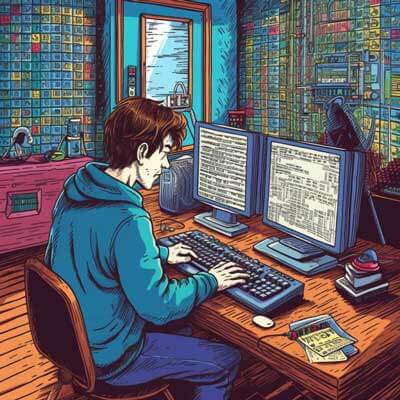
- Introduction to Not Equal Operator
- Syntax of Not Equal Operator
- Use Case 1: Comparing Numbers
- Use Case 2: Comparing Text Strings
- Use Case 3: Comparing Lists
- Use Case 4: Comparing Dictionaries
- Performance Considerations 1: Time Complexity
- Performance Considerations 2: Space Complexity
- Error Handling: Common Mistakes and How to Avoid Them
- Best Practice 1: Using Not Equal Operator in Conditional Statements
- Best Practice 2: Using Not Equal Operator in Loops
- Real World Example 1: Not Equal Operator in Data Filtering
- Real World Example 2: Not Equal Operator in User Input Validation
- Advanced Technique 1: Combining Not Equal with Other Operators
- Advanced Technique 2: Not Equal Operator in Exception Handling
- Code Snippet 1: Not Equal Operator in a Conditional Statement
- Code Snippet 2: Not Equal Operator in a Loop
- Code Snippet 3: Not Equal Operator in a Function
- Code Snippet 4: Not Equal Operator in a Class
- Code Snippet 5: Not Equal Operator in a Data Structure
Introduction to Not Equal Operator
The not equal operator is used in Python to compare two values and determine if they are not equal. It is denoted by the symbol “!=”. This operator returns a Boolean value, either True or False, based on the comparison result.
Related Article: String Comparison in Python: Best Practices and Techniques
Syntax of Not Equal Operator
The syntax of the not equal operator is as follows:
value1 != value2
Here, value1
and value2
can be any Python objects or expressions that can be compared.
Use Case 1: Comparing Numbers
The not equal operator can be used to compare numeric values in Python. Let’s consider a couple of examples:
Example 1: Comparing integers
x = 5 y = 10 if x != y: print("x is not equal to y") else: print("x is equal to y")
Output:
x is not equal to y
Example 2: Comparing floats
a = 3.14 b = 2.718 if a != b: print("a is not equal to b") else: print("a is equal to b")
Output:
a is not equal to b
Use Case 2: Comparing Text Strings
In Python, the not equal operator can also be used to compare text strings. Here are a couple of examples:
Example 1: Comparing strings
name1 = "Alice" name2 = "Bob" if name1 != name2: print("The names are different") else: print("The names are the same")
Output:
The names are different
Example 2: Comparing case-insensitive strings
text1 = "Hello" text2 = "hello" if text1.lower() != text2.lower(): print("The texts are different") else: print("The texts are the same")
Output:
The texts are different
Related Article: How To Limit Floats To Two Decimal Points In Python
Use Case 3: Comparing Lists
The not equal operator can also be used to compare lists in Python. Consider the following examples:
Example 1: Comparing lists
list1 = [1, 2, 3] list2 = [4, 5, 6] if list1 != list2: print("The lists are different") else: print("The lists are the same")
Output:
The lists are different
Example 2: Comparing lists with different lengths
list3 = [1, 2, 3] list4 = [1, 2, 3, 4, 5] if len(list3) != len(list4): print("The lists have different lengths") else: print("The lists have the same length")
Output:
The lists have different lengths
Use Case 4: Comparing Dictionaries
The not equal operator can also be used to compare dictionaries in Python. Let’s see some examples:
Example 1: Comparing dictionaries
dict1 = {"name": "Alice", "age": 25} dict2 = {"name": "Bob", "age": 30} if dict1 != dict2: print("The dictionaries are different") else: print("The dictionaries are the same")
Output:
The dictionaries are different
Example 2: Comparing dictionaries with different keys
dict3 = {"name": "Alice", "age": 25} dict4 = {"name": "Alice", "country": "USA"} if set(dict3.keys()) != set(dict4.keys()): print("The dictionaries have different keys") else: print("The dictionaries have the same keys")
Output:
The dictionaries have different keys
Performance Considerations 1: Time Complexity
When using the not equal operator, the time complexity of the operation depends on the types being compared. In general, the time complexity is O(1) for simple types like numbers and strings. However, for more complex types like lists and dictionaries, the time complexity can be O(n), where n is the size of the data structure.
Related Article: How To Rename A File With Python
Performance Considerations 2: Space Complexity
The space complexity of the not equal operator is constant, regardless of the types being compared. It does not require any additional space to perform the comparison.
Error Handling: Common Mistakes and How to Avoid Them
When using the not equal operator, there are a few common mistakes that programmers may encounter. Here are some examples and how to avoid them:
Mistake 1: Forgetting the double equal sign
x = 5 if x = 10: print("x is equal to 10") else: print("x is not equal to 10")
In this example, the assignment operator “=” is used instead of the not equal operator “!=”. To fix this, ensure that you use the correct operator for comparison.
Mistake 2: Incorrect comparison of different types
x = 5 y = "5" if x != y: print("x is not equal to y") else: print("x is equal to y")
In this example, the not equal operator is used to compare an integer and a string. The comparison will always return True because the types are different. To avoid this, make sure you are comparing values of the same type.
Best Practice 1: Using Not Equal Operator in Conditional Statements
One of the common use cases of the not equal operator is in conditional statements. Here’s an example:
x = 10 if x != 0: print("x is not equal to 0") # Perform some action
In this example, the not equal operator is used to check if the value of x
is not equal to 0. If the condition is true, the code block inside the if statement will be executed.
Related Article: How To Check If List Is Empty In Python
Best Practice 2: Using Not Equal Operator in Loops
The not equal operator can also be used in loop conditions to iterate over a sequence until a certain condition is met. Here’s an example:
numbers = [1, 2, 3, 4, 5] for num in numbers: if num != 3: print(num)
In this example, the loop will iterate over the numbers
list and print each number that is not equal to 3.
Real World Example 1: Not Equal Operator in Data Filtering
The not equal operator is commonly used in data filtering tasks. Let’s say we have a list of students and we want to filter out the students who scored less than 60 in a test. Here’s an example:
students = [ {"name": "Alice", "score": 80}, {"name": "Bob", "score": 55}, {"name": "Charlie", "score": 90} ] # Filter out students with score less than 60 filtered_students = [student for student in students if student["score"] != 60] print(filtered_students)
Output:
[{'name': 'Alice', 'score': 80}, {'name': 'Bob', 'score': 55}, {'name': 'Charlie', 'score': 90}]
In this example, the not equal operator is used to filter out the students who scored less than 60.
Real World Example 2: Not Equal Operator in User Input Validation
The not equal operator can also be used in user input validation to check if the input matches a specific value. Here’s an example:
user_input = input("Enter your favorite color: ") if user_input.lower() != "blue": print("Sorry, your favorite color is not blue.") else: print("Great, blue is a nice color!")
In this example, the not equal operator is used to check if the user’s input is not equal to the string “blue”. If the condition is true, it means the user’s favorite color is not blue.
Related Article: How To Check If a File Exists In Python
Advanced Technique 1: Combining Not Equal with Other Operators
The not equal operator can be combined with other operators to create more complex conditions. Here’s an example:
x = 10 if x > 5 and x != 8: print("x is greater than 5 and not equal to 8")
In this example, the not equal operator is combined with the greater than operator to check if x
is greater than 5 and not equal to 8.
Advanced Technique 2: Not Equal Operator in Exception Handling
The not equal operator can be used in exception handling to catch specific exceptions. Here’s an example:
try: x = 10 / 0 except Exception as e: if type(e) != ZeroDivisionError: raise e else: print("Division by zero error occurred")
In this example, the not equal operator is used to check if the type of the exception is not equal to ZeroDivisionError
. If the condition is true, it means a different type of exception occurred and it is re-raised. Otherwise, the code block inside the else statement will be executed.
Code Snippet 1: Not Equal Operator in a Conditional Statement
x = 5 if x != 0: print("x is not equal to 0")
Related Article: How to Use Inline If Statements for Print in Python
Code Snippet 2: Not Equal Operator in a Loop
numbers = [1, 2, 3, 4, 5] for num in numbers: if num != 3: print(num)
Code Snippet 3: Not Equal Operator in a Function
def is_even(number): return number % 2 != 1
Code Snippet 4: Not Equal Operator in a Class
class Person: def __init__(self, name, age): self.name = name self.age = age person1 = Person("Alice", 25) person2 = Person("Bob", 30) if person1 != person2: print("The persons are different")
Related Article: How to Use Stripchar on a String in Python
Code Snippet 5: Not Equal Operator in a Data Structure
colors = {"red", "green", "blue"} if "yellow" not in colors: print("Yellow is not in the set of colors")