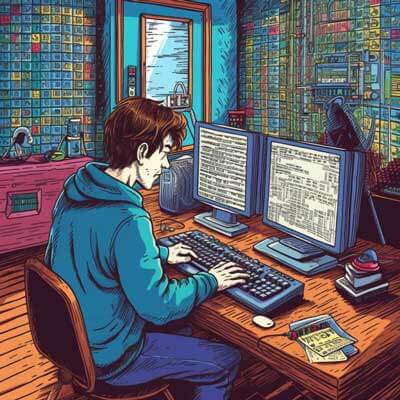
- Primary Keys in Database Tables
- Foreign Keys in Database Tables
- Writing Queries in SQL
- Relational Databases and Their Functionality
- Data Manipulation in SQL
- Retrieving Data from Databases using SQL
- Understanding Data Integrity in Databases
- Exploring Different Types of Joins in SQL
- Inner Join: A Deep Dive into Its Functionality
Primary Keys in Database Tables
In SQL, a primary key is a column or set of columns that uniquely identifies each record in a table. It is used to enforce data integrity and ensure that each record in the table is unique. The primary key constraint is applied to one or more columns in a table, and it guarantees that the values in those columns are unique and not null.
Let’s consider an example where we have a table called “Customers” with columns “CustomerID,” “FirstName,” and “LastName.” In this case, the “CustomerID” column can be designated as the primary key, as it uniquely identifies each customer.
Here’s an example of creating a table with a primary key in SQL:
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, FirstName VARCHAR(50), LastName VARCHAR(50) );
Related Article: Tutorial: ON for JOIN SQL in Databases
Foreign Keys in Database Tables
In SQL, a foreign key is a column or set of columns in a table that refers to the primary key of another table. It establishes a relationship between two tables and ensures referential integrity. By using foreign keys, we can create relationships between tables and enforce constraints on the data.
Let’s consider an example where we have two tables, “Orders” and “Customers.” The “Orders” table has a foreign key column called “CustomerID,” which references the primary key of the “Customers” table.
Here’s an example of creating tables with a foreign key relationship in SQL:
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, FirstName VARCHAR(50), LastName VARCHAR(50) ); CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerID INT, OrderDate DATE, FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID) );
Writing Queries in SQL
In SQL, queries are used to retrieve and manipulate data stored in a database. They allow us to perform various operations such as selecting, inserting, updating, and deleting data. SQL queries are written using the SELECT, INSERT, UPDATE, and DELETE statements, along with other clauses and functions.
Let’s consider an example where we want to retrieve all the customers from the “Customers” table:
SELECT * FROM Customers;
This query will return all the rows from the “Customers” table.
Relational Databases and Their Functionality
Relational databases are a type of database management system (DBMS) that organizes data into tables with rows and columns. They are based on the relational model, which represents data as a collection of relations (tables) with predefined relationships between them.
Relational databases provide several key functionalities, including:
1. Data organization: Relational databases organize data into tables, with each table representing a specific entity or concept. This allows for efficient storage and retrieval of data.
2. Data integrity: Relational databases enforce data integrity through the use of constraints such as primary keys, foreign keys, and data types. These constraints ensure that the data stored in the database is accurate and consistent.
3. Data manipulation: Relational databases support various operations for manipulating data, such as inserting, updating, and deleting records. These operations can be performed using SQL queries.
4. Data retrieval: Relational databases allow for efficient retrieval of data using SQL queries. Queries can be written to retrieve specific records or to perform complex joins and aggregations.
Related Article: Analyzing SQL Join and Its Effect on Records
Data Manipulation in SQL
Data manipulation in SQL involves performing operations on data stored in a database. The main operations include inserting new records, updating existing records, and deleting records.
Let’s consider an example where we want to insert a new customer into the “Customers” table:
INSERT INTO Customers (CustomerID, FirstName, LastName) VALUES (1, 'John', 'Doe');
This query will insert a new customer with a CustomerID of 1, FirstName of ‘John’, and LastName of ‘Doe’ into the “Customers” table.
Retrieving Data from Databases using SQL
Retrieving data from databases using SQL involves writing queries to select specific records or perform complex joins and aggregations. SQL provides a rich set of operators, functions, and clauses to retrieve data in a flexible and efficient manner.
Let’s consider an example where we want to retrieve all the orders placed by a specific customer:
SELECT * FROM Orders WHERE CustomerID = 1;
This query will return all the orders from the “Orders” table where the CustomerID is 1.
Understanding Data Integrity in Databases
Data integrity in databases refers to the accuracy, consistency, and reliability of data stored in a database. It ensures that the data is valid and conforms to predefined rules and constraints.
There are several types of data integrity constraints that can be applied to a database, including:
1. Entity integrity: Ensures that each row in a table is uniquely identified by a primary key.
2. Referential integrity: Ensures that foreign key values in a table match the primary key values in the referenced table.
3. Domain integrity: Ensures that the values stored in a column are valid and conform to the defined data type and constraints.
4. User-defined integrity: Allows for the definition of custom rules and constraints to ensure data integrity.
Related Article: Tutorial: Full Outer Join versus Join in SQL
Exploring Different Types of Joins in SQL
In SQL, joins are used to combine rows from two or more tables based on a related column between them. There are several types of joins, including inner join, left join, right join, and full outer join. Each type of join returns a different set of results based on the relationship between the tables.
Let’s consider an example where we have two tables, “Customers” and “Orders,” and we want to retrieve all the orders along with the customer information:
SELECT Orders.OrderID, Customers.FirstName, Customers.LastName FROM Orders INNER JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
This query uses an inner join to combine the “Orders” and “Customers” tables based on the matching CustomerID column. It retrieves the OrderID from the “Orders” table and the FirstName and LastName from the “Customers” table.
Inner Join: A Deep Dive into Its Functionality
The inner join is one of the most commonly used join types in SQL. It returns only the rows that have matching values in both tables being joined. The result set contains only the common records between the tables.
Let’s consider an example where we have two tables, “Customers” and “Orders,” and we want to retrieve all the orders along with the customer information:
SELECT Orders.OrderID, Customers.FirstName, Customers.LastName FROM Orders INNER JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
In this example, the inner join combines the “Orders” and “Customers” tables based on the matching CustomerID column. It retrieves the OrderID from the “Orders” table and the FirstName and LastName from the “Customers” table.
The inner join works by comparing the values in the specified columns of the two tables being joined. It includes only the rows where the values match in both tables. If there are no matching values, those rows are excluded from the result set.
The inner join can be used to combine more than two tables as well. In such cases, the join conditions should be specified for each pair of tables being joined.