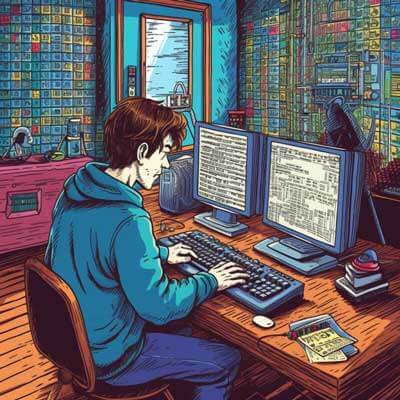
Table of Contents
Next.js Client-Side Rendering
Next.js is a popular JavaScript framework that allows you to build server-rendered React applications. While Next.js is primarily known for its server-side rendering capabilities, it also provides useful client-side rendering functionality.
Client-side rendering refers to the process of rendering a web page on the client's browser using JavaScript, as opposed to rendering it on the server and sending the fully-rendered HTML to the client. This allows for dynamic updates and interactivity without requiring a full page reload.
In Next.js, you can implement client-side rendering by using React components and JavaScript code. Here's an example of a simple Next.js component that performs client-side rendering:
import React from 'react'; const MyComponent = () => { const handleClick = () => { alert('Button clicked!'); }; return ( <div> <h1>Next.js Client-Side Rendering</h1> <button>Click me!</button> </div> ); }; export default MyComponent;
In this example, the handleClick
function is executed on the client's browser when the button is clicked, resulting in an alert being displayed. This demonstrates the ability of Next.js to handle client-side rendering and interactivity.
Related Article: How to Fetch a Parameter Value From a Query String in React
Next.js Client-Side Routing
Routing is an essential aspect of client-side applications, allowing users to navigate between different pages without requiring a full page reload. Next.js provides an intuitive and useful routing system for client-side navigation.
To implement client-side routing in Next.js, you can use the Link
component provided by the next/link
package. Here's an example of how you can use the Link
component to create client-side navigation in Next.js:
import React from 'react'; import Link from 'next/link'; const Navigation = () => { return ( <nav> Home About Contact </nav> ); }; export default Navigation;
In this example, clicking on the links will navigate to the respective pages without requiring a full page reload. This provides a seamless and efficient user experience.
Next.js Client-Side Data Fetching
Next.js allows you to fetch data on the client-side using various techniques. One common approach is to use the useEffect
hook provided by React to fetch data when a component mounts. Here's an example of how you can fetch data on the client-side in Next.js:
import React, { useEffect, useState } from 'react'; const MyComponent = () => { const [data, setData] = useState(null); useEffect(() => { const fetchData = async () => { const response = await fetch('https://api.example.com/data'); const jsonData = await response.json(); setData(jsonData); }; fetchData(); }, []); return ( <div> {data ? ( <ul> {data.map((item) => ( <li>{item.name}</li> ))} </ul> ) : ( <p>Loading...</p> )} </div> ); }; export default MyComponent;
In this example, the fetchData
function is called when the component mounts, and the fetched data is stored in the component's state. The component then renders the data when it becomes available, or displays a loading message while the data is being fetched.
Next.js also provides server-side rendering capabilities, allowing you to fetch data on the server and send it to the client as part of the initial page load. This can be useful for improving performance and SEO.
Next.js Client-Side Navigation
Next.js provides a useful and intuitive API for client-side navigation. You can use the Router
object provided by the next/router
package to programmatically navigate to different pages in your Next.js application.
Here's an example of how you can use the Router
object to navigate to a different page in Next.js:
import { useRouter } from 'next/router'; const MyComponent = () => { const router = useRouter(); const handleClick = () => { router.push('/about'); }; return ( <div> <h1>Next.js Client-Side Navigation</h1> <button>Go to About page</button> </div> ); }; export default MyComponent;
In this example, clicking on the button will navigate to the /about
page using the router.push
method. This allows for seamless client-side navigation without requiring a full page reload.
Related Article: How to Use Closures with JavaScript
Next.js Client-Side Caching
Caching is an important technique for improving the performance and efficiency of client-side applications. Next.js provides built-in support for client-side caching, allowing you to cache data and resources to reduce network requests and improve loading times.
Next.js uses the concept of "SWR" (Stale-While-Revalidate) for client-side caching. SWR is a strategy that first serves the data from the cache (stale), then sends a request to the server (revalidate), and finally comes with the up-to-date data.
Here's an example of how you can use the SWR
hook provided by the swr
package to implement client-side caching in Next.js:
import useSWR from 'swr'; const MyComponent = () => { const { data, error } = useSWR('https://api.example.com/data', fetcher); if (error) return <p>Error fetching data</p>; if (!data) return <p>Loading...</p>; return ( <div> <ul> {data.map((item) => ( <li>{item.name}</li> ))} </ul> </div> ); }; export default MyComponent;
In this example, the useSWR
hook is used to fetch data from the specified URL and cache the response. The fetcher
function is a custom function that handles the data fetching logic.
Next.js also provides support for caching static assets, such as images and stylesheets, using techniques like browser caching and CDN caching. This can help improve the performance and loading times of your Next.js application.
Next.js Client-Side Optimization
Optimizing client-side rendering is crucial for providing a fast and smooth user experience. Next.js provides several optimization techniques to improve the performance of client-side rendering in your application.
Some of the key optimization techniques for client-side rendering in Next.js include:
1. Code Splitting: Next.js automatically splits your JavaScript code into smaller chunks, allowing for faster initial page loads and improved performance. This is achieved using dynamic imports and lazy loading of components.
2. Preloading: Next.js automatically preloads pages that are likely to be visited next by the user. This helps reduce the perceived loading time and improves the overall performance of your application.
3. Image Optimization: Next.js provides built-in image optimization features that automatically optimize and serve images in the most efficient format and size. This helps reduce the bandwidth usage and improves the loading speed of your images.
4. Automatic Static Optimization: Next.js automatically optimizes static pages by prerendering them during the build process. This allows for faster loading times and improved SEO performance.
5. Server-Side Rendering: Next.js allows you to choose between client-side rendering and server-side rendering for different pages in your application. Server-side rendering can provide better performance and SEO benefits for certain pages.
Here's an example of how you can optimize your Next.js application for client-side rendering:
// next.config.js module.exports = { // Enable webpack 5 for improved performance future: { webpack5: true, }, // Enable automatic image optimization images: { domains: ['example.com'], }, };
In this example, the next.config.js
file is used to configure various optimization options for your Next.js application. This includes enabling webpack 5 for improved performance and enabling automatic image optimization.
Next.js Client-Side Development
Next.js provides a comprehensive development environment for client-side development. You can use familiar JavaScript tools and libraries to build and test your Next.js applications.
To set up a development environment for Next.js client-side development, you can follow these steps:
1. Install Node.js: Next.js requires Node.js to run. You can download and install Node.js from the official website.
2. Create a new Next.js project: You can create a new Next.js project using the create-next-app
command-line tool. This tool sets up a basic Next.js project structure for you.
npx create-next-app my-app
3. Start the development server: Once your project is set up, you can start the development server using the following command:
cd my-app npm run dev
4. Start developing: You can now start developing your Next.js application using your favorite code editor. You can write React components, add styles, and implement client-side functionality as needed.
Next.js also provides hot module replacement (HMR) functionality, which allows you to see your changes in real-time without requiring a full page reload. This makes the development process fast and efficient.
Is Next.js a Client-Side Framework?
Next.js is a versatile JavaScript framework that can be used for both client-side and server-side rendering. While Next.js is primarily known for its server-side rendering capabilities, it also provides useful functionality for client-side rendering.
Next.js can be considered a full-stack framework, as it allows you to build complete web applications using React, Node.js, and other JavaScript technologies. It provides a unified development experience for both client-side and server-side rendering, making it a popular choice for building modern web applications.
However, it's worth noting that Next.js is not limited to client-side development. It also provides server-side rendering capabilities, automatic static optimization, and other features that make it suitable for a wide range of use cases.
Related Article: How to Convert a Unix Timestamp to Time in Javascript
What are the Differences Between Client-Side and Server-Side Rendering in Next.js?
Next.js supports both client-side rendering and server-side rendering, each with its own advantages and use cases.
Client-side rendering refers to the process of rendering a web page on the client's browser using JavaScript. In Next.js, client-side rendering allows for dynamic updates and interactivity without requiring a full page reload. It is suitable for applications that require real-time updates and interactive user interfaces.
Server-side rendering, on the other hand, refers to the process of rendering a web page on the server and sending the fully-rendered HTML to the client. In Next.js, server-side rendering provides better performance and SEO benefits, as the fully-rendered HTML is sent to the client as part of the initial page load. It is suitable for applications that require fast loading times and improved search engine visibility.
The main differences between client-side and server-side rendering in Next.js can be summarized as follows:
- Client-side rendering:
- Dynamic updates and interactivity without full page reloads.
- Real-time updates and interactive user interfaces.
- Requires JavaScript support on the client's browser.
- Suitable for applications that require real-time updates and interactivity.
- Server-side rendering:
- Better performance and SEO benefits.
- Faster initial page load times.
- Improved search engine visibility.
- Suitable for applications that require fast loading times and improved search engine visibility.