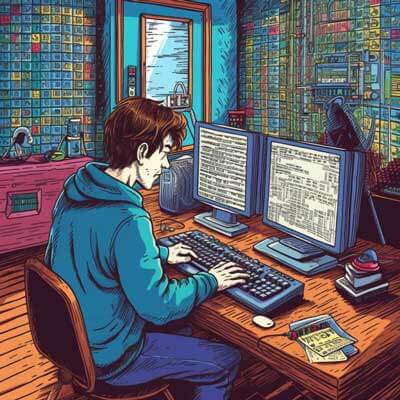
The window.onload
event in JavaScript is triggered when the entire webpage, including all its external resources such as images, stylesheets, and scripts, has finished loading. It allows you to execute a function or a block of code after the page has fully loaded. This event is commonly used to initialize or manipulate the DOM (Document Object Model) elements.
Using window.onload inline
One way to use window.onload
is by assigning a function directly to it. Here’s an example:
window.onload = function() { // Code to be executed after the page has loaded console.log("Page loaded!"); };
In this example, the anonymous function assigned to window.onload
will be called once the page has finished loading. You can replace the console.log
statement with any code you want to execute.
It’s important to note that when using this approach, if you assign a new function to window.onload
again, it will overwrite the previous one. This means that only the last assigned function will be executed.
Related Article: 25 Handy Javascript Code Snippets for Everyday Use
Using window.addEventListener
An alternative way to use window.onload
is by using the addEventListener
method. This approach allows you to assign multiple event listeners to the load
event. Here’s an example:
window.addEventListener("load", function() { // Code to be executed after the page has loaded console.log("Page loaded!"); });
In this example, the anonymous function will be executed when the load
event is triggered, which happens after the page has fully loaded.
Using addEventListener
provides more flexibility compared to the inline version. You can easily add or remove event listeners without affecting other code that might be using window.onload
.
Best Practices
When using window.onload
, keep the following best practices in mind:
1. Place your JavaScript code just before the closing tag to ensure that all DOM elements are available when the code runs. Placing the code in the
section might cause issues if the code tries to manipulate elements that haven’t been parsed yet.
2. Consider using modern JavaScript techniques such as addEventListener
instead of assigning a function directly to window.onload
. This allows for more flexibility and better separation of concerns.
3. If you need to wait for specific resources (like images) to load before executing your code, you can use the load
event on those resources instead of window.onload
. This can help improve performance by allowing your code to run as soon as possible.
4. Avoid blocking the rendering of the page by keeping your JavaScript code concise and efficient. Long-running scripts can delay the load
event and make the page appear slow to the user.
5. Test your code in different browsers to ensure cross-browser compatibility. While window.onload
is widely supported, there might be minor differences in behavior across different browsers.
Alternative Ideas
If you prefer using a JavaScript library or framework, such as jQuery or React, they often provide their own mechanisms for executing code after the page has loaded. For example, in jQuery, you can use the $(document).ready()
function, and in React, you can use the useEffect
hook with an empty dependency array.
Here’s an example using jQuery:
$(document).ready(function() { // Code to be executed after the page has loaded console.log("Page loaded!"); });
And here’s an example using React:
import React, { useEffect } from "react"; function MyComponent() { useEffect(() => { // Code to be executed after the component has mounted console.log("Component mounted!"); return () => { // Code to be executed before the component is unmounted (cleanup) console.log("Component unmounted!"); }; }, []); // JSX code for your component... return ( <div> <h1>Hello, World!</h1> </div> ); }
These alternative approaches can provide additional features and abstractions that might be useful in more complex scenarios.
Related Article: Accessing Parent State from Child Components in Next.js