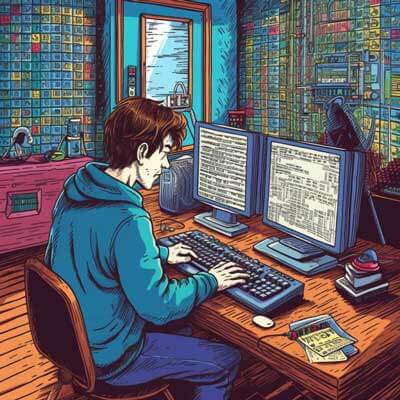
Full Outer Join
The Full Outer Join is a type of join in SQL that combines the results of both the Left Join and the Right Join. It returns all the rows from both the left and right tables, and fills in any missing values with NULL. This type of join is useful when you want to include all the data from both tables in the result set, regardless of whether there is a match between the columns being joined.
Here is an example of a Full Outer Join using the “employees” and “departments” tables:
SELECT employees.employee_name, departments.department_name FROM employees FULL <a href="https://www.squash.io/is-ansi-sql-standard-compatible-with-outer-joins/">OUTER JOIN</a> departments ON employees.department_id = departments.department_id;
This query will return all the employee names and department names from both the “employees” and “departments” tables, regardless of whether there is a match between the department IDs.
Related Article: Tutorial: ON for JOIN SQL in Databases
Join in SQL
The Join operation in SQL is used to combine rows from two or more tables based on a related column between them. It allows you to retrieve data from multiple tables in a single query, making it a useful tool for data analysis and reporting.
There are several types of joins in SQL, each with its own specific use case. In addition to the Full Outer Join, the other types of joins include Inner Join, Left Join, Right Join, Cross Join, Self Join, Natural Join, Equijoin, Non-Equijoin, and Cartesian Join.
Inner Join
The Inner Join is the most common type of join in SQL. It returns only the rows from both tables that have matching values in the specified column(s). It is used to combine data from two or more tables based on a common column or columns.
Here is an example of an Inner Join using the “employees” and “departments” tables:
SELECT employees.employee_name, departments.department_name FROM employees INNER JOIN departments ON employees.department_id = departments.department_id;
This query will return only the employee names and department names where there is a matching department ID in both the “employees” and “departments” tables.
Left Join
The Left Join returns all the rows from the left table (the table before the LEFT JOIN keyword) and the matching rows from the right table (the table after the LEFT JOIN keyword). If there is no match, NULL values are filled in for the columns of the right table.
Here is an example of a Left Join using the “employees” and “departments” tables:
SELECT employees.employee_name, departments.department_name FROM employees LEFT JOIN departments ON employees.department_id = departments.department_id;
This query will return all the employee names and department names from the “employees” table, and the matching department names from the “departments” table. If there is no match, NULL values will be filled in for the department names.
Related Article: Analyzing SQL Join and Its Effect on Records
Right Join
The Right Join is similar to the Left Join, but it returns all the rows from the right table (the table after the RIGHT JOIN keyword) and the matching rows from the left table (the table before the RIGHT JOIN keyword). If there is no match, NULL values are filled in for the columns of the left table.
Here is an example of a Right Join using the “employees” and “departments” tables:
SELECT employees.employee_name, departments.department_name FROM employees RIGHT JOIN departments ON employees.department_id = departments.department_id;
This query will return all the employee names and department names from the “departments” table, and the matching employee names from the “employees” table. If there is no match, NULL values will be filled in for the employee names.
Cross Join
The Cross Join, also known as the Cartesian Join, returns the Cartesian product of the two tables. It combines each row from the first table with each row from the second table, resulting in a new table with all possible combinations.
Here is an example of a Cross Join using the “employees” and “departments” tables:
SELECT employees.employee_name, departments.department_name FROM employees CROSS JOIN departments;
This query will return all possible combinations of employee names and department names from the “employees” and “departments” tables.
Self Join
A Self Join is a join operation where a table is joined with itself. It is useful when you want to combine rows from the same table based on a related column. To perform a Self Join, you need to use table aliases to distinguish between the two instances of the same table.
Here is an example of a Self Join using the “employees” table:
SELECT e1.employee_name, e2.employee_name FROM employees e1 JOIN employees e2 ON e1.manager_id = e2.employee_id;
This query will return the names of employees and their corresponding managers, where the manager ID matches the employee ID.
Related Article: Exploring SQL Join Conditions: The Role of Primary Keys
Natural Join
A Natural Join is a join operation that combines rows from two tables based on columns with the same name and compatible data types. It automatically matches the columns with the same name in both tables and returns the resulting rows.
Here is an example of a Natural Join using the “employees” and “departments” tables:
SELECT employee_name, department_name FROM employees NATURAL JOIN departments;
This query will return the employee names and department names where the department ID matches in both the “employees” and “departments” tables.
Equijoin
An Equijoin is a type of join operation where the join condition is based on equality between two columns. It is the most common type of join and is used to combine rows from two or more tables based on matching values in the specified columns.
Here is an example of an Equijoin using the “employees” and “departments” tables:
SELECT employee_name, department_name FROM employees JOIN departments ON employees.department_id = departments.department_id;
This query will return the employee names and department names where the department ID matches in both the “employees” and “departments” tables.
Non-Equijoin
A Non-Equijoin is a type of join operation where the join condition is based on a comparison operator other than equality. It is used to combine rows from two or more tables based on a specified condition, such as greater than, less than, or not equal to.
Here is an example of a Non-Equijoin using the “employees” and “salaries” tables:
SELECT employee_name, salary FROM employees JOIN salaries ON employees.employee_id = salaries.employee_id AND salaries.salary > 5000;
This query will return the employee names and salaries where the employee ID matches in both the “employees” and “salaries” tables, and the salary is greater than 5000.
Related Article: Merging Two Result Values in SQL
Cartesian Join
A Cartesian Join, also known as a Cross Join, is a join operation that combines each row from the first table with each row from the second table, resulting in a new table with all possible combinations. It does not require a join condition and can result in a large number of rows in the output.
Here is an example of a Cartesian Join using the “employees” and “departments” tables:
SELECT employee_name, department_name FROM employees, departments;
This query will return all possible combinations of employee names and department names from the “employees” and “departments” tables.
Join Condition
A Join Condition is the criteria used to match rows from two or more tables in a join operation. It specifies the columns that are compared to determine whether a row from one table matches a row from another table.
The join condition is specified in the ON clause of the join statement. It can be based on equality between two columns (Equijoin), a comparison operator other than equality (Non-Equijoin), or no condition at all (Cartesian Join).
Here is an example of a Join Condition using the “employees” and “departments” tables:
SELECT employee_name, department_name FROM employees JOIN departments ON employees.department_id = departments.department_id;
This query has a join condition that matches rows from the “employees” and “departments” tables based on the equality of the department ID column.