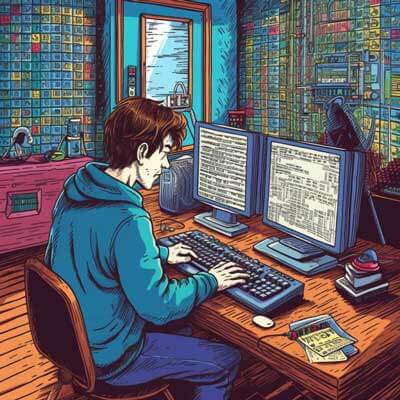
Checking if a key exists in a JavaScript object is a common task that often arises in JavaScript development. In this guide, we will explore different approaches to determine if a key exists in a JavaScript object.
1. Using the ‘in’ Operator
The simplest way to check if a key exists in a JavaScript object is by using the ‘in’ operator. The ‘in’ operator returns true if the specified key is present in the object, and false otherwise.
Here’s an example:
const obj = { name: 'John', age: 30 }; console.log('name' in obj); // Output: true console.log('city' in obj); // Output: false
In the above example, the first console.log statement returns true because the key ‘name’ is present in the object ‘obj’. On the other hand, the second console.log statement returns false because the key ‘city’ does not exist in the object.
Related Article: How To Use the Javascript Void(0) Operator
2. Using the ‘hasOwnProperty’ Method
Another way to check if a key exists in a JavaScript object is by using the ‘hasOwnProperty’ method. This method checks if the specified key exists as a direct property of the object.
Here’s an example:
const obj = { name: 'John', age: 30 }; console.log(obj.hasOwnProperty('name')); // Output: true console.log(obj.hasOwnProperty('city')); // Output: false
In the above example, the first console.log statement returns true because the key ‘name’ is a direct property of the object ‘obj’. The second console.log statement returns false because the key ‘city’ is not a direct property of the object.
It’s important to note that the ‘hasOwnProperty’ method only checks for direct properties and does not traverse the prototype chain.
3. Using the ‘Object.keys’ Method
The ‘Object.keys’ method returns an array of all the enumerable property names of an object. We can use this method to check if a key exists in a JavaScript object by checking if the array contains the desired key.
Here’s an example:
const obj = { name: 'John', age: 30 }; console.log(Object.keys(obj).includes('name')); // Output: true console.log(Object.keys(obj).includes('city')); // Output: false
In the above example, we use the ‘Object.keys’ method to get an array of all the property names of the object ‘obj. We then use the ‘includes’ method of the array to check if the desired key (‘name’ and ‘city’ in this case) is present.
It’s worth mentioning that the ‘Object.keys’ method only returns enumerable property names and does not include properties from the prototype chain.
Why is this question asked?
The question “How to check if a key exists in a JavaScript object?” is asked because it is a fundamental requirement in JavaScript development to determine if a specific key is present in an object. This knowledge is necessary for performing conditional checks, accessing object properties dynamically, and manipulating data based on the existence of specific keys.
Potential reasons for asking this question may include:
1. Validating user input: When dealing with user input, it’s essential to check if the required keys are present in the input object before further processing.
2. Data manipulation: In many cases, JavaScript objects act as data containers. To manipulate the data effectively, developers often need to check if a specific key exists before accessing or modifying its value.
3. Conditional logic: Checking for the existence of a key allows developers to execute different code paths based on whether the key is present or not. This is often used in conditional statements and dynamic property access.
Related Article: How to Check If a Value is an Object in JavaScript
Suggestions and Alternative Ideas
While the approaches mentioned above are the most common ways to check if a key exists in a JavaScript object, there are alternative techniques and suggestions to achieve the same result:
1. Optional chaining operator: If you are working with modern JavaScript environments that support ES2020 or later, you can use the optional chaining operator to check if a key exists in an object. The optional chaining operator (?.
) allows you to safely access nested properties without throwing an error if a property is undefined or null. Here’s an example:
const obj = { name: 'John', age: 30 }; console.log(obj?.name); // Output: 'John' console.log(obj?.city); // Output: undefined
In the above example, the optional chaining operator ensures that the code does not throw an error if the key is not present in the object.
2. Third-party libraries: If you are working on a large-scale project or require more advanced object manipulation capabilities, you can consider using third-party libraries such as Lodash or Underscore.js. These libraries provide additional utility functions for working with objects, including methods for checking if a key exists.
Best Practices
When checking if a key exists in a JavaScript object, it is important to consider best practices to ensure efficient and reliable code:
1. Use the appropriate method for your use case: Depending on your specific requirements, choose the method that best suits your needs. If you only need to check for direct properties, the ‘in’ operator or ‘hasOwnProperty’ method are suitable options. If you need to check for both direct and inherited properties, consider using the ‘Object.keys’ method.
2. Consider performance implications: The performance of the different approaches may vary based on the size of the object and the number of keys. If performance is a concern, consider using a method that is optimized for your specific use case. For example, if you only need to check for direct properties, using the ‘hasOwnProperty’ method may be more efficient than using the ‘Object.keys’ method.
3. Be mindful of prototype pollution: When using methods like ‘in’ or ‘hasOwnProperty’, be aware of the potential risks of prototype pollution. Prototype pollution is a security vulnerability that can occur when properties are added or modified on the object’s prototype chain. To mitigate this risk, it is recommended to sanitize and validate the object before performing any key existence checks.
4. Use consistent coding conventions: When writing code that checks for key existence, use consistent coding conventions to improve readability and maintainability. Choose a method that aligns with the codebase’s existing style and conventions to ensure consistency across the project.