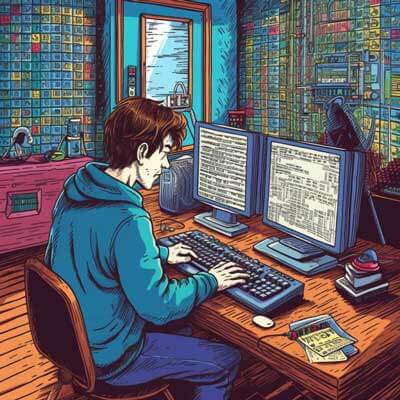
There are several ways to use loops inside React JSX. In this answer, we will explore two common approaches: using the map() function and using a for loop. These methods allow you to iterate over an array or collection of data and generate dynamic content in your React components.
Why is this question asked?
The question on how to use loops inside React JSX is often asked by developers who are new to React or who are transitioning from other frameworks. React JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript files. However, unlike traditional HTML, React JSX supports JavaScript expressions, which means you can use JavaScript loops to generate dynamic content.
Understanding how to use loops inside React JSX is crucial for building dynamic and data-driven user interfaces. By iterating over arrays or collections of data, you can dynamically render components, display lists, and generate repetitive UI elements.
Related Article: How To Fix the 'React-Scripts' Not Recognized Error
Using the map() Function
One common way to loop over an array and generate dynamic content in React JSX is by using the map() function. The map() function is a built-in JavaScript method that creates a new array by applying a provided function to each element of an existing array.
Here’s an example of how to use the map() function to render a list of items in React JSX:
import React from 'react'; function MyComponent() { const items = ['Apple', 'Banana', 'Orange']; return ( <ul> {items.map((item, index) => ( <li>{item}</li> ))} </ul> ); } export default MyComponent;
In the example above, we create an array called items
which contains three string values. Inside the JSX code, we use the map() function to iterate over each item in the items
array. For each item, we render an <li>
element with the item’s value as the content. The key
prop is used to uniquely identify each list item.
Using the map() function is a common and recommended approach when you need to generate dynamic content based on an array of data. It is especially useful when rendering lists or collections of components.
Using a for Loop
Another way to loop inside React JSX is by using a traditional for loop. While the map() function is often preferred for its simplicity and readability, there may be cases where using a for loop is more appropriate. For example, if you need to perform complex logic or conditional rendering inside the loop, a for loop provides more flexibility.
Here’s an example of how to use a for loop to render a list of items in React JSX:
import React from 'react'; function MyComponent() { const items = ['Apple', 'Banana', 'Orange']; const renderedItems = []; for (let i = 0; i < items.length; i++) { renderedItems.push(<li>{items[i]}</li>); } return <ul>{renderedItems}</ul>; } export default MyComponent;
In this example, we create an empty array called renderedItems
to store the JSX elements. Then, we use a for loop to iterate over the items
array and push a <li>
element for each item into the renderedItems
array. Finally, we render the renderedItems
array inside the <ul>
element.
Using a for loop can be useful when you need more control over the loop logic or when you want to conditionally render certain items based on specific conditions. However, it is generally recommended to use the map() function when possible, as it provides a more declarative and concise syntax.
Suggestions and Alternative Ideas
While using loops inside React JSX is a common approach for generating dynamic content, there are alternative ideas and suggestions you can consider based on your specific use case:
1. Conditional Rendering: Instead of using a loop to render all items in an array, you can conditionally render specific items based on certain conditions. React provides conditional rendering features like the if
statement, ternary operator, or logical && operator. This can be useful when you only want to display certain items that meet certain criteria.
2. Array Manipulation: Depending on the complexity of your data and rendering requirements, you may need to manipulate or transform your array data before rendering it. JavaScript provides various array methods like filter(), reduce(), or sort() that can help you modify the array before rendering.
3. External Libraries: If you find yourself working with more complex data structures or needing advanced looping capabilities, you can consider using external libraries like lodash or underscore.js. These libraries provide additional utility functions for working with arrays and collections.
Related Article: How To Upgrade Node.js To The Latest Version
Best Practices
When using loops inside React JSX, it’s essential to follow some best practices to ensure clean and efficient code:
1. Use a unique key
prop for each dynamically generated element. This helps React efficiently update and re-render the components when the array changes.
2. Avoid performing complex computations or heavy operations inside the loop. Instead, consider pre-processing or optimizing your data before rendering to improve performance.
3. Extract repeated or complex logic into separate functions or components. This helps keep your JSX code clean, readable, and maintainable.
4. Consider using memoization techniques or React’s useMemo() hook to prevent unnecessary re-rendering of components when the array data doesn’t change.
5. Follow consistent code formatting and indentation for better code readability. This makes it easier for other developers to understand and maintain your code.