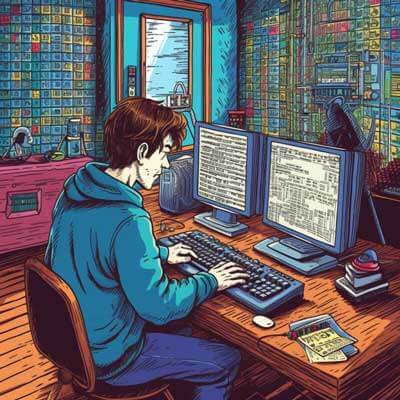
Table of Contents
How do I schedule a bash script for MySQL database backup?
Scheduling a bash script for MySQL database backup is essential to ensure regular backups of your database. One commonly used tool for scheduling tasks in Linux is cron. Cron is a time-based job scheduler that allows you to automate tasks at specific intervals.
To schedule a bash script for MySQL database backup using cron, you need to follow these steps:
1. Open a terminal and type the following command to edit the crontab file:
crontab -e
2. This will open the crontab file in your default text editor. Each line in the file represents a task to be executed by cron. To schedule a bash script for MySQL database backup, you need to add a new line to the crontab file.
3. The syntax for scheduling a task in cron is as follows:
* * * * * command
The five asterisks represent the time and date parameters for the task. Each asterisk can be replaced with a specific value or a range of values. Here's what each asterisk represents:
- Minute (0-59)
- Hour (0-23)
- Day of the month (1-31)
- Month (1-12)
- Day of the week (0-7, where both 0 and 7 represent Sunday)
For example, to schedule a bash script for MySQL database backup to run every day at 2:00 AM, you can use the following cron syntax:
0 2 * * * /path/to/backup_script.sh
4. Replace "/path/to/backup_script.sh" with the actual path to your bash script for MySQL database backup.
5. Save the crontab file and exit the text editor.
Now, the bash script for MySQL database backup will be executed automatically according to the schedule specified in the crontab file.
Related Article: Analyzing SQL Join and Its Effect on Records
What is the syntax for creating a bash script for MySQL database backup?
To create a bash script for MySQL database backup, you need to follow these steps:
1. Open a text editor and create a new file. You can name it "backup_script.sh" or any other name you prefer.
2. Start the script by specifying the interpreter, which is bash in this case. Add the following line at the beginning of the script:
#!/bin/bash
3. Next, you need to define the variables for the MySQL database connection and backup settings. Here's an example of how to define these variables in the script:
# Database connection settings DB_HOST="localhost" DB_USER="root" DB_PASSWORD="password" DB_NAME="mydatabase" # Backup settings BACKUP_DIR="/path/to/backup/directory" BACKUP_FILENAME="backup_$(date +%Y%m%d_%H%M%S).sql"
4. In the script, you can use the mysqldump command to create a backup of the MySQL database. Here's an example of how to use the mysqldump command in the script:
# Create a backup of the MySQL database mysqldump --host=$DB_HOST --user=$DB_USER --password=$DB_PASSWORD $DB_NAME > $BACKUP_DIR/$BACKUP_FILENAME
5. Save the script and make it executable by running the following command in the terminal:
chmod +x backup_script.sh
Now, you have a bash script for MySQL database backup that can be executed manually or scheduled using cron.
How can I automate MySQL database backups using a bash script?
Automating MySQL database backups using a bash script is a convenient way to ensure that your databases are regularly backed up without manual intervention. With a bash script, you can create a backup of your MySQL database and automate the process by scheduling the script to run at specific intervals using cron.
Here's an example of how you can automate MySQL database backups using a bash script:
1. Create a bash script for MySQL database backup using the steps mentioned in the previous section.
2. Open a terminal and type the following command to edit the crontab file:
crontab -e
3. Add a new line to the crontab file to schedule the execution of the backup script. For example, to run the script every day at 2:00 AM, you can use the following cron syntax:
0 2 * * * /path/to/backup_script.sh
Make sure to replace "/path/to/backup_script.sh" with the actual path to your backup script.
4. Save the crontab file and exit the text editor.
Now, the bash script for MySQL database backup will be executed automatically according to the schedule specified in the crontab file, ensuring that your MySQL databases are regularly backed up.
Are there any best practices for writing a bash script for MySQL database backup?
When writing a bash script for MySQL database backup, it's important to follow some best practices to ensure the script is efficient, reliable, and easy to maintain. Here are some best practices to consider:
1. Use variables to store database connection and backup settings: By using variables, you can easily modify the script's behavior without having to edit the script itself. This makes it easier to update the script when there are changes to the database or backup settings.
2. Validate database connection: Before creating a backup, validate the database connection to ensure that the script can connect to the database successfully. This can help prevent errors and ensure that backups are created only when the database connection is established.
3. Handle errors and failures gracefully: Implement error handling in your script to handle any errors or failures that may occur during the backup process. This can include checking for the availability of required commands, verifying the success of commands, and logging any errors or failures for troubleshooting purposes.
4. Use compression for backups: Compressing the backup files can save disk space and make it easier to transfer and store backups. Consider using tools like gzip or tar to compress the backup files.
5. Implement rotation and retention policies: To manage disk space usage, consider implementing rotation and retention policies for backups. This can involve deleting older backups or storing backups in different directories based on their age.
6. Test the script: Before relying on the script for regular backups, test it thoroughly to ensure that it works as expected. Test different scenarios, such as database connection failures, large databases, and different backup locations.
Related Article: How to Extract Data from PostgreSQL Databases: PSQL ETL
Can I use a cron job to schedule the execution of a bash script for MySQL database backup?
Yes, you can use a cron job to schedule the execution of a bash script for MySQL database backup. Cron is a time-based job scheduler in Linux that allows you to automate tasks at specific intervals.
To schedule the execution of a bash script for MySQL database backup using cron, you need to follow these steps:
1. Open a terminal and type the following command to edit the crontab file:
crontab -e
2. This will open the crontab file in your default text editor. Each line in the file represents a task to be executed by cron. To schedule the execution of a bash script for MySQL database backup, you need to add a new line to the crontab file.
3. The syntax for scheduling a task in cron is as follows:
* * * * * command
The five asterisks represent the time and date parameters for the task. Each asterisk can be replaced with a specific value or a range of values. Here's what each asterisk represents:
- Minute (0-59)
- Hour (0-23)
- Day of the month (1-31)
- Month (1-12)
- Day of the week (0-7, where both 0 and 7 represent Sunday)
For example, to schedule the execution of a bash script for MySQL database backup to run every day at 2:00 AM, you can use the following cron syntax:
0 2 * * * /path/to/backup_script.sh
Replace "/path/to/backup_script.sh" with the actual path to your bash script for MySQL database backup.
4. Save the crontab file and exit the text editor.
Now, the bash script for MySQL database backup will be executed automatically according to the schedule specified in the crontab file.
What are the recommended tools for managing MySQL database backups?
There are several recommended tools for managing MySQL database backups. These tools provide features such as automation, scheduling, compression, and encryption to enhance the backup process. Here are some popular tools for managing MySQL database backups:
1. mysqldump: mysqldump is a command-line tool that comes with MySQL and is used to create backups of MySQL databases. It allows you to specify options such as the database to be backed up, the output file format, and the backup method (e.g., full backup or incremental backup).
2. Percona XtraBackup: Percona XtraBackup is an open-source backup utility for MySQL that performs hot backups of InnoDB, XtraDB, and MyRocks databases. It supports incremental backups, parallel backups, and streaming backups.
3. Duplicity: Duplicity is a backup tool that supports various backends, including local file storage, remote file storage (e.g., SFTP, FTP, S3), and cloud storage (e.g., Google Drive, Dropbox). It provides encryption, compression, and incremental backups.
4. Bacula: Bacula is a network backup solution that supports MySQL database backups. It offers features such as client-server architecture, backup scheduling, backup verification, and data deduplication.
5. Amanda: Amanda (Advanced Maryland Automatic Network Disk Archiver) is an open-source backup solution that supports MySQL database backups. It provides features like client-server architecture, backup scheduling, media management, and tape library support.
These tools can simplify the process of managing MySQL database backups and provide additional features to enhance the backup process. Choose the tool that best fits your requirements and preferences.
Are there any security considerations when running a bash script for MySQL database backup?
Yes, there are several security considerations to keep in mind when running a bash script for MySQL database backup. These considerations help protect sensitive data and prevent unauthorized access to the backup files and the MySQL database. Here are some security considerations:
1. Limit access to the backup script: Ensure that only authorized users have access to the backup script and the server where it is stored. Restrict file permissions to prevent unauthorized modification or execution of the script.
2. Secure the database connection credentials: Avoid hardcoding the database connection credentials in the backup script. Instead, use environment variables or other secure methods to pass the credentials to the script securely. This helps prevent accidental exposure of sensitive information.
3. Encrypt the backup files: Consider encrypting the backup files to protect them from unauthorized access. You can use tools like GPG (GNU Privacy Guard) to encrypt the backup files before storing or transferring them.
4. Secure backup storage: If the backup files are stored locally, ensure that the storage location is secure. Use appropriate file permissions and access controls to prevent unauthorized access. If the backup files are stored remotely, ensure that the remote storage system is secure and properly secured.
5. Secure the backup transfer: If the backup files are transferred to a remote location or another system, ensure that the transfer is secure. Consider using secure protocols such as SSH or HTTPS to encrypt the data during transit.
6. Regularly review and test the backup process: Regularly review and test the backup process to ensure that it is working as expected and that the backups are being created successfully. Test the restoration process to ensure that backups can be restored when needed.
Can I use a shell script instead of a bash script for MySQL database backup?
Yes, you can use a shell script instead of a bash script for MySQL database backup. Shell scripts are a generic term for scripts that are interpreted by a Unix shell, which can include a variety of shell languages such as Bash, sh, csh, and more. Bash is one of the most commonly used shell languages and is the default shell in most Linux distributions.
If you prefer to use a different shell language for your MySQL database backup script, you can modify the shebang line at the beginning of the script to specify the desired shell. For example, if you want to use the sh shell, you can change the shebang line to the following:
#!/bin/sh
The rest of the script should work as expected, assuming the necessary commands and syntax are supported by the shell language you choose.
However, it's worth noting that Bash is widely supported and offers a rich set of features and capabilities that make it suitable for most scripting tasks, including MySQL database backup. Unless you have specific requirements or constraints that necessitate using a different shell language, Bash is usually a good choice for writing a MySQL database backup script.
Related Article: Exploring SQL Join Conditions: The Role of Primary Keys
Is it possible to perform a selective backup of specific tables in a MySQL database using a bash script?
Yes, it is possible to perform a selective backup of specific tables in a MySQL database using a bash script. The mysqldump command, which is commonly used for creating backups of MySQL databases, supports options to specify the tables to be included in the backup.
To perform a selective backup of specific tables in a MySQL database using a bash script, you can modify the mysqldump command in your script to include the table names. Here's an example:
# Define the tables to be backed up TABLES="table1 table2 table3" # Create a backup of the specified tables mysqldump --host=$DB_HOST --user=$DB_USER --password=$DB_PASSWORD $DB_NAME $TABLES > $BACKUP_DIR/$BACKUP_FILENAME
In this example, the TABLES variable contains a space-separated list of table names that you want to include in the backup. The mysqldump command is then modified to include the $TABLES variable after the $DB_NAME variable.
What are the alternative methods for backing up a MySQL database?
In addition to using a bash script, there are several alternative methods for backing up a MySQL database. These methods offer different levels of automation, flexibility, and scalability. Here are some alternative methods for backing up a MySQL database:
1. GUI tools: There are various GUI tools available that provide a user-friendly interface for managing MySQL database backups. These tools often offer features such as scheduling backups, selecting specific tables or databases, compression, and encryption. Examples of popular GUI tools for MySQL database backups include phpMyAdmin, MySQL Workbench, and Navicat.
2. Database management systems: Many database management systems (DBMS) provide built-in backup and restore functionality. For example, MySQL Enterprise Edition includes MySQL Enterprise Backup, which offers advanced backup features such as hot backups, point-in-time recovery, and incremental backups. Other DBMS, such as PostgreSQL, also provide built-in backup tools.
3. Replication: MySQL replication can be used as a backup mechanism by setting up a replica (slave) server that mirrors the data from the primary (master) server. The replica server can be used for backups without impacting the performance of the primary server. In case of data loss or corruption on the primary server, the replica server can be promoted to the primary server.
4. Cloud backup services: Cloud backup services provide an offsite backup solution for MySQL databases. These services often offer automated backups, scalability, and easy restoration options. Examples of cloud backup services that support MySQL databases include Amazon RDS, Google Cloud SQL, and Azure Database for MySQL.
5. Storage snapshots: Some storage systems and cloud platforms provide snapshot functionality, which allows you to create point-in-time copies of your MySQL database. These snapshots can be used as backups and provide fast and efficient restoration options. However, it's important to carefully consider the limitations and costs associated with storage snapshots.
6. Replication to external storage: You can set up replication from your MySQL database to external storage systems such as object storage or distributed file systems. This approach allows you to leverage the scalability and durability of these storage systems for backups.
Each alternative method has its own advantages and disadvantages, and the best choice depends on factors such as your specific requirements, the size of your database, the level of automation desired, and the available resources. It's important to evaluate these factors and choose the method that best suits your needs.