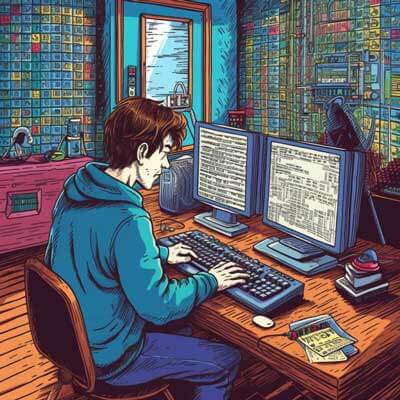
To open a URL in a new tab using JavaScript, you can use the window.open()
method. This method opens a new browser window or tab, depending on the user’s browser settings. Here are two possible ways to achieve this:
Method 1: Using the window.open() Method
You can use the window.open()
method with the URL you want to open as the first parameter and “_blank” as the second parameter. The “_blank” value specifies that the URL should be opened in a new tab. Here’s an example:
window.open("https://www.example.com", "_blank");
This code will open the URL “https://www.example.com” in a new tab.
Related Article: 25 Handy Javascript Code Snippets for Everyday Use
Method 2: Using the anchor tag with the target attribute
You can also achieve the same result by using an anchor tag with the target
attribute set to “_blank”. This will open the specified URL in a new tab when the anchor tag is clicked. Here’s an example:
<a href="https://www.example.com" target="_blank" rel="noopener">Open URL in New Tab</a>
When the user clicks on the anchor tag, the URL “https://www.example.com” will be opened in a new tab.
Best Practices
When opening a URL in a new tab using JavaScript, it is important to consider the user experience and follow best practices. Here are some tips:
1. Provide a visual indication: When you have a link that opens in a new tab, it is a good practice to indicate it to the user. You can add an icon or text near the link to let the user know that it will open in a new tab.
2. Use target=”_blank” sparingly: Opening links in new tabs should be used judiciously. It is generally best to let the user decide whether they want to open a link in a new tab or not. Only use target=”_blank” when it is necessary, such as when the link leads to external content or a resource that the user may want to reference while continuing to browse the current page.
3. Test cross-browser compatibility: The behavior of opening a URL in a new tab using JavaScript can vary across different browsers. Make sure to thoroughly test your code in different browsers to ensure a consistent experience for all users.
Alternative Ideas
While the methods mentioned above are the most common ways to open a URL in a new tab using JavaScript, there are alternative approaches you can consider:
1. Use the window.location.href
property: Instead of using window.open()
, you can set the window.location.href
property to the desired URL. This will cause the current tab to navigate to the new URL. However, note that this approach does not open the URL in a new tab; it replaces the current page with the new URL.
2. Use a JavaScript framework or library: If you are already using a JavaScript framework or library, such as React or Vue.js, there may be specific methods or components available for opening URLs in new tabs. Check the documentation of your chosen framework or library for any built-in functionality.
Overall, opening a URL in a new tab using JavaScript is a straightforward process. By using the window.open()
method or the target attribute of an anchor tag, you can provide a seamless user experience and enhance the navigation of your web application.
Related Article: Accessing Parent State from Child Components in Next.js