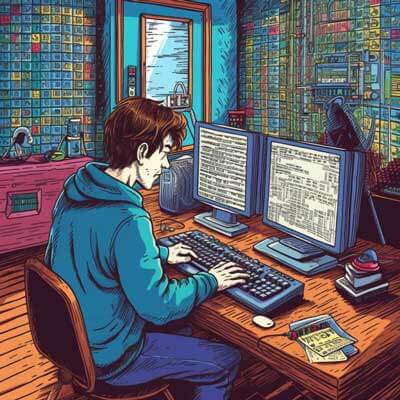
- SQL Joins: An Overview
- Types of SQL Joins
- Inner Join: Combining Data from Multiple Tables
- Outer Join: Including Unmatched Rows
- Left Join: Retrieving All Rows from the Left Table
- Right Join: Retrieving All Rows from the Right Table
- Code Snippet: Performing an Inner Join in SQL
- Code Snippet: Performing an Outer Join in SQL
- Code Snippet: Performing a Left Join in SQL
- Code Snippet: Performing a Right Join in SQL
- Additional Resources
SQL Joins: An Overview
SQL Joins are a fundamental concept in relational databases that allow you to combine data from multiple tables based on a common column. Joins enable you to retrieve data that is spread across multiple tables and create a cohesive result set.
When working with large databases, it is often necessary to combine data from multiple tables to perform complex queries and obtain meaningful insights. SQL Joins provide a way to achieve this by linking tables together based on a specified condition. By joining tables, you can access related data and perform operations on them.
There are several types of SQL Joins, each serving a specific purpose depending on the data you want to retrieve. In this article, we will explore the left-to-right operation of SQL Joins and understand how each join type works.
Related Article: Tutorial: ON for JOIN SQL in Databases
Types of SQL Joins
There are four main types of SQL Joins:
1. Inner Join: Retrieves data that exists in both tables based on a specified condition.
2. Outer Join: Retrieves data that exists in one table and may or may not have a match in the other table.
3. Left Join: Retrieves all rows from the left table and the matching rows from the right table.
4. Right Join: Retrieves all rows from the right table and the matching rows from the left table.
Each join type has its own purpose and use cases, and understanding when to use each type is crucial for effective database querying.
Inner Join: Combining Data from Multiple Tables
The Inner Join is the most commonly used join type in SQL. It retrieves only the rows that have matching values in both tables being joined. This means that only the data that exists in both tables will be included in the result set.
Let’s consider an example where we have two tables, “Customers” and “Orders”. The “Customers” table contains information about customers, while the “Orders” table contains information about orders placed by customers. We can use an Inner Join to combine these two tables and retrieve information about customers who have placed orders.
Here’s an example of an Inner Join query in SQL:
SELECT Customers.CustomerName, Orders.OrderID FROM Customers INNER JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
In this query, we specify the columns we want to retrieve from both tables, “Customers.CustomerName” and “Orders.OrderID”. We then use the INNER JOIN keyword to join the “Customers” and “Orders” tables based on the common column “CustomerID”. The result will be a combination of the customer names and their corresponding order IDs.
Outer Join: Including Unmatched Rows
The Outer Join is used to retrieve data from one table even if there is no match in the other table. It includes unmatched rows from one table and matching rows from the other table.
There are three types of Outer Joins:
1. Left Outer Join (or Left Join): Retrieves all rows from the left table and the matching rows from the right table.
2. Right Outer Join (or Right Join): Retrieves all rows from the right table and the matching rows from the left table.
3. Full Outer Join: Retrieves all rows from both tables, including unmatched rows.
In this article, we will focus on Left Join and Right Join. Let’s explore each of them in detail.
Related Article: Analyzing SQL Join and Its Effect on Records
Left Join: Retrieving All Rows from the Left Table
The Left Join, also known as Left Outer Join, retrieves all rows from the left table and the matching rows from the right table. If there is no match in the right table, NULL values are returned for the columns of the right table.
Let’s consider an example where we have two tables, “Customers” and “Orders”. The “Customers” table contains information about customers, while the “Orders” table contains information about orders placed by customers. We can use a Left Join to retrieve all customers, including those who have not placed any orders.
Here’s an example of a Left Join query in SQL:
SELECT Customers.CustomerName, Orders.OrderID FROM Customers LEFT JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
In this query, we use the LEFT JOIN keyword to perform a Left Join between the “Customers” and “Orders” tables based on the common column “CustomerID”. The result will include all customers from the “Customers” table, along with their corresponding order IDs if they have placed any orders. If a customer has not placed any orders, the order ID column will contain NULL.
Right Join: Retrieving All Rows from the Right Table
The Right Join, also known as Right Outer Join, is the opposite of the Left Join. It retrieves all rows from the right table and the matching rows from the left table. If there is no match in the left table, NULL values are returned for the columns of the left table.
Let’s consider the same example of the “Customers” and “Orders” tables. This time, we can use a Right Join to retrieve all orders, including those that do not have a corresponding customer.
Here’s an example of a Right Join query in SQL:
SELECT Customers.CustomerName, Orders.OrderID FROM Customers RIGHT JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
In this query, we use the RIGHT JOIN keyword to perform a Right Join between the “Customers” and “Orders” tables based on the common column “CustomerID”. The result will include all orders from the “Orders” table, along with the corresponding customer names if they exist. If an order does not have a corresponding customer, the customer name column will contain NULL.
Code Snippet: Performing an Inner Join in SQL
SELECT Customers.CustomerName, Orders.OrderID FROM Customers INNER JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
Related Article: Tutorial: Full Outer Join versus Join in SQL
Code Snippet: Performing an Outer Join in SQL
SELECT Customers.CustomerName, Orders.OrderID FROM Customers <a href="https://www.squash.io/how-to-perform-a-full-outer-join-in-mysql/">FULL OUTER JOIN</a> Orders ON Customers.CustomerID = Orders.CustomerID;
Code Snippet: Performing a Left Join in SQL
SELECT Customers.CustomerName, Orders.OrderID FROM Customers LEFT JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
Code Snippet: Performing a Right Join in SQL
SELECT Customers.CustomerName, Orders.OrderID FROM Customers RIGHT JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
Related Article: Exploring SQL Join Conditions: The Role of Primary Keys