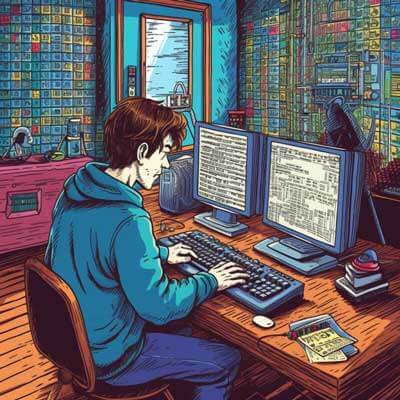
Table of Contents
Setting up a Full-Stack Application with Node.js and React.js
When developing full-stack applications, it is common to use Node.js as the backend technology and React.js as the frontend framework. This combination allows for efficient and seamless communication between the server and the client, resulting in a smooth user experience. In this section, we will explore the process of setting up a full-stack application with Node.js and React.js.
To begin, make sure you have Node.js and npm (Node Package Manager) installed on your machine. You can download and install them from the official Node.js website.
Next, create a new directory for your project and navigate to it in your terminal. Use the following command to initialize a new Node.js project:
npm init -y
This command will generate a package.json
file that will serve as the configuration file for your project.
Now, let's install the necessary dependencies. In this case, we will use Express.js as our backend framework and React.js as our frontend library. Install them using the following commands:
npm install express npm install react react-dom
Once the dependencies are installed, create a new file named server.js
in the root of your project directory. This file will serve as the entry point for your Node.js backend. Add the following code to set up a basic Express server:
const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello, World!'); }); app.listen(port, () => { console.log(`Server is running on port ${port}`); });
Save the file, and now you can start the backend server by running the following command in your terminal:
node server.js
Your server should now be running on http://localhost:3000
, and if you visit that URL in your browser, you should see the message "Hello, World!".
Now, let's set up the frontend. Create a new directory named client
in the root of your project directory. Navigate to the client
directory and run the following command to create a new React.js application:
npx create-react-app .
This command will generate a basic React.js project structure inside the client
directory.
Next, open the src/App.js
file in your favorite code editor and replace the existing code with the following:
import React from 'react'; function App() { return ( <div> <h1>Hello, World!</h1> </div> ); } export default App;
Save the file, and now you can start the frontend development server by running the following command in the client
directory:
npm start
Your frontend development server should now be running on http://localhost:3000
, and if you visit that URL in your browser, you should see the heading "Hello, World!".
Congratulations! You have successfully set up a full-stack application with Node.js and React.js. With this basic setup in place, you can now start building your application by adding more routes and components to the backend and frontend, respectively.
Related Article: JavaScript Arrays: Learn Array Slice, Array Reduce, and String to Array Conversion
Understanding Server-Side Rendering with React and Node.js
Server-side rendering (SSR) is a technique that allows you to render your React components on the server and send the generated HTML to the client. This can improve the initial page load time and provide better SEO (Search Engine Optimization) capabilities. In this section, we will explore how to implement server-side rendering with React and Node.js.
To implement server-side rendering, we will use a popular library called Next.js. Next.js is a framework built on top of React.js that provides built-in support for server-side rendering. If you haven't already, install Next.js by running the following command in your terminal:
npm install next react react-dom
Once the installation is complete, create a new directory named pages
inside the client
directory. This directory will contain the pages of your application that will be rendered on the server.
Inside the pages
directory, create a new file named index.js
. This file will serve as the entry point for the homepage of your application. Add the following code to define a simple React component:
import React from 'react'; function HomePage() { return ( <div> <h1>Welcome to my website!</h1> <p>This page is rendered on the server.</p> </div> ); } export default HomePage;
Save the file, and now you can start the server with server-side rendering enabled by running the following command in the client
directory:
npm run dev
Your server should now be running on http://localhost:3000
, and if you visit that URL in your browser, you should see the homepage of your application with the message "This page is rendered on the server.".
Optimizing API Calls and Data Flow between Node.js and React
When building full-stack applications with Node.js and React, it is important to optimize API calls and data flow between the backend and the frontend. Efficient communication between the server and the client can greatly improve the performance and user experience of your application. In this section, we will explore some techniques for optimizing API calls and data flow between Node.js and React.
One common technique for optimizing API calls is to use pagination. Instead of fetching all the data from a resource in a single API call, you can divide the data into smaller chunks and fetch them incrementally. This can reduce the response time and improve the perceived performance of your application. Here's an example of how you can implement pagination in Node.js and React:
On the server side (Node.js), you can use a library like mongoose-paginate-v2
to paginate your data. Install the library by running the following command in your terminal:
npm install mongoose-paginate-v2
Next, modify your API route to implement pagination. Here's an example using Express.js and Mongoose:
const express = require('express'); const router = express.Router(); const Product = require('../models/Product'); const { paginate } = require('mongoose-paginate-v2'); router.get('/products', async (req, res) => { const { page = 1, limit = 10 } = req.query; try { const options = { page: parseInt(page), limit: parseInt(limit), }; const result = await Product.paginate({}, options); res.json(result); } catch (error) { console.error(error); res.status(500).json({ message: 'Internal Server Error' }); } }); module.exports = router;
On the client side (React), you can use a pagination component to display and navigate through the paginated data. Here's an example using the react-paginate
library:
First, install the library by running the following command in your terminal:
npm install react-paginate
Next, create a new component named ProductList
and add the following code:
import React, { useState, useEffect } from 'react'; import axios from 'axios'; import ReactPaginate from 'react-paginate'; function ProductList() { const [products, setProducts] = useState([]); const [pageCount, setPageCount] = useState(0); useEffect(() => { const fetchData = async () => { try { const response = await axios.get('/api/products'); setProducts(response.data.docs); setPageCount(response.data.totalPages); } catch (error) { console.error(error); } }; fetchData(); }, []); const handlePageChange = async ({ selected }) => { try { const response = await axios.get(`/api/products?page=${selected + 1}`); setProducts(response.data.docs); } catch (error) { console.error(error); } }; return ( <div> <ul> {products.map((product) => ( <li>{product.name}</li> ))} </ul> </div> ); } export default ProductList;
Integrating Node.js and React.js for Full-Stack Applications
Integrating Node.js and React.js is a useful combination for building full-stack applications. With Node.js as the backend technology and React.js as the frontend framework, you can create efficient and scalable applications. In this section, we will explore how to integrate Node.js and React.js for full-stack applications.
To integrate Node.js and React.js, you need to establish a communication channel between the backend and the frontend. One common approach is to use HTTP APIs to expose the backend functionality to the frontend. Here's an example of how you can integrate Node.js and React.js using HTTP APIs:
On the server side (Node.js), you can define API routes to handle incoming requests from the frontend. These routes can be implemented using Express.js, a popular web framework for Node.js. Here's an example of an API route that fetches user data:
const express = require('express'); const router = express.Router(); const User = require('../models/User'); router.get('/users', async (req, res) => { try { const users = await User.find(); res.json(users); } catch (error) { console.error(error); res.status(500).json({ message: 'Internal Server Error' }); } }); module.exports = router;
On the client side (React.js), you can use the fetch
API or a library like axios
to make HTTP requests to the backend API. Here's an example of how you can fetch user data from the backend and display it in a React component:
import React, { useState, useEffect } from 'react'; import axios from 'axios'; function UserList() { const [users, setUsers] = useState([]); useEffect(() => { const fetchData = async () => { try { const response = await axios.get('/api/users'); setUsers(response.data); } catch (error) { console.error(error); } }; fetchData(); }, []); return ( <div> <h1>User List</h1> <ul> {users.map((user) => ( <li>{user.name}</li> ))} </ul> </div> ); } export default UserList;
Related Article: The Most Common JavaScript Errors and How to Fix Them
Frontend Frameworks for Full-Stack Applications
When building full-stack applications, choosing the right frontend framework is crucial for creating efficient and scalable solutions. While React.js is a popular choice for frontend development, there are other frameworks that can also be used in combination with Node.js for full-stack applications. In this section, we will explore some frontend frameworks that are commonly used in conjunction with Node.js for full-stack development.
1. Angular: Angular is a TypeScript-based frontend framework developed by Google. It provides a complete solution for building web applications and offers features such as two-way data binding, dependency injection, and a useful component-based architecture. Angular can be integrated with Node.js to create full-stack applications using tools like Express.js for the backend.
2. Vue.js: Vue.js is a progressive JavaScript framework that is known for its simplicity and ease of use. It allows you to build user interfaces using reusable components and offers features such as reactive data binding and a virtual DOM. Vue.js can be combined with Node.js to create full-stack applications using frameworks like Nuxt.js or Express.js.
3. Ember.js: Ember.js is a frontend framework that follows the convention-over-configuration principle. It provides a set of conventions and best practices for building web applications and offers features such as two-way data binding, automatic view updates, and a useful routing system. Ember.js can be used in combination with Node.js to create full-stack applications using frameworks like FastBoot or Express.js.
4. Backbone.js: Backbone.js is a lightweight JavaScript library that provides a simple structure for organizing code in web applications. It offers features such as models, collections, and views, and can be used with Node.js to create full-stack applications using frameworks like Express.js or Hapi.js.
While React.js is a popular choice for frontend development in full-stack applications, these frontend frameworks offer alternative approaches and can be used depending on the specific requirements of your project. It is important to consider factors such as the learning curve, community support, and ecosystem when choosing a frontend framework for your full-stack application.
Backend Considerations for Node.js and React.js
When building full-stack applications with Node.js and React.js, there are several backend considerations that you should keep in mind to ensure a robust and scalable architecture. In this section, we will explore some important considerations when developing the backend for Node.js and React.js applications.
1. API Design: Designing a well-structured and intuitive API is crucial for building a successful full-stack application. Use RESTful principles or GraphQL for designing your APIs, and ensure that your endpoints are organized logically and follow standard naming conventions. Consider using tools like Swagger or GraphQL Playground to document and test your APIs.
2. Authentication and Authorization: Implementing authentication and authorization mechanisms is essential for securing your application. Use industry-standard protocols like JSON Web Tokens (JWT) or OAuth to authenticate users and authorize access to protected resources. Consider using libraries like Passport.js or JSON Web Token (JWT) for handling authentication and authorization in your Node.js backend.
3. Database Integration: Choose the right database technology for your application based on its requirements. Node.js has excellent support for both SQL and NoSQL databases. Consider using popular databases like MongoDB or PostgreSQL, and use Object-Relational Mapping (ORM) libraries like Sequelize or Mongoose to simplify database interactions in your Node.js backend.
4. Caching and Performance Optimization: Implementing caching mechanisms can greatly improve the performance of your full-stack application. Consider using in-memory caches like Redis or caching mechanisms provided by your database technology. Additionally, optimize your backend code by following best practices, like minimizing database queries, optimizing data retrieval, and implementing server-side caching.
5. Error Handling and Logging: Proper error handling and logging are crucial for diagnosing and resolving issues in your full-stack application. Implement error handling middleware in your Node.js backend to catch and handle errors in a consistent manner. Use logging libraries like Winston or Bunyan to log relevant information about your application's behavior and errors.
6. Testing and Continuous Integration: Implement comprehensive testing strategies to ensure the stability and reliability of your full-stack application. Use testing frameworks like Jest or Mocha to write unit tests, integration tests, and end-to-end tests for both the Node.js backend and the React.js frontend. Set up a continuous integration pipeline using tools like Jenkins or Travis CI to automate the testing and deployment process.
Data Flow in Full-Stack Applications
In full-stack applications, data flows between the frontend and the backend through various layers and components. Understanding the data flow is crucial for building efficient and scalable full-stack applications. In this section, we will explore the different stages of data flow in full-stack applications built with Node.js and React.js.
1. Client-Side Data Flow: In the frontend (React.js), data flow starts with user interactions. User input, such as filling out a form or clicking a button, triggers events that update the application's state. The state is then passed down to child components through props, allowing them to render the updated data. When the state needs to be updated further, child components can communicate with their parent components by invoking callback functions passed down as props.
2. HTTP Requests: When the user performs an action that requires data from the backend, such as submitting a form or fetching additional information, the frontend (React.js) sends an HTTP request to the backend (Node.js). This request contains the necessary information, such as the endpoint and any required parameters or headers. The backend processes the request and returns a response, which includes the requested data or a status indicating the success or failure of the operation.
3. Backend Processing: In the backend (Node.js), the request is received and processed by the server. This may involve validating the request, performing business logic, and interacting with databases or external services. Once the necessary operations are complete, the backend generates a response, which is sent back to the frontend.
4. Backend Response: When the frontend (React.js) receives the response from the backend (Node.js), it updates the application state accordingly. This can involve updating the UI to display the fetched data, showing success or error messages, or triggering further actions based on the response.
5. Client-Side Rendering: After the frontend (React.js) updates the application state, it re-renders the components affected by the state change. This can involve re-rendering the entire page or specific components using React's virtual DOM diffing algorithm. The updated UI is then presented to the user, reflecting the changes made in response to their actions and the backend's response.
Server-Side Rendering Techniques with React and Node.js
Server-side rendering (SSR) is a technique that allows you to render your React components on the server and send the generated HTML to the client. This can improve the initial page load time, provide better SEO capabilities, and enhance the user experience. In this section, we will explore some techniques for implementing server-side rendering with React and Node.js.
1. Using Next.js: Next.js is a framework built on top of React.js that provides built-in support for server-side rendering. It simplifies the process of implementing SSR by handling the server-side rendering and client-side hydration automatically. To use Next.js, create a new Next.js project by running the following command in your terminal:
npx create-next-app my-app
Next.js uses file-based routing, which means you can create individual files inside the pages
directory to define your application's routes. These files can contain React components that will be rendered on the server and sent to the client. Next.js also supports dynamic routing and API routes, making it a versatile solution for server-side rendering with React and Node.js.
2. Using React Server Components (Experimental): React Server Components is an experimental feature that allows you to render React components on the server and send the generated HTML to the client. It aims to provide a more efficient and scalable approach to server-side rendering. To use React Server Components, you need to install the experimental version of React by running the following command in your terminal:
npm install react@experimental react-dom@experimental
React Server Components are similar to regular React components but have some limitations and considerations. They can be defined using the createServerComponent()
function and can be used in conjunction with other React components to compose your application. React Server Components are still in the experimental stage, so it's important to keep an eye on the official React documentation for updates and changes.
3. Using Custom SSR Implementations: If you prefer a more customized approach, you can implement server-side rendering with React and Node.js without relying on a specific framework or experimental feature. This approach requires more manual configuration and implementation but provides more flexibility. You can use libraries like ReactDOMServer
and ReactRouter
to render your React components on the server and handle routing, respectively.
To implement server-side rendering with custom implementations, you need to set up a Node.js server that handles incoming requests and renders the appropriate React components based on the requested route. This involves configuring the server to serve the generated HTML and static assets, handling routing, and managing the state of your React components on the server.
Related Article: Advanced DB Queries with Nodejs, Sequelize & Knex.js
Best Practices for API Calls in Node.js and React
When making API calls between the frontend (React) and the backend (Node.js), it is important to follow best practices to ensure reliable and efficient communication. In this section, we will explore some best practices for making API calls in Node.js and React.
1. Use Async/Await or Promises: When making API calls in Node.js and React, it is recommended to use the async/await
syntax or Promises to handle asynchronous operations. This allows you to write cleaner and more readable code by avoiding callback hell. Here is an example of making an API call using async/await
in Node.js:
async function fetchData() { try { const response = await axios.get('/api/data'); console.log(response.data); } catch (error) { console.error(error); } }
2. Handle Errors Properly: It is important to handle errors that may occur during API calls to provide a better user experience. In Node.js, use try/catch
blocks or Promises' .catch()
method to handle errors. In React, you can handle errors by using error boundaries or displaying error messages to the user. Here is an example of error handling in React:
class ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false }; } static getDerivedStateFromError(error) { return { hasError: true }; } render() { if (this.state.hasError) { return <h1>Something went wrong.</h1>; } return this.props.children; } }
3. Implement Data Validation and Sanitization: When handling user input or data received from API calls, it is important to validate and sanitize the data to prevent security vulnerabilities or unexpected behavior. Use libraries like Joi
or express-validator
in Node.js to validate incoming data, and sanitize the data before storing or using it. In React, use form validation libraries like Formik
or React Hook Form
to validate user input.
4. Use Proper HTTP Methods: Use the appropriate HTTP methods (GET
, POST
, PUT
, DELETE
, etc.) when making API calls. This ensures that the frontend and backend communicate efficiently and follow RESTful principles. For example, use POST
when creating a new resource, PUT
when updating an existing resource, and DELETE
when deleting a resource.
5. Implement Pagination or Infinite Scrolling: When fetching large amounts of data from the backend, consider implementing pagination or infinite scrolling to improve performance and user experience. This allows you to fetch and display data incrementally, reducing the load time and memory usage. Libraries like react-infinite-scroll-component
can be used in React to implement infinite scrolling.
6. Use Caching and Memoization: To improve performance and reduce unnecessary API calls, consider implementing caching mechanisms or memoization techniques. Caching can be done on the server-side using tools like Redis or on the client-side using techniques like memoization in React. This reduces the load on the backend and improves the response time.
Optimizing Data Flow between Node.js Backend and React Frontend
Optimizing the data flow between the Node.js backend and the React frontend is crucial for building efficient and scalable full-stack applications. In this section, we will explore some techniques for optimizing the data flow between the backend and the frontend.
1. Minimize API Calls: One of the most effective ways to optimize data flow is to minimize the number of API calls between the backend and the frontend. Instead of making multiple API calls for each piece of data, consider combining multiple requests into a single API call. This can be achieved by creating custom endpoints on the backend that return aggregated data or using GraphQL to fetch specific data requirements in a single request.
2. Use Caching: Implementing caching mechanisms can greatly improve the performance of your application by reducing the response time of API calls. Use tools like Redis or in-memory caches to store frequently accessed data on the backend. Additionally, consider implementing client-side caching in the frontend using techniques like memoization or local storage.
3. Implement WebSockets: WebSockets allow for real-time bidirectional communication between the backend and the frontend. By using WebSockets, you can establish a persistent connection and push data from the backend to the frontend in real-time, reducing the need for frequent API calls. Libraries like Socket.IO can be used to implement WebSockets in Node.js and React.
4. Use Server-Side Rendering: Server-side rendering (SSR) can improve the initial page load time and provide better SEO capabilities. By rendering React components on the server and sending the generated HTML to the client, you can reduce the time it takes for the user to see the content. Implement SSR using frameworks like Next.js or by building custom server-side rendering implementations.
5. Optimize Data Transfer: When transferring data between the backend and the frontend, consider optimizing the data format and size. Use compression techniques like gzip or brotli to reduce the size of the transferred data. Additionally, consider using formats like Protocol Buffers or MessagePack that provide compact binary representations of data.
6. Implement Data Validation and Transformation: Validate and transform data on the backend to ensure consistency and improve efficiency. Use libraries like Joi or JSON Schema to validate incoming data and sanitize it before storing or using it. Additionally, transform the data to match the frontend's requirements to minimize processing on the client-side.