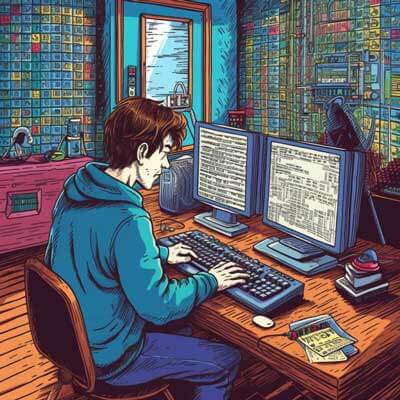
To check if a string is a valid number in JavaScript, you can use various techniques and built-in functions. Here are two possible approaches:
Approach 1: Using the isNaN() Function
One way to check if a string is a valid number is by using the isNaN()
function. The isNaN()
function returns true
if the argument is not a number, and false
if it is a number. Here’s an example:
const str = "1234"; const num = parseInt(str); if (isNaN(num)) { console.log("The string is not a valid number"); } else { console.log("The string is a valid number"); }
In this example, we first parse the string using the parseInt()
function to convert it into a number. Then, we use the isNaN()
function to check if the parsed number is NaN. If it is NaN, we print “The string is not a valid number”; otherwise, we print “The string is a valid number”.
This approach works for most cases, but it has some limitations. For example, it will return true
for empty strings or strings containing only whitespace. If you want to consider these cases as invalid numbers, you can add an additional check for empty or whitespace strings before using parseInt()
.
Related Article: Accessing Parent State from Child Components in Next.js
Approach 2: Using Regular Expressions
Another approach to check if a string is a valid number is by using regular expressions. Regular expressions provide a useful way to match patterns in strings. Here’s an example using a regular expression:
const str = "1234"; const regex = /^[0-9]+$/; if (regex.test(str)) { console.log("The string is a valid number"); } else { console.log("The string is not a valid number"); }
In this example, we define a regular expression pattern that matches any sequence of one or more digits (^[0-9]+$
). We use the test()
method of the regular expression object to check if the string matches the pattern. If it does, we print “The string is a valid number”; otherwise, we print “The string is not a valid number”.
Using regular expressions allows for more flexibility in defining what constitutes a valid number. For example, you can modify the regular expression pattern to allow for decimal numbers, negative numbers, or numbers in scientific notation.
Best Practices
When checking if a string is a valid number in JavaScript, consider the following best practices:
– Be aware of the limitations of the isNaN()
function. It may return false positives for empty or whitespace strings, as well as for certain non-numeric values like Infinity
or null
. Additional checks may be necessary depending on your specific requirements.
– If you need to convert the string to a number, consider using the parseFloat()
or Number()
functions instead of parseInt()
. parseInt()
only parses integers, while parseFloat()
and Number()
can handle decimal numbers as well.
– Regular expressions provide a flexible way to define the pattern of a valid number. However, they can be complex and may have performance implications for large strings or frequent checks. Use regular expressions judiciously and consider their impact on performance.
– If you are working with user input, validate the input on the server-side as well to ensure data integrity and security. Client-side validation can be bypassed, so server-side validation is essential.
Related Article: How to Apply Ngstyle Conditions in Angular