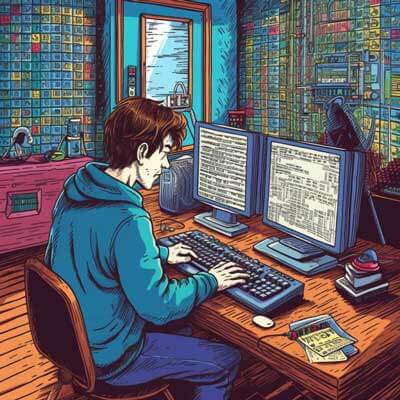
- Date Subtraction in PostgreSQL
- Finding the Difference Between Two Dates in PostgreSQL
- Date Manipulation Functions in PostgreSQL
- Performing Date Arithmetic in PostgreSQL
- Adding Days to a Date in PostgreSQL
- Adding Months to a Date in PostgreSQL
- Adding Years to a Date in PostgreSQL
- Common Date Operations in PostgreSQL
- Calculating Dates in PostgreSQL
- Syntax for Adding or Subtracting Dates in PostgreSQL
- Additional Resources
Date Subtraction in PostgreSQL
In PostgreSQL, you can subtract dates using the “-” operator. This allows you to find the difference between two dates and get the result in various units such as days, months, or years. Here are two examples of how to subtract dates in PostgreSQL:
Example 1: Subtracting two dates to get the number of days
SELECT '2022-01-01'::DATE - '2021-12-25'::DATE AS days_diff;
This will return the result as the number of days between the two dates:
days_diff ---------- 6
Example 2: Subtracting two dates to get the number of months
SELECT EXTRACT(MONTH FROM '2022-01-01'::DATE) - EXTRACT(MONTH FROM '2021-12-25'::DATE) AS months_diff;
This will return the result as the number of months between the two dates:
months_diff ------------ 0
Related Article: How to Compare & Manipulate Dates in PostgreSQL
Finding the Difference Between Two Dates in PostgreSQL
To find the difference between two dates in PostgreSQL, you can use the AGE
function. The AGE
function calculates the interval between two dates and returns the result as an interval type. Here are two examples of how to use the AGE
function to find the difference between two dates:
Example 1: Finding the difference between two dates in days
SELECT AGE('2022-01-01'::DATE, '2021-12-25'::DATE) AS days_diff;
This will return the result as an interval with the number of days:
days_diff ---------- 6 days
Example 2: Finding the difference between two dates in years
SELECT AGE('2022-01-01'::DATE, '1990-01-01'::DATE) / INTERVAL '1 year' AS years_diff;
This will return the result as a numeric value representing the number of years:
years_diff ----------- 32
Date Manipulation Functions in PostgreSQL
PostgreSQL provides various date manipulation functions that allow you to perform operations on dates. These functions can be used to add or subtract days, months, or years from a date, extract specific parts of a date, and perform other date-related operations. Here are some commonly used date manipulation functions in PostgreSQL:
– date_part(unit, date)
: This function extracts the specified part (unit) from a date. The unit can be ‘year’, ‘month’, ‘day’, ‘hour’, ‘minute’, ‘second’, etc. For example:
SELECT date_part('year', '2022-01-01'::DATE) AS year_part;
This will return the year part of the date:
year_part --------- 2022
– date_trunc(unit, date)
: This function truncates the specified part (unit) of a date, setting the rest of the parts to zero or the minimum value. The unit can be ‘year’, ‘month’, ‘day’, ‘hour’, ‘minute’, ‘second’, etc. For example:
SELECT date_trunc('month', '2022-01-15'::DATE) AS truncated_date;
This will return the date with the day part set to the minimum value of the month:
truncated_date --------------------- 2022-01-01 00:00:00
– age(date1, date2)
: This function calculates the interval between two dates and returns the result as an interval type. For example:
SELECT age('2022-01-01'::DATE, '1990-01-01'::DATE) AS age_interval;
This will return the interval between the two dates:
age_interval ------------ 32 years
Performing Date Arithmetic in PostgreSQL
In PostgreSQL, you can perform date arithmetic by adding or subtracting intervals to or from dates. This allows you to add or subtract a specific number of days, months, or years from a date. Here are two examples of how to perform date arithmetic in PostgreSQL:
Example 1: Adding days to a date
SELECT '2022-01-01'::DATE + INTERVAL '7 days' AS new_date;
This will add 7 days to the given date and return the new date:
new_date ------------ 2022-01-08
Example 2: Subtracting months from a date
SELECT '2022-01-01'::DATE - INTERVAL '1 month' AS new_date;
This will subtract 1 month from the given date and return the new date:
new_date ------------ 2021-12-01
Related Article: Extracting the Month from a Date in PostgreSQL
Adding Days to a Date in PostgreSQL
To add days to a date in PostgreSQL, you can use the “+” operator or the INTERVAL
keyword. Here are two examples of how to add days to a date in PostgreSQL:
Example 1: Using the “+” operator
SELECT '2022-01-01'::DATE + 7 AS new_date;
This will add 7 days to the given date and return the new date:
new_date ------------ 2022-01-08
Example 2: Using the INTERVAL
keyword
SELECT '2022-01-01'::DATE + INTERVAL '7 days' AS new_date;
This will add 7 days to the given date and return the new date:
new_date ------------ 2022-01-08
Adding Months to a Date in PostgreSQL
To add months to a date in PostgreSQL, you can use the “+” operator or the INTERVAL
keyword. Here are two examples of how to add months to a date in PostgreSQL:
Example 1: Using the “+” operator
SELECT '2022-01-01'::DATE + INTERVAL '1 month' AS new_date;
This will add 1 month to the given date and return the new date:
new_date ------------ 2022-02-01
Example 2: Using the INTERVAL
keyword
SELECT '2022-01-01'::DATE + INTERVAL '1 month' AS new_date;
This will add 1 month to the given date and return the new date:
new_date ------------ 2022-02-01
Adding Years to a Date in PostgreSQL
To add years to a date in PostgreSQL, you can use the “+” operator or the INTERVAL
keyword. Here are two examples of how to add years to a date in PostgreSQL:
Example 1: Using the “+” operator
SELECT '2022-01-01'::DATE + INTERVAL '1 year' AS new_date;
This will add 1 year to the given date and return the new date:
new_date ------------ 2023-01-01
Example 2: Using the INTERVAL
keyword
SELECT '2022-01-01'::DATE + INTERVAL '1 year' AS new_date;
This will add 1 year to the given date and return the new date:
new_date ------------ 2023-01-01
Related Article: Working With PostgreSQL: Extracting Day of Week
Common Date Operations in PostgreSQL
PostgreSQL provides a wide range of date operations that can be used to manipulate and work with dates. Here are some common date operations in PostgreSQL:
– Comparing dates: You can compare dates using comparison operators such as “=”, “”, “=”, etc. For example:
SELECT * FROM table_name WHERE date_column > '2022-01-01'::DATE;
This will select all records where the date column is greater than the specified date.
– Formatting dates: You can format dates using the TO_CHAR
function. This function allows you to convert a date into a specific format. For example:
SELECT TO_CHAR('2022-01-01'::DATE, 'YYYY-MM-DD') AS formatted_date;
This will return the date in the specified format:
formatted_date -------------- 2022-01-01
– Extracting parts of a date: You can extract specific parts (year, month, day, hour, minute, second, etc.) from a date using the EXTRACT
function. For example:
SELECT EXTRACT(YEAR FROM '2022-01-01'::DATE) AS year_part;
This will return the year part of the date:
year_part --------- 2022
Calculating Dates in PostgreSQL
In PostgreSQL, you can perform various calculations on dates using arithmetic operations and date functions. Here are some examples of how to calculate dates in PostgreSQL:
Example 1: Calculating the end date given a start date and a duration in days
SELECT '2022-01-01'::DATE + INTERVAL '7 days' AS end_date;
This will add 7 days to the start date and return the end date:
end_date ------------ 2022-01-08
Example 2: Calculating the start date given an end date and a duration in months
SELECT '2022-01-01'::DATE - INTERVAL '1 month' AS start_date;
This will subtract 1 month from the end date and return the start date:
start_date ------------ 2021-12-01
Syntax for Adding or Subtracting Dates in PostgreSQL
To add or subtract dates in PostgreSQL, you can use the “+” or “-” operator or the INTERVAL
keyword. Here is the syntax for adding or subtracting dates in PostgreSQL:
Adding days:
date + INTERVAL 'n days'
Subtracting days:
date - INTERVAL 'n days'
Adding months:
date + INTERVAL 'n months'
Subtracting months:
date - INTERVAL 'n months'
Adding years:
date + INTERVAL 'n years'
Subtracting years:
date - INTERVAL 'n years'
Note: Replace date
with the actual date value and n
with the number of days, months, or years you want to add or subtract.
For example, to add 7 days to a date:
SELECT date + INTERVAL '7 days' AS new_date;
To subtract 1 month from a date:
SELECT date - INTERVAL '1 month' AS new_date;
To add 1 year to a date:
SELECT date + INTERVAL '1 year' AS new_date;
Related Article: How to Set Timestamps With & Without Time Zone in PostgreSQL