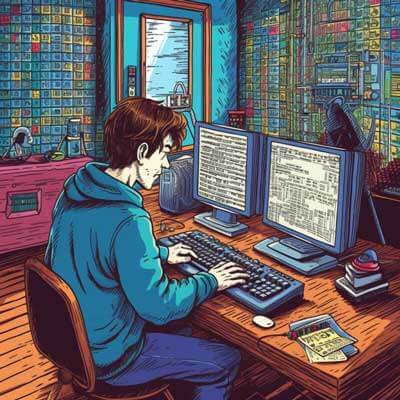
Table of Contents
Introduction to SQL IN and NOT IN Operators
The SQL IN and NOT IN operators are powerful tools for querying databases based on a set of values. These operators allow you to specify multiple values and check if a column's value matches any of those values (IN) or does not match any of those values (NOT IN).
The IN operator is commonly used when you want to filter rows based on a specific set of values. For example, you can use it to retrieve all customers whose country is either "USA", "Canada", or "Mexico".
The NOT IN operator, on the other hand, is used to exclude rows that match a set of values. For instance, you can use it to retrieve all customers whose country is not "USA", "Canada", or "Mexico".
Related Article: Tutorial: Working with SQL Data Types in MySQL
Use Cases for SQL IN Operator
The SQL IN operator is particularly useful in various scenarios. Here are a few examples:
Filtering Data Based on Multiple Values
Suppose you have a table called "employees" with a column named "department". You want to retrieve all employees who work in either the "Sales" or "Marketing" department. You can achieve this using the IN operator:
SELECT * FROM employees WHERE department IN ('Sales', 'Marketing');
This query will return all the rows where the department is either "Sales" or "Marketing".
Subqueries
The IN operator can also be used with subqueries. This allows you to retrieve data from one table based on values in another table. For example, let's say you have a table called "orders" and another table called "customers". You want to find all orders made by customers with a specific set of IDs:
SELECT * FROM orders WHERE customer_id IN (SELECT id FROM customers WHERE status = 'active');
This query will retrieve all orders made by customers whose status is "active".
Related Article: Working With PostgreSQL: Extracting Day of Week
Use Cases for SQL NOT IN Operator
The SQL NOT IN operator can be handy in various situations. Here are a few examples:
Excluding Data Based on Multiple Values
Suppose you have a table called "products" with a column named "category". You want to retrieve all products except those in the categories "Electronics" and "Clothing". The NOT IN operator can help you achieve this:
SELECT * FROM products WHERE category NOT IN ('Electronics', 'Clothing');
This query will return all the rows where the category is not "Electronics" or "Clothing".
Excluding Data Based on Subqueries
Similar to the IN operator, the NOT IN operator can also be used with subqueries. Let's say you have a table called "employees" and another table called "projects". You want to find all employees who are not assigned to any project:
SELECT * FROM employees WHERE id NOT IN (SELECT employee_id FROM projects);
This query will retrieve all employees whose ID does not appear in the "projects" table.
Best Practices for Using SQL IN Operator
To make the best use of the SQL IN operator, consider the following best practices:
Related Article: Tutorial on Database Sharding in MySQL
Use Prepared Statements
When using the IN operator with user-supplied values, always use prepared statements to prevent SQL injection attacks. Prepared statements ensure that the values are properly escaped and treated as data rather than executable code.
Limit the Number of Values
Avoid using an excessive number of values with the IN operator, as it can impact performance. If you need to match against a large number of values, consider using other techniques such as temporary tables or JOIN operations.
Best Practices for Using SQL NOT IN Operator
When working with the SQL NOT IN operator, keep the following best practices in mind:
Ensure Data Consistency
Before using the NOT IN operator, verify that the data you are excluding is consistent. In some cases, using a NOT EXISTS or LEFT JOIN approach may be more appropriate to handle NULL values or data inconsistencies.
Related Article: How to Insert Multiple Rows in a MySQL Database
Consider Performance Implications
Similar to the IN operator, avoid using a large number of values with the NOT IN operator, as it can impact performance. Evaluate alternative approaches, such as using LEFT JOINs or NOT EXISTS, for better performance.
Real World Examples of SQL IN Operator
Let's explore some real-world examples of using the SQL IN operator:
Example 1: Filtering Orders by Multiple Statuses
Suppose you have an "orders" table with a column named "status". You want to retrieve all orders with either "Shipped", "Delivered", or "In Transit" status:
SELECT * FROM orders WHERE status IN ('Shipped', 'Delivered', 'In Transit');
This query will return all orders that have a status matching any of the specified values.
Example 2: Filtering Customers by Multiple IDs
Consider a "customers" table with a column named "id". You want to retrieve all customer records with either ID 1001, 1002, or 1003:
SELECT * FROM customers WHERE id IN (1001, 1002, 1003);
This query will fetch all customers whose ID matches any of the specified values.
Related Article: How To Select Count With Distinct
Real World Examples of SQL NOT IN Operator
Let's explore some real-world examples of using the SQL NOT IN operator:
Example 1: Filtering Products by Excluded Categories
Suppose you have a "products" table with a column named "category". You want to retrieve all products except those in the categories "Books" and "Toys":
SELECT * FROM products WHERE category NOT IN ('Books', 'Toys');
This query will return all products where the category does not match any of the specified values.
Example 2: Filtering Employees without Assigned Projects
Consider an "employees" table and a "projects" table, with the "projects" table containing a column named "employee_id". You want to find all employees who are not assigned to any project:
SELECT * FROM employees WHERE id NOT IN (SELECT employee_id FROM projects);
This query will retrieve all employees whose ID does not appear in the "projects" table.
Performance Considerations for SQL IN and NOT IN Operators
While the SQL IN and NOT IN operators are useful, they can have performance implications. Here are some considerations to keep in mind:
Related Article: How to Convert Text to Uppercase in Postgresql using UCASE
Indexing
Ensure that the columns used with the IN and NOT IN operators are properly indexed. This can significantly improve query performance, especially when dealing with large datasets.
Query Optimization
Optimize your queries by avoiding unnecessary computations or redundant subqueries. Analyze the execution plans and use appropriate techniques like JOINs or EXISTS when they provide better performance.
Advanced Techniques with SQL IN Operator
In addition to the basic usage, there are advanced techniques you can employ with the SQL IN operator:
Combining IN Operators
You can combine multiple IN operators to create complex queries. For example, if you want to find all customers from either the "USA" or "Canada" who have made orders in the last month:
SELECT * FROM customers WHERE country IN ('USA', 'Canada') AND id IN (SELECT customer_id FROM orders WHERE order_date >= DATE_SUB(NOW(), INTERVAL 1 MONTH));
This query will retrieve customers matching the specified countries and who have made orders in the last month.
Related Article: Tutorial: Dealing with Non-Existent Relations in PostgreSQL
Using Subqueries with IN Operator
You can also use subqueries with the IN operator to create more dynamic queries. For instance, if you want to retrieve all products that have been ordered at least once:
SELECT * FROM products WHERE id IN (SELECT product_id FROM orders);
This query will fetch products whose ID appears in the "orders" table.
Advanced Techniques with SQL NOT IN Operator
Similar to the IN operator, the NOT IN operator can be used in advanced ways:
Combining NOT IN Operators
You can combine multiple NOT IN operators to create complex exclusion queries. For example, if you want to retrieve all customers who have not made any orders in the last month and are not from the "USA" or "Canada":
SELECT * FROM customers WHERE id NOT IN (SELECT customer_id FROM orders WHERE order_date >= DATE_SUB(NOW(), INTERVAL 1 MONTH)) AND country NOT IN ('USA', 'Canada');
This query will exclude customers who have made orders in the last month or whose country is "USA" or "Canada".
Using Subqueries with NOT IN Operator
You can also use subqueries with the NOT IN operator to perform more intricate exclusion queries. For example, if you want to retrieve all products that have never been ordered:
SELECT * FROM products WHERE id NOT IN (SELECT product_id FROM orders);
This query will fetch products whose ID does not appear in the "orders" table.
Related Article: How to Resolve Secure File Priv in MySQL
Code Snippet 1: Basic Usage of SQL IN Operator
Here's a code snippet demonstrating the basic usage of the SQL IN operator:
SELECT * FROM employees WHERE department IN ('Sales', 'Marketing');
This query retrieves all employees working in the "Sales" or "Marketing" department.
Code Snippet 2: Basic Usage of SQL NOT IN Operator
Below is a code snippet illustrating the basic usage of the SQL NOT IN operator:
SELECT * FROM products WHERE category NOT IN ('Electronics', 'Clothing');
This query returns all products where the category is neither "Electronics" nor "Clothing".
Code Snippet 3: Combining SQL IN Operator with Other Operators
This code snippet demonstrates combining the SQL IN operator with other operators:
SELECT * FROM customers WHERE country IN ('USA', 'Canada') AND age > 30;
This query retrieves customers from either the "USA" or "Canada" with an age greater than 30.
Code Snippet 4: Combining SQL NOT IN Operator with Other Operators
The following code snippet showcases combining the SQL NOT IN operator with other operators:
SELECT * FROM employees WHERE department NOT IN ('Sales', 'Marketing') OR salary < 50000;
This query fetches employees whose department is neither "Sales" nor "Marketing" or whose salary is less than 50,000.
Related Article: How to Convert Columns to Rows in PostgreSQL
Code Snippet 5: Dynamic Generation of SQL IN and NOT IN Clauses
Dynamic generation of SQL IN and NOT IN clauses is useful when dealing with user input or programmatically generated queries. Here's an example of dynamically generating the IN clause using Python:
values = ['USA', 'Canada', 'Mexico'] query = "SELECT * FROM customers WHERE country IN ({})".format(','.join(['%s'] * len(values))) cursor.execute(query, values)
This code dynamically generates the IN clause based on the values in the "values" list and executes the query using a prepared statement.
Error Handling for SQL IN and NOT IN Operators
When using the SQL IN and NOT IN operators, it's crucial to handle any potential errors properly. Implementing robust error handling can help prevent unexpected behavior and ensure the reliability of your application.
Consider using try-catch blocks or similar error handling mechanisms provided by your programming language or database framework. These mechanisms allow you to catch and handle any exceptions or errors that may occur during the execution of queries involving the IN and NOT IN operators.