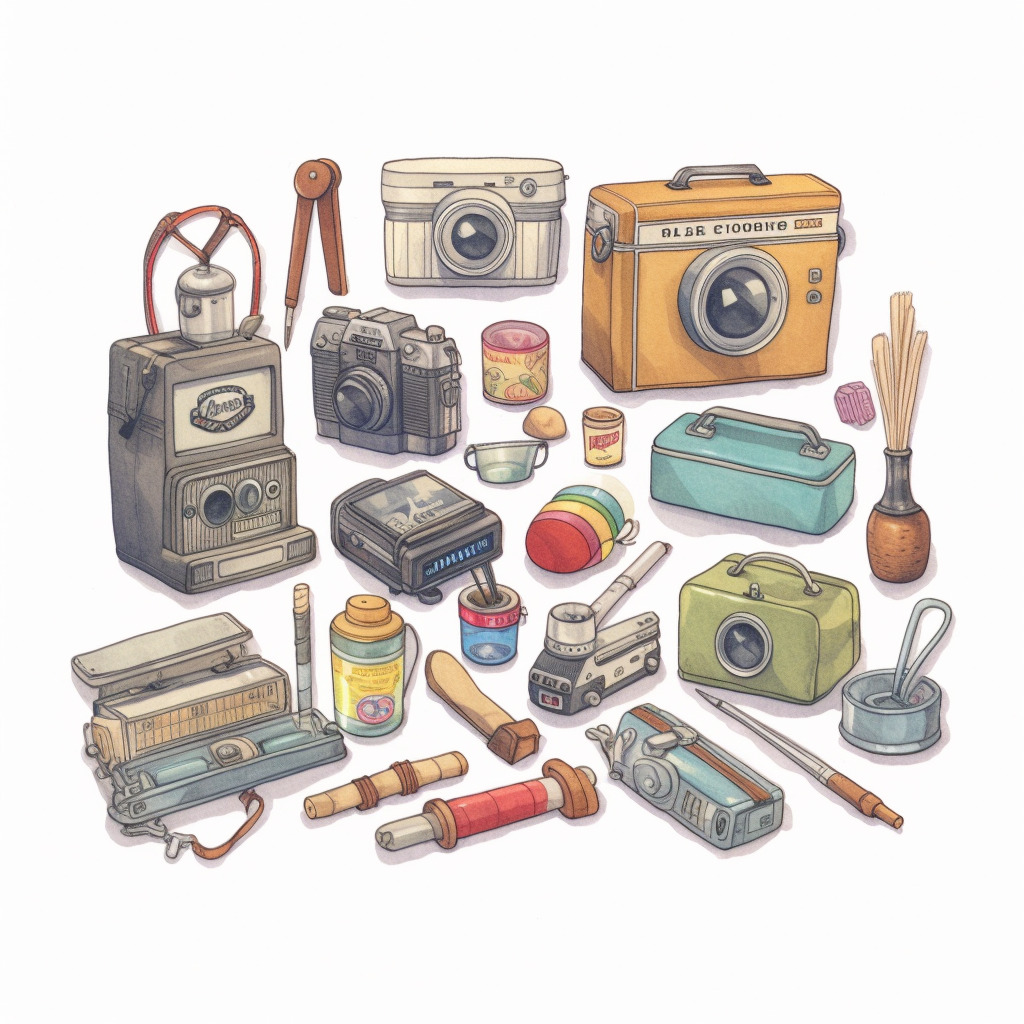
- JavaScript Objects: An Introduction
- What is an Object?
- Accessing Object Properties
- Modifying Object Properties
- Adding and Removing Object Properties
- Object Methods: Defining and Invoking
- Differences between Functions and Object Methods
- Iterating over Object Properties
- Creating Objects: Object Literals
- Creating Objects: Constructor Functions
- Property Descriptors
- Accessing Object Properties: Dot Notation vs Bracket Notation
- Dot Notation
- Bracket Notation
- When to Use Each Notation
- The ‘this’ Keyword: Context and Scope
- Context and Scope
- Function Invocation Patterns
- Arrow Functions and ‘this’
- Prototype Inheritance
- Prototype Inheritance: Object.create()
- Prototype Inheritance: Object.setPrototypeOf()
- Prototype Inheritance: Class Syntax
- Understanding Object Cloning
- Deep Cloning vs Shallow Cloning
- Object Destructuring: Unpacking Values
- Object Spread: Merging Objects
- Object Composition: Combining Objects
- Understanding Object Serialization
- JSON Serialization
- Deserialization
- Limitations of JSON Serialization
- Other Serialization Methods
- JSON.stringify() and JSON.parse()
- Working with Nested Objects
- Object Equality: Comparing Objects
- Object.freeze() and Object.seal()
- Object.freeze()
- Object.seal()
- Garbage Collection: Memory Management
- Getters and Setters: Accessing and Modifying Properties
- Getters
- Setters
- Using Getters and Setters
- Real World Examples: Creating a To-Do List App
- Real World Examples: Building a User Authentication System
- Real World Examples: Implementing a Shopping Cart
- Real World Examples: Creating an Address Book
- Advanced Techniques: Object Proxy
- Advanced Techniques: Object Reflection
JavaScript Objects: An Introduction
JavaScript is a versatile programming language that allows you to create complex data structures. One of the fundamental data types in JavaScript is the object. Understanding JavaScript objects is crucial for building scalable and maintainable applications.
Related Article: How To Merge Arrays And Remove Duplicates In Javascript
What is an Object?
An object is a collection of key-value pairs, where each value can be of any data type, including other objects. Objects are used to represent real-world entities and their properties. For example, you can use an object to represent a person with properties like name, age, and address.
Here’s an example of a simple JavaScript object representing a person:
const person = { name: 'John Doe', age: 30, address: '123 Main St' };
In this example, person
is an object with three properties: name
, age
, and address
. The values associated with these properties are a string, a number, and another string, respectively.
Accessing Object Properties
Properties are the characteristics or attributes of an object. They define the object’s state or data. Each property consists of a key-value pair, where the key is a string (or symbol in ES6) and the value can be any valid JavaScript value.
To access an object’s property, you can use dot notation or bracket notation. Dot notation is more commonly used when the property name is a valid identifier, whereas bracket notation is used when the property name contains special characters or spaces.
console.log(person.name); // Output: John Doe console.log(person.age); // Output: 30 console.log(person.address); // Output: 123 Main St
In the above example, dot notation is used to access the name
, age
, and address
properties of the person
object.
Alternatively, you can also use bracket notation to access object properties. This is especially useful when the property name contains special characters or when using a variable to dynamically access the property.
console.log(person['name']); // Output: John Doe console.log(person['age']); // Output: 30 console.log(person['address']); // Output: 123 Main St const propertyName = 'name'; console.log(person[propertyName]); // Output: John Doe
Modifying Object Properties
You can modify the values of object properties by directly assigning new values to them.
person.name = 'Jane Smith'; person.age = 35; person.address = '456 Elm St'; console.log(person.name); // Output: Jane Smith console.log(person.age); // Output: 35 console.log(person.address); // Output: 456 Elm St
In this example, the values of the name
, age
, and address
properties of the person
object are modified.
Related Article: JavaScript HashMap: A Complete Guide
Adding and Removing Object Properties
You can add new properties to an object by simply assigning a value to a new property name.
person.email = 'jane@example.com'; console.log(person.email); // Output: jane@example.com
In this example, the email
property is added to the person
object.
To remove a property from an object, you can use the delete
keyword.
delete person.address; console.log(person.address); // Output: undefined
In this example, the address
property is removed from the person
object using the delete
keyword.
Object Methods: Defining and Invoking
Objects can have methods, which are functions that are associated with an object. These methods can be used to perform actions or manipulate data within the object. In this chapter, we will learn how to define and invoke object methods.
To define a method for an object, we simply assign a function to a property of the object. Let’s consider an example where we have an object called “person” with properties like “name” and “age”. We can define a method called “greet” that will log a greeting message to the console:
let person = { name: "John", age: 30, greet: function() { console.log("Hello, my name is " + this.name + " and I am " + this.age + " years old."); } };
In the above code snippet, we defined the “greet” method as a function within the “person” object. Inside the method, we can access the object’s properties using the this
keyword.
To invoke a method, we can simply use dot notation to access the method and call it using parentheses. Continuing with our previous example, we can invoke the “greet” method as follows:
person.greet();
When we invoke the “greet” method, it will log the greeting message to the console:
Hello, my name is John and I am 30 years old.
Invoking an object method executes the code within the method. The method can access the object’s properties and perform any necessary operations.
Object methods can also accept arguments, just like regular functions. These arguments can be used within the method to perform specific actions or calculations. Let’s modify our “person” object to include a method called “calculateBirthYear” that accepts the current year as an argument:
let person = { name: "John", age: 30, calculateBirthYear: function(currentYear) { return currentYear - this.age; } };
In the above code snippet, the “calculateBirthYear” method subtracts the person’s age from the current year to calculate their birth year. The result is returned by the method.
To invoke a method with arguments, we pass the arguments inside the parentheses when calling the method. For example, to calculate the birth year of our person using the current year of 2022, we can do the following:
let currentYear = 2022; let birthYear = person.calculateBirthYear(currentYear); console.log("The person was born in " + birthYear + ".");
This will output:
The person was born in 1992.
Differences between Functions and Object Methods
While both functions and object methods can perform actions, there are some key differences between them:
– Functions are standalone entities, while object methods are tied to a specific object. Object methods can access the object’s properties using the this
keyword.
– Object methods can be called using the dot notation on the object, while functions are called directly.
– Object methods can be added or removed from an object dynamically, while functions are defined separately and can be reused in multiple contexts.
It’s important to understand the distinction between functions and object methods in JavaScript, as it affects how you organize and structure your code.
Related Article: How To Remove Duplicates From A Javascript Array
Iterating over Object Properties
To work with objects effectively, it is often necessary to iterate over their properties. In this chapter, we will explore different ways to iterate over object properties in JavaScript.
for…in loop
The for...in
loop is the most common way to iterate over the properties of an object. It allows you to loop through all enumerable properties of an object, including its prototype chain. Here’s an example:
const person = { name: 'John', age: 30, city: 'New York' }; for (let key in person) { console.log(key + ': ' + person[key]); }
In the above example, the for...in
loop iterates over each property of the person
object and logs the key-value pairs to the console. The output will be:
name: John age: 30 city: New York
It is important to note that the for...in
loop does not guarantee the order in which the properties are iterated. Also, it iterates over all enumerable properties, including inherited ones from the object’s prototype.
Object.keys()
The Object.keys()
method returns an array of a given object’s own enumerable property names. It allows you to iterate over only the object’s own properties, excluding any inherited ones. Here’s an example:
const car = { make: 'Toyota', model: 'Camry', year: 2020 }; const keys = Object.keys(car); for (let key of keys) { console.log(key + ': ' + car[key]); }
In the above example, Object.keys()
returns an array containing the keys of the car
object. The for...of
loop is then used to iterate over the keys and log the corresponding values to the console. The output will be:
make: Toyota model: Camry year: 2020
By using Object.keys()
, you can ensure that only the object’s own properties are iterated, without including any inherited properties.
Object.entries()
The Object.entries()
method returns an array of a given object’s own enumerable property key-value pairs. It allows you to iterate over both the keys and values of the object’s properties. Here’s an example:
const fruit = { name: 'Apple', color: 'Red', taste: 'Sweet' }; const entries = Object.entries(fruit); for (let [key, value] of entries) { console.log(key + ': ' + value); }
In the above example, Object.entries()
returns an array containing the key-value pairs of the fruit
object. The for...of
loop is then used to iterate over the entries and log them to the console. The output will be:
name: Apple color: Red taste: Sweet
Using Object.entries()
allows you to easily access both the keys and values of an object’s properties within a loop.
Object.entries() and Object.values()
The built-in Object.entries() and Object.values() methods provide convenient ways to access the values of an object.
The Object.entries() method returns an array of key-value pairs from an object. Each key-value pair is represented as an array with two elements: the key and the corresponding value. This method is useful when you want to iterate over an object and perform operations on both the keys and values.
Here’s an example that demonstrates how to use Object.entries() to iterate over an object:
const obj = { name: 'John', age: 30, city: 'New York' }; for (const [key, value] of Object.entries(obj)) { console.log(`${key}: ${value}`); }
This will output:
name: John age: 30 city: New York
In this example, the Object.entries(obj) returns an array of key-value pairs from the obj
object. The for…of loop then iterates over each key-value pair, and the destructuring assignment [key, value]
allows us to easily access the key and value of each pair.
The Object.values() method, on the other hand, returns an array of values from an object. This is useful when you are only interested in the values of an object and don’t need to access the corresponding keys.
Here’s an example that demonstrates how to use Object.values() to retrieve the values of an object:
const obj = { name: 'John', age: 30, city: 'New York' }; const values = Object.values(obj); console.log(values);
This will output:
[ 'John', 30, 'New York' ]
In this example, the Object.values(obj) returns an array containing the values from the obj
object. These values are then assigned to the values
variable, which is logged to the console.
Both Object.entries() and Object.values() methods are part of the ECMAScript 2017 specification and are supported by modern browsers. However, if you need to support older browsers, you may need to use a polyfill or transpile your code using a tool like Babel.
These methods provide convenient ways to access the keys and values of an object in JavaScript and can be particularly useful when working with complex data structures.
Object.getOwnPropertyDescriptors()
The Object.getOwnPropertyDescriptors()
method is a powerful tool in JavaScript that allows you to retrieve all own property descriptors of an object. It returns an object containing all the property descriptors of the specified object, both enumerable and non-enumerable.
The method takes an object as its parameter and returns a new object with property descriptors for each own property of the input object. Each property descriptor is represented by a key-value pair, where the key is the name of the property and the value is the corresponding property descriptor object.
Here’s a simple example to illustrate its usage:
const person = { name: 'John', age: 30, get fullName() { return this.name; } }; const descriptors = Object.getOwnPropertyDescriptors(person); console.log(descriptors);
In this example, we define an object called person
with three properties: name
, age
, and a getter method fullName
. We then use the Object.getOwnPropertyDescriptors()
method to retrieve the property descriptors of the person
object.
The resulting descriptors
object will contain the following key-value pairs:
{ name: { value: 'John', writable: true, enumerable: true, configurable: true }, age: { value: 30, writable: true, enumerable: true, configurable: true }, fullName: { get: [Function: get fullName], set: undefined, enumerable: true, configurable: true } }
As you can see, each property descriptor object contains information about the property, such as its value, whether it is writable, enumerable, and configurable. In the case of the getter method fullName
, the descriptor includes the get
function.
This method is particularly useful when you need to clone an object and preserve its property descriptors. By using Object.getOwnPropertyDescriptors()
in combination with Object.create()
, you can create a new object with the same property descriptors as the original object.
const clonedPerson = Object.create( Object.getPrototypeOf(person), Object.getOwnPropertyDescriptors(person) ); console.log(clonedPerson);
In the above example, we create a new object called clonedPerson
using Object.create()
. The first argument to Object.create()
is the prototype of the new object, which we obtain using Object.getPrototypeOf()
. The second argument is the property descriptors obtained from the original person
object using Object.getOwnPropertyDescriptors()
.
By using Object.getOwnPropertyDescriptors()
, you can manipulate property descriptors in more advanced ways, such as defining non-enumerable properties or freezing objects, among others. This method provides a deeper understanding of the properties of an object and enables fine-grained control over its behavior.
To learn more about the Object.getOwnPropertyDescriptors()
method, you can refer to the MDN web docs.
That’s it for the Object.getOwnPropertyDescriptors()
method! You now have a better understanding of how to retrieve property descriptors of an object and use them to manipulate and clone objects in JavaScript.
Understanding Symbol Properties
In JavaScript, symbols are a unique data type introduced in ECMAScript 2015. They are often used to define properties on objects that are meant to be private and inaccessible from other parts of the code. Symbol properties are ideal for scenarios where you want to add metadata to an object without the risk of name collisions.
To create a symbol, you can use the Symbol()
function. Each call to Symbol()
returns a new and unique symbol. Here’s an example:
const mySymbol = Symbol();
You can also provide a description to the symbol, which can be useful for debugging purposes:
const mySymbol = Symbol('my description');
Symbol properties can be added to objects using square bracket notation. Let’s see an example:
const obj = {}; const mySymbol = Symbol('my symbol'); obj[mySymbol] = 'Hello, symbol!'; console.log(obj[mySymbol]); // Output: Hello, symbol!
To access the value of a symbol property, you need to use the same symbol that was used to define it. Symbol properties are not enumerable in for...in
loops or Object.keys()
, which means they won’t be included when iterating over an object’s properties.
console.log(Object.keys(obj)); // Output: [] for (const key in obj) { console.log(key); // No output }
Another way to define symbol properties is by using the Object.defineProperty()
method. This allows you to provide additional configuration options, such as making the property read-only or non-enumerable. Here’s an example:
const obj = {}; const mySymbol = Symbol('my symbol'); Object.defineProperty(obj, mySymbol, { value: 'Hello, symbol!', writable: false, enumerable: false }); console.log(obj[mySymbol]); // Output: Hello, symbol!
Symbols can also be used as keys in object literals:
const mySymbol = Symbol('my symbol'); const obj = { [mySymbol]: 'Hello, symbol!' }; console.log(obj[mySymbol]); // Output: Hello, symbol!
It’s worth noting that you cannot convert a symbol to a string directly. However, you can use the toString()
method to get a string representation of a symbol:
const mySymbol = Symbol('my symbol'); console.log(mySymbol.toString()); // Output: Symbol(my symbol)
Symbol properties provide a way to add private and unique properties to objects, helping to prevent accidental name collisions and providing better encapsulation in your code. They are a powerful feature of JavaScript that can be leveraged to create robust and maintainable applications.
Using Symbols as Object Properties
Symbols are a unique data type introduced in ES6 that allow you to create property keys that are guaranteed to be unique. Unlike strings or numbers, symbols are completely unique and cannot be duplicated. This makes them useful for creating private or hidden properties on objects.
To create a symbol, you can use the Symbol()
function. Each time you call Symbol()
, you get a new unique symbol:
const mySymbol = Symbol(); console.log(typeof mySymbol); // "symbol"
Symbols can also have a description, which is useful for debugging purposes:
const mySymbol = Symbol('my description'); console.log(mySymbol.toString()); // "Symbol(my description)"
Symbols can be used as property keys in objects, just like strings or numbers:
const obj = {}; const mySymbol = Symbol('my symbol'); obj[mySymbol] = 'Hello, symbol!'; console.log(obj[mySymbol]); // "Hello, symbol!"
One advantage of using symbols as property keys is that they are not enumerable in for…in loops or with the Object.keys()
method. This means that symbols won’t be accidentally accessed or modified by other parts of your code:
const obj = { [Symbol('mySymbol')]: 'Hello, symbol!', foo: 'Hello, string!' }; console.log(Object.keys(obj)); // ["foo"]
Symbols can also be used to define private properties in classes or modules. By using symbols, you can create properties that are not accessible from outside the class or module:
const _privateSymbol = Symbol('private'); class MyClass { constructor() { this[_privateSymbol] = 'Private property'; } getPrivateProperty() { return this[_privateSymbol]; } } const myInstance = new MyClass(); console.log(myInstance.getPrivateProperty()); // "Private property" console.log(myInstance[_privateSymbol]); // undefined
In this example, the _privateSymbol
symbol is used to create a private property in the MyClass
class. The getPrivateProperty()
method allows accessing the private property, but it cannot be accessed directly from outside the class.
Symbols are a powerful tool in JavaScript for creating unique property keys and defining private properties. By using symbols, you can avoid naming collisions and create more robust and encapsulated code.
Object.getOwnPropertySymbols()
The Object.getOwnPropertySymbols()
method is a built-in JavaScript function that returns an array of all symbol properties found on a given object.
Symbols are a unique data type introduced in ECMAScript 2015 (ES6) and are used as property keys for object properties. Unlike strings or numbers, symbols are guaranteed to be unique, which makes them useful for creating private or hidden properties.
To illustrate how Object.getOwnPropertySymbols()
works, consider the following example:
const firstName = Symbol('first name'); const person = { [firstName]: 'John', age: 30 }; const symbols = Object.getOwnPropertySymbols(person); console.log(symbols); // Output: [Symbol(first name)]
In this example, we define a symbol firstName
and use it as a property key for the person
object. When we call Object.getOwnPropertySymbols()
on the person
object, it returns an array containing the firstName
symbol.
The Object.getOwnPropertySymbols()
method only returns symbol properties that are directly defined on the object itself, not inherited from its prototype chain. If you want to retrieve all symbol properties, including inherited ones, you can use the Reflect.ownKeys()
method instead.
Here’s an example that demonstrates how to use Object.getOwnPropertySymbols()
with both directly defined and inherited symbol properties:
const firstName = Symbol('first name'); const lastName = Symbol('last name'); const person = { [firstName]: 'John' }; const employee = Object.create(person, { [lastName]: { value: 'Doe' } }); const symbols = Object.getOwnPropertySymbols(employee); console.log(symbols); // Output: [Symbol(first name)]
In this example, we define two symbols, firstName
and lastName
. We then create an employee
object using Object.create()
, with person
as its prototype. The employee
object inherits the firstName
symbol property from the person
object, but the lastName
symbol property is directly defined on the employee
object.
When we call Object.getOwnPropertySymbols()
on the employee
object, it only returns the firstName
symbol, since it is the only symbol property directly defined on the object.
Overall, Object.getOwnPropertySymbols()
provides a way to access the symbol properties of an object. This can be useful in scenarios where symbols are used to create private or hidden properties, allowing you to retrieve and manipulate them programmatically.
Creating Objects: Object Literals
One of the simplest ways to create a JavaScript object is by using the object literal syntax. Object literals allow you to define an object and its properties in a concise and readable way.
To create an object literal, you enclose a list of key-value pairs inside curly braces. Each key-value pair represents a property of the object. The key is a string that acts as the name of the property, and the value can be any valid JavaScript expression.
Let’s take a look at an example:
const person = { name: 'John', age: 30, city: 'New York' };
In this example, we have created an object called person
with three properties: name
, age
, and city
. The values of these properties are 'John'
, 30
, and 'New York'
, respectively.
You can access the properties of an object using dot notation or square bracket notation. For example, to access the name
property of the person
object, you can use either person.name
or person['name']
. Both will return the value 'John'
.
Object literals can also contain nested objects as properties. For instance:
const car = { make: 'Toyota', model: 'Camry', year: 2020, owner: { name: 'Alice', age: 25, city: 'Los Angeles' } };
In this example, the car
object has a nested object called owner
, which has its own properties such as name
, age
, and city
.
Object literals are a convenient way to create objects with a fixed set of properties. However, if you need to create multiple objects with the same properties, it can be tedious to repeat the object literal syntax. In such cases, you might consider using constructor functions or ES6 classes, which we will cover in later chapters.
To learn more about object literals and their usage in JavaScript, you can refer to the MDN documentation.
Now that we have seen how to create objects using object literals, let’s move on to exploring different ways to add and modify properties in JavaScript objects.
Creating Objects: Constructor Functions
Constructor functions are a way to create multiple objects with the same properties and methods. They are similar to classes in other programming languages. Constructor functions are used to define and initialize properties and methods for objects.
To create an object using a constructor function, you need to follow these steps:
1. Define the constructor function:
function Person(name, age) { this.name = name; this.age = age; }
2. Use the new
keyword to create an instance of the object:
let person1 = new Person("John Doe", 25); let person2 = new Person("Jane Smith", 30);
In the above example, the Person
constructor function takes two parameters, name
and age
. It assigns these values to the newly created object using the this
keyword.
By convention, constructor functions start with an uppercase letter to distinguish them from regular functions.
You can add methods to the objects created by the constructor function by extending the prototype of the constructor function:
Person.prototype.greet = function() { return "Hello, my name is " + this.name + " and I am " + this.age + " years old."; };
Now, both person1
and person2
objects have access to the greet
method.
console.log(person1.greet()); // Output: Hello, my name is John Doe and I am 25 years old. console.log(person2.greet()); // Output: Hello, my name is Jane Smith and I am 30 years old.
Constructor functions can be useful when you need to create multiple objects with similar properties and methods. They provide a way to define a blueprint for creating objects and allow you to easily create instances of that blueprint.
It’s important to note that constructor functions are just one way to create objects in JavaScript. Another approach is to use the class
syntax introduced in ES6, which provides a more familiar syntax for those coming from other programming languages. However, under the hood, classes in JavaScript are still based on constructor functions.
Related Article: How To Sum An Array Of Numbers In Javascript
Property Descriptors
Properties in JavaScript objects are not just simple key-value pairs. They also have additional attributes known as property descriptors. These descriptors define the behavior and characteristics of each property. Understanding property descriptors is crucial when working with objects in JavaScript.
A property descriptor is an object that contains the attributes of a property. These attributes include:
– value: The value of the property.
– writable: A boolean indicating whether the property can be changed.
– enumerable: A boolean indicating whether the property can be enumerated.
– configurable: A boolean indicating whether the property can be deleted or its attributes can be changed.
By default, when we create a new property in an object, its descriptors are set to true for all attributes except for writable, which is set to true.
Let’s take a look at an example:
const person = { name: 'John', age: 30 }; console.log(Object.getOwnPropertyDescriptor(person, 'name'));
This code snippet uses the getOwnPropertyDescriptor
method to retrieve the property descriptor for the property ‘name’ in the person
object. The output will be an object with the attributes of the property.
Modifying Property Descriptors
We can modify the descriptors of an existing property using the Object.defineProperty
method. This method allows us to change the value, writable, enumerable, and configurable attributes of a property.
Here’s an example:
const person = { name: 'John', age: 30 }; Object.defineProperty(person, 'name', { writable: false }); person.name = 'Jane'; // Error: Cannot assign to read only property 'name' of object '#<Object>' console.log(Object.getOwnPropertyDescriptor(person, 'name'));
In this example, we set the writable attribute of the name
property to false, making it read-only. When we try to assign a new value to the name
property, it throws an error.
Property Descriptors and Object Manipulation
Property descriptors are particularly useful when working with object manipulation methods, such as Object.keys
, Object.values
, and Object.entries
. These methods iterate over the properties of an object and return an array of keys, values, or key-value pairs, respectively.
By default, these methods only return the enumerable properties of an object. If we want to include non-enumerable properties in the result, we can modify the enumerable attribute of the properties.
Here’s an example:
const person = { name: 'John', age: 30 }; Object.defineProperty(person, 'address', { value: '123 Main St.', enumerable: false }); console.log(Object.keys(person)); // ['name', 'age'] console.log(Object.entries(person)); // [['name', 'John'], ['age', 30]]
In this example, we added a new property called address
to the person
object and set its enumerable attribute to false. As a result, when we use Object.keys
, the address
property is not included in the resulting array.
Accessing Object Properties: Dot Notation vs Bracket Notation
To access the properties of an object, there are two commonly used notations: dot notation and bracket notation.
Dot Notation
Dot notation is the most commonly used method to access object properties. It involves using a dot (.) followed by the property name to access the value stored in the object.
Here’s an example:
const person = { name: 'John', age: 30, address: { street: '123 Main St', city: 'New York', }, }; console.log(person.name); // Output: John console.log(person.age); // Output: 30 console.log(person.address.street); // Output: 123 Main St console.log(person.address.city); // Output: New York
In the above example, we access the properties of the person
object using dot notation. We can directly access the top-level properties (name
and age
) as well as nested properties (address.street
and address.city
).
Related Article: How To Check If an Array Contains a Value In JavaScript
Bracket Notation
Bracket notation is an alternative way to access object properties. It involves using square brackets ([]), enclosing the property name as a string, to access the value stored in the object.
Here’s an example:
const person = { name: 'John', age: 30, address: { street: '123 Main St', city: 'New York', }, }; console.log(person['name']); // Output: John console.log(person['age']); // Output: 30 console.log(person['address']['street']); // Output: 123 Main St console.log(person['address']['city']); // Output: New York
In the above example, we access the properties of the person
object using bracket notation. The property name is provided as a string inside the square brackets. Like dot notation, bracket notation can be used to access both top-level and nested properties.
When to Use Each Notation
Both dot notation and bracket notation can be used interchangeably in most cases. However, there are some scenarios where one notation might be preferred over the other.
– Dot notation is generally more concise and easier to read. It is the recommended choice for accessing properties when the property name is known and does not contain any special characters.
– Bracket notation is useful when working with dynamic property names or when the property name contains special characters, such as spaces or hyphens. In such cases, dot notation will result in a syntax error, and bracket notation must be used.
const person = { 'first name': 'John', 'last name': 'Doe', }; console.log(person['first name']); // Output: John console.log(person['last name']); // Output: Doe
In the above example, the properties of the person
object have spaces in their names. To access these properties, we must use bracket notation.
Both dot notation and bracket notation can be used to access object properties in JavaScript. Dot notation is generally more common and easier to read, while bracket notation is useful for dynamic or special character property names.
The ‘this’ Keyword: Context and Scope
The ‘this’ keyword in JavaScript is a powerful and often misunderstood feature. It allows you to refer to the current object or context within a function. Understanding how ‘this’ works is essential for writing clean and efficient JavaScript code.
Related Article: How to Use the JavaScript Filter Array Method
Context and Scope
The ‘this’ keyword in JavaScript is used to refer to the object that the function is a property of or is being called on. It provides a way to access properties and methods within an object. The value of ‘this’ is determined by how a function is called, which can vary depending on the context and scope.
Let’s consider an example to better understand the concept of ‘this’. Suppose we have an object called person
with a property called name
and a method called sayHello()
:
const person = { name: 'John', sayHello: function() { console.log(`Hello, my name is ${this.name}.`); } };
In this example, the ‘this’ keyword inside the sayHello()
method refers to the person
object. When we call person.sayHello()
, it will output “Hello, my name is John.” The value of ‘this’ is resolved at runtime based on how the function is called.
Function Invocation Patterns
The value of ‘this’ can change depending on how a function is invoked. There are four common ways to invoke a function in JavaScript: function invocation, method invocation, constructor invocation, and using the ‘apply’ or ‘call’ methods.
1. Function Invocation:
When a function is invoked as a standalone function, without any context or object, the value of ‘this’ inside the function will be the global object (e.g., window
in a browser or global
in Node.js).
function sayHello() { console.log(`Hello, my name is ${this.name}.`); } sayHello(); // Output: Hello, my name is undefined.
In this example, since the function is invoked without any context, the value of ‘this.name’ is undefined.
2. Method Invocation:
When a function is invoked as a method of an object, the value of ‘this’ inside the function will be the object itself.
const person = { name: 'John', sayHello: function() { console.log(`Hello, my name is ${this.name}.`); } }; person.sayHello(); // Output: Hello, my name is John.
In this example, when we call person.sayHello()
, the value of ‘this’ inside the sayHello()
method refers to the person
object.
3. Constructor Invocation:
When a function is invoked with the ‘new’ keyword to create an instance of an object, the value of ‘this’ inside the function will be the newly created object.
function Person(name) { this.name = name; this.sayHello = function() { console.log(`Hello, my name is ${this.name}.`); } } const john = new Person('John'); john.sayHello(); // Output: Hello, my name is John.
In this example, when we create a new instance of the Person
object using the ‘new’ keyword, the value of ‘this’ inside the constructor function refers to the newly created object.
4. Using ‘apply’ or ‘call’ methods:
The ‘apply’ and ‘call’ methods allow you to invoke a function with a specific context by explicitly setting the value of ‘this’.
function sayHello() { console.log(`Hello, my name is ${this.name}.`); } const person = { name: 'John' }; sayHello.apply(person); // Output: Hello, my name is John.
In this example, we use the apply
method to invoke the sayHello
function with the person
object as the context. The value of ‘this’ inside the function will be set to the person
object.
Arrow Functions and ‘this’
It’s important to note that arrow functions in JavaScript do not have their own ‘this’ value. Instead, they inherit the ‘this’ value from the enclosing lexical scope. This behavior is different from regular functions, where ‘this’ is determined by how the function is called.
const person = { name: 'John', sayHello: function() { setTimeout(() => { console.log(`Hello, my name is ${this.name}.`); }, 1000); } }; person.sayHello(); // Output: Hello, my name is John.
In this example, the arrow function inside the setTimeout
function retains the ‘this’ value from the sayHello
method, allowing us to access the name
property of the person
object.
Understanding the ‘this’ keyword and its various invocation patterns is crucial for writing effective JavaScript code. It allows you to access and manipulate object properties and methods within functions.
Related Article: How To Loop Through An Array In Javascript
Prototype Inheritance
JavaScript is a prototype-based language, which means that objects can inherit properties and methods from other objects. This concept is known as prototype inheritance. Understanding prototype inheritance is essential in JavaScript, as it allows you to create reusable code and build complex object hierarchies.
Each object has an internal property called [[Prototype]]
, which is a reference to another object. When you access a property or method on an object, JavaScript first checks if the object itself has that property. If it doesn’t, it looks up the prototype chain until it finds the property or reaches the end of the chain.
The prototype chain is a sequence of objects where each object’s [[Prototype]]
property references its parent object. This process continues until the end of the chain, where the last object’s [[Prototype]]
is null
. This is known as the prototype chain’s root.
Let’s look at an example to understand how prototype inheritance works:
// Creating an object using object literal syntax const person = { name: 'John', age: 30, }; // Adding a method to the person object person.sayHello = function() { console.log(`Hello, my name is ${this.name}!`); }; // Creating a new object using the person object as its prototype const john = Object.create(person); // Accessing properties and methods console.log(john.name); // Output: 'John' john.sayHello(); // Output: 'Hello, my name is John!'
In the above example, we create an object person
using object literal syntax. We then add a method sayHello
to the person
object. Next, we create a new object john
using Object.create()
, passing person
as the prototype. This means that john
inherits all the properties and methods from person
.
When we access the name
property on the john
object, JavaScript first checks if john
has the property directly. Since it doesn’t, it looks up the prototype chain and finds the name
property on the person
object.
Similarly, when we call the sayHello
method on the john
object, JavaScript checks if john
has the method directly. Since it doesn’t, it looks up the prototype chain and finds the sayHello
method on the person
object.
Prototype inheritance allows us to create hierarchical relationships between objects. We can create multiple levels of inheritance by setting the [[Prototype]]
of an object to another object, effectively creating a chain of prototypes.
It’s important to note that changes to the prototype object will affect all objects that inherit from it. If we modify a property on the prototype object, the change will be reflected in all the derived objects. This behavior can be advantageous in some cases, but it requires caution to avoid unexpected side effects.
Prototype inheritance is a fundamental concept in JavaScript that allows objects to inherit properties and methods from other objects. Understanding how the prototype chain works is crucial for building complex object hierarchies and creating reusable code.
Prototype Inheritance: Object.create()
Objects can inherit properties and methods from other objects. Prototype inheritance allows us to create a chain of objects, where each object inherits properties and methods from its prototype. One way to create objects with a specific prototype is by using the Object.create()
method.
The Object.create()
method creates a new object with the specified prototype object and properties. It takes two arguments: the prototype object and an optional object containing additional properties for the newly created object.
Here’s an example of using Object.create()
to create a new object with a specified prototype:
const vehicle = { wheels: 4, start() { console.log("Starting the vehicle..."); } }; const car = Object.create(vehicle); car.make = "Toyota"; car.model = "Camry"; console.log(car.make); // Output: Toyota console.log(car.model); // Output: Camry console.log(car.wheels); // Output: 4 car.start(); // Output: Starting the vehicle...
In the example above, we have a vehicle
object with properties wheels
and start
. We then create a new object car
using Object.create(vehicle)
. The car
object inherits the properties and methods from the vehicle
object.
We can also add additional properties to the car
object. In this case, we added make
and model
properties. These properties are specific to the car
object and are not inherited from the prototype.
When we access the properties of the car
object, it first checks if the property exists in the object itself. If it doesn’t, it looks for the property in the prototype chain. In this example, the car
object has the make
and model
properties, which are specific to the car
object, while the wheels
property is inherited from the vehicle
prototype.
The Object.create()
method is a powerful tool for creating objects with specific prototypes. It allows us to create a hierarchy of objects, where each object inherits properties and methods from its prototype. By utilizing prototype inheritance, we can create more efficient and reusable code.
To learn more about prototype inheritance and object-oriented programming in JavaScript, you can refer to the following resources:
– MDN Web Docs: Inheritance and the prototype chain
– JavaScript.info: Prototypes, inheritance
That’s it for this chapter! We have explored prototype inheritance in JavaScript and learned how to create objects with a specific prototype using the Object.create()
method. In the next chapter, we will dive deeper into object-oriented programming concepts in JavaScript.
Prototype Inheritance: Object.setPrototypeOf()
Objects can inherit properties and methods from other objects through a mechanism called prototype inheritance. The prototype of an object serves as a blueprint for creating new objects, and it provides a way to share common functionality across multiple objects.
One way to establish prototype inheritance is by using the Object.setPrototypeOf() method. This method allows you to set the prototype (i.e., the parent object) of a given object.
The syntax for Object.setPrototypeOf() is as follows:
Object.setPrototypeOf(obj, prototype)
Here, “obj” is the object whose prototype we want to set, and “prototype” is the object that will become the new prototype of “obj”.
Let’s look at an example to understand how Object.setPrototypeOf() works:
const parent = { sayHello() { console.log('Hello!'); } }; const child = { name: 'John' }; Object.setPrototypeOf(child, parent); child.sayHello(); // Output: Hello!
In this example, we have two objects: “parent” and “child”. The “parent” object has a method called “sayHello” that logs ‘Hello!’ to the console. The “child” object has a property called “name”.
By calling Object.setPrototypeOf(child, parent), we set the prototype of “child” to be “parent”. As a result, the “child” object now inherits the “sayHello” method from the “parent” object.
When we invoke the “sayHello” method on the “child” object (child.sayHello()), it logs ‘Hello!’ to the console.
It’s important to note that changing an object’s prototype at runtime can have performance implications, as the JavaScript engine needs to look up properties and methods on the prototype chain. Therefore, it’s generally recommended to set the prototype of an object when it’s created, rather than modifying it later.
Additionally, it’s worth mentioning that Object.setPrototypeOf() is not supported in older versions of Internet Explorer. If you need to support older browsers, you can use other techniques, such as Object.create() or constructor functions, to achieve prototype inheritance.
To learn more about prototype inheritance and other related concepts in JavaScript, you can refer to the MDN documentation on Object.prototype and Prototype-based programming].
That covers the basics of prototype inheritance and how to use Object.setPrototypeOf() to establish prototype relationships between objects. In the next chapter, we will explore another important concept in JavaScript objects: the “this” keyword.
Related Article: How To Convert Array To Object In Javascript
Prototype Inheritance: Class Syntax
One way to create objects in JavaScript is by using the class syntax. The class syntax was introduced in ECMAScript 2015 (ES6) and provides a more familiar syntax for creating objects that resemble classes in other programming languages. However, it’s important to note that JavaScript classes are still based on prototypes under the hood.
To create a class, you use the class
keyword followed by the name of the class. Inside the class body, you can define methods and properties just like you would in a regular object. Here’s an example:
class Person { constructor(name, age) { this.name = name; this.age = age; } sayHello() { console.log(`Hello, my name is ${this.name} and I'm ${this.age} years old.`); } }
In the example above, we define a Person
class with a constructor that takes name
and age
as parameters. We also define a sayHello
method that logs a message to the console.
To create an instance of a class, you use the new
keyword followed by the name of the class, just like you would with constructors in other languages. Here’s an example:
const john = new Person("John Doe", 30); john.sayHello(); // Output: Hello, my name is John Doe and I'm 30 years old.
In this example, we create a new instance of the Person
class called john
and pass in the name “John Doe” and age 30 as arguments to the constructor. We then call the sayHello
method on the john
object, which logs the message to the console.
Under the hood, JavaScript classes still use prototype inheritance. The methods and properties defined inside a class are added to the prototype of any instances created from that class. This allows all instances to share the same methods and properties without duplicating them in memory.
The class syntax in JavaScript provides a more familiar way to create objects that resemble classes in other programming languages. However, it’s important to understand that JavaScript classes are still based on prototype inheritance.
Understanding Object Cloning
When working with JavaScript objects, you may come across situations where you need to create a copy, or clone, of an existing object. Object cloning is the process of creating a new object with the same properties and values as the original object. This can be useful in scenarios where you want to preserve the state of an object or pass a copy of an object to a function without modifying the original.
In JavaScript, there are a few different ways to clone an object. Let’s explore some of these methods.
1. Using the Spread Operator:
The spread operator (…) is a convenient way to clone an object. It creates a shallow copy of the object, meaning that any nested objects or arrays within the original object will still be referenced by the new object.
const originalObject = { name: 'John', age: 30 }; const clonedObject = { ...originalObject }; console.log(clonedObject); // { name: 'John', age: 30 }
2. Using the Object.assign() Method:
The Object.assign() method can also be used to clone an object. It takes one or more source objects and merges them into a target object. In the case of cloning, an empty object can be used as the target object to create a new object with the same properties as the original object.
const originalObject = { name: 'John', age: 30 }; const clonedObject = Object.assign({}, originalObject); console.log(clonedObject); // { name: 'John', age: 30 }
3. Using JSON.stringify() and JSON.parse():
Another way to clone an object is by using JSON.stringify() and JSON.parse(). This method converts the object to a JSON string and then parses it back into an object. Note that this method only works for objects that can be serialized to JSON, so any functions or non-serializable values will be lost in the cloning process.
const originalObject = { name: 'John', age: 30 }; const clonedObject = JSON.parse(JSON.stringify(originalObject)); console.log(clonedObject); // { name: 'John', age: 30 }
It’s important to note that all of these cloning methods create a shallow copy of the object. If the original object contains nested objects or arrays, those nested references will still be shared between the original and cloned objects. To create a deep copy that includes all nested objects, you would need to implement a custom cloning function or use a library like lodash.
Understanding how to clone objects in JavaScript is essential for managing state and data immutability in your applications. Whether you choose to use the spread operator, Object.assign(), or JSON methods, object cloning allows you to create copies of objects and work with them separately from the original.
Now that we have a grasp of object cloning, let’s move on to the next chapter where we will explore another important concept: object inheritance.
Deep Cloning vs Shallow Cloning
When working with JavaScript objects, it is important to understand the difference between deep cloning and shallow cloning. Both techniques are used to create a copy of an object, but they differ in how they handle nested objects and arrays.
Shallow Cloning
Shallow cloning creates a new object that is a copy of the original object, but the properties of the new object still reference the same objects as the original object. In other words, any changes made to the nested objects or arrays in the new object will also affect the original object.
One way to perform shallow cloning in JavaScript is by using the spread operator (...
). Here’s an example:
const originalObject = { name: 'John', age: 30, hobbies: ['reading', 'coding'] }; const clonedObject = { ...originalObject }; clonedObject.name = 'Jane'; clonedObject.hobbies.push('painting'); console.log(originalObject); console.log(clonedObject);
In this example, the clonedObject
is a shallow clone of the originalObject
. When we change the name
property in the clonedObject
, it does not affect the originalObject
. However, when we push a new hobby to the hobbies
array in the clonedObject
, it also affects the originalObject
.
Deep Cloning
Deep cloning creates a completely independent copy of an object, including all nested objects and arrays. Any changes made to the cloned object or its nested objects will not affect the original object.
There are several ways to perform deep cloning in JavaScript. One common approach is to use the JSON.stringify()
and JSON.parse()
methods. Here’s an example:
const originalObject = { name: 'John', age: 30, hobbies: ['reading', 'coding'] }; const clonedObject = JSON.parse(JSON.stringify(originalObject)); clonedObject.name = 'Jane'; clonedObject.hobbies.push('painting'); console.log(originalObject); console.log(clonedObject);
In this example, the clonedObject
is a deep clone of the originalObject
. When we change the name
property in the clonedObject
or push a new hobby to the hobbies
array, it does not affect the originalObject
.
It’s important to note that the JSON.stringify()
and JSON.parse()
method approach for deep cloning has limitations. It cannot clone functions, undefined values, or circular references.
Another way to perform deep cloning is by using libraries like lodash or jQuery, which provide utility functions for deep cloning objects.
Understanding the difference between deep cloning and shallow cloning is crucial when working with complex objects in JavaScript. Choosing the appropriate cloning technique can help ensure that your code behaves as expected and avoids unintended side effects.
Related Article: How To Add A Class To A Given Element In Javascript
Object Destructuring: Unpacking Values
Object destructuring is a convenient way to extract values from objects and assign them to variables. It allows you to unpack properties from an object into distinct variables, making your code more readable and concise.
Object destructuring is especially useful when working with complex objects or when you only need a few specific properties from an object. It provides a concise syntax for extracting values and assigning them to variables in a single line of code.
To perform object destructuring, you use curly braces ({}) on the left-hand side of the assignment operator (=) and specify the properties you want to extract from the object. The variable names inside the curly braces should match the property names in the object.
Here’s a simple example that demonstrates object destructuring:
const person = { name: 'John Doe', age: 25, location: 'New York' }; const { name, age } = person; console.log(name); // Output: John Doe console.log(age); // Output: 25
In this example, we have an object person
with properties like name
, age
, and location
. By using object destructuring, we extract the name
and age
properties from the person
object and assign them to separate variables with the same names.
You can also assign the extracted values to variables with different names by using the :
operator. Here’s an example:
const { name: fullName, age: years } = person; console.log(fullName); // Output: John Doe console.log(years); // Output: 25
In this case, we extract the name
property from the person
object and assign it to a variable called fullName
. Similarly, the age
property is assigned to a variable called years
.
Object destructuring also works with nested objects. You can extract properties from nested objects by using dot notation. Here’s an example:
const student = { name: 'Jane Doe', age: 21, university: { name: 'XYZ University', location: 'London' } }; const { name, university: { location } } = student; console.log(name); // Output: Jane Doe console.log(location); // Output: London
In this example, we have a nested object university
inside the student
object. By using object destructuring, we extract the name
property from the student
object and the location
property from the nested university
object.
Object destructuring can also be used with function parameters to extract values from objects directly. Here’s an example:
function displayPersonInfo({ name, age }) { console.log(`Name: ${name}`); console.log(`Age: ${age}`); } const person = { name: 'John Doe', age: 25, location: 'New York' }; displayPersonInfo(person);
In this example, we define a function displayPersonInfo
that expects an object as a parameter. By using object destructuring in the function parameter, we extract the name
and age
properties from the passed object directly.
Object destructuring is a powerful feature in JavaScript that allows you to extract values from objects easily. It improves code readability and reduces the amount of boilerplate code needed to access object properties. By mastering object destructuring, you can write cleaner and more concise code in your JavaScript applications.
Object Spread: Merging Objects
The object spread syntax was introduced in ECMAScript 2018 as a concise way to merge objects. It allows you to create a new object by copying the properties from one or more source objects into a target object. This can be especially handy when you want to combine the properties of multiple objects into a single object.
To use the object spread syntax, you simply use three dots (…) followed by the object you want to spread. Let’s take a look at a basic example:
const object1 = { x: 1, y: 2 }; const object2 = { z: 3 }; const mergedObject = { ...object1, ...object2 }; console.log(mergedObject); // Output: { x: 1, y: 2, z: 3 }
In this example, we have two objects, object1
and object2
, with different properties. By using the object spread syntax, we can merge these objects into a new object called mergedObject
. The resulting object will contain all the properties from both object1
and object2
.
It’s important to note that if there are duplicate property names in the source objects, the value of the property from the object that appears last in the spread will overwrite any previous values. Let’s see an example:
const object1 = { x: 1, y: 2 }; const object2 = { y: 3 }; const mergedObject = { ...object1, ...object2 }; console.log(mergedObject); // Output: { x: 1, y: 3 }
In this case, the value of the y
property in object2
overwrites the value of the y
property in object1
, resulting in a merged object with { x: 1, y: 3 }
.
You can also use the object spread syntax to add new properties to an existing object. Let’s see an example:
const object1 = { x: 1, y: 2 }; const mergedObject = { ...object1, z: 3 }; console.log(mergedObject); // Output: { x: 1, y: 2, z: 3 }
In this example, we add a new property z
to object1
by using the object spread syntax. The resulting object mergedObject
will contain all the properties from object1
and the new property z
.
In addition to spreading individual objects, you can also spread an array of objects. This allows you to merge multiple objects into a single object in a concise way. Here’s an example:
const objects = [ { x: 1 }, { y: 2 }, { z: 3 } ]; const mergedObject = { ...objects }; console.log(mergedObject); // Output: { x: 1, y: 2, z: 3 }
In this example, we have an array of objects called objects
. By using the object spread syntax with the array, we can merge all the objects into a single object called mergedObject
.
The object spread syntax is a powerful tool in JavaScript that allows you to easily merge objects together. It provides a concise and expressive way to create new objects by spreading the properties from one or more source objects. Whether you need to merge objects or add new properties, the object spread syntax is a valuable addition to your JavaScript toolkit.
Object Composition: Combining Objects
Object composition is a technique that allows you to build complex objects by combining simpler objects together. It is a powerful concept that promotes code reusability and modularity. Instead of creating large, monolithic objects, you can create small, focused objects and compose them together to form more complex structures.
There are multiple ways to combine objects in JavaScript, and each method has its own benefits and use cases. Let’s explore some of the common techniques:
1. Object.assign()
The Object.assign()
method is a built-in function that allows you to copy the values of all enumerable properties from one or more source objects to a target object. It returns the modified target object.
const person = { name: 'John', age: 30 }; const details = { occupation: 'Developer', location: 'New York' }; const mergedObject = Object.assign({}, person, details); console.log(mergedObject);
In the example above, we create two objects: person
and details
. We then use Object.assign()
to merge the properties of both objects into a new object called mergedObject
. The resulting object contains all the properties from both person
and details
.
2. Spread Operator
The spread operator (...
) can be used to copy the properties from one object to another. It is similar to Object.assign()
, but it provides a more concise syntax.
const person = { name: 'John', age: 30 }; const details = { occupation: 'Developer', location: 'New York' }; const mergedObject = { ...person, ...details }; console.log(mergedObject);
In this example, we use the spread operator to merge the properties of person
and details
into mergedObject
. The result is the same as the previous example using Object.assign()
.
3. Mixins
Mixins are a way to combine the properties and methods of multiple objects into a single object. They can be used to add functionality to an existing object or create a new object with combined features.
const canEat = { eat: function() { console.log('Eating...'); } }; const canSleep = { sleep: function() { console.log('Sleeping...'); } }; const person = {}; Object.assign(person, canEat, canSleep); person.eat(); // Output: Eating... person.sleep(); // Output: Sleeping...
In this example, we define two mixins: canEat
and canSleep
. We then use Object.assign()
to add the properties and methods from both mixins to the person
object. As a result, the person
object gains the ability to eat and sleep.
4. Factory Functions
Factory functions are functions that return new objects. They can be used to create objects with specific properties and behaviors.
function createPerson(name, age) { return { name: name, age: age, sayHello: function() { console.log('Hello, my name is ' + this.name); } }; } const person = createPerson('John', 30); person.sayHello(); // Output: Hello, my name is John
In this example, we define a factory function createPerson()
that takes name
and age
as parameters and returns a new object with those properties. The returned object also has a sayHello()
method that logs a greeting message.
These are just a few examples of how you can combine objects in JavaScript. Object composition is a flexible and powerful technique that can be used to create complex and reusable code structures. By breaking down objects into smaller, focused pieces and combining them together, you can create more maintainable and modular code.
Related Article: How To Append To A Javascript Array
Understanding Object Serialization
Serialization is the process of converting an object into a format that can be stored or transmitted. In JavaScript, object serialization is commonly used to send data over the network or to store it in a file. It allows us to easily recreate an object from its serialized form.
There are different ways to serialize objects in JavaScript, but two of the most commonly used methods are JSON (JavaScript Object Notation) and the JSON.stringify()
method.
JSON Serialization
JSON is a lightweight data interchange format that is easy for humans to read and write. It is widely supported by various programming languages, making it an excellent choice for object serialization.
To serialize an object using JSON, you can simply call the JSON.stringify()
method and pass the object as a parameter. This method converts the object into a JSON string representation. Here’s an example:
const person = { name: "John", age: 30, city: "New York" }; const serializedPerson = JSON.stringify(person); console.log(serializedPerson); // Output: {"name":"John","age":30,"city":"New York"}
In the example above, the person
object is serialized into a JSON string representation using JSON.stringify()
. The resulting string can be easily transmitted or stored as needed.
Deserialization
Deserialization is the process of recreating an object from its serialized form. In JavaScript, we can deserialize a JSON string back into an object using the JSON.parse()
method.
Here’s an example:
const serializedPerson = '{"name":"John","age":30,"city":"New York"}'; const person = JSON.parse(serializedPerson); console.log(person); // Output: { name: 'John', age: 30, city: 'New York' }
In the example above, the serializedPerson
JSON string is deserialized using JSON.parse()
, and the resulting object is stored in the person
variable.
Related Article: How to Extract Values from Arrays in JavaScript
Limitations of JSON Serialization
While JSON serialization is widely supported and easy to use, there are some limitations to be aware of. JSON cannot handle circular references, which occur when an object references itself. If you try to serialize an object with a circular reference, an error will occur.
Also, JSON cannot serialize functions or undefined values. Any functions or undefined values within an object will be omitted during serialization.
Other Serialization Methods
In addition to JSON serialization, there are other methods available in JavaScript for object serialization. Some of these methods include using the toString()
method, which converts an object into a string representation, and using the XMLHttpRequest
object to send objects over the network.
However, JSON serialization is the most commonly used method due to its simplicity, widespread support, and ability to handle complex data structures.
In this chapter, you learned about object serialization and specifically how to serialize objects using JSON. You also learned about deserialization and the limitations of JSON serialization. In the next chapter, we will explore object cloning and copying in JavaScript.
JSON.stringify() and JSON.parse()
JavaScript Object Notation (JSON) is a popular data interchange format that is used to represent structured data. It is commonly used to transmit data between a server and a web application, as it is lightweight and easy to parse.
JSON.stringify() is a method in JavaScript that converts a JavaScript object or value into a JSON string. This is useful when you want to send data to a server or store it in a file. Let’s take a look at an example:
const person = { name: "John", age: 30, city: "New York" }; const jsonStr = JSON.stringify(person); console.log(jsonStr);
Output:
{"name":"John","age":30,"city":"New York"}
In the above example, the person
object is converted into a JSON string using the JSON.stringify() method. The resulting JSON string represents the object’s properties and values.
It is important to note that JSON.stringify() will only include enumerable properties in the JSON string. Non-enumerable properties, such as methods defined on an object’s prototype, will be ignored.
JSON.parse() is the counterpart to JSON.stringify(). It takes a JSON string and converts it into a JavaScript object or value. This is useful when you receive data from a server or retrieve it from a file. Let’s see an example:
const jsonStr = '{"name":"John","age":30,"city":"New York"}'; const person = JSON.parse(jsonStr); console.log(person);
Output:
{ name: 'John', age: 30, city: 'New York' }
In the above example, the JSON string jsonStr
is parsed using the JSON.parse() method, and the resulting object is stored in the person
variable.
It’s important to ensure that the JSON string is valid before using JSON.parse(). Otherwise, it will throw an error. JSON strings must be well-formed and adhere to the JSON specification.
JSON.stringify() and JSON.parse() are powerful methods that allow you to convert JavaScript objects to JSON strings and vice versa. They provide a convenient way to transmit and store structured data, making them essential tools in web development.
To learn more about JSON.stringify() and JSON.parse(), refer to the official documentation:
– JSON.stringify()
– JSON.parse()
Related Article: JavaScript Prototype Inheritance Explained (with Examples)
Working with Nested Objects
In JavaScript, objects can contain other objects, creating what is called a nested object structure. This allows you to organize and access data in a hierarchical manner. Working with nested objects involves accessing and modifying properties within the nested structure.
To access properties in a nested object, you can use dot notation or bracket notation. Dot notation is used when you know the property name beforehand, while bracket notation is used when the property name is dynamic or when it contains special characters.
Let’s consider an example of a nested object representing a person’s information:
const person = { name: 'John', age: 30, address: { street: '123 Main St', city: 'New York', state: 'NY' } };
To access the person’s name, we can use dot notation:
console.log(person.name); // Output: John
If we want to access the person’s city, we can use dot notation for the outer object and then dot notation again for the nested object:
console.log(person.address.city); // Output: New York
Alternatively, we can use bracket notation to access the city property:
console.log(person['address']['city']); // Output: New York
To modify properties within a nested object, you can follow a similar approach. For example, to update the person’s age:
person.age = 35; console.log(person.age); // Output: 35
To add a new property to the nested object, you can use dot notation or bracket notation:
person.address.zipCode = '10001'; console.log(person.address.zipCode); // Output: 10001
Nested objects can also be iterated over using loops, such as the for…in loop. This allows you to perform operations on each property within the nested structure.
for (let key in person) { if (typeof person[key] === 'object') { for (let nestedKey in person[key]) { console.log(`${nestedKey}: ${person[key][nestedKey]}`); } } else { console.log(`${key}: ${person[key]}`); } }
This loop will output all the properties and their values in the nested object.
Working with nested objects can be powerful when dealing with complex data structures. It allows you to organize and access data in a convenient and hierarchical manner, making your code more readable and maintainable.
To learn more about JavaScript objects, you can refer to the MDN documentation on working with objects.
Object Equality: Comparing Objects
When working with JavaScript objects, you may come across situations where you need to compare two objects to check if they are equal or not. However, comparing objects in JavaScript is not as straightforward as comparing primitive data types like numbers or strings.
In JavaScript, objects are reference types, which means that when you create an object and assign it to a variable, the variable holds a reference to the object in memory. When you compare two objects using the equality operator (==
or ===
), you are actually comparing the references, not the actual objects.
Let’s consider an example to understand this better:
const person1 = { name: "John", age: 30 }; const person2 = { name: "John", age: 30 }; console.log(person1 === person2); // false console.log(person1 == person2); // false
In the above example, even though person1
and person2
have the same properties and values, the comparison using the equality operator returns false
. This is because they are two different objects with different references in memory.
If you want to compare the properties and values of two objects, you need to do a deep comparison. One way to achieve this is by manually comparing each property using a loop or a library like lodash. However, this can become cumbersome and error-prone, especially if the objects have nested properties.
Another approach is to use the JSON.stringify()
method to convert the objects to JSON strings and then compare the strings. This method works well for simple objects without circular references or functions as properties.
Let’s see an example of comparing objects using JSON.stringify()
:
const person1 = { name: "John", age: 30 }; const person2 = { name: "John", age: 30 }; console.log(JSON.stringify(person1) === JSON.stringify(person2)); // true
In this example, we convert both person1
and person2
objects to JSON strings using JSON.stringify()
and then compare the strings using the equality operator. This time the comparison returns true
because the properties and values are the same.
It’s important to note that this approach has limitations. It does not work for objects with circular references or functions as properties. Additionally, it does not consider the order of properties, as JSON strings are represented as key-value pairs.
In some cases, you may need to perform a shallow comparison, where only the references of the top-level properties are compared. For this, you can use the Object.is()
method, which compares two values for equality.
Let’s see an example of using Object.is()
to compare objects:
const person1 = { name: "John", age: 30 }; const person2 = { name: "John", age: 30 }; console.log(Object.is(person1, person2)); // false
In this example, Object.is()
compares the references of person1
and person2
and returns false
because they are different objects.
Comparing objects in JavaScript can be tricky due to their reference nature. To compare objects for equality, you can use deep comparison techniques like JSON.stringify()
or shallow comparison using Object.is()
. However, it’s important to consider the limitations and potential issues with these approaches depending on the complexity of the objects being compared.
Object.freeze() and Object.seal()
In JavaScript, objects are mutable by default, meaning that their properties can be added, modified, or deleted at any time. However, in certain cases, you may want to prevent any changes to an object or its properties. JavaScript provides two methods, Object.freeze()
and Object.seal()
, that allow you to achieve this level of immutability.
Related Article: JavaScript Arrays: Learn Array Slice, Array Reduce, and String to Array Conversion
Object.freeze()
The Object.freeze()
method is used to freeze an object, making it immutable. Once an object is frozen, you cannot add, modify, or delete any of its properties. Any attempt to do so will simply be ignored or result in an error in strict mode.
Here’s an example of how to use Object.freeze()
:
const person = { name: 'John', age: 30 }; Object.freeze(person); person.age = 40; // This will be ignored person.address = '123 Main St'; // This will be ignored console.log(person); // Output: { name: 'John', age: 30 }
In the above example, the person
object is frozen using Object.freeze()
. As a result, the attempts to update the age
property to 40 and add the address
property are ignored.
It’s important to note that Object.freeze()
only freezes the top-level properties of an object. If an object contains nested objects, those nested objects are not automatically frozen and can still be modified.
Object.seal()
The Object.seal()
method is similar to Object.freeze()
in that it prevents the addition and deletion of properties. However, it allows you to modify the values of existing properties.
Here’s an example of how to use Object.seal()
:
const car = { make: 'Toyota', model: 'Camry', year: 2021 }; Object.seal(car); car.year = 2022; // This is allowed car.color = 'blue'; // This will be ignored console.log(car); // Output: { make: 'Toyota', model: 'Camry', year: 2022 }
In the above example, the car
object is sealed using Object.seal()
. This prevents the addition of the color
property, but allows the modification of the year
property.
Similar to Object.freeze()
, Object.seal()
only seals the top-level properties of an object and does not seal nested objects.
Both Object.freeze()
and Object.seal()
are useful when you want to ensure that an object’s properties remain unchanged. They provide different levels of immutability, allowing you to choose the one that best fits your needs.
In the next chapter, we will explore another important concept related to JavaScript objects: object cloning.
Garbage Collection: Memory Management
JavaScript is a high-level, garbage-collected programming language. This means that memory management is taken care of automatically by the JavaScript engine, allowing developers to focus on writing code without worrying about memory allocation and deallocation.
Garbage collection is the process of automatically reclaiming memory that is no longer needed by a program. In JavaScript, this is done by the JavaScript engine’s garbage collector. The garbage collector keeps track of all objects created by the program and periodically identifies and frees up memory that is no longer being used.
There are different garbage collection algorithms used by different JavaScript engines, but they all have the same goal: to identify and remove objects from memory that are no longer reachable. An object is considered reachable if it is still referenced by another object or by the program’s execution context. If an object is no longer reachable, it is considered garbage and can be safely removed from memory.
Let’s take a look at a simple example to understand how garbage collection works in JavaScript:
function createObject() { var obj = {}; return obj; } var obj1 = createObject(); var obj2 = createObject(); obj1 = null;
In this example, the createObject
function creates a new object and returns it. We then call this function twice and assign the returned objects to obj1
and obj2
. After that, we set obj1
to null
.
At this point, the object originally referenced by obj1
is no longer reachable, as there are no more references to it. The garbage collector will identify this object as garbage and free up the memory it was occupying.
It’s important to note that the exact timing of when garbage collection occurs is implementation-dependent and varies between JavaScript engines. However, most engines employ an algorithm called “mark and sweep.” This algorithm traverses all reachable objects starting from a set of root objects (such as global variables, function scopes, and object properties), marking them as reachable. Any objects that are not marked as reachable are considered garbage and are subsequently removed from memory.
Understanding how garbage collection works in JavaScript can help developers write more efficient and memory-friendly code. It is important to avoid unnecessary object references and to be mindful of object lifecycles to prevent memory leaks.
In this chapter, we’ve explored the concept of garbage collection and how it is used for memory management in JavaScript. The JavaScript engine’s garbage collector automatically takes care of freeing up memory that is no longer needed by the program. Being aware of how garbage collection works can help you write more efficient and reliable JavaScript code.
Getters and Setters: Accessing and Modifying Properties
JavaScript objects have properties that hold values, but sometimes we need more control over how these properties are accessed or modified. This is where getters and setters come into play.
Getters
A getter is a special function that allows us to access the value of a property. It is defined using the get
keyword followed by the property name. Let’s see an example:
const person = { firstName: 'John', lastName: 'Doe', get fullName() { return this.firstName + ' ' + this.lastName; } }; console.log(person.fullName); // Output: John Doe
In the code snippet above, we define a fullName
getter on the person
object. When we access person.fullName
, the getter function is automatically called and returns the concatenated value of firstName
and lastName
.
Getters are useful when we want to perform some calculations or manipulations before returning the value of a property. They provide a way to retrieve a property’s value dynamically.
Setters
While getters allow us to retrieve the value of a property, setters enable us to modify the value of a property. Similar to getters, setters are defined using the set
keyword followed by the property name. Let’s take a look at an example:
const person = { firstName: 'John', lastName: 'Doe', set fullName(name) { const names = name.split(' '); this.firstName = names[0]; this.lastName = names[1]; } }; person.fullName = 'Jane Smith'; console.log(person.firstName); // Output: Jane console.log(person.lastName); // Output: Smith
In the code snippet above, we define a fullName
setter on the person
object. When we assign a value to person.fullName
, the setter function is automatically called with the assigned value as its argument. In this case, the setter splits the assigned value into first and last names, and then updates the firstName
and lastName
properties accordingly.
Setters allow us to perform validation or additional logic when setting the value of a property. They provide a way to control the modification of a property’s value.
Using Getters and Setters
Getters and setters can be used together to create a more controlled interface for accessing and modifying properties. Let’s see an example:
const person = { firstName: 'John', lastName: 'Doe', get fullName() { return this.firstName + ' ' + this.lastName; }, set fullName(name) { const names = name.split(' '); this.firstName = names[0]; this.lastName = names[1]; } }; console.log(person.fullName); // Output: John Doe person.fullName = 'Jane Smith'; console.log(person.firstName); // Output: Jane console.log(person.lastName); // Output: Smith
In the code snippet above, we define both a fullName
getter and setter on the person
object. The getter allows us to retrieve the full name, while the setter allows us to set the full name by splitting it into first and last names.
Getters and setters provide a way to encapsulate the logic of accessing and modifying properties, allowing us to define custom behavior for our objects.
In this chapter, we explored the concept of getters and setters in JavaScript objects. Getters enable us to retrieve the value of a property with custom logic, while setters allow us to modify the value of a property with validation or additional logic. By using getters and setters, we can create more controlled interfaces for accessing and modifying object properties.
Real World Examples: Creating a To-Do List App
In this chapter, we will explore a real-world example of creating a to-do list app using JavaScript objects. This example will help us understand how objects can be used to organize and manage data in a practical application.
To start with, let’s define the structure of our to-do list app. We can represent each task as an object with properties such as a unique identifier, a description of the task, and a boolean value indicating whether the task is completed or not.
const task1 = { id: 1, description: 'Buy groceries', completed: false }; const task2 = { id: 2, description: 'Do laundry', completed: true };
In the above code snippet, we have created two tasks using JavaScript objects. The id
property serves as a unique identifier for each task, while the description
property holds the task description, and the completed
property determines whether the task is completed or not.
We can then create an array to store all the tasks in our to-do list.
const tasks = [task1, task2];
Now, let’s say we want to display all the tasks in our to-do list. We can use a loop to iterate over the tasks
array and display the information for each task.
for (let i = 0; i task.id === taskId); if (task) { task.completed = true; } } completeTask(1); // Mark task with ID 1 as completed
In the above code snippet, we use the find
method to search for the task with the given ID in the tasks
array. If a matching task is found, we update its completed
property to true
. In this example, we mark the task with ID 1 as completed.
These are just a few examples of how JavaScript objects can be used to create a to-do list app. With objects, we can easily organize and manage data in a structured manner, making it easier to build complex applications.
In the next chapter, we will explore advanced object concepts such as inheritance and prototypes. Stay tuned!
Real World Examples: Building a User Authentication System
In this chapter, we will explore a real-world example of using JavaScript objects to build a user authentication system. User authentication is a crucial aspect of many web applications, as it allows users to securely access their accounts and protect their personal information.
To demonstrate this concept, we will create a simple user authentication system using JavaScript objects. This system will allow users to register for an account, log in, and log out.
Let’s start by defining a User class that will serve as the blueprint for our user objects. Each user will have a unique username and password.
class User { constructor(username, password) { this.username = username; this.password = password; } login() { // Logic for logging in a user } logout() { // Logic for logging out a user } }
Next, we can create an instance of the User class for each registered user. We will store these instances in an array called users
.
const users = []; // Register a new user function registerUser(username, password) { const newUser = new User(username, password); users.push(newUser); }
To allow users to log in, we need to implement the login()
method of the User class. This method will compare the provided username and password with the ones stored in the users
array.
User.prototype.login = function(username, password) { const user = users.find(user => user.username === username && user.password === password); if (user) { console.log('Login successful!'); } else { console.log('Invalid username or password.'); } }
Finally, to log out a user, we can simply remove the user’s session information.
User.prototype.logout = function() { // Logic for logging out a user }
With these simple examples, we have built a basic user authentication system using JavaScript objects. Of course, in a real-world application, we would need to consider additional security measures, such as encrypting passwords and implementing session management.
In the next chapter, we will explore more advanced concepts related to JavaScript objects, including inheritance and prototypes. Stay tuned for more exciting examples and explanations!
Real World Examples: Implementing a Shopping Cart
In this chapter, we will explore a real-world example of implementing a shopping cart using JavaScript objects. A shopping cart is a common feature in e-commerce websites that allows users to add products to their cart and proceed to checkout.
To implement a shopping cart, we can use JavaScript objects to represent the cart and the products. Each product can be represented as an object with properties such as name, price, and quantity. The cart can be represented as an object that contains an array of products.
Let’s start by creating a Product object:
function Product(name, price, quantity) { this.name = name; this.price = price; this.quantity = quantity; }
Next, let’s create a Cart object that will contain an array of products:
function Cart() { this.products = []; }
To add a product to the cart, we can define a method called addProduct
on the Cart object:
Cart.prototype.addProduct = function(product) { this.products.push(product); };
To calculate the total price of the products in the cart, we can define a method called getTotalPrice
on the Cart object:
Cart.prototype.getTotalPrice = function() { let totalPrice = 0; for (let i = 0; i < this.products.length; i++) { totalPrice += this.products[i].price * this.products[i].quantity; } return totalPrice; };
Now, let's create some products and add them to the cart:
const product1 = new Product("iPhone", 999, 1); const product2 = new Product("MacBook", 1999, 1); const cart = new Cart(); cart.addProduct(product1); cart.addProduct(product2);
We can now calculate the total price of the products in the cart:
console.log(cart.getTotalPrice()); // Output: 2998
This is a simple example of implementing a shopping cart using JavaScript objects. In a real-world scenario, you would also need to implement functionalities such as removing products from the cart, updating quantities, and handling the checkout process.
By using JavaScript objects, you can easily manage and manipulate data in your shopping cart, making it a crucial tool for building e-commerce websites.
Real World Examples: Creating an Address Book
In this chapter, we will explore a real-world example of using JavaScript objects by creating an address book. An address book is a common application that allows users to store and manage their contacts’ information, such as names, phone numbers, and addresses.
To begin, let’s create a simple address book object using the object literal syntax:
const addressBook = { contacts: [], addContact: function(name, phoneNumber, address) { this.contacts.push({ name, phoneNumber, address }); }, getContact: function(name) { return this.contacts.find(contact => contact.name === name); }, deleteContact: function(name) { const index = this.contacts.findIndex(contact => contact.name === name); if (index !== -1) { this.contacts.splice(index, 1); } } };
In the code above, we define an addressBook
object with three methods: addContact
, getContact
, and deleteContact
. The contacts
property is an array that will store all the contacts.
The addContact
method takes in the contact’s name, phone number, and address as arguments and adds a new contact object to the contacts
array.
The getContact
method takes in the contact’s name as an argument and returns the contact object that matches the given name.
The deleteContact
method takes in the contact’s name as an argument and removes the contact object from the contacts
array.
Let’s now use our address book object to perform some operations:
addressBook.addContact("John Doe", "123-456-7890", "123 Main St"); addressBook.addContact("Jane Smith", "987-654-3210", "456 Elm St"); console.log(addressBook.getContact("John Doe")); // { name: "John Doe", phoneNumber: "123-456-7890", address: "123 Main St" } addressBook.deleteContact("Jane Smith"); console.log(addressBook.contacts); // [{ name: "John Doe", phoneNumber: "123-456-7890", address: "123 Main St" }]
In the code above, we add two contacts to the address book using the addContact
method. We then retrieve the contact object for “John Doe” using the getContact
method and log it to the console. Finally, we delete the contact for “Jane Smith” using the deleteContact
method and log the contacts
array to the console to verify the deletion.
This is just a simple example of how JavaScript objects can be used to create an address book. In a real-world application, you would likely have more advanced features such as searching, sorting, and updating contacts. Nonetheless, this example should give you a good starting point for understanding the basics of using objects in JavaScript.
In the next chapter, we will explore object constructors and prototypes, which provide a more flexible and efficient way to create objects with shared properties and methods.
Advanced Techniques: Object Proxy
JavaScript objects are versatile and powerful, but sometimes you may need more control over their behavior. This is where object proxies come in handy.
An object proxy is an intermediary between a JavaScript object and the code that interacts with it. It allows you to intercept and customize various operations, such as property access, property assignment, function invocation, and more. With object proxies, you can implement advanced features like lazy loading, data validation, and access control.
To create a proxy, you use the Proxy
constructor, which takes two arguments: the target object and a handler object. The target object is the object you want to proxy, and the handler object contains the traps, which are methods that get called when certain operations are performed on the proxy.
Here’s a simple example that demonstrates how to create a proxy for an object:
const target = { name: 'John', age: 30 }; const handler = { get(target, property) { console.log(`Getting property '${property}'`); return target[property]; }, set(target, property, value) { console.log(`Setting property '${property}' to '${value}'`); target[property] = value; } }; const proxy = new Proxy(target, handler); console.log(proxy.name); // Output: Getting property 'name', John proxy.age = 35; // Output: Setting property 'age' to '35' console.log(proxy.age); // Output: Getting property 'age', 35
In this example, the get
trap is called whenever a property is accessed on the proxy, and the set
trap is called whenever a property is assigned a value. The traps can intercept and modify the behavior of these operations.
You can customize other operations by adding more traps to the handler object. Some of the available traps include has
for the in
operator, deleteProperty
for the delete
operator, apply
for function invocation, and many more. The full list of traps can be found in the MDN documentation.
One useful application of object proxies is to implement data validation. You can use the set
trap to validate the value being assigned to a property and prevent invalid values from being set. Here’s an example:
const target = { age: 30 }; const handler = { set(target, property, value) { if (property === 'age' && value < 0) { throw new Error('Age must be a positive number'); } target[property] = value; } }; const proxy = new Proxy(target, handler); proxy.age = -10; // Throws an error: Age must be a positive number
In this example, the set
trap checks if the property being set is age
and if the value is less than 0. If it is, an error is thrown, preventing the assignment of an invalid value.
Object proxies provide a powerful mechanism to customize and control the behavior of JavaScript objects. They can be used to implement a wide range of advanced features and ensure data integrity and security. However, it's important to use them judiciously, as they can add complexity to your code if misused.
Remember to check the MDN documentation for more information on object proxies and their available traps.
Advanced Techniques: Object Reflection
Object reflection is a powerful feature of JavaScript that allows you to inspect and manipulate objects dynamically at runtime. It provides the ability to examine an object’s properties and methods, as well as modify or add new ones.
1. Object.keys()
The Object.keys()
method returns an array of a given object’s own enumerable property names. This can be useful when you need to iterate over an object’s properties or perform operations based on its keys:
const person = { name: 'John Doe', age: 30, occupation: 'Engineer' }; const keys = Object.keys(person); console.log(keys); // Output: ['name', 'age', 'occupation']
2. Object.values()
Similar to Object.keys()
, the Object.values()
method returns an array of a given object’s own enumerable property values. It can be handy when you want to extract the values of an object’s properties:
const person = { name: 'John Doe', age: 30, occupation: 'Engineer' }; const values = Object.values(person); console.log(values); // Output: ['John Doe', 30, 'Engineer']
3. Object.entries()
The Object.entries()
method returns an array of a given object’s own enumerable property key-value pairs as arrays. This can be useful when you need to iterate over both the keys and values of an object:
const person = { name: 'John Doe', age: 30, occupation: 'Engineer' }; const entries = Object.entries(person); console.log(entries); // Output: [['name', 'John Doe'], ['age', 30], ['occupation', 'Engineer']]
4. Object.getOwnPropertyNames()
The Object.getOwnPropertyNames()
method returns an array of all properties (including non-enumerable properties) found directly on a given object. It can be helpful when you need to access all properties of an object, regardless of their enumerability:
const person = { name: 'John Doe', age: 30 }; Object.defineProperty(person, 'occupation', { value: 'Engineer', enumerable: false }); const properties = Object.getOwnPropertyNames(person); console.log(properties); // Output: ['name', 'age', 'occupation']
5. Object.getOwnPropertyDescriptor()
The Object.getOwnPropertyDescriptor()
method returns an object describing the configuration of a specific property in an object. It provides information about various attributes of the property, such as its value, writability, and enumerability:
const person = { name: 'John Doe', age: 30 }; const propertyDescriptor = Object.getOwnPropertyDescriptor(person, 'name'); console.log(propertyDescriptor); // Output: { // value: 'John Doe', // writable: true, // enumerable: true, // configurable: true // }
These are just a few examples of the powerful object reflection capabilities available in JavaScript. Understanding and utilizing these techniques can greatly enhance your ability to work with and manipulate objects dynamically.