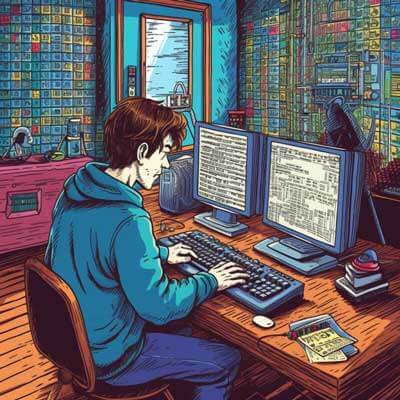
Table of Contents
Overview of Numpy Concatenate
Numpy is a useful library in Python for scientific computing and data manipulation. One of its key features is the ability to concatenate arrays, which allows you to combine multiple arrays into a single array. This can be particularly useful when working with large datasets or when you need to perform operations on arrays with different dimensions.
In this article, we will explore the different ways to concatenate arrays using Numpy. We will cover the syntax for concatenating arrays, how to handle arrays with different dimensions, the use of the axis parameter, and various techniques for reshaping and flattening arrays before concatenating them. We will also discuss the difference between vstack and hstack, the dstack function, and the importance of transposing arrays before concatenation.
Related Article: How To Write Pandas Dataframe To CSV File
Syntax for Concatenating Arrays
Before diving into the different techniques for concatenating arrays, let's start with the basic syntax for concatenation in Numpy. The concatenate function in Numpy is used to combine two or more arrays into a single array.
Here is the syntax for concatenating two arrays using the concatenate function:
numpy.concatenate((array1, array2), axis=0)
The array1
and array2
parameters are the arrays that you want to concatenate. The axis
parameter specifies the axis along which the arrays will be joined. By default, the axis
parameter is set to 0, which means the arrays will be concatenated along the first axis.
Concatenating Arrays with Different Dimensions
In some cases, you may need to concatenate arrays with different dimensions. Numpy provides a convenient way to handle this situation by automatically reshaping the arrays to match before concatenation.
For example, let's say we have two arrays: array1
with shape (3,) and array2
with shape (2, 3). When we try to concatenate these arrays, Numpy will automatically reshape array1
to have shape (1, 3) so that it matches the shape of array2
.
Here is an example:
import numpy as np array1 = np.array([1, 2, 3]) array2 = np.array([[4, 5, 6], [7, 8, 9]]) result = np.concatenate((array1, array2)) print(result)
Output:
[[1 2 3] [4 5 6] [7 8 9]]
As you can see, Numpy reshapes array1
to have shape (1, 3) before concatenating it with array2
. This allows us to concatenate arrays with different dimensions without any issues.
Concatenating Arrays with Axis Parameter
The axis
parameter in the concatenate function allows you to specify the axis along which the arrays will be joined. By default, the axis
parameter is set to 0, which means the arrays will be concatenated along the first axis.
Let's take a look at an example to understand how the axis
parameter works:
import numpy as np array1 = np.array([[1, 2, 3], [4, 5, 6]]) array2 = np.array([[7, 8, 9], [10, 11, 12]]) result = np.concatenate((array1, array2), axis=0) print(result)
Output:
[[ 1 2 3] [ 4 5 6] [ 7 8 9] [10 11 12]]
In this example, both array1
and array2
have shape (2, 3). When we concatenate them along axis=0
, the result is a new array with shape (4, 3). The arrays are stacked vertically, one on top of the other.
If we change the axis
parameter to 1, the arrays will be concatenated along the second axis:
import numpy as np array1 = np.array([[1, 2, 3], [4, 5, 6]]) array2 = np.array([[7, 8, 9], [10, 11, 12]]) result = np.concatenate((array1, array2), axis=1) print(result)
Output:
[[ 1 2 3 7 8 9] [ 4 5 6 10 11 12]]
In this case, the result is a new array with shape (2, 6). The arrays are stacked horizontally, side by side.
Related Article: Advance Django Forms: Dynamic Generation, Processing, and Custom Widgets
Reshaping Arrays Before Concatenating
In some cases, you may need to reshape the arrays before concatenating them to ensure they have compatible shapes. Numpy provides the reshape
function to reshape arrays.
Let's take a look at an example:
import numpy as np array1 = np.array([[1, 2, 3], [4, 5, 6]]) array2 = np.array([7, 8, 9]) reshaped_array2 = np.reshape(array2, (1, 3)) result = np.concatenate((array1, reshaped_array2), axis=0) print(result)
Output:
[[1 2 3] [4 5 6] [7 8 9]]
In this example, we reshape array2
to have shape (1, 3) using the reshape
function before concatenating it with array1
. This ensures that the arrays have compatible shapes and can be concatenated along axis=0
.
Flattening Arrays Before Concatenating
Another useful technique when concatenating arrays is to flatten them before concatenation. Flattening an array means converting it from a multi-dimensional array to a one-dimensional array.
Numpy provides the flatten
function to flatten arrays. Let's take a look at an example:
import numpy as np array1 = np.array([[1, 2, 3], [4, 5, 6]]) array2 = np.array([[7, 8, 9], [10, 11, 12]]) flattened_array1 = np.flatten(array1) flattened_array2 = np.flatten(array2) result = np.concatenate((flattened_array1, flattened_array2)) print(result)
Output:
[ 1 2 3 4 5 6 7 8 9 10 11 12]
In this example, we use the flatten
function to flatten array1
and array2
before concatenating them. The resulting array is a one-dimensional array that contains all the elements from both arrays.
Code Snippet for Concatenating Arrays
Here is a code snippet that demonstrates how to concatenate arrays using Numpy:
import numpy as np array1 = np.array([1, 2, 3]) array2 = np.array([4, 5, 6]) result = np.concatenate((array1, array2)) print(result)
Output:
[1 2 3 4 5 6]
In this example, we concatenate array1
and array2
to create a new array that contains all the elements from both arrays.
Joining Arrays Along a Specified Axis
Numpy provides two additional functions, vstack
and hstack
, for joining arrays along a specified axis. These functions are similar to the concatenate
function but provide a more intuitive way to join arrays vertically and horizontally.
The vstack
function is used to vertically stack arrays, meaning they are joined along the first axis (rows). Let's take a look at an example:
import numpy as np array1 = np.array([[1, 2, 3], [4, 5, 6]]) array2 = np.array([[7, 8, 9], [10, 11, 12]]) result = np.vstack((array1, array2)) print(result)
Output:
[[ 1 2 3] [ 4 5 6] [ 7 8 9] [10 11 12]]
In this example, we use the vstack
function to vertically stack array1
and array2
, resulting in a new array with shape (4, 3).
The hstack
function is used to horizontally stack arrays, meaning they are joined along the second axis (columns). Let's take a look at an example:
import numpy as np array1 = np.array([[1, 2], [3, 4]]) array2 = np.array([[5, 6], [7, 8]]) result = np.hstack((array1, array2)) print(result)
Output:
[[1 2 5 6] [3 4 7 8]]
In this example, we use the hstack
function to horizontally stack array1
and array2
, resulting in a new array with shape (2, 4).
Related Article: How To Get Row Count Of Pandas Dataframe
Difference Between vstack and hstack
The main difference between vstack
and hstack
is the axis along which the arrays are joined. vstack
joins arrays along the first axis (rows), while hstack
joins arrays along the second axis (columns).
Let's take a look at an example to understand the difference:
import numpy as np array1 = np.array([[1, 2], [3, 4]]) array2 = np.array([[5, 6], [7, 8]]) result_vstack = np.vstack((array1, array2)) result_hstack = np.hstack((array1, array2)) print(result_vstack) print(result_hstack)
Output:
[[1 2] [3 4] [5 6] [7 8]] [[1 2 5 6] [3 4 7 8]]
As you can see, vstack
joins the arrays along the first axis, resulting in a new array with shape (4, 2). hstack
joins the arrays along the second axis, resulting in a new array with shape (2, 4).
dstack in Numpy Concatenation
In addition to vstack
and hstack
, Numpy provides the dstack
function for joining arrays along the third axis. This is useful when working with multi-dimensional arrays.
Let's take a look at an example to understand how dstack
works:
import numpy as np array1 = np.array([[1, 2], [3, 4]]) array2 = np.array([[5, 6], [7, 8]]) result = np.dstack((array1, array2)) print(result)
Output:
[[[1 5] [2 6]] [[3 7] [4 8]]]
In this example, we use the dstack
function to join array1
and array2
along the third axis. The resulting array is a new three-dimensional array with shape (2, 2, 2).
Transposing Arrays Before Concatenating
Sometimes, you may need to transpose arrays before concatenating them to ensure they have compatible shapes. Numpy provides the transpose
function to transpose arrays.
Let's take a look at an example:
import numpy as np array1 = np.array([[1, 2, 3], [4, 5, 6]]) array2 = np.array([[7, 8, 9], [10, 11, 12]]) transposed_array1 = np.transpose(array1) transposed_array2 = np.transpose(array2) result = np.concatenate((transposed_array1, transposed_array2), axis=1) print(result)
Output:
[[ 1 4 7 10] [ 2 5 8 11] [ 3 6 9 12]]
In this example, we transpose array1
and array2
using the transpose
function before concatenating them. This ensures that the arrays have compatible shapes and can be concatenated along axis=1
.
Additional Resources
- Concatenating Arrays in NumPy