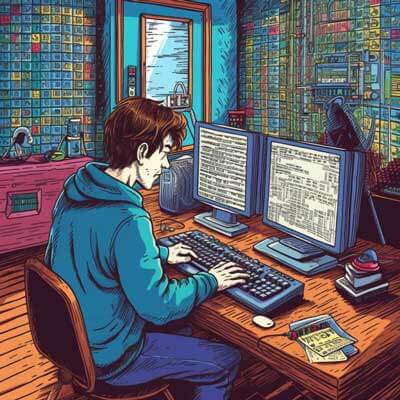
Filtering dataframe rows based on column values is a common task in data analysis and manipulation using the Python library, pandas. There are several ways to accomplish this, and in this answer, we will explore two popular methods: boolean indexing and the query function.
Why is this question asked?
This question is often asked by data analysts and data scientists who work with tabular data in Python using the pandas library. Filtering rows based on column values is a fundamental operation in data analysis, as it allows for selecting specific subsets of data that meet certain conditions. By filtering data, analysts can focus on the relevant information and perform further analysis or visualization.
There are various reasons why someone would want to filter dataframe rows based on column values:
1. Data cleaning: Filtering can be used to remove rows with missing or incorrect data, ensuring the quality and integrity of the dataset.
2. Data exploration: Analysts often want to focus on a subset of data that meets specific criteria to gain insights or investigate patterns.
3. Data preprocessing: Filtering can be used as a preprocessing step before performing statistical analysis or building machine learning models.
Related Article: How To Convert a Python Dict To a Dataframe
Method 1: Boolean Indexing
One of the most common methods to filter dataframe rows based on column values in pandas is using boolean indexing. Boolean indexing allows you to filter rows based on a condition or a set of conditions, resulting in a new dataframe that only includes the rows that satisfy the condition(s).
To demonstrate this method, let’s consider a simple example where we have a dataframe containing information about students, including their names, ages, and grades:
import pandas as pd data = {'Name': ['Alice', 'Bob', 'Charlie', 'David'], 'Age': [21, 22, 23, 24], 'Grade': ['A', 'B', 'A', 'C']} df = pd.DataFrame(data)
To filter the dataframe and select only the rows where the students have received an ‘A’ grade, we can use boolean indexing as follows:
filtered_df = df[df['Grade'] == 'A']
In this example, df['Grade'] == 'A'
creates a boolean series with True
values for rows where the grade is ‘A’ and False
values otherwise. By passing this boolean series as an index to the dataframe df
, we obtain a new dataframe (filtered_df
) that contains only the rows where the condition is True
.
It is also possible to apply multiple conditions using boolean operators. For example, to filter the dataframe and select only the rows where students have an ‘A’ grade and are older than 21, we can do the following:
filtered_df = df[(df['Grade'] == 'A') & (df['Age'] > 21)]
In this case, we use the &
operator to combine the two conditions. The resulting filtered_df
dataframe will contain only the rows that satisfy both conditions.
Method 2: The Query Function
Another method to filter dataframe rows based on column values is by using the query
function provided by pandas. The query
function allows you to filter rows using a SQL-like syntax, making it easier to express complex conditions.
To demonstrate this method, let’s continue with the previous example and filter the dataframe using the query
function:
filtered_df = df.query("Grade == 'A'")
In this example, we pass the condition "Grade == 'A'"
as a string to the query
function. The query
function evaluates the condition and returns a new dataframe (filtered_df
) that contains only the rows where the condition is true.
Similar to boolean indexing, you can also apply multiple conditions using the query
function. For example, to filter the dataframe and select only the rows where students have an ‘A’ grade and are older than 21, we can do the following:
filtered_df = df.query("Grade == 'A' and Age > 21")
In this case, we use the and
keyword to combine the two conditions. The resulting filtered_df
dataframe will contain only the rows that satisfy both conditions.
Best Practices and Suggestions
When filtering dataframe rows based on column values, it is important to keep in mind some best practices and suggestions:
1. Use descriptive column names: Make sure your dataframe has meaningful column names that reflect the data they contain. This will make it easier to write and understand the filtering conditions.
2. Avoid chained indexing: Chained indexing refers to the practice of using multiple indexing operations one after another (e.g., df[condition1][condition2]
). While it may seem convenient, it can lead to unpredictable results and should be avoided. Instead, use boolean indexing or the query
function to apply multiple conditions in a single operation.
3. Handle missing values: When filtering rows based on column values, consider how missing values (NaN
) should be treated. By default, missing values are treated as False
in boolean indexing and are excluded from the result. However, you can handle missing values differently by using the isna
or notna
functions to check for missing values explicitly.
4. Avoid unnecessary copying: When filtering dataframe rows, pandas returns a new dataframe that contains only the selected rows. However, this new dataframe shares the same underlying data as the original dataframe. If you plan to modify the filtered dataframe extensively, consider making a copy of it using the copy
method to avoid unintended modifications to the original dataframe.
5. Consider performance implications: Depending on the size of your dataframe and the complexity of the filtering conditions, filtering rows can be computationally expensive. If performance is a concern, consider using alternative techniques, such as using numpy arrays or leveraging pandas’ built-in functions for faster filtering.
Related Article: How To Get Row Count Of Pandas Dataframe