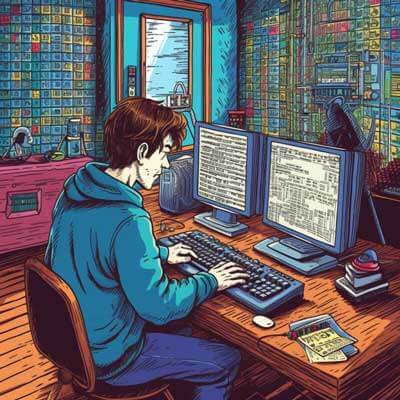
Table of Contents
Joining Tables in SQL
One of the fundamental operations in SQL is joining tables. Joining tables allows us to combine data from multiple tables based on a common column or relationship. This is especially useful when we want to retrieve data that is spread across different tables and consolidate it into a single result set.
There are different types of joins that we can use in SQL, including inner join, left join, right join, and full outer join. Let's take a look at each of these join types and see how they work.
Related Article: Exploring Natural Join in PostgreSQL Databases
Inner Join
The inner join is the most commonly used join type in SQL. It returns only the rows that have matching values in both tables being joined. Here's an example:
SELECT * FROM customers INNER JOIN orders ON customers.customer_id = orders.customer_id;
In this example, we are joining the "customers" table with the "orders" table based on the "customer_id" column. Only the rows that have the same "customer_id" value in both tables will be included in the result set.
Left Join
The left join returns all the rows from the left table and the matching rows from the right table. If there is no match, NULL values are returned for the columns of the right table. Here's an example:
SELECT * FROM customers LEFT JOIN orders ON customers.customer_id = orders.customer_id;
In this example, all the rows from the "customers" table will be included in the result set, regardless of whether there is a matching row in the "orders" table. If there is no match, the columns of the "orders" table will have NULL values.
Right Join
The right join is the opposite of the left join. It returns all the rows from the right table and the matching rows from the left table. If there is no match, NULL values are returned for the columns of the left table. Here's an example:
SELECT * FROM customers RIGHT JOIN orders ON customers.customer_id = orders.customer_id;
In this example, all the rows from the "orders" table will be included in the result set, regardless of whether there is a matching row in the "customers" table. If there is no match, the columns of the "customers" table will have NULL values.
Related Article: How to Update Records in MySQL with a Select Query
Full Outer Join
The full outer join returns all the rows from both tables, regardless of whether there is a match or not. If there is no match, NULL values are returned for the columns of the non-matching table. Here's an example:
SELECT * FROM customers FULL OUTER JOIN orders ON customers.customer_id = orders.customer_id;
In this example, all the rows from both the "customers" table and the "orders" table will be included in the result set. If there is no match, the columns of the non-matching table will have NULL values.
Understanding Where Clauses in SQL
In SQL, the WHERE clause is used to filter the rows returned by a query based on a specified condition. It allows us to define criteria that the rows must meet in order to be included in the result set.
The WHERE clause can be used with any SQL statement that retrieves data, such as SELECT, UPDATE, and DELETE. It is typically used in conjunction with the SELECT statement to filter the rows returned by a query.
The WHERE clause uses comparison operators such as "=", "", "", "=", and logical operators such as "AND", "OR", and "NOT" to define the conditions that the rows must meet.
Let's take a look at some examples to understand how the WHERE clause works.
Example 1: Filtering Rows Based on a Single Condition
Suppose we have a table "employees" with the following columns: "employee_id", "first_name", "last_name", and "salary". We want to retrieve all the employees whose salary is greater than 50000.
SELECT * FROM employees WHERE salary > 50000;
In this example, the WHERE clause specifies the condition "salary > 50000". Only the rows that meet this condition will be included in the result set.
Example 2: Filtering Rows Based on Multiple Conditions
Suppose we have a table "employees" with the following columns: "employee_id", "first_name", "last_name", "salary", and "department_id". We want to retrieve all the employees whose salary is greater than 50000 and are in the department with ID 100.
SELECT * FROM employees WHERE salary > 50000 AND department_id = 100;
In this example, the WHERE clause specifies the conditions "salary > 50000" and "department_id = 100" using the logical operator "AND". Only the rows that meet both conditions will be included in the result set.
Related Article: Using SQL Between for Date Ranges in MySQL and PostgreSQL
Merging Join and Where Clauses
In SQL, we can combine join and WHERE clauses to further refine our queries and retrieve more specific data. When merging join and WHERE clauses, the join operation is performed first, and then the WHERE clause is applied to the result set.
Let's take a look at an example to understand how to merge join and WHERE clauses.
Suppose we have two tables: "customers" and "orders". The "customers" table has columns "customer_id", "first_name", "last_name", and "city". The "orders" table has columns "order_id", "customer_id", "order_date", and "total_amount".
We want to retrieve all the orders made by customers from the city "New York". We can achieve this by merging an inner join with a WHERE clause.
SELECT * FROM customers INNER JOIN orders ON customers.customer_id = orders.customer_id WHERE customers.city = 'New York';
In this example, we first perform an inner join between the "customers" and "orders" tables based on the "customer_id" column. Then, we apply the WHERE clause to the result set to filter the rows where the customer's city is "New York". Only the orders made by customers from "New York" will be included in the final result set.
Example 1: Merging Join and WHERE Clauses with Inner Join
Suppose we have two tables: "employees" and "departments". The "employees" table has columns "employee_id", "first_name", "last_name", and "department_id". The "departments" table has columns "department_id" and "department_name".
We want to retrieve all the employees who work in the department with name "Sales". We can achieve this by merging an inner join with a WHERE clause.
SELECT * FROM employees INNER JOIN departments ON employees.department_id = departments.department_id WHERE departments.department_name = 'Sales';
In this example, we first perform an inner join between the "employees" and "departments" tables based on the "department_id" column. Then, we apply the WHERE clause to the result set to filter the rows where the department's name is "Sales". Only the employees who work in the "Sales" department will be included in the final result set.
Example 2: Merging Join and WHERE Clauses with Left Join
Suppose we have two tables: "customers" and "orders". The "customers" table has columns "customer_id", "first_name", "last_name", and "city". The "orders" table has columns "order_id", "customer_id", "order_date", and "total_amount".
We want to retrieve all the customers and their orders, including those who haven't placed any orders. We can achieve this by merging a left join with a WHERE clause.
SELECT * FROM customers LEFT JOIN orders ON customers.customer_id = orders.customer_id WHERE orders.order_id IS NULL;
In this example, we perform a left join between the "customers" and "orders" tables based on the "customer_id" column. Then, we apply the WHERE clause to the result set to filter the rows where the order's ID is NULL. This will include the customers who haven't placed any orders. Only the customers and their orders, including those without any orders, will be included in the final result set.
Advanced SQL Join Techniques
In addition to the basic join types mentioned earlier (inner join, left join, right join, and full outer join), there are some advanced join techniques that can be used in SQL to solve more complex problems. These techniques include self-joins, subquery joins, and cross joins.
Related Article: Tutorial: Role of PostgreSQL Rollup in Databases
Self-Joins
A self-join is a join operation where a table is joined with itself. It can be used to retrieve relationships between rows within a single table. Self-joins are useful when we have hierarchical data or when we need to compare rows within the same table.
Let's take a look at an example to understand how self-joins work.
Suppose we have a table "employees" with the following columns: "employee_id", "first_name", "last_name", and "manager_id". The "manager_id" column represents the ID of the employee's manager.
We want to retrieve the names of all employees along with the names of their managers. We can achieve this by performing a self-join on the "employees" table.
SELECT e.first_name AS employee_name, m.first_name AS manager_name FROM employees e INNER JOIN employees m ON e.manager_id = m.employee_id;
In this example, we perform a self-join on the "employees" table. The join condition is based on the "manager_id" column of the employee being joined and the "employee_id" column of the manager. This allows us to retrieve the names of all employees along with the names of their managers.
Subquery Joins
A subquery join is a join operation where the source of one of the joined tables is a subquery. It can be used to combine the results of a subquery with the rows of another table. Subquery joins are useful when we need to filter or aggregate data before joining it with another table.
Let's take a look at an example to understand how subquery joins work.
Suppose we have two tables: "orders" and "order_items". The "orders" table has columns "order_id", "customer_id", and "order_date". The "order_items" table has columns "order_id", "product_id", and "quantity".
We want to retrieve the total quantity of each product ordered by each customer. We can achieve this by performing a subquery join.
SELECT o.customer_id, oi.product_id, SUM(oi.quantity) AS total_quantity FROM orders o INNER JOIN ( SELECT order_id, product_id, quantity FROM order_items ) oi ON o.order_id = oi.order_id GROUP BY o.customer_id, oi.product_id;
In this example, we perform a subquery join by using a subquery as the source of the "order_items" table. The subquery selects the "order_id", "product_id", and "quantity" columns from the "order_items" table. Then, we join the result of the subquery with the "orders" table based on the "order_id" column. Finally, we use the GROUP BY clause to group the result by the "customer_id" and "product_id" columns, and calculate the total quantity of each product ordered by each customer.
Cross Joins
A cross join is a join operation that returns the Cartesian product of the joined tables. It can be used to combine every row from one table with every row from another table. Cross joins are useful when we need to generate all possible combinations of rows between two tables.
Let's take a look at an example to understand how cross joins work.
Suppose we have two tables: "colors" and "sizes". The "colors" table has columns "color_id" and "color_name". The "sizes" table has columns "size_id" and "size_name".
We want to retrieve all possible combinations of colors and sizes. We can achieve this by performing a cross join.
SELECT c.color_name, s.size_name FROM colors c CROSS JOIN sizes s;
In this example, we perform a cross join between the "colors" and "sizes" tables. The result will include all possible combinations of colors and sizes.
Using Join and Where Clauses in SQL Server
SQL Server provides comprehensive support for join and WHERE clauses, allowing us to perform complex queries and retrieve specific data. Let's explore how to use join and WHERE clauses in SQL Server.
Related Article: Exploring SQL Join Conditions: The Role of Primary Keys
Example 1: Inner Join
Suppose we have two tables: "orders" and "customers". The "orders" table has columns "order_id", "customer_id", and "order_date". The "customers" table has columns "customer_id", "first_name", and "last_name".
We want to retrieve all the orders along with the names of the customers who placed the orders. We can achieve this by performing an inner join between the "orders" and "customers" tables.
SELECT o.order_id, c.first_name, c.last_name FROM orders o INNER JOIN customers c ON o.customer_id = c.customer_id;
In this example, we perform an inner join on the "orders" and "customers" tables based on the "customer_id" column. This allows us to retrieve the orders along with the names of the customers who placed the orders.
Example 2: Where Clause
Suppose we have a table "products" with the following columns: "product_id", "product_name", and "price".
We want to retrieve all the products with a price greater than 100. We can achieve this by using the WHERE clause.
SELECT * FROM products WHERE price > 100;
In this example, the WHERE clause specifies the condition "price > 100". Only the products that meet this condition will be included in the result set.
Utilizing Join and Where Clauses in MySQL
MySQL is a popular open-source relational database management system that provides robust support for join and WHERE clauses. Let's explore how to utilize join and WHERE clauses in MySQL.
Example 1: Left Join
Suppose we have two tables: "customers" and "orders". The "customers" table has columns "customer_id", "first_name", and "last_name". The "orders" table has columns "order_id", "customer_id", and "order_date".
We want to retrieve all the customers along with their orders, including those who haven't placed any orders. We can achieve this by performing a left join between the "customers" and "orders" tables.
SELECT c.customer_id, c.first_name, c.last_name, o.order_id, o.order_date FROM customers c LEFT JOIN orders o ON c.customer_id = o.customer_id;
In this example, we perform a left join on the "customers" and "orders" tables based on the "customer_id" column. This allows us to retrieve all the customers along with their orders, including those who haven't placed any orders.
Related Article: Tutorial: the Functionality of Inner Join in SQL
Example 2: Where Clause with Multiple Conditions
Suppose we have a table "employees" with the following columns: "employee_id", "first_name", "last_name", "salary", and "department_id".
We want to retrieve all the employees whose salary is greater than 50000 and are in the department with ID 100. We can achieve this by using the WHERE clause with multiple conditions.
SELECT * FROM employees WHERE salary > 50000 AND department_id = 100;
In this example, the WHERE clause specifies the conditions "salary > 50000" and "department_id = 100" using the logical operator "AND". Only the employees who meet both conditions will be included in the result set.
Exploring Join and Where Clauses in Oracle
Oracle is a useful and widely used relational database management system that provides extensive support for join and WHERE clauses. Let's explore how to use join and WHERE clauses in Oracle.
Example 1: Right Join
Suppose we have two tables: "departments" and "employees". The "departments" table has columns "department_id" and "department_name". The "employees" table has columns "employee_id", "first_name", "last_name", and "department_id".
We want to retrieve all the departments along with the names of the employees who work in each department, including departments with no employees. We can achieve this by performing a right join between the "departments" and "employees" tables.
SELECT d.department_id, d.department_name, e.first_name, e.last_name FROM departments d RIGHT JOIN employees e ON d.department_id = e.department_id;
In this example, we perform a right join on the "departments" and "employees" tables based on the "department_id" column. This allows us to retrieve all the departments along with the names of the employees who work in each department, including departments with no employees.
Example 2: Where Clause with NULL Values
Suppose we have a table "orders" with the following columns: "order_id", "customer_id", and "order_date".
We want to retrieve all the orders that have a NULL value for the "customer_id" column. We can achieve this by using the WHERE clause.
SELECT * FROM orders WHERE customer_id IS NULL;
In this example, the WHERE clause specifies the condition "customer_id IS NULL". Only the orders that meet this condition will be included in the result set.
Related Article: How to Restore a Postgresql Backup File Using the Command Line
Best Practices for Join and Where Clauses in SQL
When using join and WHERE clauses in SQL, it's important to follow best practices to ensure efficient and optimized queries. Here are some best practices to consider:
1. Use the appropriate join type: Choose the join type that best suits your data and query requirements. Inner join is the most commonly used join type, but left join, right join, and full outer join can also be useful in certain scenarios.
2. Use indexes: Indexes can significantly improve the performance of join and WHERE clauses. Make sure to create indexes on the columns used in the join and WHERE conditions to speed up the query execution.
3. Use aliases: When joining multiple tables, it's a good practice to use aliases for table names to make the query more readable. This also helps to avoid naming conflicts between columns with the same name in different tables.
4. Be cautious with NULL values: When using WHERE clauses, be aware of the behavior of NULL values. NULL values may not always behave as expected in comparison operations. Use the IS NULL or IS NOT NULL operators to handle NULL values explicitly.
5. Optimize query execution: Analyze the query execution plan to identify any performance bottlenecks and optimize the query accordingly. Consider using query hints or rewriting the query if necessary.
6. Test and measure performance: Test your queries with different data sets and measure their performance to ensure they meet your requirements. Monitor the query execution time and make adjustments as needed.
Following these best practices can help you write efficient and optimized queries using join and WHERE clauses in SQL.
Additional Resources
- What is SQL? - W3Schools