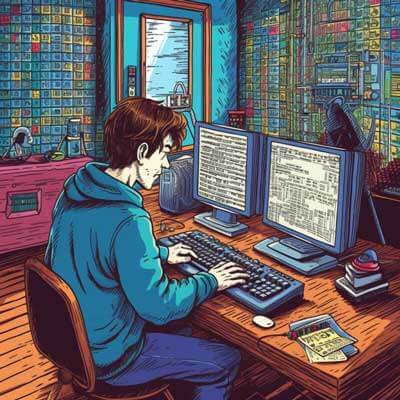
Table of Contents
In Python, the NoneType object represents the absence of a value. It is commonly used to indicate that a variable does not currently hold any data. However, working with NoneType objects can sometimes lead to unexpected errors or inconsistencies in your code. In this guide, we will explore various techniques and best practices for handling NoneType objects in Python.
Understanding NoneType
The NoneType object is a built-in type in Python that represents the absence of a value. It is similar to other programming languages' null or nil values. When a function or method does not explicitly return a value, Python automatically assigns None to it. None can also be explicitly assigned to a variable to indicate that it does not currently hold any data.
Here's an example that demonstrates the use of NoneType:
def get_name(): return None name = get_name() if name is None: print("Name is not available.") else: print(f"Name: {name}")
In this example, the get_name()
function returns None, indicating that the name is not available. The code then checks if the name
variable holds a None value and prints an appropriate message.
Related Article: Build a Chat Web App with Flask, MongoDB, Reactjs & Docker
Checking for NoneType
To handle NoneType objects in Python, you can use conditional statements to check if a variable contains None. This allows you to perform different actions based on whether the variable holds a value or None.
Here's an example that demonstrates how to check for NoneType:
def get_name(): return None name = get_name() if name is None: print("Name is not available.") else: print(f"Name: {name}")
In this example, the is
keyword is used to check if the name
variable contains None. If it does, the code prints the "Name is not available." message. Otherwise, it prints the actual name.
Handling NoneType Errors
When working with NoneType objects, it is important to handle potential errors that may arise. Performing operations on a NoneType object can result in a TypeError
. To avoid such errors, you can use conditional statements to ensure that the object is not None before performing any operations on it.
Here's an example that demonstrates how to handle NoneType errors:
def get_length(string): if string is None: return 0 else: return len(string) name = None length = get_length(name) print(f"Length of name: {length}")
In this example, the get_length()
function checks if the string
parameter is None. If it is, the function returns 0. Otherwise, it returns the length of the string using the len()
function. By handling the NoneType case explicitly, the code avoids a potential TypeError
when trying to calculate the length of a None object.
Alternative Approaches
Apart from using conditional statements, there are other approaches you can consider when handling NoneType objects in Python:
1. Default Values: You can use the or
operator to provide a default value for a variable that may be None. For example:
name = None default_name = name or "Unknown" print(f"Name: {default_name}")
In this example, if the name
variable is None, the default_name
variable is assigned the value "Unknown". This technique can be useful when you want to assign a default value to a variable that may be None.
2. Exception Handling: You can use exception handling to catch and handle NoneType errors. For example:
def get_length(string): try: return len(string) except TypeError: return 0 name = None length = get_length(name) print(f"Length of name: {length}")
In this example, the len()
function is called within a try-except block. If a TypeError
occurs, indicating that the object is None, the code returns a default value of 0. This approach allows you to handle NoneType errors more gracefully.
Related Article: How to Convert a String to Lowercase in Python
Best Practices
When working with NoneType objects in Python, keep the following best practices in mind:
- Always check if a variable is None before performing operations on it to avoid potential TypeError
errors.
- Use conditional statements or the is
keyword to check for NoneType.
- Consider providing default values for variables that may be None using the or
operator.
- Handle NoneType errors using try-except blocks for more graceful error handling.
For more information on NoneType and handling None values in Python, you can refer to the official Python documentation: https://docs.python.org/3/library/constants.html#None.