/*.log server.js
Nodemon will now exclude the specified files and directories from its monitoring process.
Code Snippet: Nodemon with Custom Scripts
Nodemon can also be used with custom scripts that are not necessarily related to Node.js or web development. You can use Nodemon to monitor any script or file that you want to automatically restart upon changes.
For example, if you have a Python script named script.py
, you can use Nodemon to monitor it by running the following command:
nodemon script.py
Nodemon will now watch the script.py
file and restart it whenever changes are made.
Best Practices: Nodemon Configuration
When configuring Nodemon, consider the following best practices:
1. Specify the file extensions to monitor wisely. Only include the necessary file types to avoid unnecessary restarts.
2. Exclude directories that contain files that do not need to be monitored, such as node_modules
or build output directories.
3. Use the verbose
option when debugging or troubleshooting issues. It provides detailed output that can help identify problems.
4. Regularly review and update your Nodemon configuration as your project evolves and new requirements arise.
Best Practices: Nodemon in Development Environment
To make the most out of Nodemon in a development environment, consider the following best practices:
1. Use Nodemon in conjunction with a code editor that supports automatic saving upon file changes. This allows you to see the changes in real-time without manually saving the file.
2. Leverage Nodemon's ability to ignore specific files or directories to avoid unnecessary restarts.
3. Utilize Nodemon's verbose output to gain insights into the application's behavior and potential issues.
4. Regularly save your work to avoid losing unsaved changes when Nodemon restarts the application.
Related Article: How To Convert A String To An Integer In Javascript
Real-World Example: Nodemon in Production
While Nodemon is primarily designed for development environments, it can also be used in certain production scenarios. One such scenario is when deploying applications to a staging environment where continuous integration and deployment (CI/CD) pipelines are in place.
In this scenario, Nodemon can be used to monitor the application in the staging environment, automatically restarting it whenever new changes are deployed. This ensures that the latest version of the application is always running and provides immediate feedback on the deployed changes.
However, using Nodemon in production requires careful consideration and testing to ensure that it does not negatively impact performance or stability.
Performance Consideration: Nodemon and System Resources
While Nodemon provides a convenient way to monitor and restart applications, it can consume system resources, especially when monitoring large codebases or frequently changing files.
To mitigate any potential performance issues, consider the following:
1. Avoid monitoring unnecessary files or directories by using the --ignore
option to exclude them from Nodemon's monitoring process.
2. Configure Nodemon to monitor only the file types that are essential for your application.
3. Optimize your code and avoid unnecessary file changes to minimize the frequency of restarts triggered by Nodemon.
4. Monitor system resource usage and adjust Nodemon's configuration accordingly if you notice any performance degradation.
Performance Consideration: Nodemon and Large Codebases
For large codebases, Nodemon's monitoring process may introduce some overhead due to the increased number of files being watched.
To improve performance when working with large codebases, consider the following:
1. Utilize Nodemon's --ignore
option to exclude directories or files that are not relevant to your current development tasks.
2. Split your codebase into smaller modules or services, each with its own Nodemon instance, to reduce the number of files being monitored by a single Nodemon process.
3. Optimize your code to reduce the number of file changes or use techniques like caching to minimize the need for frequent restarts.
Advanced Technique: Using Nodemon in Docker Containers
Nodemon can also be used in Docker containers to monitor code changes and automatically restart the application within the container.
To use Nodemon in a Docker container, you need to create a Dockerfile that includes Nodemon as a dependency and specifies the entry point script.
Here is an example Dockerfile:
FROM node:14 WORKDIR /app COPY package*.json ./ RUN npm install COPY . . CMD ["nodemon", "app.js"]
In this example, Nodemon is installed as part of the Docker image, and the app.js
script is specified as the entry point for Nodemon.
When building the Docker image, Nodemon will be installed, and any changes made to the code will trigger Nodemon to restart the application within the container.
Related Article: How to Scroll to the Top of a Page Using Javascript
Advanced Technique: Nodemon with Continuous Integration Tools
Nodemon can be integrated with continuous integration (CI) tools to automate the monitoring and restarting process during the CI/CD pipeline.
To use Nodemon with CI tools, you need to configure the CI environment to run Nodemon as part of the build or deployment process. This ensures that any changes made to the application code trigger Nodemon to restart the application in the CI environment.
The exact configuration steps may vary depending on the CI tool you are using, so consult the documentation or seek guidance specific to your CI tool.
Error Handling: Common Nodemon Errors and Solutions
While Nodemon generally works smoothly, you may encounter some errors during its usage. Here are some common Nodemon errors and their possible solutions:
1. Error: watch ENOSPC
: This error occurs when the system's limit for watching files is reached. To resolve this, you can increase the maximum number of files that can be watched by the system.
2. Error: ENOENT: no such file or directory
: This error indicates that the specified file does not exist. Double-check the file path and ensure that the file is present.
3. Error: spawn ENOENT
: This error occurs when the specified command cannot be found. Make sure that the command is installed and available in the system's PATH.
4. Error: listen EADDRINUSE: address already in use
: This error indicates that the specified port is already in use by another application. Choose a different port or terminate the conflicting application.
Error Handling: Debugging Nodemon
If you encounter any issues or unexpected behavior with Nodemon, you can enable debugging mode to get more detailed information about the internal workings of Nodemon.
To enable debugging, set the DEBUG
environment variable to nodemon
before running the Nodemon command:
DEBUG=nodemon nodemon script.js
This will display additional debugging information that can help identify the cause of any problems you may be experiencing.