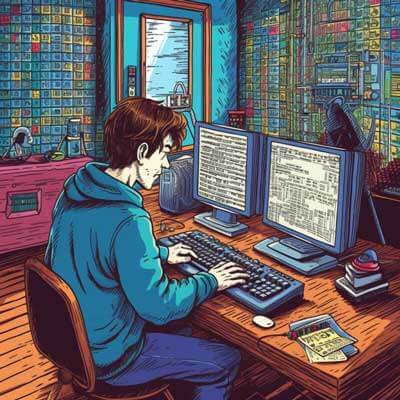
Table of Contents
Rounding numbers to a specific decimal place is a common requirement in many JavaScript applications. Whether you are working on financial calculations, data analytics, or simply formatting numbers for display, rounding to two decimal places is often necessary. In this guide, we will explore different approaches to achieve this in JavaScript.
Method 1: toFixed()
The simplest way to round a number to two decimal places in JavaScript is by using the toFixed()
method. This method returns a string representation of the number with the specified number of decimal places.
Here's an example:
let number = 5.6789; let roundedNumber = number.toFixed(2); console.log(roundedNumber); // Output: 5.68
In the above code, we first declare a variable number
and assign it a value of 5.6789. We then use the toFixed()
method with the argument 2
to round the number to two decimal places. The result is stored in the variable roundedNumber
and printed to the console.
It's important to note that the toFixed()
method returns a string, so if you need to perform further calculations or comparisons with the rounded number, you may need to convert it back to a numeric data type using methods like parseFloat()
or Number()
.
Related Article: How to Play Audio Files Using JavaScript
Method 2: Math.round() and Math.pow()
Another approach to rounding numbers to two decimal places in JavaScript is by using the Math.round()
function in combination with the Math.pow()
function.
Here's an example:
let number = 5.6789; let roundedNumber = Math.round(number * 100) / 100; console.log(roundedNumber); // Output: 5.68
In this code snippet, we first multiply the number by 100 to shift the decimal point two places to the right. Then, we use Math.round()
to round the result to the nearest whole number. Finally, we divide the rounded number by 100 to shift the decimal point back to its original position, effectively rounding it to two decimal places.
This approach gives us more control over the rounding process and can be useful when you need to apply different rounding rules, such as rounding up or down to the nearest two decimal places.
Why is this question asked?
The question of how to round to two decimal places in JavaScript is commonly asked because JavaScript, by default, performs floating-point arithmetic with a precision of up to 15 decimal places. However, in many cases, it is necessary to round numbers to a specific decimal place for various purposes, such as financial calculations or data presentation.
Potential reasons for asking this question include:
1. Financial calculations: When dealing with monetary values, it is often required to round the numbers to two decimal places to ensure accurate calculations and consistent display of currency values.
2. Data formatting: When presenting data to users, it is common to round numbers to a specific decimal place to improve readability and avoid excessive precision that may not be relevant or necessary.
Alternative Ideas and Suggestions
While the methods described above are commonly used to round numbers to two decimal places in JavaScript, there are a few alternative ideas and suggestions worth considering:
1. Custom rounding functions: If you need to apply specific rounding rules, such as round up or round down, you can create custom rounding functions using JavaScript's Math.ceil()
, Math.floor()
, or Math.trunc()
methods in combination with multiplication and division operations.
2. External libraries: If you are working on a project that requires advanced rounding capabilities or additional formatting options, you may consider using external libraries like Numeral.js
or accounting.js
. These libraries provide comprehensive number formatting and rounding options.
Related Article: How To Validate An Email Address In Javascript
Best Practices
When rounding numbers to two decimal places in JavaScript, it is important to keep the following best practices in mind:
1. Consistency: Ensure that you consistently round numbers to two decimal places throughout your application to avoid confusion and inconsistencies in calculations or data presentation.
2. Data types: Be aware of the data types you are working with. If you need to perform further calculations or comparisons with the rounded number, make sure to convert it back to a numeric data type using methods like parseFloat()
or Number()
.
3. Consider precision issues: Due to the nature of floating-point arithmetic, rounding errors can occur when manipulating decimal numbers. Keep in mind that rounding to two decimal places may not always result in exact values, especially when performing multiple arithmetic operations.
4. Test thoroughly: When working with rounding functions or external libraries, thoroughly test your code with different input values to ensure the desired rounding behavior and accuracy.