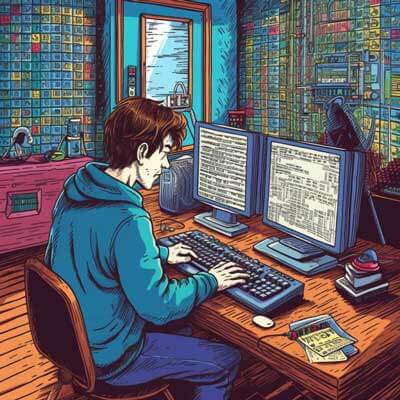
- Introduction to the Concept of Language Selection
- Language Syntax and Style
- Data Types and Variables
- Overview of Ecosystems
- PHP Composer
- Python Package Manager (pip)
- Analysis of Active Libraries
- PHP Image Manipulation with Intervention Image
- Python Image Manipulation with Pillow
- Productivity Comparison
- PHP Framework Laravel
- Python Framework Flask
- Performance Evaluation
- PHP Fibonacci Sequence
- Python Fibonacci Sequence
- Third-Party Tools Comparison
- PHPUnit
- Python pytest
- Security Considerations in Language Selection
- PHP Prepared Statements
- Python Parameterized Queries
- Scalability: A Comparative Analysis
- PHP Scaling with Horizon
- Python Scaling with Celery
- Use Case 1: Web Development
- PHP Framework Laravel
- Python Framework Django
- Use Case 2: Data Analysis
- PHP Data Analysis with PhpSpreadsheet
- Python Data Analysis with pandas
- Use Case 3: Automation Scripts
- PHP Automation Script
- Python Automation Script
- Best Practice: Code Organization
- PHP Code Organization
- Python Code Organization
- Best Practice: Testing and Debugging
- PHP Unit Testing with PHPUnit
- Python Unit Testing with pytest
- Real World Example: E-commerce Platform Development
- PHP E-commerce Platform
- Python E-commerce Platform
- Real World Example: Machine Learning Application
- PHP Machine Learning with PHP-ML
- Python Machine Learning with scikit-learn
- Performance Consideration: Execution Speed
- PHP Execution Speed
- Python Execution Speed
- Performance Consideration: Database Interaction Efficiency
- PHP Database Interaction with PDO
- Python Database Interaction with SQLAlchemy
- Advanced Technique: Multithreading
- PHP Multithreading with pthreads
- Python Multithreading with threading
- Advanced Technique: Asynchronous Programming
- PHP Asynchronous Programming with ReactPHP
- Python Asynchronous Programming with asyncio
- Creating a REST API
- PHP REST API with Laravel
- Python REST API with Flask
- Database Connection
- PHP Database Connection with PDO
- Python Database Connection with SQLAlchemy
- User Authentication
- PHP User Authentication with Laravel
- Python User Authentication with Django
- Data Visualization
- PHP Data Visualization with Chart.js
- Python Data Visualization with Matplotlib
- Web Scraping
- PHP Web Scraping with Guzzle and DOMXPath
- Python Web Scraping with BeautifulSoup
- Error Handling
- PHP Error Handling with try-catch
- Python Error Handling with try-except
Introduction to the Concept of Language Selection
When embarking on a new software project, one of the crucial decisions to make is choosing the right programming language. In this chapter, we will explore the factors to consider when selecting between PHP and Python, two popular languages in the world of web development and beyond.
Language Syntax and Style
The syntax and style of a programming language play a significant role in developer productivity and code maintainability. Let’s compare the syntax of PHP and Python using some code snippets.
PHP Syntax
Python Syntax
print("Hello, World!")
As you can see, PHP uses echo
to output text, while Python uses print
. Python’s syntax is generally considered to be more concise and readable than PHP’s. This simplicity can lead to increased developer productivity and faster code comprehension.
Data Types and Variables
PHP and Python have different approaches to data types and variable declaration. Let’s take a look at how each language handles this:
PHP Data Types and Variables
Python Data Types and Variables
name = "John" age = 25 is_student = True
In PHP, variables are declared using the $
symbol, while Python does not require any explicit declaration. Additionally, Python uses snake_case for variable names, while PHP traditionally uses camelCase. These differences may influence your preference depending on your coding style and habits.
Overview of Ecosystems
The ecosystem surrounding a programming language plays a crucial role in its overall usability and support. In this section, we will compare the ecosystems of PHP and Python and explore the available tools and resources.
PHP Composer
PHP’s ecosystem is centered around Composer, a dependency management tool. With Composer, you can easily manage and install third-party libraries for your PHP projects. Here’s an example of using Composer to install the Guzzle HTTP client library:
composer require guzzlehttp/guzzle
Python Package Manager (pip)
Python’s ecosystem revolves around pip, the package manager for installing third-party libraries. Let’s see an example of using pip to install the requests library:
pip install requests
Both Composer and pip provide a vast array of libraries and packages that can enhance your development experience and speed up project implementation.
Analysis of Active Libraries
The availability and quality of libraries can significantly impact the development process. In this section, we will compare the active libraries in PHP and Python for common use cases.
PHP Image Manipulation with Intervention Image
PHP offers a popular library called Intervention Image, which simplifies image manipulation tasks. Here’s an example of resizing an image using Intervention Image:
resize(800, 600); $image->save('path/to/resized_image.jpg'); ?>
Python Image Manipulation with Pillow
Python provides the Pillow library, a powerful image processing library. Let’s see how to resize an image using Pillow:
from PIL import Image image = Image.open('path/to/image.jpg') resized_image = image.resize((800, 600)) resized_image.save('path/to/resized_image.jpg')
Both Intervention Image and Pillow offer extensive functionality for image manipulation, making them valuable tools for developers in their respective languages.
Productivity Comparison
Productivity is a critical aspect to consider when choosing a programming language. In this section, we will compare PHP and Python in terms of developer productivity.
PHP Framework Laravel
PHP boasts a popular framework called Laravel, which focuses on developer productivity and elegant syntax. Here’s an example of a simple route in Laravel:
Route::get('/users', function () { return view('users'); });
Python Framework Flask
Python offers Flask, a lightweight and flexible web framework. Let’s examine a basic route definition in Flask:
from flask import Flask app = Flask(__name__) @app.route('/users') def users(): return render_template('users.html')
Both Laravel and Flask provide a solid foundation for web development, with Laravel emphasizing a more structured approach and Flask offering simplicity and flexibility.
Performance Evaluation
Performance is a crucial consideration for many applications. In this section, we will compare the performance of PHP and Python in different scenarios.
PHP Fibonacci Sequence
PHP can efficiently handle computational tasks, such as calculating a Fibonacci sequence. Here’s an example of generating the first 10 numbers of the sequence in PHP:
function fibonacci($n) { $fib = [0, 1]; for ($i = 2; $i < $n; $i++) { $fib[$i] = $fib[$i - 1] + $fib[$i - 2]; } return $fib; } $result = fibonacci(10);
Python Fibonacci Sequence
Python is known for its simplicity and ease of use. Let’s see how Python handles the Fibonacci sequence:
def fibonacci(n): fib = [0, 1] for i in range(2, n): fib.append(fib[i - 1] + fib[i - 2]) return fib result = fibonacci(10)
Both PHP and Python can handle computational tasks effectively, with Python’s concise syntax often providing a more readable solution.
Third-Party Tools Comparison
Third-party tools can greatly influence the development process. In this section, we will compare the availability and capabilities of third-party tools for PHP and Python.
PHPUnit
PHPUnit is a widely-used testing framework for PHP. Let’s see how to define a basic test case using PHPUnit:
use PHPUnit\Framework\TestCase; class MyTest extends TestCase { public function testAddition() { $result = 2 + 2; $this->assertEquals(4, $result); } }
Python pytest
Python offers pytest, a flexible and powerful testing framework. Here’s an example of a pytest test case:
def test_addition(): result = 2 + 2 assert result == 4
Both PHPUnit and pytest provide comprehensive testing capabilities, allowing developers to ensure the quality and correctness of their code.
Security Considerations in Language Selection
Security is of utmost importance in software development. In this section, we will compare the security considerations of PHP and Python.
PHP Prepared Statements
PHP offers prepared statements as a defense against SQL injection attacks. Here’s an example of using prepared statements in PHP:
$stmt = $pdo->prepare('SELECT * FROM users WHERE username = ?'); $stmt->execute([$username]); $result = $stmt->fetch();
Python Parameterized Queries
Python’s libraries provide similar protection against SQL injection through parameterized queries. Let’s see an example using the psycopg2 library with PostgreSQL:
cursor.execute('SELECT * FROM users WHERE username = %s', (username,)) result = cursor.fetchone()
Both PHP and Python offer robust mechanisms to protect against common security vulnerabilities, ensuring the integrity and confidentiality of data.
Scalability: A Comparative Analysis
Scalability is crucial for applications that experience high traffic and user demand. In this section, we will compare the scalability of PHP and Python.
PHP Scaling with Horizon
PHP provides Horizon, a powerful queue manager that can scale applications by efficiently processing background jobs. Here’s an example of using Horizon to scale a Laravel application:
php artisan horizon
Python Scaling with Celery
Python offers Celery, a distributed task queue framework that enables horizontal scaling. Let’s see an example of configuring and running Celery:
celery -A myapp worker --loglevel=info
Both Horizon and Celery provide robust solutions for scaling applications, ensuring smooth operation under heavy workloads.
Use Case 1: Web Development
Web development is one of the primary use cases for both PHP and Python. In this section, we will compare the capabilities and tools available for web development in each language.
PHP Framework Laravel
Laravel is a popular PHP framework known for its elegance and simplicity. Here’s a code snippet showcasing a basic route definition in Laravel:
Route::get('/users', function () { return view('users'); });
Python Framework Django
Django is a robust Python web framework that emphasizes rapid development and clean design. Let’s see an example of a basic route definition in Django:
from django.urls import path from . import views urlpatterns = [ path('users/', views.users), ]
Both Laravel and Django offer comprehensive features and tools for web development, catering to different preferences and requirements.
Use Case 2: Data Analysis
Data analysis is a vital aspect of many applications. In this section, we will compare the capabilities of PHP and Python for data analysis tasks.
PHP Data Analysis with PhpSpreadsheet
PhpSpreadsheet is a powerful library that allows PHP developers to read, write, and manipulate spreadsheet files. Here’s an example of loading a CSV file and calculating the average of a column:
use PhpOffice\PhpSpreadsheet\IOFactory; $spreadsheet = IOFactory::load('path/to/data.csv'); $worksheet = $spreadsheet->getActiveSheet(); $columnData = $worksheet->getColumnIterator('A')->current(); $data = iterator_to_array($columnData->getCellIterator()); $sum = array_sum($data); $average = $sum / count($data);
Python Data Analysis with pandas
Python’s pandas library is widely used for data analysis and manipulation. Let’s see an example of loading a CSV file and calculating the average of a column using pandas:
import pandas as pd data = pd.read_csv('path/to/data.csv') average = data['column_name'].mean()
Both PhpSpreadsheet and pandas offer powerful capabilities for data analysis, providing developers with the tools they need to extract insights from their data.
Use Case 3: Automation Scripts
Automation scripts can streamline repetitive tasks and increase efficiency. In this section, we will compare PHP and Python for writing automation scripts.
PHP Automation Script
PHP can be used to write automation scripts that interact with databases, APIs, and other systems. Here’s an example of a simple PHP script that fetches data from an API:
Python Automation Script
Python’s simplicity and extensive library support make it an excellent choice for automation scripts. Let’s see an example of a Python script that fetches data from an API:
import requests url = 'https://api.example.com/data' response = requests.get(url) data = response.json() # Process the data
Both PHP and Python provide the necessary tools and libraries to automate tasks efficiently, allowing developers to save time and effort.
Best Practice: Code Organization
Maintaining well-organized code is crucial for readability, maintainability, and collaboration. In this section, we will explore best practices for code organization in PHP and Python.
PHP Code Organization
PHP offers namespaces and autoloaders to organize code into logical units. Here’s an example of using namespaces and an autoloader in PHP:
namespace MyProject; spl_autoload_register(function ($className) { $file = __DIR__ . '/' . str_replace('\\', '/', $className) . '.php'; if (file_exists($file)) { require $file; } }); use MyProject\Models\User;
Python Code Organization
Python encourages the use of modules and packages for code organization. Let’s see an example of importing modules and classes in Python:
from myproject.models import User
Both PHP and Python provide mechanisms for organizing code effectively, ensuring clarity and maintainability throughout the development process.
Best Practice: Testing and Debugging
Testing and debugging are vital aspects of software development. In this section, we will explore best practices for testing and debugging in PHP and Python.
PHP Unit Testing with PHPUnit
PHPUnit is a widely-used testing framework for PHP. Here’s an example of writing a test case using PHPUnit:
use PHPUnit\Framework\TestCase; class MyTest extends TestCase { public function testAddition() { $result = 2 + 2; $this->assertEquals(4, $result); } }
Python Unit Testing with pytest
Python’s pytest framework offers a simple and expressive way to write tests. Let’s see an example of a pytest test case:
def test_addition(): result = 2 + 2 assert result == 4
Both PHPUnit and pytest provide powerful features for testing and debugging, helping developers identify and fix issues in their code.
Real World Example: E-commerce Platform Development
To demonstrate the capabilities of PHP and Python, let’s consider the development of an e-commerce platform. In this section, we will explore how PHP and Python can be used for this real-world scenario.
PHP E-commerce Platform
PHP, with frameworks like Laravel and popular e-commerce packages like WooCommerce, provides a solid foundation for developing an e-commerce platform. Here’s an example of defining a product model and retrieving product data in PHP:
get(); ?>
Python E-commerce Platform
Python, with frameworks like Django and libraries like Django-Oscar, offers a robust solution for building an e-commerce platform. Let’s see an example of defining a product model and retrieving product data in Python:
from django.db import models class Product(models.Model): name = models.CharField(max_length=255) category = models.CharField(max_length=255) # Retrieving product data products = Product.objects.filter(category='electronics')
Both PHP and Python provide the necessary tools and frameworks to develop an e-commerce platform, allowing developers to create feature-rich and scalable solutions.
Real World Example: Machine Learning Application
Machine learning is a rapidly growing field that finds applications in various domains. In this section, we will compare the capabilities of PHP and Python for developing machine learning applications.
PHP Machine Learning with PHP-ML
PHP-ML is a machine learning library for PHP that provides various algorithms and tools. Here’s an example of training a decision tree classifier using PHP-ML:
use Phpml\Classification\DecisionTree; use Phpml\Dataset\CsvDataset; $dataset = new CsvDataset('path/to/data.csv', 1, true); $classifier = new DecisionTree(); $classifier->train($dataset->getSamples(), $dataset->getTargets());
Python Machine Learning with scikit-learn
Python’s scikit-learn library is widely used for machine learning tasks. Let’s see an example of training a decision tree classifier using scikit-learn:
from sklearn import datasets from sklearn.tree import DecisionTreeClassifier iris = datasets.load_iris() classifier = DecisionTreeClassifier() classifier.fit(iris.data, iris.target)
Python, with libraries like scikit-learn, provides a more mature and extensive ecosystem for machine learning, making it the preferred choice for such applications.
Performance Consideration: Execution Speed
Execution speed is crucial for applications that require real-time processing or handle large volumes of data. In this section, we will compare the execution speed of PHP and Python.
PHP Execution Speed
PHP is renowned for its fast execution speed, especially when handling web requests. Here’s an example of a PHP script that calculates the sum of numbers:
$start = microtime(true); $sum = 0; for ($i = 1; $i <= 1000000; $i++) { $sum += $i; } $end = microtime(true); $executionTime = $end - $start;
Python Execution Speed
Python, although not as fast as PHP in some cases, offers excellent execution speed for most applications. Let’s see an example of calculating the sum of numbers in Python:
import time start = time.time() total_sum = sum(range(1, 1000001)) end = time.time() execution_time = end - start
While PHP may have a slight edge in execution speed, both PHP and Python are performant enough for most applications, and the choice between the two should be based on other factors like ecosystem, readability, and developer familiarity.
Performance Consideration: Database Interaction Efficiency
Efficient database interactions are crucial for applications that heavily rely on data storage and retrieval. In this section, we will compare the efficiency of PHP and Python when interacting with databases.
PHP Database Interaction with PDO
PHP provides the PDO (PHP Data Objects) extension for interacting with databases. Here’s an example of querying a database using PDO:
$pdo = new PDO('mysql:host=localhost;dbname=mydatabase', 'username', 'password'); $stmt = $pdo->prepare('SELECT * FROM users WHERE id = :id'); $stmt->bindParam(':id', $id, PDO::PARAM_INT); $stmt->execute();
Python Database Interaction with SQLAlchemy
Python’s SQLAlchemy library provides a powerful and flexible way to interact with databases. Let’s see an example of querying a database using SQLAlchemy:
from sqlalchemy import create_engine, text engine = create_engine('mysql://username:password@localhost/mydatabase') with engine.connect() as conn: query = text('SELECT * FROM users WHERE id = :id') result = conn.execute(query, id=id)
Both PHP’s PDO and Python’s SQLAlchemy offer efficient and secure ways to interact with databases, ensuring optimal performance and data integrity.
Advanced Technique: Multithreading
Multithreading allows applications to execute multiple tasks concurrently, improving performance and responsiveness. In this section, we will compare PHP and Python in terms of multithreading capabilities.
PHP Multithreading with pthreads
PHP’s pthreads extension enables multithreading in PHP applications. Here’s an example of creating a simple multithreaded application using pthreads:
class MyThread extends Thread { public function run() { // Thread logic here } } $thread1 = new MyThread(); $thread2 = new MyThread(); $thread1->start(); $thread2->start(); $thread1->join(); $thread2->join();
Python Multithreading with threading
Python’s threading module provides a straightforward way to implement multithreading. Let’s see an example of creating and running multiple threads in Python:
import threading def my_function(): # Thread logic here thread1 = threading.Thread(target=my_function) thread2 = threading.Thread(target=my_function) thread1.start() thread2.start() thread1.join() thread2.join()
Both PHP and Python offer multithreading capabilities, allowing developers to leverage the power of concurrency and improve application performance.
Advanced Technique: Asynchronous Programming
Asynchronous programming enables applications to handle multiple tasks concurrently without blocking execution. In this section, we will compare PHP and Python in terms of asynchronous programming capabilities.
PHP Asynchronous Programming with ReactPHP
ReactPHP is a popular library that brings asynchronous programming to PHP. Here’s an example of using ReactPHP to perform asynchronous HTTP requests:
$loop = React\EventLoop\Factory::create(); $client = new React\HttpClient\Client($loop); $request1 = $client->request('GET', 'https://api.example.com/data/1'); $request2 = $client->request('GET', 'https://api.example.com/data/2'); $request1->on('response', function ($response) { // Process response }); $request2->on('response', function ($response) { // Process response }); $loop->run();
Python Asynchronous Programming with asyncio
Python’s asyncio library provides a powerful framework for asynchronous programming. Let’s see an example of performing asynchronous HTTP requests using asyncio:
import aiohttp import asyncio async def fetch(session, url): async with session.get(url) as response: return await response.text() async def main(): async with aiohttp.ClientSession() as session: tasks = [ asyncio.create_task(fetch(session, 'https://api.example.com/data/1')), asyncio.create_task(fetch(session, 'https://api.example.com/data/2')) ] responses = await asyncio.gather(*tasks) # Process responses asyncio.run(main())
Both ReactPHP and asyncio provide powerful tools for asynchronous programming, enabling developers to build high-performance and responsive applications.
Creating a REST API
Creating a REST API is a common task in web development. In this section, we will compare PHP and Python in terms of building a REST API.
PHP REST API with Laravel
Laravel, a popular PHP framework, simplifies the process of building REST APIs. Here’s an example of defining a route and handling a POST request in Laravel:
use Illuminate\Http\Request; use Illuminate\Support\Facades\Route; Route::post('/users', function (Request $request) { // Handle the request and return the response });
Python REST API with Flask
Flask, a lightweight Python web framework, offers a concise way to build REST APIs. Let’s see an example of defining a route and handling a POST request in Flask:
from flask import Flask, request app = Flask(__name__) @app.route('/users', methods=['POST']) def create_user(): # Handle the request and return the response
Both Laravel and Flask provide a solid foundation for building REST APIs, with Laravel offering a more structured approach and Flask providing simplicity and flexibility.
Database Connection
Establishing a connection to a database is a fundamental step in many applications. In this section, we will compare PHP and Python in terms of connecting to a database.
PHP Database Connection with PDO
PHP’s PDO (PHP Data Objects) extension allows developers to connect to various databases. Here’s an example of establishing a MySQL database connection using PDO:
$pdo = new PDO('mysql:host=localhost;dbname=mydatabase', 'username', 'password');
Python Database Connection with SQLAlchemy
Python’s SQLAlchemy library provides a powerful and flexible way to connect to databases. Let’s see an example of establishing a MySQL database connection using SQLAlchemy:
from sqlalchemy import create_engine engine = create_engine('mysql://username:password@localhost/mydatabase')
Both PHP’s PDO and Python’s SQLAlchemy offer efficient ways to connect to databases, ensuring secure and reliable data access.
User Authentication
User authentication is a critical aspect of many applications. In this section, we will compare PHP and Python in terms of implementing user authentication.
PHP User Authentication with Laravel
Laravel, with its built-in authentication features, simplifies the process of implementing user authentication. Here’s an example of authenticating a user in Laravel:
use Illuminate\Support\Facades\Auth; if (Auth::attempt(['email' => $email, 'password' => $password])) { // Authentication successful } else { // Authentication failed }
Python User Authentication with Django
Django, a powerful Python web framework, provides a robust authentication system. Let’s see an example of authenticating a user in Django:
from django.contrib.auth import authenticate, login user = authenticate(request, username=username, password=password) if user is not None: login(request, user) # Authentication successful else: # Authentication failed
Both Laravel and Django offer comprehensive solutions for user authentication, ensuring secure access to application resources.
Data Visualization
Data visualization is essential for presenting complex data in a meaningful way. In this section, we will compare PHP and Python in terms of data visualization capabilities.
PHP Data Visualization with Chart.js
PHP can leverage JavaScript libraries like Chart.js for data visualization. Here’s an example of creating a bar chart using Chart.js in PHP:
['January', 'February', 'March', 'April', 'May', 'June', 'July'], 'datasets' => [ [ 'label' => 'Sales', 'backgroundColor' => 'rgba(75, 192, 192, 0.2)', 'borderColor' => 'rgba(75, 192, 192, 1)', 'borderWidth' => 1, 'data' => [65, 59, 80, 81, 56, 55, 40], ], ], ]; ?> var ctx = document.getElementById('myChart').getContext('2d'); var chart = new Chart(ctx, { type: 'bar', data: , });
Python Data Visualization with Matplotlib
Python’s Matplotlib library provides a wide range of data visualization capabilities. Let’s see an example of creating a bar chart using Matplotlib in Python:
import matplotlib.pyplot as plt labels = ['January', 'February', 'March', 'April', 'May', 'June', 'July'] data = [65, 59, 80, 81, 56, 55, 40] plt.bar(labels, data) plt.xlabel('Months') plt.ylabel('Sales') plt.title('Sales by Month') plt.show()
Both PHP and Python offer numerous tools and libraries for data visualization, allowing developers to create visually appealing and informative charts.
Web Scraping
Web scraping is a technique used to extract data from websites. In this section, we will compare PHP and Python in terms of web scraping capabilities.
PHP Web Scraping with Guzzle and DOMXPath
PHP can leverage libraries like Guzzle and DOMXPath for web scraping tasks. Here’s an example of scraping data from a website using Guzzle and DOMXPath in PHP:
use GuzzleHttp\Client; use Symfony\Component\DomCrawler\Crawler; $client = new Client(); $response = $client->request('GET', 'https://example.com'); $html = $response->getBody()->getContents(); $crawler = new Crawler($html); $data = $crawler->filter('.class-name')->text();
Python Web Scraping with BeautifulSoup
Python’s BeautifulSoup library provides a convenient way to parse and extract data from HTML. Let’s see an example of scraping data from a website using BeautifulSoup in Python:
import requests from bs4 import BeautifulSoup response = requests.get('https://example.com') html = response.content soup = BeautifulSoup(html, 'html.parser') data = soup.select_one('.class-name').get_text()
Both PHP and Python offer powerful tools for web scraping, enabling developers to extract data from websites effectively.
Error Handling
Proper error handling is crucial for identifying and resolving issues in software applications. In this section, we will compare PHP and Python in terms of error handling techniques.
PHP Error Handling with try-catch
PHP provides try-catch blocks for catching and handling exceptions. Here’s an example of error handling using try-catch in PHP:
try { // Code that may throw an exception } catch (Exception $e) { // Handle the exception }
Python Error Handling with try-except
Python uses try-except blocks for handling exceptions. Let’s see an example of error handling using try-except in Python:
try: # Code that may raise an exception except Exception as e: # Handle the exception
Both PHP and Python offer robust error handling mechanisms, allowing developers to gracefully handle exceptions and ensure the stability of their applications.