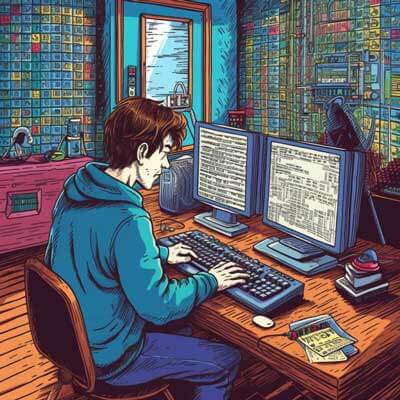
- What are JavaScript closures and why are they important?
- Understanding lexical scope in JavaScript
- How closures work in JavaScript
- The benefits of using closures
- Common use cases for closures in JavaScript
- Data privacy
- Memoization
- Event handlers
- Real world examples of closures in action
- Creating closures in different scenarios
- Creating closures in a function
- Creating closures with event listeners
- Creating closures with asynchronous operations
- Using closures for data privacy
- Closure pitfalls to be aware of
- Accidental closure in loops
- Memory leaks with closures
- Modifying closed-over variables
- Advanced closure techniques
- Immediately Invoked Function Expressions (IIFE)
- Closures in Event Listeners
- Memoization
- Using closures for asynchronous operations
- Closure performance considerations
- Exploring closure alternatives in JavaScript
- Object Factories
- Modules
- Partial Application and Currying
- Understanding closure memory management
What are JavaScript closures and why are they important?
JavaScript closures are an essential concept to understand in order to become proficient in JavaScript programming. They are a powerful feature of the language that allows variables to be accessible outside of their original scope.
In simple terms, a closure is created when an inner function references variables from its outer function, even after the outer function has finished executing. This means that the inner function “closes over” the variables it references, hence the name “closure”.
Closures are important because they enable several powerful programming techniques, such as data privacy and the creation of modules. They allow you to encapsulate variables and functions, preventing them from polluting the global scope.
Let’s take a look at a simple example to understand closures better:
function outerFunction() { let outerVariable = 'I am from the outer function'; function innerFunction() { console.log(outerVariable); } return innerFunction; } const closureExample = outerFunction(); closureExample(); // Output: 'I am from the outer function'
In the example above, the outerFunction
creates an inner function innerFunction
that references the outerVariable
. Even after the outerFunction
finishes executing and the outerVariable
would normally go out of scope, the closure allows innerFunction
to still access and log the value of outerVariable
.
Closures are particularly useful when dealing with asynchronous operations, such as handling events or making AJAX requests. They help in preserving the values of variables that are needed in the callback functions.
Understanding closures is essential for writing clean and efficient JavaScript code. They provide a way to manage state and create self-contained modules, improving code organization and maintainability.
In the next section, we will explore some common use cases and practical examples of closures in JavaScript.
Related Article: How To Generate Random String Characters In Javascript
Understanding lexical scope in JavaScript
Lexical scope is an important concept to understand when working with JavaScript closures. It refers to how variables and functions are resolved at compile time based on the location where they are defined. In simpler terms, lexical scope determines the accessibility of variables and functions in your code.
In JavaScript, lexical scope is defined by the placement of variables and functions in your code. When you declare a variable or a function inside another function, the inner function has access to the variables and functions of the outer function. This is known as the concept of “nested” or “nested” functions.
Let’s take a look at an example to understand lexical scope better:
function outerFunction() { var outerVariable = 'I am an outer variable'; function innerFunction() { console.log(outerVariable); } innerFunction(); } outerFunction();
In this example, we have an outer function called outerFunction
that declares a variable outerVariable
. Inside this outer function, we have an inner function called innerFunction
that logs the value of outerVariable
to the console.
When we invoke outerFunction
, it calls innerFunction
and logs the value of outerVariable
. This works because innerFunction
has access to the variables of its outer function due to lexical scoping.
Lexical scope allows us to create closures in JavaScript. A closure is created when an inner function has access to its outer function’s variables, even after the outer function has finished executing. This is because the inner function maintains a reference to its outer function’s variables in memory.
Let’s see an example of closures in action:
function outerFunction() { var outerVariable = 'I am an outer variable'; return function innerFunction() { console.log(outerVariable); }; } var closure = outerFunction(); closure();
In this example, outerFunction
returns the inner function innerFunction
. We assign the returned function to a variable called closure
. When we invoke closure
, it logs the value of outerVariable
to the console.
The important thing to note here is that even though outerFunction
has already finished executing, the inner function closure
still has access to outerVariable
due to the closure created by lexical scoping.
Understanding lexical scope is crucial when working with closures in JavaScript. It allows you to control the accessibility of variables and functions in your code, and enables the creation of powerful and flexible code structures.
We will explore the concept of closures in more detail and see how they can be used in practical scenarios.
How closures work in JavaScript
Closures are a powerful and fundamental concept in JavaScript. Understanding how closures work is crucial for writing efficient and maintainable code.
A closure is created when a function is defined within another function, and the inner function has access to the outer function’s variables and scope, even after the outer function has finished executing. This means that the inner function “closes over” the variables of the outer function, hence the name “closure”.
To better understand closures, let’s look at an example:
function outerFunction() { let outerVariable = 'I am outside!'; function innerFunction() { console.log(outerVariable); } return innerFunction; } const closure = outerFunction(); closure(); // Output: I am outside!
In this example, the outerFunction
defines a variable called outerVariable
and a nested function called innerFunction
. The innerFunction
has access to the outerVariable
, even after outerFunction
has finished executing.
When outerFunction
is called, it returns innerFunction
, which is then assigned to the variable closure
. When we invoke closure()
, it logs the value of outerVariable
, which is still accessible due to the closure.
Closures are useful in many scenarios. They allow us to create private variables and encapsulate functionality. Let’s see another example:
function counter() { let count = 0; return { increment: function() { count++; }, decrement: function() { count--; }, getCount: function() { return count; } }; } const myCounter = counter(); myCounter.increment(); myCounter.increment(); console.log(myCounter.getCount()); // Output: 2
In this example, the counter
function returns an object with three methods: increment
, decrement
, and getCount
. The count
variable is accessible to all these methods due to the closure. This allows us to maintain a private state within the counter
function and control access to it through the returned object.
Closures can be tricky to understand fully, especially when dealing with asynchronous code and memory management. It’s important to be mindful of memory leaks when using closures, as they can inadvertently keep references to objects and prevent them from being garbage collected.
The benefits of using closures
Closures are a powerful feature in JavaScript that provide numerous benefits. Understanding these benefits can help you write cleaner, more efficient code. We will explore some of the advantages of using closures.
1. Encapsulation and data privacy: Closures allow you to create private variables and functions within a scope. This means that you can encapsulate certain data and functionality, preventing them from being accessed or modified from outside the closure. This is particularly useful when you want to hide implementation details and expose only a limited interface to other parts of your code.
function createCounter() { let count = 0; return { increment() { count++; }, decrement() { count--; }, getCount() { return count; } }; } const counter = createCounter(); counter.increment(); counter.increment(); console.log(counter.getCount()); // Output: 2 console.log(counter.count); // Output: undefined (private variable)
In the example above, the createCounter
function returns an object with three methods: increment
, decrement
, and getCount
. The count
variable is encapsulated within the closure and can only be accessed through these methods. This provides data privacy and prevents direct manipulation of the count
variable.
2. Persistent state: Closures allow functions to retain their state even after they have finished executing. This means that variables inside a closure will still be accessible when the closure is invoked later on. This can be particularly useful when dealing with event handlers or asynchronous operations.
function createTimer() { let seconds = 0; setInterval(() => { seconds++; console.log(`Elapsed time: ${seconds} seconds`); }, 1000); } const timer = createTimer(); // Starts the timer
In this example, the createTimer
function creates a closure that increments the seconds
variable every second using setInterval
. The closure retains the value of seconds
even after the createTimer
function has finished executing. This allows the timer to continue running and displaying the elapsed time.
3. Memory efficiency: Closures can help optimize memory usage by allowing variables and functions to be freed from memory when they are no longer needed. When a closure is created, it maintains references to the variables and functions it needs, even if those variables and functions are no longer accessible from other parts of the code. However, when the closure is no longer accessible itself, these references can be garbage-collected, freeing up memory.
function heavyOperation() { const data = // some large data array return function() { // Perform operations on data }; } const operation = heavyOperation(); // Creates the closure // Perform other operations operation(); // Invoke the closure
In this example, the heavyOperation
function creates a closure that holds a reference to a large data array. Once the closure is invoked and the operation is completed, the closure can be garbage-collected, releasing the memory occupied by the data array. This helps optimize memory usage, especially when dealing with large amounts of data.
These are just a few of the benefits of using closures in JavaScript. They provide a powerful way to encapsulate data, retain state, and optimize memory usage. By understanding and leveraging closures effectively, you can write more robust and efficient code.
Related Article: How to Work with Async Calls in JavaScript
Common use cases for closures in JavaScript
Closures in JavaScript are a powerful feature that allows you to create unique and persistent references to variables. This makes closures extremely useful in a variety of situations. Here are some common use cases for closures in JavaScript:
Data privacy
One of the main benefits of closures is that they allow you to create private variables and functions. By encapsulating data within a closure, you can prevent it from being accessed or modified from outside the closure. This is often referred to as creating a “private” scope.
function counter() { let count = 0; return function() { count++; console.log(count); } } const increment = counter(); increment(); // Output: 1 increment(); // Output: 2
In the example above, the count
variable is only accessible within the counter
function. The inner function returned by counter
forms a closure that retains access to the count
variable even after the counter
function has finished executing. This allows us to increment the count
variable and log its value each time the inner function is invoked, effectively creating a private counter.
Memoization
Closures can also be used for memoization, which is a technique to optimize the performance of functions by caching their results. By caching the results of expensive calculations, you can avoid redundant computations and improve the overall performance of your code.
function memoizedAdd() { const cache = {}; return function(x, y) { const key = `${x}-${y}`; if (cache[key]) { return cache[key]; } const result = x + y; cache[key] = result; return result; } } const add = memoizedAdd(); console.log(add(2, 3)); // Output: 5 console.log(add(2, 3)); // Output: 5 (cached result)
In the example above, the memoizedAdd
function returns an inner function that calculates the sum of two numbers. The results of previous calculations are stored in the cache
object. Before performing a new calculation, the inner function checks if the result for the given input parameters already exists in the cache. If it does, it returns the cached result instead of recomputing it.
Related Article: Understanding JavaScript Execution Context and Hoisting
Event handlers
Closures are commonly used in event handlers to preserve the state of variables. When you attach an event handler to an HTML element, you often need to access the element’s properties or other variables within the event handler. Closures allow you to do this by capturing the variables in the lexical environment at the time the event handler is defined.
function createButton() { const button = document.createElement('button'); button.innerText = 'Click me'; let count = 0; button.addEventListener('click', function() { count++; console.log(`Button clicked ${count} times`); }); return button; } const button = createButton(); document.body.appendChild(button);
In the example above, the createButton
function creates a button element and attaches a click event handler to it. The event handler function increments the count
variable and logs the number of times the button has been clicked. Since the event handler is defined within the createButton
function, it forms a closure that retains access to the count
variable.
These are just a few examples of how closures can be used in JavaScript. Understanding closures and their use cases is essential for writing clean and efficient JavaScript code.
Real world examples of closures in action
Closures are a powerful concept in JavaScript that allows functions to retain access to the variables and scope from where they were defined, even when they are invoked in a different scope. This can be extremely useful in situations where you need to keep track of state or data across multiple function calls.
Let’s explore some real-world examples of closures in action to better understand how they work.
Example 1: Private variables
One common use case for closures is to create private variables in JavaScript. By using a closure, we can encapsulate variables within a function and prevent them from being accessed or modified from outside the function.
function counter() { let count = 0; return function() { count++; console.log(count); } } const increment = counter(); increment(); // Output: 1 increment(); // Output: 2
In this example, the counter
function returns an inner function that has access to the count
variable. Each time the inner function is invoked, it increments the count
variable and logs the updated value to the console.
Since the count
variable is enclosed within the counter
function, it cannot be accessed or modified from outside the function. This provides a way to create private variables in JavaScript.
Example 2: Event listeners
Closures are often used in event handling to maintain access to variables or data within the event callback function. This is especially useful when dealing with asynchronous operations or when handling multiple events.
function createButton() { const button = document.createElement('button'); button.innerText = 'Click me'; button.addEventListener('click', function() { console.log('Button clicked:', button.innerText); }); document.body.appendChild(button); } createButton();
In this example, the createButton
function creates a button element and adds a click event listener to it. The event listener function uses a closure to access the button
variable, even though it is defined outside the scope of the event listener.
This allows us to log the button’s text when it is clicked, without having to define the button
variable globally or pass it as an argument to the event listener function.
Example 3: Memoization
Closures can also be used for memoization, which is a technique to cache the results of expensive function calls and return the cached result when the same inputs occur again.
function memoize(func) { const cache = {}; return function(...args) { const key = JSON.stringify(args); if (cache[key]) { console.log('Fetching result from cache...'); return cache[key]; } console.log('Executing function...'); const result = func(...args); cache[key] = result; return result; } } function fibonacci(n) { if (n <= 1) { return n; } return fibonacci(n - 1) + fibonacci(n - 2); } const memoizedFibonacci = memoize(fibonacci); console.log(memoizedFibonacci(5)); // Output: 5 console.log(memoizedFibonacci(5)); // Output: Fetching result from cache... 5
In this example, the memoize
function takes a function as an argument and returns a memoized version of it. The memoized function uses a closure to store the results of previous function calls in the cache
object.
When the memoized function is called with the same arguments again, it checks if the result is already cached and returns it directly instead of re-executing the function.
These are just a few examples of how closures can be used in real-world scenarios. Understanding closures and how they work is essential for writing clean and efficient JavaScript code.
Creating closures in different scenarios
One of the key features of JavaScript is its ability to create closures. We will explore different scenarios where closures can be created and how they can be used effectively.
Related Article: How to Implement Functional Programming in JavaScript: A Practical Guide
Creating closures in a function
The most common scenario for creating closures is within a function. When a function is defined inside another function, it has access to the variables and parameters of the outer function. The inner function, along with its lexical environment, forms a closure.
Let’s consider the following example:
function outerFunction() { let outerVariable = 'I am from the outer function'; function innerFunction() { console.log(outerVariable); } return innerFunction; } const closure = outerFunction(); closure(); // Output: I am from the outer function
In this example, the innerFunction
has access to the outerVariable
even after the outerFunction
has finished executing. The closure
variable holds a reference to the innerFunction
, which still retains access to the outerVariable
. When we invoke closure()
, it logs the value of outerVariable
to the console.
Creating closures with event listeners
Another common scenario where closures are created is when using event listeners. Event listeners are functions that are executed when a specific event occurs, such as a button click or a keypress.
function createButton() { let count = 0; const button = document.createElement('button'); button.innerText = 'Click me'; button.addEventListener('click', function() { count++; console.log(`Button clicked ${count} times.`); }); return button; } const container = document.getElementById('container'); container.appendChild(createButton());
In this example, when we create a button using the createButton
function, the event listener function inside it creates a closure. The count
variable is accessible within the event listener, even though the createButton
function has already finished executing. Each time the button is clicked, the count
variable is incremented and logged to the console.
Creating closures with asynchronous operations
Closures can also be created with asynchronous operations such as setTimeout or AJAX requests. In these scenarios, closures are often used to maintain state or data between different asynchronous calls.
function fetchData() { let data = null; setTimeout(function() { data = 'Data fetched successfully'; console.log(data); }, 1000); return function() { console.log(data); } } const closure = fetchData(); closure(); // Output: null (immediately) // Output after 1 second: Data fetched successfully
In this example, the closure function returned by fetchData
has access to the data
variable, even though it changes after a delay of 1 second. When we invoke closure()
, it logs the current value of data
, which initially is null
, but after 1 second, it logs the updated value of data
.
Understanding closures and how they are created in different scenarios is crucial for writing clean and efficient JavaScript code. Closures provide a powerful mechanism for encapsulating data and functionality, and they play a significant role in many JavaScript applications.
Related Article: How to Work with Big Data using JavaScript
Using closures for data privacy
Closures in JavaScript have a powerful ability to create data privacy. By using closures, we can encapsulate data within a function and prevent it from being accessed or modified from outside the function. This concept is essential in JavaScript development, as it allows us to control access to our variables and protect them from unintended modifications.
Let’s take a look at an example to understand how closures can be used for data privacy:
function createCounter() { let count = 0; return function() { return ++count; }; } const counter = createCounter(); console.log(counter()); // Output: 1 console.log(counter()); // Output: 2 console.log(counter()); // Output: 3
In the above example, the createCounter
function returns an inner function that has access to the count
variable. The count
variable is defined within the scope of the createCounter
function and is not accessible from the outside. This means that the count
variable is private and cannot be modified directly.
When we invoke the createCounter
function and assign the returned inner function to the counter
variable, we create a closure. The closure retains a reference to the count
variable even after the createCounter
function has finished executing.
Every time we call the counter
function, it increments the count
variable by one and returns the updated value. The count
variable is only accessible through the counter
function, providing data privacy.
By using closures, we can create multiple instances of the counter
function, each with its own private count
variable. This allows us to have independent counters that do not interfere with each other.
Closures are also useful when dealing with asynchronous operations. They can help in maintaining state across multiple function calls without exposing the state to the global scope. This ensures that the data remains private and secure.
Closure pitfalls to be aware of
Understanding closures is crucial for writing clean and efficient JavaScript code. However, there are some common pitfalls that developers may encounter when working with closures. In this section, we will explore these pitfalls and discuss how to avoid them.
Accidental closure in loops
One common mistake that developers make is creating closures inside loops without being aware of the consequences. Consider the following example:
for (var i = 0; i < 5; i++) { setTimeout(function() { console.log(i); }, 1000); }
In this code snippet, we have a loop that sets up a series of timeouts, each logging the value of i
after 1 second. However, when the timeouts actually execute, they will all log the value 5
instead of the expected values 0
, 1
, 2
, 3
, and 4
. This happens because the closures created by the anonymous function inside the loop capture the reference to the same i
variable, which has a value of 5
by the time the timeouts fire.
To fix this issue, we can create a new scope by introducing an immediately-invoked function expression (IIFE) inside the loop. This way, each closure will capture a separate copy of i
:
for (var i = 0; i < 5; i++) { (function(j) { setTimeout(function() { console.log(j); }, 1000); })(i); }
Now, each timeout callback will log the correct value of i
, resulting in the expected output.
Related Article: How to Use Classes in JavaScript
Memory leaks with closures
Closures can also lead to memory leaks if not used carefully. When a closure is created, it maintains a reference to all variables in its lexical scope, including those that are no longer needed. If we’re not mindful of this, it can result in unnecessary memory consumption.
Consider the following example:
function calculateSum() { var data = [1, 2, 3, 4, 5]; var sum = 0; data.forEach(function(num) { sum += num; }); return function() { return sum; }; } var sumClosure = calculateSum();
In this code snippet, the calculateSum
function returns a closure that captures the sum
variable. The closure is assigned to the sumClosure
variable and can be invoked later to retrieve the calculated sum. However, the closure also maintains a reference to the data
array, even after the calculateSum
function has finished executing. This can result in unnecessary memory consumption, especially if the data
array is large.
To avoid memory leaks in such cases, we can manually release unnecessary references. In this example, we can modify the code to nullify the data
variable after it’s no longer needed:
function calculateSum() { var data = [1, 2, 3, 4, 5]; var sum = 0; data.forEach(function(num) { sum += num; }); data = null; // Release the reference to data return function() { return sum; }; } var sumClosure = calculateSum();
By nullifying the data
variable, we ensure that the closure only maintains a reference to the necessary variables, preventing memory leaks.
Modifying closed-over variables
Another potential pitfall is modifying closed-over variables. Closures are designed to capture a snapshot of the variables at the time of their creation. Modifying closed-over variables can lead to unexpected behavior and bugs.
Consider the following example:
function createCounter() { var count = 0; return function() { return ++count; }; } var counter = createCounter(); console.log(counter()); // Output: 1 console.log(counter()); // Output: 2 counter.count = 10; // Modifying closed-over variable (avoid this) console.log(counter()); // Output: 11
In this code snippet, we create a closure that increments a count
variable each time it’s invoked. However, if we mistakenly try to modify the count
variable directly on the closure object (counter.count = 10
), it won’t affect the closed-over count
variable. Instead, it will create a new property on the closure object called count
. As a result, subsequent invocations of the closure will continue to increment the original closed-over count
variable.
To avoid such issues, it’s important to understand that closures are read-only with respect to their closed-over variables. If you need to modify a closed-over variable, you should provide an explicit setter function or use other appropriate mechanisms.
Understanding and being aware of these closure pitfalls will help you write more robust and bug-free JavaScript code. By avoiding accidental closures in loops, handling memory leaks, and refraining from modifying closed-over variables, you can effectively harness the power of closures in your applications.
Advanced closure techniques
We learned about the basic concept of closures in JavaScript and how they work. Now, let’s dive into some advanced closure techniques that can be useful in more complex scenarios.
Related Article: JavaScript Spread and Rest Operators Explained
Immediately Invoked Function Expressions (IIFE)
One common use case for closures is to create private variables and functions. In JavaScript, there is no built-in support for private members, but closures can help us achieve that effect. One way to create private members is by using an Immediately Invoked Function Expression (IIFE).
An IIFE is a function that is immediately executed after it is defined. It is often used to create a new scope and prevent variable names from polluting the global scope. By using an IIFE, we can encapsulate variables and functions inside the closure, making them inaccessible from outside.
Here’s an example of how an IIFE can be used to create private variables:
var counter = (function() { var count = 0; return { increment: function() { count++; }, decrement: function() { count--; }, getCount: function() { return count; } }; })(); console.log(counter.getCount()); // Output: 0 counter.increment(); console.log(counter.getCount()); // Output: 1 counter.decrement(); console.log(counter.getCount()); // Output: 0
In this example, the count
variable is enclosed within the IIFE, making it inaccessible from outside. The returned object contains three methods: increment
, decrement
, and getCount
, which can access the private count
variable.
Closures in Event Listeners
Another common use case for closures is in event listeners. When attaching an event listener to an element, the callback function forms a closure. This allows the callback function to access variables from the outer scope, even after the outer function has finished executing.
Here’s an example of how closures can be used in event listeners:
function createButton() { var button = document.createElement("button"); var count = 0; button.innerText = "Click me"; button.addEventListener("click", function() { count++; console.log("Button clicked " + count + " times"); }); return button; } var button = createButton(); document.body.appendChild(button);
In this example, the click
event listener callback function forms a closure that has access to the count
variable. Every time the button is clicked, the callback function increments the count
variable and logs the updated count to the console.
Memoization
Memoization is a technique used to optimize function execution by caching the results of expensive function calls. Closures can be used to implement memoization in JavaScript.
Here’s an example of how memoization can be implemented using closures:
function memoize(func) { var cache = {}; return function(...args) { var key = JSON.stringify(args); if (cache[key]) { return cache[key]; } var result = func(...args); cache[key] = result; return result; }; } function fibonacci(n) { if (n < 2) { return n; } return fibonacci(n - 1) + fibonacci(n - 2); } var memoizedFibonacci = memoize(fibonacci); console.log(memoizedFibonacci(5)); // Output: 5 console.log(memoizedFibonacci(10)); // Output: 55 console.log(memoizedFibonacci(15)); // Output: 610
In this example, the memoize
function takes another function as an argument and returns a memoized version of that function. The memoized function uses a closure to store the results of previous function calls in the cache
object. If the function is called with the same arguments again, it checks the cache first and returns the cached result instead of recomputing it.
These are just a few examples of advanced closure techniques in JavaScript. Closures are a powerful concept that can be used in various ways to solve complex problems and improve code efficiency.
Related Article: Javascript Template Literals: A String Interpolation Guide
Using closures for asynchronous operations
JavaScript closures are not only useful for encapsulating data and creating private variables, but they are also powerful tools for handling asynchronous operations. In this section, we will explore how closures can be used to manage asynchronous tasks in JavaScript.
When working with asynchronous operations, such as making API requests or fetching data from a database, it is common to encounter situations where you need to handle the result of the operation once it completes. Closures can help you manage this by capturing the state of variables at the time the asynchronous operation is initiated.
Let’s consider an example where we want to fetch data from an API and perform some actions once the data is received. We can use closures to achieve this:
function fetchData(url) { return new Promise((resolve, reject) => { // Simulating an asynchronous API request setTimeout(() => { const data = { name: "John", age: 30 }; resolve(data); }, 2000); }); } function processData() { // Variable defined outside the closure let processedData = null; fetchData("https://api.example.com/data") .then((data) => { processedData = data; // Closure captures the state of processedData console.log("Data processed:", processedData); }) .catch((error) => { console.error("Error:", error); }); } processData();
In the code snippet above, we have two functions: fetchData
and processData
. The fetchData
function simulates an asynchronous API request using a setTimeout
function. It returns a promise that resolves with the fetched data after a delay of 2000 milliseconds.
The processData
function calls the fetchData
function and handles the resolved data inside a closure. The variable processedData
is declared outside the closure and is initially set to null
. Once the data is received from the API, it is assigned to the processedData
variable inside the closure. The closure captures the state of processedData
at the time the asynchronous operation is initiated.
By using closures, we can access and manipulate the processedData
variable even after the asynchronous operation has completed. This allows us to perform further actions or computations with the data once it is available.
Closures are particularly useful when dealing with multiple asynchronous operations that depend on each other. By capturing the state of variables within closures, you can ensure that the correct data is available at each step of the asynchronous process.
Closure performance considerations
When working with JavaScript closures, it’s important to consider their impact on performance. While closures provide powerful functionality, they can also introduce potential performance bottlenecks if not used carefully. In this section, we will explore some performance considerations to keep in mind when working with closures.
1.Memory usage
Closures can consume a significant amount of memory, especially when they hold references to large objects or variables. Each closure retains a reference to its outer function’s variables and scope chain, which can result in memory leaks if not managed properly.
To optimize memory usage, it’s important to minimize the size of closures and avoid unnecessary references. Ensure that closures only hold references to the variables they actually need, and avoid creating closures inside loops or frequently called functions.
Here’s an example that demonstrates how closures can lead to memory leaks:
function createCounter() { let count = 0; return function increment() { count++; console.log(count); }; } const incrementCounter = createCounter();
In this example, the incrementCounter
function captures the count
variable, creating a closure that holds a reference to it. If incrementCounter
is called multiple times, the count
variable will remain in memory, even if it’s no longer needed.
To prevent memory leaks, make sure to release closures when they are no longer needed. This can be done by setting variables to null
or using techniques like object pooling.
2.Performance overhead
Closures introduce an additional layer of indirection, which can result in a performance overhead compared to regular function calls. Each time a closure is invoked, JavaScript needs to resolve its scope chain to access the variables it references.
While this overhead is usually negligible, it can become noticeable in performance-critical scenarios. If you find that closures are impacting the performance of your code, consider alternative approaches like using plain functions, memoization, or optimizing the closure usage.
3.Garbage collection
Closures can also affect garbage collection in JavaScript. If closures capture variables that are no longer needed, they can prevent those variables from being garbage-collected, leading to increased memory usage.
To avoid potential memory leaks, be mindful of the variables captured by closures and ensure they are released when no longer needed. Additionally, consider using techniques like weak references or event-based patterns to minimize the impact on garbage collection.
Exploring closure alternatives in JavaScript
While closures are a powerful and commonly used feature in JavaScript, there are alternative ways to achieve similar functionality in certain scenarios. In this section, we will explore some of these alternatives and discuss when they might be more appropriate.
Related Article: High Performance JavaScript with Generators and Iterators
Object Factories
One way to avoid using closures is by using object factories. An object factory is a function that creates and returns a new object with its own properties and methods. This allows us to encapsulate data and behavior within each instance of the object.
Here’s an example:
function createPerson(name) { return { getName: function() { return name; }, setName: function(newName) { name = newName; } }; } const person = createPerson('John'); console.log(person.getName()); // Output: John person.setName('Jane'); console.log(person.getName()); // Output: Jane
In the above code, the createPerson
function acts as an object factory that creates a new person object with its own name
property. The object also has getter and setter methods to access and modify the name
property.
Modules
Another alternative to closures is using modules. Modules provide a way to encapsulate private data and expose a public interface for interacting with that data.
Here’s an example:
const counterModule = (function() { let count = 0; function increment() { count++; } function decrement() { count--; } function getCount() { return count; } return { increment, decrement, getCount }; })(); console.log(counterModule.getCount()); // Output: 0 counterModule.increment(); console.log(counterModule.getCount()); // Output: 1
In the above code, we define a module using an immediately invoked function expression (IIFE). The module has private data count
and three public methods: increment
, decrement
, and getCount
. The private data is encapsulated within the module and can only be accessed or modified through the public methods.
Partial Application and Currying
Partial application and currying are techniques that allow us to create specialized functions from existing functions by fixing some of the arguments. This can be useful when we want to create reusable functions with predefined behavior.
Here’s an example using partial application:
function multiply(a, b) { return a * b; } const double = multiply.bind(null, 2); console.log(double(5)); // Output: 10
In the above code, we use the bind
method to create a new function double
from the multiply
function. The first argument to bind
is the value of this
(which we don’t need in this case) and the following arguments are the fixed arguments for the new function. The resulting function double
multiplies any given number by 2.
These closure alternatives can be useful in different scenarios, depending on the specific requirements of your application. Understanding closures and their alternatives will help you write more efficient and maintainable JavaScript code.
Related Article: JavaScript Arrow Functions Explained (with examples)
Understanding closure memory management
In JavaScript, closures are a powerful feature that allows functions to retain access to variables from their parent scope even after the parent function has finished executing. This can be extremely useful, but it also introduces some considerations for memory management.
When a function creates a closure, it holds on to a reference of the variables it needs from its parent scope. This means that the variables will not be garbage collected by the JavaScript engine as long as the closure exists. It’s important to be aware of this behavior to avoid potential memory leaks.
Let’s take a look at an example:
function outerFunction() { var outerVariable = 'I am from outer function'; function innerFunction() { console.log(outerVariable); } return innerFunction; } var closure = outerFunction(); closure(); // Output: I am from outer function
In this example, innerFunction
creates a closure that retains a reference to outerVariable
. Even though outerFunction
has finished executing, outerVariable
is still accessible through the closure created by innerFunction
.
Now, let’s consider a scenario where closures can cause memory leaks if not handled properly:
function createEventListener() { var element = document.getElementById('myButton'); element.addEventListener('click', function() { console.log('Button clicked'); }); } createEventListener();
In this example, the event listener function is created within the createEventListener
function. The closure created by the event listener function retains a reference to the element
variable, preventing it from being garbage collected.
If the createEventListener
function is called multiple times, each time creating a new event listener, we will end up with multiple closures retaining references to their respective element
variables. This can lead to a significant memory consumption if not handled properly.
To mitigate this, we can explicitly remove event listeners or nullify any references to variables that are no longer needed. For example:
function createEventListener() { var element = document.getElementById('myButton'); function handleClick() { console.log('Button clicked'); element.removeEventListener('click', handleClick); element = null; } element.addEventListener('click', handleClick); }
In this modified example, the event listener function handleClick
removes itself as an event listener and nullifies the reference to element
once it’s no longer needed. This ensures that the closure and the associated variables can be garbage collected.
Understanding closure memory management is crucial to prevent memory leaks in JavaScript applications. By being mindful of the variables retained by closures, and properly cleaning up references when they are no longer needed, we can ensure efficient memory usage in our code.