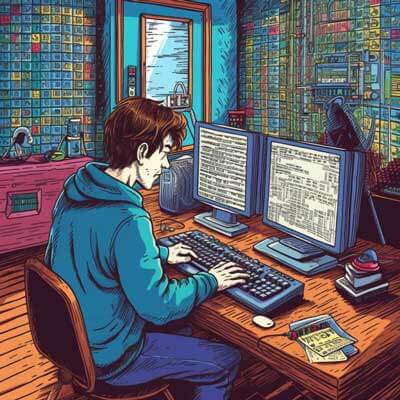
- Date Comparison in PostgreSQL
- Example 1
- Example 2
- Date Functions in PostgreSQL
- Example 1
- Example 2
- Date Format in PostgreSQL
- Example 1
- Example 2
- Date Arithmetic in PostgreSQL
- Example 1
- Example 2
- Date Comparison Operators in PostgreSQL
- Example 1
- Example 2
- Date/Time Data Types in PostgreSQL
- Example 1
- Example 2
- Timestamp Comparison in PostgreSQL
- Example 1
- Example 2
- Interval Data Type in PostgreSQL
- Example 1
- Example 2
- Extracting Date Parts in PostgreSQL
- Example 1
- Example 2
- Date/Time Functions in PostgreSQL
- Example 1
- Example 2
- Additional Resources
Date Comparison in PostgreSQL
When working with dates in PostgreSQL databases, it is often necessary to compare them to perform various operations and queries. PostgreSQL provides several methods to compare dates, allowing you to determine if one date is greater than, equal to, or less than another date. This section will explore the different ways to compare dates in PostgreSQL.
One way to compare dates in PostgreSQL is by using the comparison operators, such as ,
=
, =
, and . These operators work with the
date
data type and allow you to compare two dates.
Here is an example that demonstrates how to compare dates using the comparison operators:
SELECT * FROM events WHERE event_date > '2022-01-01';
This query selects all events where the event_date
is greater than January 1, 2022.
In addition to the comparison operators, PostgreSQL provides several date functions that can be used to compare dates. These functions include date_part()
, extract()
, and to_char()
, among others. These functions allow you to extract specific parts of a date, such as the year, month, or day, and compare them.
Here is an example that demonstrates how to compare dates using the date_part()
function:
SELECT * FROM events WHERE date_part('year', event_date) = 2022;
This query selects all events where the year of the event_date
is equal to 2022.
Related Article: Extracting the Month from a Date in PostgreSQL
Example 1:
Let’s consider a scenario where we have a table named orders
with a column order_date
of type date
. We want to retrieve all orders that were placed after a specific date.
SELECT * FROM orders WHERE order_date > '2022-01-01';
This query will return all orders where the order_date
is greater than January 1, 2022.
Example 2:
Now, let’s assume we have a table named appointments
with a column appointment_date
of type date
. We want to retrieve all appointments that were scheduled for a specific month.
SELECT * FROM appointments WHERE date_part('month', appointment_date) = 6;
This query will return all appointments where the month of the appointment_date
is equal to June.
Date Functions in PostgreSQL
PostgreSQL provides a wide range of date functions that can be used to manipulate and perform calculations on dates. These functions allow you to extract specific parts of a date, perform date arithmetic, format dates, and much more.
Here are some commonly used date functions in PostgreSQL:
– date_part(field, source)
– Extracts a specific part of a date, such as the year, month, or day.
– extract(field FROM source)
– Extracts a specific part of a date, similar to date_part()
.
– to_char(source, format)
– Formats a date according to the specified format.
– date_trunc(field, source)
– Truncates a date to the specified precision.
– current_date
– Returns the current date.
– current_timestamp
– Returns the current date and time.
– age(end, start)
– Calculates the difference between two dates.
– interval
– Constructs a time interval.
– now()
– Returns the current date and time.
Here are some examples that demonstrate the usage of date functions in PostgreSQL:
SELECT date_part('year', current_date) AS current_year;
This query extracts the current year from the current_date
function.
SELECT to_char(current_date, 'YYYY-MM-DD') AS formatted_date;
This query formats the current_date
using the to_char()
function.
SELECT date_trunc('month', current_date) AS truncated_date;
This query truncates the current_date
to the month precision.
Related Article: Working With PostgreSQL: Extracting Day of Week
Example 1:
Let’s consider a scenario where we have a table named employees
with a column birth_date
of type date
. We want to retrieve the age of each employee.
SELECT first_name, last_name, age(current_date, birth_date) AS age FROM employees;
This query calculates the age of each employee using the age()
function and returns the result in years.
Example 2:
Now, let’s assume we have a table named orders
with a column order_date
of type date
. We want to retrieve the total number of orders placed in the current month.
SELECT count(*) FROM orders WHERE date_trunc('month', order_date) = date_trunc('month', current_date);
This query compares the month and year part of the order_date
and current_date
using the date_trunc()
function and returns the count of orders placed in the current month.
Date Format in PostgreSQL
When working with dates in PostgreSQL, it is important to understand the different date formats that can be used to store and represent dates. PostgreSQL supports various date formats, including ISO-8601, European, and American formats.
The ISO-8601 format is the recommended format for date representation in PostgreSQL. It follows the pattern YYYY-MM-DD
, where YYYY
represents the year, MM
represents the month, and DD
represents the day.
Here are some examples of dates in ISO-8601 format:
– 2022-01-01
– January 1, 2022
– 2022-06-15
– June 15, 2022
– 2023-12-31
– December 31, 2023
In addition to the ISO-8601 format, PostgreSQL also supports other date formats, such as the European format (DD.MM.YYYY
) and the American format (MM/DD/YYYY
). However, it is recommended to use the ISO-8601 format for consistency and interoperability.
When inserting or updating dates in PostgreSQL, it is important to ensure that the date values are in the correct format. PostgreSQL will automatically parse and convert valid date strings into the date
data type.
Related Article: Methods to Add Dates in PostgreSQL Databases
Example 1:
Let’s consider a scenario where we have a table named events
with a column event_date
of type date
. We want to insert a new event with the date “June 15, 2022”.
INSERT INTO events (event_date) VALUES ('2022-06-15');
This query inserts a new event with the date “June 15, 2022” into the events
table. The date value is provided in the ISO-8601 format.
Example 2:
Now, let’s assume we have a table named tasks
with a column due_date
of type date
. We want to update the due date of a task to “December 31, 2023”.
UPDATE tasks SET due_date = '2023-12-31' WHERE id = 1;
This query updates the due date of the task with id 1 to “December 31, 2023” in the tasks
table. The date value is provided in the ISO-8601 format.
Date Arithmetic in PostgreSQL
PostgreSQL provides various operators and functions that can be used to perform date arithmetic, allowing you to add or subtract intervals from dates, calculate the difference between two dates, and more. Date arithmetic is useful when working with dates to perform calculations or manipulate dates.
One way to perform date arithmetic in PostgreSQL is by using the +
and -
operators. These operators allow you to add or subtract intervals from dates.
Here is an example that demonstrates how to perform date arithmetic using the +
and -
operators:
SELECT current_date + INTERVAL '1 day' AS tomorrow;
This query adds one day to the current_date
using the +
operator and returns the result as tomorrow
.
In addition to the operators, PostgreSQL provides several date functions that can be used for date arithmetic. These functions include date_part()
, extract()
, date_trunc()
, date_add()
, and date_sub()
, among others. These functions allow you to perform various calculations and manipulations on dates.
Here is an example that demonstrates how to perform date arithmetic using the date_part()
function:
SELECT date_part('year', current_date) + 1 AS next_year;
This query adds one to the year part of the current_date
using the date_part()
function and returns the result as next_year
.
Related Article: How to Set Timestamps With & Without Time Zone in PostgreSQL
Example 1:
Let’s consider a scenario where we have a table named tasks
with a column due_date
of type date
. We want to retrieve all tasks that are due within the next week.
SELECT * FROM tasks WHERE due_date <= current_date + INTERVAL '7 days';
This query adds seven days to the current_date
using the +
operator and selects all tasks where the due date is less than or equal to the calculated date.
Example 2:
Now, let’s assume we have a table named events
with a column event_date
of type date
. We want to calculate the number of days between the event date and the current date.
SELECT event_date - current_date AS days_until_event FROM events;
This query subtracts the current_date
from the event_date
using the -
operator and returns the result as days_until_event
.
Date Comparison Operators in PostgreSQL
PostgreSQL provides a set of comparison operators that can be used to compare dates. These operators allow you to determine if one date is greater than, equal to, or less than another date. The comparison operators work with the date
data type and can be used in various contexts, such as in WHERE
clauses or in ORDER BY
clauses.
Here are the date comparison operators in PostgreSQL:
– – Greater than
– =
– Greater than or equal to
– =
– Equal to
– or
!=
– Not equal to
These operators can be used to compare two dates or to compare a date with a constant value or a subquery result.
Here is an example that demonstrates the usage of date comparison operators:
SELECT * FROM events WHERE event_date > '2022-01-01';
This query selects all events where the event_date
is greater than January 1, 2022.
Example 1:
Let’s consider a scenario where we have a table named tasks
with a column due_date
of type date
. We want to retrieve all tasks that are overdue.
SELECT * FROM tasks WHERE due_date < current_date;
This query selects all tasks where the due_date
is less than the current_date
.
Example 2:
Now, let’s assume we have a table named appointments
with a column appointment_date
of type date
. We want to retrieve all appointments that are scheduled for today.
SELECT * FROM appointments WHERE appointment_date = current_date;
This query selects all appointments where the appointment_date
is equal to the current_date
.
Date/Time Data Types in PostgreSQL
PostgreSQL provides several data types for working with dates and times. These data types allow you to store and manipulate date and time values in a database. The most commonly used date/time data types in PostgreSQL are date
, time
, timestamp
, interval
, and timestamptz
.
– date
– Represents a date (year, month, and day).
– time
– Represents a time of day (hour, minute, second, and optional fractional seconds).
– timestamp
– Represents a date and time (year, month, day, hour, minute, second, and optional fractional seconds).
– interval
– Represents a time interval.
– timestamptz
– Represents a date and time with time zone.
These data types allow you to store and manipulate date and time values with various levels of precision and timezone awareness.
Here is an example that demonstrates the usage of date/time data types in PostgreSQL:
CREATE TABLE events ( id SERIAL <a href="https://www.squash.io/exploring-sql-join-conditions-the-role-of-primary-keys/">PRIMARY KEY</a>, event_date date, event_time time, event_timestamp timestamp, event_interval interval, event_timestamptz timestamptz );
This query creates a table named events
with columns of different date/time data types.
Example 1:
Let’s consider a scenario where we have a table named tasks
with a column due_date
of type date
. We want to store the due date of each task.
CREATE TABLE tasks ( id SERIAL PRIMARY KEY, due_date date );
This query creates a table named tasks
with a column due_date
of type date
. The date
data type allows us to store and manipulate dates.
Example 2:
Now, let’s assume we have a table named appointments
with a column start_time
of type time
. We want to store the start time of each appointment.
CREATE TABLE appointments ( id SERIAL PRIMARY KEY, start_time time );
This query creates a table named appointments
with a column start_time
of type time
. The time
data type allows us to store and manipulate times of day.
Timestamp Comparison in PostgreSQL
In addition to comparing dates, PostgreSQL also allows you to compare timestamps. A timestamp represents a date and time, including the year, month, day, hour, minute, second, and optional fractional seconds. Timestamps are useful when you need to work with both date and time information in a single value.
To compare timestamps in PostgreSQL, you can use the same comparison operators (,
=
, =
, ) that are used for comparing dates. These operators work with the
timestamp
data type and allow you to compare two timestamps or compare a timestamp with a constant value or a subquery result.
Here is an example that demonstrates how to compare timestamps in PostgreSQL:
SELECT * FROM events WHERE event_timestamp > '2022-01-01 10:00:00';
This query selects all events where the event_timestamp
is greater than January 1, 2022, at 10:00:00.
Example 1:
Let’s consider a scenario where we have a table named tasks
with a column created_at
of type timestamp
. We want to retrieve all tasks that were created after a specific timestamp.
SELECT * FROM tasks WHERE created_at > '2022-01-01 10:00:00';
This query selects all tasks where the created_at
is greater than January 1, 2022, at 10:00:00.
Example 2:
Now, let’s assume we have a table named appointments
with a column start_time
of type timestamp
. We want to retrieve all appointments that are scheduled for a specific date and time.
SELECT * FROM appointments WHERE start_time = '2022-06-15 15:30:00';
This query selects all appointments where the start_time
is equal to June 15, 2022, at 15:30:00.
Interval Data Type in PostgreSQL
The interval
data type in PostgreSQL represents a time interval, such as a duration or a period of time. It allows you to store and manipulate intervals in a database. Intervals can be positive or negative and can represent various units of time, such as seconds, minutes, hours, days, weeks, months, or years.
To work with intervals in PostgreSQL, you can use the interval
keyword followed by a quoted interval value. The interval value can include a number and a unit of time, such as 1 day
, 2 weeks
, or 3 months
.
Here is an example that demonstrates the usage of the interval
data type in PostgreSQL:
SELECT current_timestamp + INTERVAL '1 day' AS tomorrow;
This query adds one day to the current_timestamp
using the +
operator and the interval
data type.
Example 1:
Let’s consider a scenario where we have a table named tasks
with a column duration
of type interval
. We want to store the duration of each task.
CREATE TABLE tasks ( id SERIAL PRIMARY KEY, duration interval );
This query creates a table named tasks
with a column duration
of type interval
. The interval
data type allows us to store and manipulate durations.
Example 2:
Now, let’s assume we have a table named events
with a column event_duration
of type interval
. We want to retrieve all events that have a duration longer than one hour.
SELECT * FROM events WHERE event_duration > INTERVAL '1 hour';
This query selects all events where the event_duration
is greater than one hour.
Extracting Date Parts in PostgreSQL
When working with dates in PostgreSQL, you may need to extract specific parts of a date, such as the year, month, day, hour, minute, or second. PostgreSQL provides several functions that allow you to extract date parts from a date or timestamp.
The date_part()
function is commonly used to extract date parts in PostgreSQL. It returns the specified part of a date or timestamp as a double precision number.
Here is an example that demonstrates how to extract date parts in PostgreSQL:
SELECT date_part('year', current_date) AS current_year;
This query extracts the year part from the current_date
using the date_part()
function and returns the result as current_year
.
In addition to the date_part()
function, PostgreSQL also provides the extract()
function that can be used to extract date parts. The extract()
function is similar to the date_part()
function and returns the specified part of a date or timestamp.
Here is an example that demonstrates how to extract date parts using the extract()
function:
SELECT extract(year FROM current_date) AS current_year;
This query extracts the year part from the current_date
using the extract()
function and returns the result as current_year
.
Example 1:
Let’s consider a scenario where we have a table named events
with a column event_date
of type date
. We want to retrieve the year and month of each event.
SELECT date_part('year', event_date) AS event_year, date_part('month', event_date) AS event_month FROM events;
This query extracts the year and month parts from the event_date
using the date_part()
function and returns the result as event_year
and event_month
.
Example 2:
Now, let’s assume we have a table named appointments
with a column start_time
of type timestamp
. We want to retrieve the hour and minute of each appointment.
SELECT extract(hour FROM start_time) AS appointment_hour, extract(minute FROM start_time) AS appointment_minute FROM appointments;
This query extracts the hour and minute parts from the start_time
using the extract()
function and returns the result as appointment_hour
and appointment_minute
.
Date/Time Functions in PostgreSQL
PostgreSQL provides a rich set of date/time functions that can be used to perform various operations and calculations on dates and times. These functions allow you to manipulate dates, perform date arithmetic, format dates, extract date parts, and much more.
Here are some commonly used date/time functions in PostgreSQL:
– current_date()
– Returns the current date.
– current_time()
– Returns the current time.
– current_timestamp()
– Returns the current date and time.
– date_trunc(field, source)
– Truncates a date or timestamp to the specified precision.
– date_part(field, source)
– Extracts the specified part of a date or timestamp.
– extract(field FROM source)
– Extracts the specified part of a date or timestamp.
– to_char(source, format)
– Formats a date or timestamp according to the specified format.
– age(end, start)
– Calculates the difference between two dates or timestamps.
– interval
– Constructs a time interval.
– now()
– Returns the current date and time.
These functions can be used to perform various operations on dates and times, such as calculating the difference between two dates, formatting dates, extracting specific parts of dates, and more.
Here is an example that demonstrates the usage of date/time functions in PostgreSQL:
SELECT current_date() AS current_date, current_time() AS current_time, current_timestamp() AS current_timestamp;
This query uses the current_date()
, current_time()
, and current_timestamp()
functions to retrieve the current date, time, and timestamp.
Example 1:
Let’s consider a scenario where we have a table named events
with a column event_date
of type date
. We want to calculate the number of days between each event date and the current date.
SELECT event_date, age(current_date, event_date) AS days_until_event FROM events;
This query uses the age()
function to calculate the difference between the current_date
and the event_date
for each event.
Example 2:
Now, let’s assume we have a table named appointments
with a column start_time
of type timestamp
. We want to format the start_time
column to display only the date and time in a specific format.
SELECT to_char(start_time, 'YYYY-MM-DD HH:MI:SS') AS formatted_start_time FROM appointments;
This query uses the to_char()
function to format the start_time
column according to the specified format.
Additional Resources
– PostgreSQL Date/Time Functions and Operators
– PostgreSQL Date/Time Functions