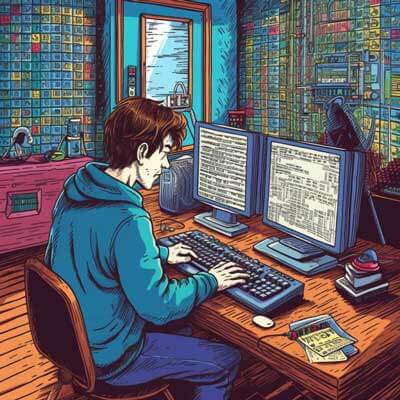
Reloading a page using JavaScript can be accomplished in a few different ways. Here are two common approaches:
Approach 1: Using the location.reload() method
One straightforward way to reload a page in JavaScript is by using the location.reload()
method. This method reloads the current document, including any changes made to it. It is important to note that this method will also re-request any resources (such as images or stylesheets) that are part of the page.
Here’s an example of how to use the location.reload()
method:
location.reload();
This code snippet can be placed inside a function or event handler to trigger the page reload. For example, you can use it in response to a button click or after a certain condition is met in your code.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Approach 2: Using the location.href property
Another way to reload a page is by assigning the current URL to the location.href
property. This effectively triggers a page refresh.
Here’s an example:
location.href = location.href;
Suggestions and Best Practices
– When using location.reload()
, it is worth noting that it has an optional parameter called forceGet
. Setting forceGet
to true
will cause the browser to bypass the cache and retrieve all resources from the server again. This can be useful if you want to ensure that all resources are reloaded, even if they are already cached by the browser. Example: location.reload(true);
– Reloading a page can disrupt the user experience, so it’s important to use it judiciously. Consider whether there are alternative approaches, such as updating specific parts of the page with new data using AJAX or manipulating the DOM directly.
– In some cases, you may need to perform additional actions before or after the page reloads. You can achieve this by wrapping the reload code in a function and using callback functions or Promise-based approaches to handle the sequence of actions.
– Always test the reliability and performance of your page reload code across different browsers and devices.
Related Article: How to Use the forEach Loop with JavaScript