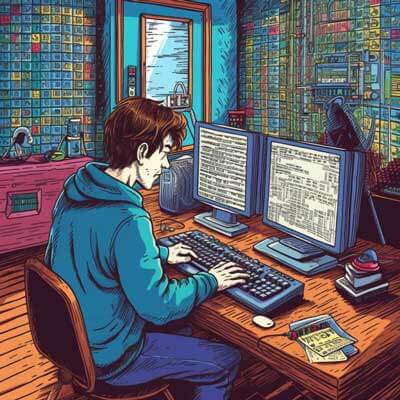
- What is React?
- What is a functional component in JavaScript?
- How do you define state in a functional component?
- What are props in React?
- What is JSX?
- What is functional programming and how does it relate to JavaScript?
- What is the role of UI in web development?
- How does rendering work in React?
- What is the virtual DOM in React?
- How do you handle events in JavaScript?
- Code Snippet: Grouping Functional Components in JavaScript
- Code Snippet: Defining State in a Functional Component
- Code Snippet: Using Props in React
- Code Snippet: Rendering JSX in React
- Code Snippet: Handling Events in JavaScript
What is React?
React is a JavaScript library that is used for building user interfaces. It was developed by Facebook and has gained immense popularity due to its efficiency and reusability. React allows developers to create reusable UI components and efficiently update the UI when the underlying data changes. One of the key features of React is its virtual DOM, which allows for efficient rendering of components.
Related Article: 25 Handy Javascript Code Snippets for Everyday Use
What is a functional component in JavaScript?
In React, a functional component is a JavaScript function that returns JSX (JavaScript XML) to define the structure and content of the component. Functional components are a simpler and more lightweight alternative to class components and are widely used in React applications. They are typically used for presenting static content or for components that don’t require state or lifecycle methods.
Here’s an example of a functional component in React:
function Welcome(props) { return <h1>Hello, {props.name}!</h1>; }
In the above example, the Welcome
component takes in a props
object as an argument and returns a JSX element that displays a greeting message with the value of the name
prop.
How do you define state in a functional component?
In React, state is a JavaScript object that represents the data that a component needs to keep track of. While functional components don’t have a built-in way to define state, React provides the useState
hook that allows functional components to manage state.
The useState
hook takes an initial value as an argument and returns an array with two elements: the current state value and a function to update the state. By calling the update function, React will re-render the component with the new state value.
Here’s an example of how to use the useState
hook in a functional component:
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button> setCount(count + 1)}>Increment</button> </div> ); }
In the above example, the Counter
component uses the useState
hook to define a count
state variable with an initial value of 0. The setCount
function is used to update the count
state when the button is clicked.
What are props in React?
Props (short for properties) are a way to pass data to React components. They are read-only and cannot be modified within the component. Props are typically used to pass data from a parent component to a child component.
In the parent component, you can pass props to a child component by adding attributes to the JSX element when rendering the child component. The child component can then access the props through the props
object.
Here’s an example of how to pass and access props in React:
function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } function App() { return ; }
In the above example, the Greeting
component receives the name
prop and displays a greeting message with the value of the name
prop. The App
component renders the Greeting
component and passes the name
prop with a value of “John”.
Related Article: Accessing Parent State from Child Components in Next.js
What is JSX?
JSX (JavaScript XML) is an extension to JavaScript syntax that allows you to write HTML-like code in your JavaScript files. It is a syntax extension provided by React and is used to define the structure and content of React components.
JSX looks similar to HTML, but it is not valid JavaScript. However, it can be transformed into valid JavaScript using a transpiler like Babel. JSX allows you to write expressive and declarative code, making it easier to understand and maintain your React components.
Here’s an example of JSX code:
function Welcome(props) { return <h1>Hello, {props.name}!</h1>; }
In the above example, the Welcome
component returns a JSX element that displays a greeting message with the value of the name
prop.
What is functional programming and how does it relate to JavaScript?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions. It emphasizes the use of pure functions, immutability, and avoiding shared state and mutable data.
JavaScript is a multi-paradigm language that supports functional programming along with object-oriented programming and procedural programming. JavaScript provides several features that are commonly used in functional programming, such as first-class functions, higher-order functions, and immutable data structures.
Functional programming in JavaScript involves writing functions that take in arguments and return a value without modifying any external state. This can lead to code that is easier to reason about, test, and maintain.
Here’s an example of a higher-order function in JavaScript:
function multiplyBy(factor) { return function(number) { return number * factor; }; } const double = multiplyBy(2); console.log(double(5)); // Output: 10
In the above example, the multiplyBy
function is a higher-order function that takes a factor
argument and returns a new function that multiplies its argument by the factor
value. The double
constant is assigned the returned function with a factor
value of 2, and when it is called with an argument of 5, it returns the result of multiplying 5 by 2, which is 10.
What is the role of UI in web development?
The user interface (UI) in web development refers to the visual elements and interactions that users see and interact with on a website or web application. The UI plays a crucial role in delivering a positive user experience and ensuring that users can effectively and efficiently interact with the website or application.
The role of UI in web development includes:
– Presenting information: The UI is responsible for displaying the relevant information to the user in a clear and organized manner. This includes text, images, videos, and other media.
– Interactions: The UI allows users to interact with the website or application through various input methods, such as clicking buttons, filling out forms, and navigating through menus. The UI should provide intuitive and responsive interactions to enhance the user experience.
– Navigation: The UI provides navigation elements, such as menus, links, and buttons, to help users navigate through different sections and pages of the website or application. A well-designed navigation system can make it easier for users to find the information they need.
– Visual appeal: The UI is responsible for the visual design of the website or application. It involves choosing colors, typography, layouts, and other visual elements to create an aesthetically pleasing and cohesive user interface.
Related Article: Advanced DB Queries with Nodejs, Sequelize & Knex.js
How does rendering work in React?
In React, rendering refers to the process of updating the UI to reflect changes in the underlying data. When a component’s state or props change, React re-renders the component and updates the DOM to reflect the new state or prop values.
React uses a diffing algorithm to efficiently update only the parts of the DOM that have changed. This approach, known as the virtual DOM, allows React to minimize the number of updates required and improve the performance of the application.
The rendering process in React can be summarized as follows:
1. React determines if a component needs to be re-rendered by comparing the current state and props with the previous state and props.
2. If the state or props have changed, React generates a new virtual DOM representation of the component and its children.
3. React then compares the new virtual DOM with the previous virtual DOM to identify the differences (or “diffs”).
4. React applies the diffs to the actual DOM, updating only the parts that have changed.
5. Finally, React triggers any necessary lifecycle methods or effects to handle the updated component.
This efficient rendering process allows React to provide a smooth and responsive user interface even in complex applications with frequent data updates.
What is the virtual DOM in React?
The virtual DOM in React is a lightweight and efficient representation of the actual DOM (Document Object Model). It is a JavaScript object tree that mirrors the structure of the actual DOM elements.
When a component’s state or props change, React generates a new virtual DOM representation of the component and its children. React then compares this new virtual DOM with the previous virtual DOM to identify the differences (or “diffs”).
Once the differences have been identified, React applies the changes to the actual DOM, updating only the necessary elements and attributes. This efficient diffing and updating process helps improve the performance of React applications and provides a smooth user interface.
How do you handle events in JavaScript?
In JavaScript, events are actions or occurrences that happen in the browser, such as a button click, mouse movement, or keyboard input. Handling events involves writing code that responds to these events and performs the desired actions or logic.
There are several ways to handle events in JavaScript, including:
1. Inline event handlers: You can add event handlers directly to HTML elements by using the on
prefix followed by the event name as an attribute. For example, to handle a button click event, you can use the onclick
attribute:
<button>Click me</button>
2. DOM event listeners: You can use the addEventListener
method to attach event listeners to HTML elements. This method allows you to add multiple event listeners to the same element and provides more flexibility compared to inline event handlers. Here’s an example of adding a click event listener to a button element:
const button = document.querySelector('button'); button.addEventListener('click', () => { alert('Button clicked!'); });
3. Event delegation: Event delegation is a technique where you attach a single event listener to a parent element and handle events that occur on its child elements. This approach is useful when you have dynamically created or updated elements. Here’s an example of event delegation for handling click events on dynamically created buttons:
const parentElement = document.querySelector('#parent'); parentElement.addEventListener('click', (event) => { if (event.target.tagName === 'BUTTON') { alert('Button clicked!'); } });
4. Framework-specific event handling: If you are using a JavaScript framework like React or Angular, they provide their own event handling mechanisms. For example, in React, you can use the onClick
prop to specify a function to be called when a button is clicked.
These are just a few examples of how events can be handled in JavaScript. The approach you choose depends on your specific use case and the requirements of your application.
Related Article: Advanced Node.js: Event Loop, Async, Buffer, Stream & More
Code Snippet: Grouping Functional Components in JavaScript
import React from 'react'; function Header() { return <h1>My App</h1>; } function Sidebar() { return ( <ul> <li>Home</li> <li>About</li> <li>Contact</li> </ul> ); } function Content() { return <p>Welcome to my app!</p>; } function App() { return ( <div> <Header /> <div> </div> </div> ); } export default App;
In the above code snippet, we have three functional components: Header
, Sidebar
, and Content
. The App
component is the parent component that groups these components together.
The Header
component renders a heading element with the text “My App”. The Sidebar
component renders an unordered list with three list items. The Content
component renders a paragraph element with the text “Welcome to my app!”.
The App
component renders the Header
, Sidebar
, and Content
components, grouping them together within a div
element.
Code Snippet: Defining State in a Functional Component
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; const decrement = () => { setCount(count - 1); }; return ( <div> <p>Count: {count}</p> <button>Increment</button> <button>Decrement</button> </div> ); } export default Counter;
In the above code snippet, the Counter
component uses the useState
hook to define a count
state variable with an initial value of 0. The setCount
function is used to update the count
state.
The increment
function is called when the “Increment” button is clicked, and it updates the count
state by incrementing it by 1. The decrement
function is called when the “Decrement” button is clicked, and it updates the count
state by decrementing it by 1.
The current value of the count
state is displayed in a paragraph element, and the “Increment” and “Decrement” buttons trigger the respective functions when clicked.
Code Snippet: Using Props in React
import React from 'react'; function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } function App() { return ; }
In the above code snippet, we have two components: Greeting
and App
. The Greeting
component receives the name
prop and displays a greeting message with the value of the name
prop.
The App
component renders the Greeting
component and passes the name
prop with a value of “John”. When the Greeting
component is rendered, it will display the message “Hello, John!”.
Props allow data to be passed from a parent component to a child component, enabling the reusability of components and making it easy to customize their behavior or content.
Related Article: AI Implementations in Node.js with TensorFlow.js and NLP
Code Snippet: Rendering JSX in React
import React from 'react'; function App() { const name = 'John'; const age = 30; return ( <div> <h1>Welcome to my app</h1> <p>Name: {name}</p> <p>Age: {age}</p> </div> ); } export default App;
In the above code snippet, the App
component renders JSX elements to define the structure and content of the component. The JSX elements include heading and paragraph elements, as well as interpolated variables (name
and age
) using curly braces {}
.
When the App
component is rendered, it will display the heading “Welcome to my app”, followed by two paragraphs showing the values of the name
and age
variables.
JSX allows for the declarative and expressive definition of the UI in React components, making it easier to understand and maintain the code.
Code Snippet: Handling Events in JavaScript
const button = document.querySelector('button'); button.addEventListener('click', () => { alert('Button clicked!'); });
In the above code snippet, we have a button element selected using the querySelector
method. An event listener is added to the button using the addEventListener
method. The event listener listens for the ‘click’ event and calls an anonymous function that displays an alert with the message “Button clicked!” when the button is clicked.
This is a basic example of handling a click event in JavaScript. You can replace the alert
function with any custom logic or action you want to perform in response to the event.