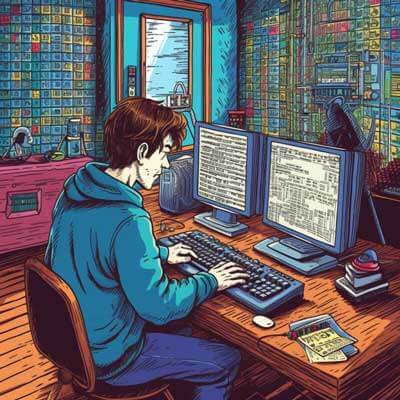
Table of Contents
To retrieve the value of a text input field in JavaScript, you can use the value
property of the input element. This property provides access to the current value entered by the user in the text field. There are multiple ways to access this value, depending on your use case. In this answer, we will explore two common approaches.
1. Using the getElementById() Method
One way to get the value of a text input field is by using the getElementById()
method to retrieve the input element, and then accessing its value
property. This method is particularly useful when you have assigned an id
attribute to the input element. Here's an example:
// HTML // JavaScript var inputElement = document.getElementById("myInput"); var value = inputElement.value; console.log(value);
In this example, we first select the input element using getElementById("myInput")
, where "myInput" is the id
assigned to the input element. We then access the value
property of the input element to retrieve the current value entered by the user. Finally, we log the value to the console.
Related Article: How To Check If a Key Exists In a Javascript Object
2. Using the querySelector() Method
Another approach to retrieve the value of a text input field is by using the querySelector()
method, which allows you to select elements using CSS-like selectors. With this method, you can select the input element by its tag name, class, or any other CSS selector. Here's an example:
// HTML // JavaScript var inputElement = document.querySelector(".myInput"); var value = inputElement.value; console.log(value);
In this example, we use the querySelector()
method to select the input element with the class "myInput". We then access the value
property of the input element to retrieve the current value entered by the user. Again, we log the value to the console.
Best Practices
Related Article: How to Enable/Disable an HTML Input Button Using JavaScript
When retrieving the value of a text input field in JavaScript, keep the following best practices in mind:
1. Always check if the input element exists before accessing its value. This helps prevent errors when the input element is not present on the page.
2. Consider using event listeners to retrieve the value of the input field dynamically. For example, you can listen for the "input" event to capture changes made by the user in real-time.
3. Validate the input value if necessary. Depending on your application's requirements, you may need to check if the input value meets certain criteria, such as length, format, or data type.
4. Use descriptive variable names to improve code readability. Instead of using generic variable names like inputElement
or value
, consider using more meaningful names that reflect the purpose of the variable.